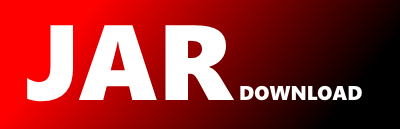
com.pulumi.gcp.cloudtasks.kotlin.inputs.QueueRetryConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.cloudtasks.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.cloudtasks.inputs.QueueRetryConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property maxAttempts Number of attempts per task.
* Cloud Tasks will attempt the task maxAttempts times (that is, if
* the first attempt fails, then there will be maxAttempts - 1
* retries). Must be >= -1.
* If unspecified when the queue is created, Cloud Tasks will pick
* the default.
* -1 indicates unlimited attempts.
* @property maxBackoff A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
* @property maxDoublings The time between retries will double maxDoublings times.
* A task's retry interval starts at minBackoff, then doubles maxDoublings times,
* then increases linearly, and finally retries retries at intervals of maxBackoff
* up to maxAttempts times.
* @property maxRetryDuration If positive, maxRetryDuration specifies the time limit for
* retrying a failed task, measured from when the task was first
* attempted. Once maxRetryDuration time has passed and the task has
* been attempted maxAttempts times, no further attempts will be
* made and the task will be deleted.
* If zero, then the task age is unlimited.
* @property minBackoff A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
*/
public data class QueueRetryConfigArgs(
public val maxAttempts: Output? = null,
public val maxBackoff: Output? = null,
public val maxDoublings: Output? = null,
public val maxRetryDuration: Output? = null,
public val minBackoff: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.cloudtasks.inputs.QueueRetryConfigArgs =
com.pulumi.gcp.cloudtasks.inputs.QueueRetryConfigArgs.builder()
.maxAttempts(maxAttempts?.applyValue({ args0 -> args0 }))
.maxBackoff(maxBackoff?.applyValue({ args0 -> args0 }))
.maxDoublings(maxDoublings?.applyValue({ args0 -> args0 }))
.maxRetryDuration(maxRetryDuration?.applyValue({ args0 -> args0 }))
.minBackoff(minBackoff?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [QueueRetryConfigArgs].
*/
@PulumiTagMarker
public class QueueRetryConfigArgsBuilder internal constructor() {
private var maxAttempts: Output? = null
private var maxBackoff: Output? = null
private var maxDoublings: Output? = null
private var maxRetryDuration: Output? = null
private var minBackoff: Output? = null
/**
* @param value Number of attempts per task.
* Cloud Tasks will attempt the task maxAttempts times (that is, if
* the first attempt fails, then there will be maxAttempts - 1
* retries). Must be >= -1.
* If unspecified when the queue is created, Cloud Tasks will pick
* the default.
* -1 indicates unlimited attempts.
*/
@JvmName("qjsmkvucrfwbdxfm")
public suspend fun maxAttempts(`value`: Output) {
this.maxAttempts = value
}
/**
* @param value A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
*/
@JvmName("towcmrybpxyrmokx")
public suspend fun maxBackoff(`value`: Output) {
this.maxBackoff = value
}
/**
* @param value The time between retries will double maxDoublings times.
* A task's retry interval starts at minBackoff, then doubles maxDoublings times,
* then increases linearly, and finally retries retries at intervals of maxBackoff
* up to maxAttempts times.
*/
@JvmName("gmvudgprdctceqeg")
public suspend fun maxDoublings(`value`: Output) {
this.maxDoublings = value
}
/**
* @param value If positive, maxRetryDuration specifies the time limit for
* retrying a failed task, measured from when the task was first
* attempted. Once maxRetryDuration time has passed and the task has
* been attempted maxAttempts times, no further attempts will be
* made and the task will be deleted.
* If zero, then the task age is unlimited.
*/
@JvmName("fhoybbhuldmyakyn")
public suspend fun maxRetryDuration(`value`: Output) {
this.maxRetryDuration = value
}
/**
* @param value A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
*/
@JvmName("lopdrtqnqelmwgru")
public suspend fun minBackoff(`value`: Output) {
this.minBackoff = value
}
/**
* @param value Number of attempts per task.
* Cloud Tasks will attempt the task maxAttempts times (that is, if
* the first attempt fails, then there will be maxAttempts - 1
* retries). Must be >= -1.
* If unspecified when the queue is created, Cloud Tasks will pick
* the default.
* -1 indicates unlimited attempts.
*/
@JvmName("mpxlotkmosfwputg")
public suspend fun maxAttempts(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxAttempts = mapped
}
/**
* @param value A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
*/
@JvmName("jmxytqbrafbegori")
public suspend fun maxBackoff(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxBackoff = mapped
}
/**
* @param value The time between retries will double maxDoublings times.
* A task's retry interval starts at minBackoff, then doubles maxDoublings times,
* then increases linearly, and finally retries retries at intervals of maxBackoff
* up to maxAttempts times.
*/
@JvmName("olavfoixitobxnjy")
public suspend fun maxDoublings(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxDoublings = mapped
}
/**
* @param value If positive, maxRetryDuration specifies the time limit for
* retrying a failed task, measured from when the task was first
* attempted. Once maxRetryDuration time has passed and the task has
* been attempted maxAttempts times, no further attempts will be
* made and the task will be deleted.
* If zero, then the task age is unlimited.
*/
@JvmName("jpmpyoxrkukihugk")
public suspend fun maxRetryDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRetryDuration = mapped
}
/**
* @param value A task will be scheduled for retry between minBackoff and
* maxBackoff duration after it fails, if the queue's RetryConfig
* specifies that the task should be retried.
*/
@JvmName("muodlyqqrkrnelsb")
public suspend fun minBackoff(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minBackoff = mapped
}
internal fun build(): QueueRetryConfigArgs = QueueRetryConfigArgs(
maxAttempts = maxAttempts,
maxBackoff = maxBackoff,
maxDoublings = maxDoublings,
maxRetryDuration = maxRetryDuration,
minBackoff = minBackoff,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy