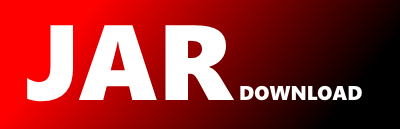
com.pulumi.gcp.cloudrunv2.kotlin.inputs.JobTemplateTemplateVolumeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrunv2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudrunv2.inputs.JobTemplateTemplateVolumeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property cloudSqlInstance For Cloud SQL volumes, contains the specific instances that should be mounted. Visit https://cloud.google.com/sql/docs/mysql/connect-run for more information on how to connect Cloud SQL and Cloud Run.
* Structure is documented below.
* @property emptyDir Ephemeral storage used as a shared volume.
* Structure is documented below.
* @property gcs Cloud Storage bucket mounted as a volume using GCSFuse. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
* @property name Volume's name.
* @property nfs NFS share mounted as a volume. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
* @property secret Secret represents a secret that should populate this volume. More info: https://kubernetes.io/docs/concepts/storage/volumes#secret
* Structure is documented below.
*/
public data class JobTemplateTemplateVolumeArgs(
public val cloudSqlInstance: Output? = null,
public val emptyDir: Output? = null,
public val gcs: Output? = null,
public val name: Output,
public val nfs: Output? = null,
public val secret: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudrunv2.inputs.JobTemplateTemplateVolumeArgs =
com.pulumi.gcp.cloudrunv2.inputs.JobTemplateTemplateVolumeArgs.builder()
.cloudSqlInstance(cloudSqlInstance?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.emptyDir(emptyDir?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.gcs(gcs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.nfs(nfs?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.secret(secret?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [JobTemplateTemplateVolumeArgs].
*/
@PulumiTagMarker
public class JobTemplateTemplateVolumeArgsBuilder internal constructor() {
private var cloudSqlInstance: Output? = null
private var emptyDir: Output? = null
private var gcs: Output? = null
private var name: Output? = null
private var nfs: Output? = null
private var secret: Output? = null
/**
* @param value For Cloud SQL volumes, contains the specific instances that should be mounted. Visit https://cloud.google.com/sql/docs/mysql/connect-run for more information on how to connect Cloud SQL and Cloud Run.
* Structure is documented below.
*/
@JvmName("qjmkahbbbydsjhsx")
public suspend fun cloudSqlInstance(`value`: Output) {
this.cloudSqlInstance = value
}
/**
* @param value Ephemeral storage used as a shared volume.
* Structure is documented below.
*/
@JvmName("bjwraadeynmwlykf")
public suspend fun emptyDir(`value`: Output) {
this.emptyDir = value
}
/**
* @param value Cloud Storage bucket mounted as a volume using GCSFuse. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("wntxckxdwijmwofd")
public suspend fun gcs(`value`: Output) {
this.gcs = value
}
/**
* @param value Volume's name.
*/
@JvmName("ygnbuawnrvoadoje")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value NFS share mounted as a volume. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("tbamggwgeudpwhyi")
public suspend fun nfs(`value`: Output) {
this.nfs = value
}
/**
* @param value Secret represents a secret that should populate this volume. More info: https://kubernetes.io/docs/concepts/storage/volumes#secret
* Structure is documented below.
*/
@JvmName("mllwicedbitquqqk")
public suspend fun secret(`value`: Output) {
this.secret = value
}
/**
* @param value For Cloud SQL volumes, contains the specific instances that should be mounted. Visit https://cloud.google.com/sql/docs/mysql/connect-run for more information on how to connect Cloud SQL and Cloud Run.
* Structure is documented below.
*/
@JvmName("kmwklybmcsuksqlm")
public suspend fun cloudSqlInstance(`value`: JobTemplateTemplateVolumeCloudSqlInstanceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cloudSqlInstance = mapped
}
/**
* @param argument For Cloud SQL volumes, contains the specific instances that should be mounted. Visit https://cloud.google.com/sql/docs/mysql/connect-run for more information on how to connect Cloud SQL and Cloud Run.
* Structure is documented below.
*/
@JvmName("ntocfreryyhnhyaw")
public suspend fun cloudSqlInstance(argument: suspend JobTemplateTemplateVolumeCloudSqlInstanceArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateTemplateVolumeCloudSqlInstanceArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.cloudSqlInstance = mapped
}
/**
* @param value Ephemeral storage used as a shared volume.
* Structure is documented below.
*/
@JvmName("vbxxmbuxnxopuhbq")
public suspend fun emptyDir(`value`: JobTemplateTemplateVolumeEmptyDirArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.emptyDir = mapped
}
/**
* @param argument Ephemeral storage used as a shared volume.
* Structure is documented below.
*/
@JvmName("kvoeimgddfsebuxg")
public suspend fun emptyDir(argument: suspend JobTemplateTemplateVolumeEmptyDirArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateTemplateVolumeEmptyDirArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.emptyDir = mapped
}
/**
* @param value Cloud Storage bucket mounted as a volume using GCSFuse. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("pyvrsuohdistuqug")
public suspend fun gcs(`value`: JobTemplateTemplateVolumeGcsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gcs = mapped
}
/**
* @param argument Cloud Storage bucket mounted as a volume using GCSFuse. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("yjanarqyyynouutc")
public suspend fun gcs(argument: suspend JobTemplateTemplateVolumeGcsArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateTemplateVolumeGcsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.gcs = mapped
}
/**
* @param value Volume's name.
*/
@JvmName("htkdkqqppvurnwep")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value NFS share mounted as a volume. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("cutyjdshlxyydvhs")
public suspend fun nfs(`value`: JobTemplateTemplateVolumeNfsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nfs = mapped
}
/**
* @param argument NFS share mounted as a volume. This feature requires the launch stage to be set to ALPHA or BETA.
* Structure is documented below.
*/
@JvmName("jjwhgxowfxcsmxys")
public suspend fun nfs(argument: suspend JobTemplateTemplateVolumeNfsArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateTemplateVolumeNfsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.nfs = mapped
}
/**
* @param value Secret represents a secret that should populate this volume. More info: https://kubernetes.io/docs/concepts/storage/volumes#secret
* Structure is documented below.
*/
@JvmName("ohblmluckljtymxt")
public suspend fun secret(`value`: JobTemplateTemplateVolumeSecretArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secret = mapped
}
/**
* @param argument Secret represents a secret that should populate this volume. More info: https://kubernetes.io/docs/concepts/storage/volumes#secret
* Structure is documented below.
*/
@JvmName("iuygcdnkawjtmhes")
public suspend fun secret(argument: suspend JobTemplateTemplateVolumeSecretArgsBuilder.() -> Unit) {
val toBeMapped = JobTemplateTemplateVolumeSecretArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.secret = mapped
}
internal fun build(): JobTemplateTemplateVolumeArgs = JobTemplateTemplateVolumeArgs(
cloudSqlInstance = cloudSqlInstance,
emptyDir = emptyDir,
gcs = gcs,
name = name ?: throw PulumiNullFieldException("name"),
nfs = nfs,
secret = secret,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy