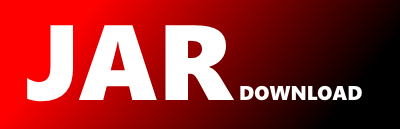
com.pulumi.gcp.composer.kotlin.inputs.EnvironmentConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.composer.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.composer.inputs.EnvironmentConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property airflowUri The URI of the Apache Airflow Web UI hosted within this
* environment.
* @property dagGcsPrefix The Cloud Storage prefix of the DAGs for this environment.
* Although Cloud Storage objects reside in a flat namespace, a
* hierarchical file tree can be simulated using '/'-delimited
* object name prefixes. DAG objects for this environment
* reside in a simulated directory with this prefix.
* @property dataRetentionConfig The configuration setting for Airflow data retention mechanism. This field is supported for Cloud Composer environments in versions composer-2.0.32-airflow-2.1.4. or newer
* @property databaseConfig The configuration of Cloud SQL instance that is used by the Apache Airflow software. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property enablePrivateBuildsOnly Optional. If true, builds performed during operations that install Python packages have only private connectivity to Google services. If false, the builds also have access to the internet.
* @property enablePrivateEnvironment Optional. If true, a private Composer environment will be created.
* @property encryptionConfig The encryption options for the Composer environment and its dependencies.
* @property environmentSize The size of the Cloud Composer environment. This field is supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
* @property gkeCluster The Kubernetes Engine cluster used to run this environment.
* @property maintenanceWindow The configuration for Cloud Composer maintenance window.
* @property masterAuthorizedNetworksConfig Configuration options for the master authorized networks feature. Enabled master authorized networks will disallow all external traffic to access Kubernetes master through HTTPS except traffic from the given CIDR blocks, Google Compute Engine Public IPs and Google Prod IPs.
* @property nodeConfig The configuration used for the Kubernetes Engine cluster.
* @property nodeCount The number of nodes in the Kubernetes Engine cluster that will be used to run this environment. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property privateEnvironmentConfig The configuration used for the Private IP Cloud Composer environment.
* @property recoveryConfig The recovery configuration settings for the Cloud Composer environment
* @property resilienceMode Whether high resilience is enabled or not. This field is supported for Cloud Composer environments in versions composer-2.1.15-airflow-*.*.* and newer.
* @property softwareConfig The configuration settings for software inside the environment.
* @property webServerConfig The configuration settings for the Airflow web server App Engine instance. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
* @property webServerNetworkAccessControl Network-level access control policy for the Airflow web server.
* @property workloadsConfig The workloads configuration settings for the GKE cluster associated with the Cloud Composer environment. Supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
public data class EnvironmentConfigArgs(
public val airflowUri: Output? = null,
public val dagGcsPrefix: Output? = null,
public val dataRetentionConfig: Output? = null,
public val databaseConfig: Output? = null,
public val enablePrivateBuildsOnly: Output? = null,
public val enablePrivateEnvironment: Output? = null,
public val encryptionConfig: Output? = null,
public val environmentSize: Output? = null,
public val gkeCluster: Output? = null,
public val maintenanceWindow: Output? = null,
public val masterAuthorizedNetworksConfig: Output? = null,
public val nodeConfig: Output? = null,
public val nodeCount: Output? = null,
public val privateEnvironmentConfig: Output? =
null,
public val recoveryConfig: Output? = null,
public val resilienceMode: Output? = null,
public val softwareConfig: Output? = null,
public val webServerConfig: Output? = null,
public val webServerNetworkAccessControl: Output? = null,
public val workloadsConfig: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.composer.inputs.EnvironmentConfigArgs =
com.pulumi.gcp.composer.inputs.EnvironmentConfigArgs.builder()
.airflowUri(airflowUri?.applyValue({ args0 -> args0 }))
.dagGcsPrefix(dagGcsPrefix?.applyValue({ args0 -> args0 }))
.dataRetentionConfig(
dataRetentionConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.databaseConfig(databaseConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.enablePrivateBuildsOnly(enablePrivateBuildsOnly?.applyValue({ args0 -> args0 }))
.enablePrivateEnvironment(enablePrivateEnvironment?.applyValue({ args0 -> args0 }))
.encryptionConfig(encryptionConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.environmentSize(environmentSize?.applyValue({ args0 -> args0 }))
.gkeCluster(gkeCluster?.applyValue({ args0 -> args0 }))
.maintenanceWindow(maintenanceWindow?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.masterAuthorizedNetworksConfig(
masterAuthorizedNetworksConfig?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.nodeConfig(nodeConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.nodeCount(nodeCount?.applyValue({ args0 -> args0 }))
.privateEnvironmentConfig(
privateEnvironmentConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.recoveryConfig(recoveryConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resilienceMode(resilienceMode?.applyValue({ args0 -> args0 }))
.softwareConfig(softwareConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.webServerConfig(webServerConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.webServerNetworkAccessControl(
webServerNetworkAccessControl?.applyValue({ args0 ->
args0.let({ args0 -> args0.toJava() })
}),
)
.workloadsConfig(
workloadsConfig?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [EnvironmentConfigArgs].
*/
@PulumiTagMarker
public class EnvironmentConfigArgsBuilder internal constructor() {
private var airflowUri: Output? = null
private var dagGcsPrefix: Output? = null
private var dataRetentionConfig: Output? = null
private var databaseConfig: Output? = null
private var enablePrivateBuildsOnly: Output? = null
private var enablePrivateEnvironment: Output? = null
private var encryptionConfig: Output? = null
private var environmentSize: Output? = null
private var gkeCluster: Output? = null
private var maintenanceWindow: Output? = null
private var masterAuthorizedNetworksConfig:
Output? = null
private var nodeConfig: Output? = null
private var nodeCount: Output? = null
private var privateEnvironmentConfig: Output? =
null
private var recoveryConfig: Output? = null
private var resilienceMode: Output? = null
private var softwareConfig: Output? = null
private var webServerConfig: Output? = null
private var webServerNetworkAccessControl:
Output? = null
private var workloadsConfig: Output? = null
/**
* @param value The URI of the Apache Airflow Web UI hosted within this
* environment.
*/
@JvmName("atjvadcyuafskghi")
public suspend fun airflowUri(`value`: Output) {
this.airflowUri = value
}
/**
* @param value The Cloud Storage prefix of the DAGs for this environment.
* Although Cloud Storage objects reside in a flat namespace, a
* hierarchical file tree can be simulated using '/'-delimited
* object name prefixes. DAG objects for this environment
* reside in a simulated directory with this prefix.
*/
@JvmName("batlvouswfnsgifk")
public suspend fun dagGcsPrefix(`value`: Output) {
this.dagGcsPrefix = value
}
/**
* @param value The configuration setting for Airflow data retention mechanism. This field is supported for Cloud Composer environments in versions composer-2.0.32-airflow-2.1.4. or newer
*/
@JvmName("bfcyshdqntvwjyls")
public suspend fun dataRetentionConfig(`value`: Output) {
this.dataRetentionConfig = value
}
/**
* @param value The configuration of Cloud SQL instance that is used by the Apache Airflow software. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("lteyouxrdugursjv")
public suspend fun databaseConfig(`value`: Output) {
this.databaseConfig = value
}
/**
* @param value Optional. If true, builds performed during operations that install Python packages have only private connectivity to Google services. If false, the builds also have access to the internet.
*/
@JvmName("thrmaugoqjrmhccq")
public suspend fun enablePrivateBuildsOnly(`value`: Output) {
this.enablePrivateBuildsOnly = value
}
/**
* @param value Optional. If true, a private Composer environment will be created.
*/
@JvmName("hjytgveqfgeybari")
public suspend fun enablePrivateEnvironment(`value`: Output) {
this.enablePrivateEnvironment = value
}
/**
* @param value The encryption options for the Composer environment and its dependencies.
*/
@JvmName("gnmwmbdqcijjjnrw")
public suspend fun encryptionConfig(`value`: Output) {
this.encryptionConfig = value
}
/**
* @param value The size of the Cloud Composer environment. This field is supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
@JvmName("cyldficlfvrokysl")
public suspend fun environmentSize(`value`: Output) {
this.environmentSize = value
}
/**
* @param value The Kubernetes Engine cluster used to run this environment.
*/
@JvmName("cftujiagrpycadbq")
public suspend fun gkeCluster(`value`: Output) {
this.gkeCluster = value
}
/**
* @param value The configuration for Cloud Composer maintenance window.
*/
@JvmName("hqctqqyvqotgnsuw")
public suspend fun maintenanceWindow(`value`: Output) {
this.maintenanceWindow = value
}
/**
* @param value Configuration options for the master authorized networks feature. Enabled master authorized networks will disallow all external traffic to access Kubernetes master through HTTPS except traffic from the given CIDR blocks, Google Compute Engine Public IPs and Google Prod IPs.
*/
@JvmName("ndvetkokhoekcriw")
public suspend fun masterAuthorizedNetworksConfig(`value`: Output) {
this.masterAuthorizedNetworksConfig = value
}
/**
* @param value The configuration used for the Kubernetes Engine cluster.
*/
@JvmName("hyvcnuhrvvjqxqav")
public suspend fun nodeConfig(`value`: Output) {
this.nodeConfig = value
}
/**
* @param value The number of nodes in the Kubernetes Engine cluster that will be used to run this environment. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("llkunhtjronbsbak")
public suspend fun nodeCount(`value`: Output) {
this.nodeCount = value
}
/**
* @param value The configuration used for the Private IP Cloud Composer environment.
*/
@JvmName("nihvmeuyaedtaeuq")
public suspend fun privateEnvironmentConfig(`value`: Output) {
this.privateEnvironmentConfig = value
}
/**
* @param value The recovery configuration settings for the Cloud Composer environment
*/
@JvmName("gtoknlkfhrxyctbh")
public suspend fun recoveryConfig(`value`: Output) {
this.recoveryConfig = value
}
/**
* @param value Whether high resilience is enabled or not. This field is supported for Cloud Composer environments in versions composer-2.1.15-airflow-*.*.* and newer.
*/
@JvmName("ptwskuorjbvfjcgu")
public suspend fun resilienceMode(`value`: Output) {
this.resilienceMode = value
}
/**
* @param value The configuration settings for software inside the environment.
*/
@JvmName("yacbjdsvtgvyaywr")
public suspend fun softwareConfig(`value`: Output) {
this.softwareConfig = value
}
/**
* @param value The configuration settings for the Airflow web server App Engine instance. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("wnmtuqxtrowliywk")
public suspend fun webServerConfig(`value`: Output) {
this.webServerConfig = value
}
/**
* @param value Network-level access control policy for the Airflow web server.
*/
@JvmName("mepthfxxcsuubgxl")
public suspend fun webServerNetworkAccessControl(`value`: Output) {
this.webServerNetworkAccessControl = value
}
/**
* @param value The workloads configuration settings for the GKE cluster associated with the Cloud Composer environment. Supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
@JvmName("tucekunjxmywturi")
public suspend fun workloadsConfig(`value`: Output) {
this.workloadsConfig = value
}
/**
* @param value The URI of the Apache Airflow Web UI hosted within this
* environment.
*/
@JvmName("hlkxrxtrqsnbptgl")
public suspend fun airflowUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.airflowUri = mapped
}
/**
* @param value The Cloud Storage prefix of the DAGs for this environment.
* Although Cloud Storage objects reside in a flat namespace, a
* hierarchical file tree can be simulated using '/'-delimited
* object name prefixes. DAG objects for this environment
* reside in a simulated directory with this prefix.
*/
@JvmName("rblycdcuqrcfwdmu")
public suspend fun dagGcsPrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dagGcsPrefix = mapped
}
/**
* @param value The configuration setting for Airflow data retention mechanism. This field is supported for Cloud Composer environments in versions composer-2.0.32-airflow-2.1.4. or newer
*/
@JvmName("dolomaivkufgmqoj")
public suspend fun dataRetentionConfig(`value`: EnvironmentConfigDataRetentionConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.dataRetentionConfig = mapped
}
/**
* @param argument The configuration setting for Airflow data retention mechanism. This field is supported for Cloud Composer environments in versions composer-2.0.32-airflow-2.1.4. or newer
*/
@JvmName("xlpbinnkolvqrtlo")
public suspend fun dataRetentionConfig(argument: suspend EnvironmentConfigDataRetentionConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigDataRetentionConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.dataRetentionConfig = mapped
}
/**
* @param value The configuration of Cloud SQL instance that is used by the Apache Airflow software. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("grqshttyndemanxg")
public suspend fun databaseConfig(`value`: EnvironmentConfigDatabaseConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.databaseConfig = mapped
}
/**
* @param argument The configuration of Cloud SQL instance that is used by the Apache Airflow software. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("shlfxlkvaqdpmnlu")
public suspend fun databaseConfig(argument: suspend EnvironmentConfigDatabaseConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigDatabaseConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.databaseConfig = mapped
}
/**
* @param value Optional. If true, builds performed during operations that install Python packages have only private connectivity to Google services. If false, the builds also have access to the internet.
*/
@JvmName("fnyuefatyvdtdxpj")
public suspend fun enablePrivateBuildsOnly(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateBuildsOnly = mapped
}
/**
* @param value Optional. If true, a private Composer environment will be created.
*/
@JvmName("nlwvxedbbqqnkfaw")
public suspend fun enablePrivateEnvironment(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enablePrivateEnvironment = mapped
}
/**
* @param value The encryption options for the Composer environment and its dependencies.
*/
@JvmName("pkighxjvilddchyy")
public suspend fun encryptionConfig(`value`: EnvironmentConfigEncryptionConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.encryptionConfig = mapped
}
/**
* @param argument The encryption options for the Composer environment and its dependencies.
*/
@JvmName("iyiaujqwlukaxtmk")
public suspend fun encryptionConfig(argument: suspend EnvironmentConfigEncryptionConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigEncryptionConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.encryptionConfig = mapped
}
/**
* @param value The size of the Cloud Composer environment. This field is supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
@JvmName("hrwgwunflmvwjcft")
public suspend fun environmentSize(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.environmentSize = mapped
}
/**
* @param value The Kubernetes Engine cluster used to run this environment.
*/
@JvmName("xksvqmllpcxbimqu")
public suspend fun gkeCluster(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.gkeCluster = mapped
}
/**
* @param value The configuration for Cloud Composer maintenance window.
*/
@JvmName("ftbglsfhmqioprua")
public suspend fun maintenanceWindow(`value`: EnvironmentConfigMaintenanceWindowArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maintenanceWindow = mapped
}
/**
* @param argument The configuration for Cloud Composer maintenance window.
*/
@JvmName("wvxiyrudxgjgebgv")
public suspend fun maintenanceWindow(argument: suspend EnvironmentConfigMaintenanceWindowArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigMaintenanceWindowArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.maintenanceWindow = mapped
}
/**
* @param value Configuration options for the master authorized networks feature. Enabled master authorized networks will disallow all external traffic to access Kubernetes master through HTTPS except traffic from the given CIDR blocks, Google Compute Engine Public IPs and Google Prod IPs.
*/
@JvmName("skidlpiohppibind")
public suspend fun masterAuthorizedNetworksConfig(`value`: EnvironmentConfigMasterAuthorizedNetworksConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.masterAuthorizedNetworksConfig = mapped
}
/**
* @param argument Configuration options for the master authorized networks feature. Enabled master authorized networks will disallow all external traffic to access Kubernetes master through HTTPS except traffic from the given CIDR blocks, Google Compute Engine Public IPs and Google Prod IPs.
*/
@JvmName("etylfwedfgqxlhgo")
public suspend fun masterAuthorizedNetworksConfig(argument: suspend EnvironmentConfigMasterAuthorizedNetworksConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigMasterAuthorizedNetworksConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.masterAuthorizedNetworksConfig = mapped
}
/**
* @param value The configuration used for the Kubernetes Engine cluster.
*/
@JvmName("ehxsjjysykbtocag")
public suspend fun nodeConfig(`value`: EnvironmentConfigNodeConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeConfig = mapped
}
/**
* @param argument The configuration used for the Kubernetes Engine cluster.
*/
@JvmName("uxkldsnghyarxywx")
public suspend fun nodeConfig(argument: suspend EnvironmentConfigNodeConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigNodeConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.nodeConfig = mapped
}
/**
* @param value The number of nodes in the Kubernetes Engine cluster that will be used to run this environment. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("jwbopvdfbhpddxge")
public suspend fun nodeCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeCount = mapped
}
/**
* @param value The configuration used for the Private IP Cloud Composer environment.
*/
@JvmName("kgkduyroxdopwoep")
public suspend fun privateEnvironmentConfig(`value`: EnvironmentConfigPrivateEnvironmentConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateEnvironmentConfig = mapped
}
/**
* @param argument The configuration used for the Private IP Cloud Composer environment.
*/
@JvmName("fwofynurgmokxlhq")
public suspend fun privateEnvironmentConfig(argument: suspend EnvironmentConfigPrivateEnvironmentConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigPrivateEnvironmentConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.privateEnvironmentConfig = mapped
}
/**
* @param value The recovery configuration settings for the Cloud Composer environment
*/
@JvmName("qbftrbhhaoasshvt")
public suspend fun recoveryConfig(`value`: EnvironmentConfigRecoveryConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.recoveryConfig = mapped
}
/**
* @param argument The recovery configuration settings for the Cloud Composer environment
*/
@JvmName("fbabyjnbiuiacmxy")
public suspend fun recoveryConfig(argument: suspend EnvironmentConfigRecoveryConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigRecoveryConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.recoveryConfig = mapped
}
/**
* @param value Whether high resilience is enabled or not. This field is supported for Cloud Composer environments in versions composer-2.1.15-airflow-*.*.* and newer.
*/
@JvmName("mcohrlyxavsitcls")
public suspend fun resilienceMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resilienceMode = mapped
}
/**
* @param value The configuration settings for software inside the environment.
*/
@JvmName("ccigxuipxxhskxbs")
public suspend fun softwareConfig(`value`: EnvironmentConfigSoftwareConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.softwareConfig = mapped
}
/**
* @param argument The configuration settings for software inside the environment.
*/
@JvmName("xxwihuweeyfimvdf")
public suspend fun softwareConfig(argument: suspend EnvironmentConfigSoftwareConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigSoftwareConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.softwareConfig = mapped
}
/**
* @param value The configuration settings for the Airflow web server App Engine instance. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("jvciltfvxbdeolrf")
public suspend fun webServerConfig(`value`: EnvironmentConfigWebServerConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webServerConfig = mapped
}
/**
* @param argument The configuration settings for the Airflow web server App Engine instance. This field is supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*.
*/
@JvmName("nqjkgwwiinjeqepe")
public suspend fun webServerConfig(argument: suspend EnvironmentConfigWebServerConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigWebServerConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.webServerConfig = mapped
}
/**
* @param value Network-level access control policy for the Airflow web server.
*/
@JvmName("grjyumwdoguavytp")
public suspend fun webServerNetworkAccessControl(`value`: EnvironmentConfigWebServerNetworkAccessControlArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.webServerNetworkAccessControl = mapped
}
/**
* @param argument Network-level access control policy for the Airflow web server.
*/
@JvmName("xmppfceqgsdajghk")
public suspend fun webServerNetworkAccessControl(argument: suspend EnvironmentConfigWebServerNetworkAccessControlArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigWebServerNetworkAccessControlArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.webServerNetworkAccessControl = mapped
}
/**
* @param value The workloads configuration settings for the GKE cluster associated with the Cloud Composer environment. Supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
@JvmName("kehpvivdjqyevgdj")
public suspend fun workloadsConfig(`value`: EnvironmentConfigWorkloadsConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.workloadsConfig = mapped
}
/**
* @param argument The workloads configuration settings for the GKE cluster associated with the Cloud Composer environment. Supported for Cloud Composer environments in versions composer-2.*.*-airflow-*.*.* and newer.
*/
@JvmName("ihfgnpgnocotpqsi")
public suspend fun workloadsConfig(argument: suspend EnvironmentConfigWorkloadsConfigArgsBuilder.() -> Unit) {
val toBeMapped = EnvironmentConfigWorkloadsConfigArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.workloadsConfig = mapped
}
internal fun build(): EnvironmentConfigArgs = EnvironmentConfigArgs(
airflowUri = airflowUri,
dagGcsPrefix = dagGcsPrefix,
dataRetentionConfig = dataRetentionConfig,
databaseConfig = databaseConfig,
enablePrivateBuildsOnly = enablePrivateBuildsOnly,
enablePrivateEnvironment = enablePrivateEnvironment,
encryptionConfig = encryptionConfig,
environmentSize = environmentSize,
gkeCluster = gkeCluster,
maintenanceWindow = maintenanceWindow,
masterAuthorizedNetworksConfig = masterAuthorizedNetworksConfig,
nodeConfig = nodeConfig,
nodeCount = nodeCount,
privateEnvironmentConfig = privateEnvironmentConfig,
recoveryConfig = recoveryConfig,
resilienceMode = resilienceMode,
softwareConfig = softwareConfig,
webServerConfig = webServerConfig,
webServerNetworkAccessControl = webServerNetworkAccessControl,
workloadsConfig = workloadsConfig,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy