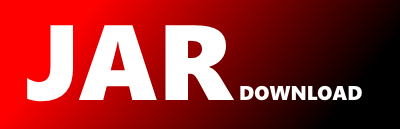
com.pulumi.gcp.cloudquota.kotlin.outputs.GetSQuotaInfoResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudquota.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getSQuotaInfo.
* @property containerType (Output) The container type of the QuotaInfo.
* @property dimensions The map of dimensions for this dimensions info. The key of a map entry is "region", "zone" or the name of a service specific dimension, and the value of a map entry is the value of the dimension. If a dimension does not appear in the map of dimensions, the dimensions info applies to all the dimension values except for those that have another DimenisonInfo instance configured for the specific value. Example: {"provider" : "Foo Inc"} where "provider" is a service specific dimension of a quota.
* @property dimensionsInfos (Output) The collection of dimensions info ordered by their dimensions from more specific ones to less specific ones.
* @property id The provider-assigned unique ID for this managed resource.
* @property isConcurrent (Output) Whether the quota is a concurrent quota. Concurrent quotas are enforced on the total number of concurrent operations in flight at any given time.
* @property isFixed (Output) Whether the quota value is fixed or adjustable.
* @property isPrecise (Output) Whether this is a precise quota. A precise quota is tracked with absolute precision. In contrast, an imprecise quota is not tracked with precision.
* @property metric (Output) The metric of the quota. It specifies the resources consumption the quota is defined for, for example: `compute.googleapis.com/cpus`.
* @property metricDisplayName (Output) The display name of the quota metric.
* @property metricUnit (Output) The unit in which the metric value is reported, e.g., `MByte`.
* @property name (Output) Resource name of this QuotaInfo, for example: `projects/123/locations/global/services/compute.googleapis.com/quotaInfos/CpusPerProjectPerRegion`.
* @property parent
* @property quotaDisplayName (Output) The display name of the quota.
* @property quotaId
* @property quotaIncreaseEligibilities (Output) Whether it is eligible to request a higher quota value for this quota.
* @property refreshInterval (Output) The reset time interval for the quota. Refresh interval applies to rate quota only. Example: "minute" for per minute, "day" for per day, or "10 seconds" for every 10 seconds.
* @property service
* @property serviceRequestQuotaUri (Output) URI to the page where users can request more quota for the cloud service, for example: `https://console.cloud.google.com/iam-admin/quotas`.
*/
public data class GetSQuotaInfoResult(
public val containerType: String,
public val dimensions: List,
public val dimensionsInfos: List,
public val id: String,
public val isConcurrent: Boolean,
public val isFixed: Boolean,
public val isPrecise: Boolean,
public val metric: String,
public val metricDisplayName: String,
public val metricUnit: String,
public val name: String,
public val parent: String,
public val quotaDisplayName: String,
public val quotaId: String,
public val quotaIncreaseEligibilities: List,
public val refreshInterval: String,
public val service: String,
public val serviceRequestQuotaUri: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudquota.outputs.GetSQuotaInfoResult): GetSQuotaInfoResult = GetSQuotaInfoResult(
containerType = javaType.containerType(),
dimensions = javaType.dimensions().map({ args0 -> args0 }),
dimensionsInfos = javaType.dimensionsInfos().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudquota.kotlin.outputs.GetSQuotaInfoDimensionsInfo.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
isConcurrent = javaType.isConcurrent(),
isFixed = javaType.isFixed(),
isPrecise = javaType.isPrecise(),
metric = javaType.metric(),
metricDisplayName = javaType.metricDisplayName(),
metricUnit = javaType.metricUnit(),
name = javaType.name(),
parent = javaType.parent(),
quotaDisplayName = javaType.quotaDisplayName(),
quotaId = javaType.quotaId(),
quotaIncreaseEligibilities = javaType.quotaIncreaseEligibilities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudquota.kotlin.outputs.GetSQuotaInfoQuotaIncreaseEligibility.Companion.toKotlin(args0)
})
}),
refreshInterval = javaType.refreshInterval(),
service = javaType.service(),
serviceRequestQuotaUri = javaType.serviceRequestQuotaUri(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy