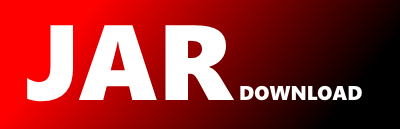
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateMetadata.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrun.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
/**
*
* @property annotations Annotations is a key value map stored with a resource that
* may be set by external tools to store and retrieve arbitrary metadata. More
* info: https://kubernetes.io/docs/concepts/overview/working-with-objects/annotations
* **Note**: The Cloud Run API may add additional annotations that were not provided in your config.
* If the provider plan shows a diff where a server-side annotation is added, you can add it to your config
* or apply the lifecycle.ignore_changes rule to the metadata.0.annotations field.
* Annotations with `run.googleapis.com/` and `autoscaling.knative.dev` are restricted. Use the following annotation
* keys to configure features on a Service:
* - `run.googleapis.com/binary-authorization-breakglass` sets the [Binary Authorization breakglass](https://cloud.google.com/sdk/gcloud/reference/run/deploy#--breakglass).
* - `run.googleapis.com/binary-authorization` sets the [Binary Authorization](https://cloud.google.com/sdk/gcloud/reference/run/deploy#--binary-authorization).
* - `run.googleapis.com/client-name` sets the client name calling the Cloud Run API.
* - `run.googleapis.com/custom-audiences` sets the [custom audiences](https://cloud.google.com/sdk/gcloud/reference/alpha/run/deploy#--add-custom-audiences)
* that can be used in the audience field of ID token for authenticated requests.
* - `run.googleapis.com/description` sets a user defined description for the Service.
* - `run.googleapis.com/ingress` sets the [ingress settings](https://cloud.google.com/sdk/gcloud/reference/run/deploy#--ingress)
* for the Service. For example, `"run.googleapis.com/ingress" = "all"`.
* - `run.googleapis.com/launch-stage` sets the [launch stage](https://cloud.google.com/run/docs/troubleshooting#launch-stage-validation)
* when a preview feature is used. For example, `"run.googleapis.com/launch-stage": "BETA"`
* **Note**: This field is non-authoritative, and will only manage the annotations present in your configuration.
* Please refer to the field `effective_annotations` for all of the annotations present on the resource.
* @property generation (Output)
* A sequence number representing a specific generation of the desired state.
* @property labels Map of string keys and values that can be used to organize and categorize
* (scope and select) objects. May match selectors of replication controllers
* and routes.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property name Name must be unique within a Google Cloud project and region.
* Is required when creating resources. Name is primarily intended
* for creation idempotence and configuration definition. Cannot be updated.
* @property namespace In Cloud Run the namespace must be equal to either the
* project ID or project number.
* @property resourceVersion (Output)
* An opaque value that represents the internal version of this object that
* can be used by clients to determine when objects have changed. May be used
* for optimistic concurrency, change detection, and the watch operation on a
* resource or set of resources. They may only be valid for a
* particular resource or set of resources.
* @property selfLink (Output)
* SelfLink is a URL representing this object.
* @property uid (Output)
* UID is a unique id generated by the server on successful creation of a resource and is not
* allowed to change on PUT operations.
*/
public data class ServiceTemplateMetadata(
public val annotations: Map? = null,
public val generation: Int? = null,
public val labels: Map? = null,
public val name: String? = null,
public val namespace: String? = null,
public val resourceVersion: String? = null,
public val selfLink: String? = null,
public val uid: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudrun.outputs.ServiceTemplateMetadata): ServiceTemplateMetadata = ServiceTemplateMetadata(
annotations = javaType.annotations().map({ args0 -> args0.key.to(args0.value) }).toMap(),
generation = javaType.generation().map({ args0 -> args0 }).orElse(null),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
namespace = javaType.namespace().map({ args0 -> args0 }).orElse(null),
resourceVersion = javaType.resourceVersion().map({ args0 -> args0 }).orElse(null),
selfLink = javaType.selfLink().map({ args0 -> args0 }).orElse(null),
uid = javaType.uid().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy