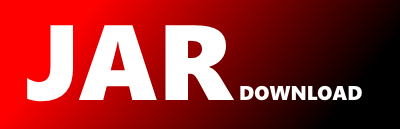
com.pulumi.gcp.cloudscheduler.kotlin.inputs.JobRetryConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudscheduler.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudscheduler.inputs.JobRetryConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property maxBackoffDuration The maximum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
* @property maxDoublings The time between retries will double maxDoublings times.
* A job's retry interval starts at minBackoffDuration,
* then doubles maxDoublings times, then increases linearly,
* and finally retries retries at intervals of maxBackoffDuration up to retryCount times.
* @property maxRetryDuration The time limit for retrying a failed job, measured from time when an execution was first attempted.
* If specified with retryCount, the job will be retried until both limits are reached.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
* @property minBackoffDuration The minimum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
* @property retryCount The number of attempts that the system will make to run a
* job using the exponential backoff procedure described by maxDoublings.
* Values greater than 5 and negative values are not allowed.
*/
public data class JobRetryConfigArgs(
public val maxBackoffDuration: Output? = null,
public val maxDoublings: Output? = null,
public val maxRetryDuration: Output? = null,
public val minBackoffDuration: Output? = null,
public val retryCount: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudscheduler.inputs.JobRetryConfigArgs =
com.pulumi.gcp.cloudscheduler.inputs.JobRetryConfigArgs.builder()
.maxBackoffDuration(maxBackoffDuration?.applyValue({ args0 -> args0 }))
.maxDoublings(maxDoublings?.applyValue({ args0 -> args0 }))
.maxRetryDuration(maxRetryDuration?.applyValue({ args0 -> args0 }))
.minBackoffDuration(minBackoffDuration?.applyValue({ args0 -> args0 }))
.retryCount(retryCount?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JobRetryConfigArgs].
*/
@PulumiTagMarker
public class JobRetryConfigArgsBuilder internal constructor() {
private var maxBackoffDuration: Output? = null
private var maxDoublings: Output? = null
private var maxRetryDuration: Output? = null
private var minBackoffDuration: Output? = null
private var retryCount: Output? = null
/**
* @param value The maximum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("oxwpvevoiwplxwks")
public suspend fun maxBackoffDuration(`value`: Output) {
this.maxBackoffDuration = value
}
/**
* @param value The time between retries will double maxDoublings times.
* A job's retry interval starts at minBackoffDuration,
* then doubles maxDoublings times, then increases linearly,
* and finally retries retries at intervals of maxBackoffDuration up to retryCount times.
*/
@JvmName("ppqbeophmpncmtuf")
public suspend fun maxDoublings(`value`: Output) {
this.maxDoublings = value
}
/**
* @param value The time limit for retrying a failed job, measured from time when an execution was first attempted.
* If specified with retryCount, the job will be retried until both limits are reached.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("kuhfsjnimtdemkfl")
public suspend fun maxRetryDuration(`value`: Output) {
this.maxRetryDuration = value
}
/**
* @param value The minimum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("ndjhaeitkbvsgkpi")
public suspend fun minBackoffDuration(`value`: Output) {
this.minBackoffDuration = value
}
/**
* @param value The number of attempts that the system will make to run a
* job using the exponential backoff procedure described by maxDoublings.
* Values greater than 5 and negative values are not allowed.
*/
@JvmName("oypmvpuwjmmrttyw")
public suspend fun retryCount(`value`: Output) {
this.retryCount = value
}
/**
* @param value The maximum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("yhbdwvlspvrusvqa")
public suspend fun maxBackoffDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxBackoffDuration = mapped
}
/**
* @param value The time between retries will double maxDoublings times.
* A job's retry interval starts at minBackoffDuration,
* then doubles maxDoublings times, then increases linearly,
* and finally retries retries at intervals of maxBackoffDuration up to retryCount times.
*/
@JvmName("xvmjccyinlocfhpr")
public suspend fun maxDoublings(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxDoublings = mapped
}
/**
* @param value The time limit for retrying a failed job, measured from time when an execution was first attempted.
* If specified with retryCount, the job will be retried until both limits are reached.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("rrbklwhojvpsnqan")
public suspend fun maxRetryDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRetryDuration = mapped
}
/**
* @param value The minimum amount of time to wait before retrying a job after it fails.
* A duration in seconds with up to nine fractional digits, terminated by 's'.
*/
@JvmName("ecmehayqgybwrmgb")
public suspend fun minBackoffDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minBackoffDuration = mapped
}
/**
* @param value The number of attempts that the system will make to run a
* job using the exponential backoff procedure described by maxDoublings.
* Values greater than 5 and negative values are not allowed.
*/
@JvmName("amgiuwxqdfgxnpum")
public suspend fun retryCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryCount = mapped
}
internal fun build(): JobRetryConfigArgs = JobRetryConfigArgs(
maxBackoffDuration = maxBackoffDuration,
maxDoublings = maxDoublings,
maxRetryDuration = maxRetryDuration,
minBackoffDuration = minBackoffDuration,
retryCount = retryCount,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy