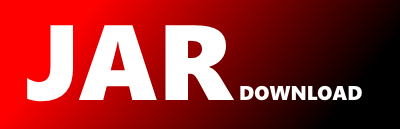
com.pulumi.gcp.cloudrun.kotlin.inputs.ServiceTemplateSpecContainerLivenessProbeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrun.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecContainerLivenessProbeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property failureThreshold Minimum consecutive failures for the probe to be considered failed after
* having succeeded. Defaults to 3. Minimum value is 1.
* @property grpc GRPC specifies an action involving a GRPC port.
* Structure is documented below.
* @property httpGet HttpGet specifies the http request to perform.
* Structure is documented below.
* @property initialDelaySeconds Number of seconds after the container has started before the probe is
* initiated.
* Defaults to 0 seconds. Minimum value is 0. Maximum value is 3600.
* @property periodSeconds How often (in seconds) to perform the probe.
* Default to 10 seconds. Minimum value is 1. Maximum value is 3600.
* @property timeoutSeconds Number of seconds after which the probe times out.
* Defaults to 1 second. Minimum value is 1. Maximum value is 3600.
* Must be smaller than period_seconds.
*/
public data class ServiceTemplateSpecContainerLivenessProbeArgs(
public val failureThreshold: Output? = null,
public val grpc: Output? = null,
public val httpGet: Output? = null,
public val initialDelaySeconds: Output? = null,
public val periodSeconds: Output? = null,
public val timeoutSeconds: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecContainerLivenessProbeArgs =
com.pulumi.gcp.cloudrun.inputs.ServiceTemplateSpecContainerLivenessProbeArgs.builder()
.failureThreshold(failureThreshold?.applyValue({ args0 -> args0 }))
.grpc(grpc?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpGet(httpGet?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.initialDelaySeconds(initialDelaySeconds?.applyValue({ args0 -> args0 }))
.periodSeconds(periodSeconds?.applyValue({ args0 -> args0 }))
.timeoutSeconds(timeoutSeconds?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceTemplateSpecContainerLivenessProbeArgs].
*/
@PulumiTagMarker
public class ServiceTemplateSpecContainerLivenessProbeArgsBuilder internal constructor() {
private var failureThreshold: Output? = null
private var grpc: Output? = null
private var httpGet: Output? = null
private var initialDelaySeconds: Output? = null
private var periodSeconds: Output? = null
private var timeoutSeconds: Output? = null
/**
* @param value Minimum consecutive failures for the probe to be considered failed after
* having succeeded. Defaults to 3. Minimum value is 1.
*/
@JvmName("balbgqgtwqvdnqyk")
public suspend fun failureThreshold(`value`: Output) {
this.failureThreshold = value
}
/**
* @param value GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("bqekgsmixevwwxhx")
public suspend fun grpc(`value`: Output) {
this.grpc = value
}
/**
* @param value HttpGet specifies the http request to perform.
* Structure is documented below.
*/
@JvmName("wjdrepgjmfacqssr")
public suspend fun httpGet(`value`: Output) {
this.httpGet = value
}
/**
* @param value Number of seconds after the container has started before the probe is
* initiated.
* Defaults to 0 seconds. Minimum value is 0. Maximum value is 3600.
*/
@JvmName("phslwgojvtuqgvdg")
public suspend fun initialDelaySeconds(`value`: Output) {
this.initialDelaySeconds = value
}
/**
* @param value How often (in seconds) to perform the probe.
* Default to 10 seconds. Minimum value is 1. Maximum value is 3600.
*/
@JvmName("gqflicqcotfixuvh")
public suspend fun periodSeconds(`value`: Output) {
this.periodSeconds = value
}
/**
* @param value Number of seconds after which the probe times out.
* Defaults to 1 second. Minimum value is 1. Maximum value is 3600.
* Must be smaller than period_seconds.
*/
@JvmName("skqqacedbtvehshc")
public suspend fun timeoutSeconds(`value`: Output) {
this.timeoutSeconds = value
}
/**
* @param value Minimum consecutive failures for the probe to be considered failed after
* having succeeded. Defaults to 3. Minimum value is 1.
*/
@JvmName("edickhugxcylntoo")
public suspend fun failureThreshold(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureThreshold = mapped
}
/**
* @param value GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("ogrgjrxaicrcvhcy")
public suspend fun grpc(`value`: ServiceTemplateSpecContainerLivenessProbeGrpcArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.grpc = mapped
}
/**
* @param argument GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("piwkxhiultcowxce")
public suspend fun grpc(argument: suspend ServiceTemplateSpecContainerLivenessProbeGrpcArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecContainerLivenessProbeGrpcArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.grpc = mapped
}
/**
* @param value HttpGet specifies the http request to perform.
* Structure is documented below.
*/
@JvmName("ewgfcsoubjlcidje")
public suspend fun httpGet(`value`: ServiceTemplateSpecContainerLivenessProbeHttpGetArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpGet = mapped
}
/**
* @param argument HttpGet specifies the http request to perform.
* Structure is documented below.
*/
@JvmName("najnpnnkyuqlwkac")
public suspend fun httpGet(argument: suspend ServiceTemplateSpecContainerLivenessProbeHttpGetArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateSpecContainerLivenessProbeHttpGetArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpGet = mapped
}
/**
* @param value Number of seconds after the container has started before the probe is
* initiated.
* Defaults to 0 seconds. Minimum value is 0. Maximum value is 3600.
*/
@JvmName("rfhyhetoibgweeid")
public suspend fun initialDelaySeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.initialDelaySeconds = mapped
}
/**
* @param value How often (in seconds) to perform the probe.
* Default to 10 seconds. Minimum value is 1. Maximum value is 3600.
*/
@JvmName("hgtfgjqmrnomhrgl")
public suspend fun periodSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.periodSeconds = mapped
}
/**
* @param value Number of seconds after which the probe times out.
* Defaults to 1 second. Minimum value is 1. Maximum value is 3600.
* Must be smaller than period_seconds.
*/
@JvmName("ybngjaijgvrbeisy")
public suspend fun timeoutSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutSeconds = mapped
}
internal fun build(): ServiceTemplateSpecContainerLivenessProbeArgs =
ServiceTemplateSpecContainerLivenessProbeArgs(
failureThreshold = failureThreshold,
grpc = grpc,
httpGet = httpGet,
initialDelaySeconds = initialDelaySeconds,
periodSeconds = periodSeconds,
timeoutSeconds = timeoutSeconds,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy