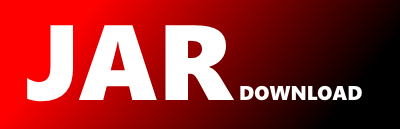
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudrunv2.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getJob.
* @property annotations
* @property binaryAuthorizations
* @property client
* @property clientVersion
* @property conditions
* @property createTime
* @property creator
* @property deleteTime
* @property effectiveAnnotations
* @property effectiveLabels
* @property etag
* @property executionCount
* @property expireTime
* @property generation
* @property id The provider-assigned unique ID for this managed resource.
* @property labels
* @property lastModifier
* @property latestCreatedExecutions
* @property launchStage
* @property location
* @property name
* @property observedGeneration
* @property project
* @property pulumiLabels
* @property reconciling
* @property templates
* @property terminalConditions
* @property uid
* @property updateTime
*/
public data class GetJobResult(
public val annotations: Map,
public val binaryAuthorizations: List,
public val client: String,
public val clientVersion: String,
public val conditions: List,
public val createTime: String,
public val creator: String,
public val deleteTime: String,
public val effectiveAnnotations: Map,
public val effectiveLabels: Map,
public val etag: String,
public val executionCount: Int,
public val expireTime: String,
public val generation: String,
public val id: String,
public val labels: Map,
public val lastModifier: String,
public val latestCreatedExecutions: List,
public val launchStage: String,
public val location: String? = null,
public val name: String,
public val observedGeneration: String,
public val project: String? = null,
public val pulumiLabels: Map,
public val reconciling: Boolean,
public val templates: List,
public val terminalConditions: List,
public val uid: String,
public val updateTime: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.cloudrunv2.outputs.GetJobResult): GetJobResult =
GetJobResult(
annotations = javaType.annotations().map({ args0 -> args0.key.to(args0.value) }).toMap(),
binaryAuthorizations = javaType.binaryAuthorizations().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobBinaryAuthorization.Companion.toKotlin(args0)
})
}),
client = javaType.client(),
clientVersion = javaType.clientVersion(),
conditions = javaType.conditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobCondition.Companion.toKotlin(args0)
})
}),
createTime = javaType.createTime(),
creator = javaType.creator(),
deleteTime = javaType.deleteTime(),
effectiveAnnotations = javaType.effectiveAnnotations().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
etag = javaType.etag(),
executionCount = javaType.executionCount(),
expireTime = javaType.expireTime(),
generation = javaType.generation(),
id = javaType.id(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
lastModifier = javaType.lastModifier(),
latestCreatedExecutions = javaType.latestCreatedExecutions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobLatestCreatedExecution.Companion.toKotlin(args0)
})
}),
launchStage = javaType.launchStage(),
location = javaType.location().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
observedGeneration = javaType.observedGeneration(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
reconciling = javaType.reconciling(),
templates = javaType.templates().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobTemplate.Companion.toKotlin(args0)
})
}),
terminalConditions = javaType.terminalConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.cloudrunv2.kotlin.outputs.GetJobTerminalCondition.Companion.toKotlin(args0)
})
}),
uid = javaType.uid(),
updateTime = javaType.updateTime(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy