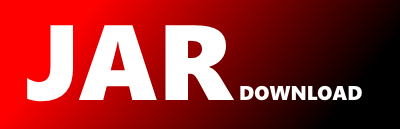
com.pulumi.gcp.cloudrunv2.kotlin.inputs.ServiceTemplateContainerStartupProbeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrunv2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudrunv2.inputs.ServiceTemplateContainerStartupProbeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property failureThreshold Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1.
* @property grpc GRPC specifies an action involving a GRPC port.
* Structure is documented below.
* @property httpGet HTTPGet specifies the http request to perform. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
* @property initialDelaySeconds Number of seconds after the container has started before the probe is initiated. Defaults to 0 seconds. Minimum value is 0. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
* @property periodSeconds How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. Must be greater or equal than timeoutSeconds
* @property tcpSocket TCPSocket specifies an action involving a TCP port. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
* @property timeoutSeconds Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is 3600. Must be smaller than periodSeconds. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
*/
public data class ServiceTemplateContainerStartupProbeArgs(
public val failureThreshold: Output? = null,
public val grpc: Output? = null,
public val httpGet: Output? = null,
public val initialDelaySeconds: Output? = null,
public val periodSeconds: Output? = null,
public val tcpSocket: Output? = null,
public val timeoutSeconds: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudrunv2.inputs.ServiceTemplateContainerStartupProbeArgs =
com.pulumi.gcp.cloudrunv2.inputs.ServiceTemplateContainerStartupProbeArgs.builder()
.failureThreshold(failureThreshold?.applyValue({ args0 -> args0 }))
.grpc(grpc?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpGet(httpGet?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.initialDelaySeconds(initialDelaySeconds?.applyValue({ args0 -> args0 }))
.periodSeconds(periodSeconds?.applyValue({ args0 -> args0 }))
.tcpSocket(tcpSocket?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.timeoutSeconds(timeoutSeconds?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceTemplateContainerStartupProbeArgs].
*/
@PulumiTagMarker
public class ServiceTemplateContainerStartupProbeArgsBuilder internal constructor() {
private var failureThreshold: Output? = null
private var grpc: Output? = null
private var httpGet: Output? = null
private var initialDelaySeconds: Output? = null
private var periodSeconds: Output? = null
private var tcpSocket: Output? = null
private var timeoutSeconds: Output? = null
/**
* @param value Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1.
*/
@JvmName("rlojnlfadbceggck")
public suspend fun failureThreshold(`value`: Output) {
this.failureThreshold = value
}
/**
* @param value GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("knkabapfrlddhbhm")
public suspend fun grpc(`value`: Output) {
this.grpc = value
}
/**
* @param value HTTPGet specifies the http request to perform. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("erunxiyugksryocx")
public suspend fun httpGet(`value`: Output) {
this.httpGet = value
}
/**
* @param value Number of seconds after the container has started before the probe is initiated. Defaults to 0 seconds. Minimum value is 0. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
*/
@JvmName("stdljnvxnsfxfwtq")
public suspend fun initialDelaySeconds(`value`: Output) {
this.initialDelaySeconds = value
}
/**
* @param value How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. Must be greater or equal than timeoutSeconds
*/
@JvmName("pbkdnvkrjpqstujl")
public suspend fun periodSeconds(`value`: Output) {
this.periodSeconds = value
}
/**
* @param value TCPSocket specifies an action involving a TCP port. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("hgldodgpchosvqio")
public suspend fun tcpSocket(`value`: Output) {
this.tcpSocket = value
}
/**
* @param value Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is 3600. Must be smaller than periodSeconds. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
*/
@JvmName("lwkvjqskoclgkvqw")
public suspend fun timeoutSeconds(`value`: Output) {
this.timeoutSeconds = value
}
/**
* @param value Minimum consecutive failures for the probe to be considered failed after having succeeded. Defaults to 3. Minimum value is 1.
*/
@JvmName("ynbwfxfkjtqwqowi")
public suspend fun failureThreshold(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.failureThreshold = mapped
}
/**
* @param value GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("dnoegnooepidvdnl")
public suspend fun grpc(`value`: ServiceTemplateContainerStartupProbeGrpcArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.grpc = mapped
}
/**
* @param argument GRPC specifies an action involving a GRPC port.
* Structure is documented below.
*/
@JvmName("ugtbhmqipsnfwgil")
public suspend fun grpc(argument: suspend ServiceTemplateContainerStartupProbeGrpcArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateContainerStartupProbeGrpcArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.grpc = mapped
}
/**
* @param value HTTPGet specifies the http request to perform. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("tteornxrelwvsobe")
public suspend fun httpGet(`value`: ServiceTemplateContainerStartupProbeHttpGetArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpGet = mapped
}
/**
* @param argument HTTPGet specifies the http request to perform. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("qkacggfpvvcrdfmp")
public suspend fun httpGet(argument: suspend ServiceTemplateContainerStartupProbeHttpGetArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateContainerStartupProbeHttpGetArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.httpGet = mapped
}
/**
* @param value Number of seconds after the container has started before the probe is initiated. Defaults to 0 seconds. Minimum value is 0. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
*/
@JvmName("vwqvctlavtjtqree")
public suspend fun initialDelaySeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.initialDelaySeconds = mapped
}
/**
* @param value How often (in seconds) to perform the probe. Default to 10 seconds. Minimum value is 1. Maximum value for liveness probe is 3600. Maximum value for startup probe is 240. Must be greater or equal than timeoutSeconds
*/
@JvmName("rcaxoxljnfskarod")
public suspend fun periodSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.periodSeconds = mapped
}
/**
* @param value TCPSocket specifies an action involving a TCP port. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("vujrkggqpmwenyon")
public suspend fun tcpSocket(`value`: ServiceTemplateContainerStartupProbeTcpSocketArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tcpSocket = mapped
}
/**
* @param argument TCPSocket specifies an action involving a TCP port. Exactly one of HTTPGet or TCPSocket must be specified.
* Structure is documented below.
*/
@JvmName("valeattggvovqwed")
public suspend fun tcpSocket(argument: suspend ServiceTemplateContainerStartupProbeTcpSocketArgsBuilder.() -> Unit) {
val toBeMapped = ServiceTemplateContainerStartupProbeTcpSocketArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.tcpSocket = mapped
}
/**
* @param value Number of seconds after which the probe times out. Defaults to 1 second. Minimum value is 1. Maximum value is 3600. Must be smaller than periodSeconds. More info: https://kubernetes.io/docs/concepts/workloads/pods/pod-lifecycle#container-probes
*/
@JvmName("omybqxjqvlotrwad")
public suspend fun timeoutSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutSeconds = mapped
}
internal fun build(): ServiceTemplateContainerStartupProbeArgs =
ServiceTemplateContainerStartupProbeArgs(
failureThreshold = failureThreshold,
grpc = grpc,
httpGet = httpGet,
initialDelaySeconds = initialDelaySeconds,
periodSeconds = periodSeconds,
tcpSocket = tcpSocket,
timeoutSeconds = timeoutSeconds,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy