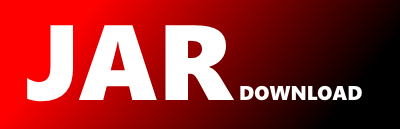
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainer.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrun.kotlin.outputs
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property args Arguments to the entrypoint.
* The docker image's CMD is used if this is not provided.
* @property commands Entrypoint array. Not executed within a shell.
* The docker image's ENTRYPOINT is used if this is not provided.
* @property envFroms (Optional, Deprecated)
* List of sources to populate environment variables in the container.
* All invalid keys will be reported as an event when the container is starting.
* When a key exists in multiple sources, the value associated with the last source will
* take precedence. Values defined by an Env with a duplicate key will take
* precedence.
* Structure is documented below.
* > **Warning:** `env_from` is deprecated and will be removed in a future major release. This field is not supported by the Cloud Run API.
* @property envs List of environment variables to set in the container.
* Structure is documented below.
* @property image Docker image name. This is most often a reference to a container located
* in the container registry, such as gcr.io/cloudrun/hello
* @property livenessProbe Periodic probe of container liveness. Container will be restarted if the probe fails. More info:
* https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
* Structure is documented below.
* @property name Name of the container
* @property ports List of open ports in the container.
* Structure is documented below.
* @property resources Compute Resources required by this container. Used to set values such as max memory
* Structure is documented below.
* @property startupProbe Startup probe of application within the container.
* All other probes are disabled if a startup probe is provided, until it
* succeeds. Container will not be added to service endpoints if the probe fails.
* Structure is documented below.
* @property volumeMounts Volume to mount into the container's filesystem.
* Only supports SecretVolumeSources.
* Structure is documented below.
* @property workingDir (Optional, Deprecated)
* Container's working directory.
* If not specified, the container runtime's default will be used, which
* might be configured in the container image.
* > **Warning:** `working_dir` is deprecated and will be removed in a future major release. This field is not supported by the Cloud Run API.
*/
public data class ServiceTemplateSpecContainer(
public val args: List? = null,
public val commands: List? = null,
@Deprecated(
message = """
`env_from` is deprecated and will be removed in a future major release. This field is not
supported by the Cloud Run API.
""",
)
public val envFroms: List? = null,
public val envs: List? = null,
public val image: String,
public val livenessProbe: ServiceTemplateSpecContainerLivenessProbe? = null,
public val name: String? = null,
public val ports: List? = null,
public val resources: ServiceTemplateSpecContainerResources? = null,
public val startupProbe: ServiceTemplateSpecContainerStartupProbe? = null,
public val volumeMounts: List? = null,
@Deprecated(
message = """
`working_dir` is deprecated and will be removed in a future major release. This field is not
supported by the Cloud Run API.
""",
)
public val workingDir: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudrun.outputs.ServiceTemplateSpecContainer): ServiceTemplateSpecContainer = ServiceTemplateSpecContainer(
args = javaType.args().map({ args0 -> args0 }),
commands = javaType.commands().map({ args0 -> args0 }),
envFroms = javaType.envFroms().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerEnvFrom.Companion.toKotlin(args0)
})
}),
envs = javaType.envs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerEnv.Companion.toKotlin(args0)
})
}),
image = javaType.image(),
livenessProbe = javaType.livenessProbe().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerLivenessProbe.Companion.toKotlin(args0)
})
}).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
ports = javaType.ports().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerPort.Companion.toKotlin(args0)
})
}),
resources = javaType.resources().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerResources.Companion.toKotlin(args0)
})
}).orElse(null),
startupProbe = javaType.startupProbe().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerStartupProbe.Companion.toKotlin(args0)
})
}).orElse(null),
volumeMounts = javaType.volumeMounts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrun.kotlin.outputs.ServiceTemplateSpecContainerVolumeMount.Companion.toKotlin(args0)
})
}),
workingDir = javaType.workingDir().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy