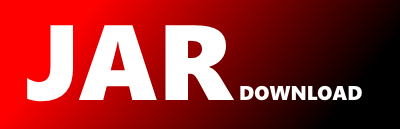
com.pulumi.gcp.cloudquota.kotlin.SQuotaPreference.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudquota.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.cloudquota.kotlin.outputs.SQuotaPreferenceQuotaConfig
import com.pulumi.gcp.cloudquota.kotlin.outputs.SQuotaPreferenceQuotaConfig.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [SQuotaPreference].
*/
@PulumiTagMarker
public class SQuotaPreferenceResourceBuilder internal constructor() {
public var name: String? = null
public var args: SQuotaPreferenceArgs = SQuotaPreferenceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SQuotaPreferenceArgsBuilder.() -> Unit) {
val builder = SQuotaPreferenceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SQuotaPreference {
val builtJavaResource = com.pulumi.gcp.cloudquota.SQuotaPreference(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SQuotaPreference(builtJavaResource)
}
}
/**
* QuotaPreference represents the preferred quota configuration specified for a project, folder or organization. There is only one QuotaPreference resource for a quota value targeting a unique set of dimensions.
* To get more information about QuotaPreference, see:
* * [API documentation](https://cloud.google.com/docs/quotas/reference/rest/v1/projects.locations.quotaPreferences)
* * How-to Guides
* * [Cloud Quotas Overview](https://cloud.google.com/docs/quotas/overview)
* ## Example Usage
* ### Cloudquotas Quota Preference Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const preference = new gcp.cloudquota.SQuotaPreference("preference", {
* parent: "projects/my-project-name",
* name: "compute_googleapis_com-CPUS-per-project_us-east1",
* dimensions: {
* region: "us-east1",
* },
* service: "compute.googleapis.com",
* quotaId: "CPUS-per-project-region",
* contactEmail: "[email protected]",
* quotaConfig: {
* preferredValue: "200",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* preference = gcp.cloudquota.SQuotaPreference("preference",
* parent="projects/my-project-name",
* name="compute_googleapis_com-CPUS-per-project_us-east1",
* dimensions={
* "region": "us-east1",
* },
* service="compute.googleapis.com",
* quota_id="CPUS-per-project-region",
* contact_email="[email protected]",
* quota_config=gcp.cloudquota.SQuotaPreferenceQuotaConfigArgs(
* preferred_value="200",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var preference = new Gcp.CloudQuota.SQuotaPreference("preference", new()
* {
* Parent = "projects/my-project-name",
* Name = "compute_googleapis_com-CPUS-per-project_us-east1",
* Dimensions =
* {
* { "region", "us-east1" },
* },
* Service = "compute.googleapis.com",
* QuotaId = "CPUS-per-project-region",
* ContactEmail = "[email protected]",
* QuotaConfig = new Gcp.CloudQuota.Inputs.SQuotaPreferenceQuotaConfigArgs
* {
* PreferredValue = "200",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/cloudquota"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := cloudquota.NewSQuotaPreference(ctx, "preference", &cloudquota.SQuotaPreferenceArgs{
* Parent: pulumi.String("projects/my-project-name"),
* Name: pulumi.String("compute_googleapis_com-CPUS-per-project_us-east1"),
* Dimensions: pulumi.StringMap{
* "region": pulumi.String("us-east1"),
* },
* Service: pulumi.String("compute.googleapis.com"),
* QuotaId: pulumi.String("CPUS-per-project-region"),
* ContactEmail: pulumi.String("[email protected]"),
* QuotaConfig: &cloudquota.SQuotaPreferenceQuotaConfigArgs{
* PreferredValue: pulumi.String("200"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.cloudquota.SQuotaPreference;
* import com.pulumi.gcp.cloudquota.SQuotaPreferenceArgs;
* import com.pulumi.gcp.cloudquota.inputs.SQuotaPreferenceQuotaConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var preference = new SQuotaPreference("preference", SQuotaPreferenceArgs.builder()
* .parent("projects/my-project-name")
* .name("compute_googleapis_com-CPUS-per-project_us-east1")
* .dimensions(Map.of("region", "us-east1"))
* .service("compute.googleapis.com")
* .quotaId("CPUS-per-project-region")
* .contactEmail("[email protected]")
* .quotaConfig(SQuotaPreferenceQuotaConfigArgs.builder()
* .preferredValue(200)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* preference:
* type: gcp:cloudquota:SQuotaPreference
* properties:
* parent: projects/my-project-name
* name: compute_googleapis_com-CPUS-per-project_us-east1
* dimensions:
* region: us-east1
* service: compute.googleapis.com
* quotaId: CPUS-per-project-region
* contactEmail: [email protected]
* quotaConfig:
* preferredValue: 200
* ```
*
* ## Import
* QuotaPreference can be imported using any of these accepted formats:
* * `{{parent}}/locations/global/quotaPreferences/{{name}}`
* When using the `pulumi import` command, QuotaPreference can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:cloudquota/sQuotaPreference:SQuotaPreference default {{parent}}/locations/global/quotaPreferences/{{name}}
* ```
*/
public class SQuotaPreference internal constructor(
override val javaResource: com.pulumi.gcp.cloudquota.SQuotaPreference,
) : KotlinCustomResource(javaResource, SQuotaPreferenceMapper) {
/**
* An email address that can be used for quota related communication between the Google Cloud and the user in case the
* Google Cloud needs further information to make a decision on whether the user preferred quota can be granted. The Google
* account for the email address must have quota update permission for the project, folder or organization this quota
* preference is for.
*/
public val contactEmail: Output?
get() = javaResource.contactEmail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Create time stamp.
* A timestamp in RFC3339 UTC "Zulu" format, with nanosecond resolution and up to nine fractional digits. Examples: `2014-10-02T15:01:23Z` and `2014-10-02T15:01:23.045123456Z`.
*/
public val createTime: Output
get() = javaResource.createTime().applyValue({ args0 -> args0 })
/**
* The dimensions that this quota preference applies to. The key of the map entry is the name of a dimension, such as
* "region", "zone", "network_id", and the value of the map entry is the dimension value. If a dimension is missing from
* the map of dimensions, the quota preference applies to all the dimension values except for those that have other quota
* preferences configured for the specific value. NOTE: QuotaPreferences can only be applied across all values of "user"
* and "resource" dimension. Do not set values for "user" or "resource" in the dimension map. Example: '{"provider": "Foo
* Inc"}' where "provider" is a service specific dimension.
*/
public val dimensions: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy