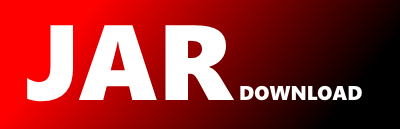
com.pulumi.gcp.cloudids.kotlin.EndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudids.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudids.EndpointArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Cloud IDS is an intrusion detection service that provides threat detection for intrusions, malware, spyware, and command-and-control attacks on your network.
* To get more information about Endpoint, see:
* * [API documentation](https://cloud.google.com/intrusion-detection-system/docs/configuring-ids)
* ## Example Usage
* ### Cloudids Endpoint
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {name: "tf-test-my-network"});
* const serviceRange = new gcp.compute.GlobalAddress("service_range", {
* name: "address",
* purpose: "VPC_PEERING",
* addressType: "INTERNAL",
* prefixLength: 16,
* network: _default.id,
* });
* const privateServiceConnection = new gcp.servicenetworking.Connection("private_service_connection", {
* network: _default.id,
* service: "servicenetworking.googleapis.com",
* reservedPeeringRanges: [serviceRange.name],
* });
* const example_endpoint = new gcp.cloudids.Endpoint("example-endpoint", {
* name: "test",
* location: "us-central1-f",
* network: _default.id,
* severity: "INFORMATIONAL",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default", name="tf-test-my-network")
* service_range = gcp.compute.GlobalAddress("service_range",
* name="address",
* purpose="VPC_PEERING",
* address_type="INTERNAL",
* prefix_length=16,
* network=default.id)
* private_service_connection = gcp.servicenetworking.Connection("private_service_connection",
* network=default.id,
* service="servicenetworking.googleapis.com",
* reserved_peering_ranges=[service_range.name])
* example_endpoint = gcp.cloudids.Endpoint("example-endpoint",
* name="test",
* location="us-central1-f",
* network=default.id,
* severity="INFORMATIONAL")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "tf-test-my-network",
* });
* var serviceRange = new Gcp.Compute.GlobalAddress("service_range", new()
* {
* Name = "address",
* Purpose = "VPC_PEERING",
* AddressType = "INTERNAL",
* PrefixLength = 16,
* Network = @default.Id,
* });
* var privateServiceConnection = new Gcp.ServiceNetworking.Connection("private_service_connection", new()
* {
* Network = @default.Id,
* Service = "servicenetworking.googleapis.com",
* ReservedPeeringRanges = new[]
* {
* serviceRange.Name,
* },
* });
* var example_endpoint = new Gcp.CloudIds.Endpoint("example-endpoint", new()
* {
* Name = "test",
* Location = "us-central1-f",
* Network = @default.Id,
* Severity = "INFORMATIONAL",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudids"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/servicenetworking"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("tf-test-my-network"),
* })
* if err != nil {
* return err
* }
* serviceRange, err := compute.NewGlobalAddress(ctx, "service_range", &compute.GlobalAddressArgs{
* Name: pulumi.String("address"),
* Purpose: pulumi.String("VPC_PEERING"),
* AddressType: pulumi.String("INTERNAL"),
* PrefixLength: pulumi.Int(16),
* Network: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = servicenetworking.NewConnection(ctx, "private_service_connection", &servicenetworking.ConnectionArgs{
* Network: _default.ID(),
* Service: pulumi.String("servicenetworking.googleapis.com"),
* ReservedPeeringRanges: pulumi.StringArray{
* serviceRange.Name,
* },
* })
* if err != nil {
* return err
* }
* _, err = cloudids.NewEndpoint(ctx, "example-endpoint", &cloudids.EndpointArgs{
* Name: pulumi.String("test"),
* Location: pulumi.String("us-central1-f"),
* Network: _default.ID(),
* Severity: pulumi.String("INFORMATIONAL"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.GlobalAddress;
* import com.pulumi.gcp.compute.GlobalAddressArgs;
* import com.pulumi.gcp.servicenetworking.Connection;
* import com.pulumi.gcp.servicenetworking.ConnectionArgs;
* import com.pulumi.gcp.cloudids.Endpoint;
* import com.pulumi.gcp.cloudids.EndpointArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("tf-test-my-network")
* .build());
* var serviceRange = new GlobalAddress("serviceRange", GlobalAddressArgs.builder()
* .name("address")
* .purpose("VPC_PEERING")
* .addressType("INTERNAL")
* .prefixLength(16)
* .network(default_.id())
* .build());
* var privateServiceConnection = new Connection("privateServiceConnection", ConnectionArgs.builder()
* .network(default_.id())
* .service("servicenetworking.googleapis.com")
* .reservedPeeringRanges(serviceRange.name())
* .build());
* var example_endpoint = new Endpoint("example-endpoint", EndpointArgs.builder()
* .name("test")
* .location("us-central1-f")
* .network(default_.id())
* .severity("INFORMATIONAL")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:Network
* properties:
* name: tf-test-my-network
* serviceRange:
* type: gcp:compute:GlobalAddress
* name: service_range
* properties:
* name: address
* purpose: VPC_PEERING
* addressType: INTERNAL
* prefixLength: 16
* network: ${default.id}
* privateServiceConnection:
* type: gcp:servicenetworking:Connection
* name: private_service_connection
* properties:
* network: ${default.id}
* service: servicenetworking.googleapis.com
* reservedPeeringRanges:
* - ${serviceRange.name}
* example-endpoint:
* type: gcp:cloudids:Endpoint
* properties:
* name: test
* location: us-central1-f
* network: ${default.id}
* severity: INFORMATIONAL
* ```
*
* ## Import
* Endpoint can be imported using any of these accepted formats:
* * `projects/{{project}}/locations/{{location}}/endpoints/{{name}}`
* * `{{project}}/{{location}}/{{name}}`
* * `{{location}}/{{name}}`
* When using the `pulumi import` command, Endpoint can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:cloudids/endpoint:Endpoint default projects/{{project}}/locations/{{location}}/endpoints/{{name}}
* ```
* ```sh
* $ pulumi import gcp:cloudids/endpoint:Endpoint default {{project}}/{{location}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:cloudids/endpoint:Endpoint default {{location}}/{{name}}
* ```
* @property description An optional description of the endpoint.
* @property location The location for the endpoint.
* - - -
* @property name Name of the endpoint in the format projects/{project_id}/locations/{locationId}/endpoints/{endpointId}.
* @property network Name of the VPC network that is connected to the IDS endpoint. This can either contain the VPC network name itself (like "src-net") or the full URL to the network (like "projects/{project_id}/global/networks/src-net").
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property severity The minimum alert severity level that is reported by the endpoint.
* Possible values are: `INFORMATIONAL`, `LOW`, `MEDIUM`, `HIGH`, `CRITICAL`.
* @property threatExceptions Configuration for threat IDs excluded from generating alerts. Limit: 99 IDs.
*/
public data class EndpointArgs(
public val description: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val network: Output? = null,
public val project: Output? = null,
public val severity: Output? = null,
public val threatExceptions: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudids.EndpointArgs =
com.pulumi.gcp.cloudids.EndpointArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.location(location?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.network(network?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.severity(severity?.applyValue({ args0 -> args0 }))
.threatExceptions(threatExceptions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [EndpointArgs].
*/
@PulumiTagMarker
public class EndpointArgsBuilder internal constructor() {
private var description: Output? = null
private var location: Output? = null
private var name: Output? = null
private var network: Output? = null
private var project: Output? = null
private var severity: Output? = null
private var threatExceptions: Output>? = null
/**
* @param value An optional description of the endpoint.
*/
@JvmName("lbsyicgraokvuxbn")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The location for the endpoint.
* - - -
*/
@JvmName("pnceojsckevwkfmf")
public suspend fun location(`value`: Output) {
this.location = value
}
/**
* @param value Name of the endpoint in the format projects/{project_id}/locations/{locationId}/endpoints/{endpointId}.
*/
@JvmName("iwmostxyqjlabrem")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Name of the VPC network that is connected to the IDS endpoint. This can either contain the VPC network name itself (like "src-net") or the full URL to the network (like "projects/{project_id}/global/networks/src-net").
*/
@JvmName("rncihtffgqngfpjs")
public suspend fun network(`value`: Output) {
this.network = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("xcvycfowseglhhym")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The minimum alert severity level that is reported by the endpoint.
* Possible values are: `INFORMATIONAL`, `LOW`, `MEDIUM`, `HIGH`, `CRITICAL`.
*/
@JvmName("ydfowsysxfagqcny")
public suspend fun severity(`value`: Output) {
this.severity = value
}
/**
* @param value Configuration for threat IDs excluded from generating alerts. Limit: 99 IDs.
*/
@JvmName("jldljdbxkdvlrfrl")
public suspend fun threatExceptions(`value`: Output>) {
this.threatExceptions = value
}
@JvmName("pbaaejoyfvaoqwok")
public suspend fun threatExceptions(vararg values: Output) {
this.threatExceptions = Output.all(values.asList())
}
/**
* @param values Configuration for threat IDs excluded from generating alerts. Limit: 99 IDs.
*/
@JvmName("sdwkitgxffmsnajo")
public suspend fun threatExceptions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy