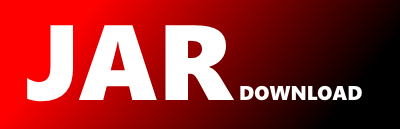
com.pulumi.gcp.composer.kotlin.inputs.EnvironmentConfigNodeConfigIpAllocationPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.composer.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.composer.inputs.EnvironmentConfigNodeConfigIpAllocationPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property clusterIpv4CidrBlock The IP address range used to allocate IP addresses to pods in the cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both.
* @property clusterSecondaryRangeName The name of the cluster's secondary range used to allocate IP addresses to pods. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
* @property servicesIpv4CidrBlock The IP address range used to allocate IP addresses in this cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both.
* @property servicesSecondaryRangeName The name of the services' secondary range used to allocate IP addresses to the cluster. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
* @property useIpAliases Whether or not to enable Alias IPs in the GKE cluster. If true, a VPC-native cluster is created. Defaults to true if the ip_allocation_policy block is present in config. This field is only supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*. Environments in newer versions always use VPC-native GKE clusters.
*/
public data class EnvironmentConfigNodeConfigIpAllocationPolicyArgs(
public val clusterIpv4CidrBlock: Output? = null,
public val clusterSecondaryRangeName: Output? = null,
public val servicesIpv4CidrBlock: Output? = null,
public val servicesSecondaryRangeName: Output? = null,
public val useIpAliases: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.composer.inputs.EnvironmentConfigNodeConfigIpAllocationPolicyArgs =
com.pulumi.gcp.composer.inputs.EnvironmentConfigNodeConfigIpAllocationPolicyArgs.builder()
.clusterIpv4CidrBlock(clusterIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.clusterSecondaryRangeName(clusterSecondaryRangeName?.applyValue({ args0 -> args0 }))
.servicesIpv4CidrBlock(servicesIpv4CidrBlock?.applyValue({ args0 -> args0 }))
.servicesSecondaryRangeName(servicesSecondaryRangeName?.applyValue({ args0 -> args0 }))
.useIpAliases(useIpAliases?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EnvironmentConfigNodeConfigIpAllocationPolicyArgs].
*/
@PulumiTagMarker
public class EnvironmentConfigNodeConfigIpAllocationPolicyArgsBuilder internal constructor() {
private var clusterIpv4CidrBlock: Output? = null
private var clusterSecondaryRangeName: Output? = null
private var servicesIpv4CidrBlock: Output? = null
private var servicesSecondaryRangeName: Output? = null
private var useIpAliases: Output? = null
/**
* @param value The IP address range used to allocate IP addresses to pods in the cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both.
*/
@JvmName("qyuhrirrroerbick")
public suspend fun clusterIpv4CidrBlock(`value`: Output) {
this.clusterIpv4CidrBlock = value
}
/**
* @param value The name of the cluster's secondary range used to allocate IP addresses to pods. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
*/
@JvmName("tnunlmkkdqhvnyhy")
public suspend fun clusterSecondaryRangeName(`value`: Output) {
this.clusterSecondaryRangeName = value
}
/**
* @param value The IP address range used to allocate IP addresses in this cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both.
*/
@JvmName("sijpsptdrohxlnid")
public suspend fun servicesIpv4CidrBlock(`value`: Output) {
this.servicesIpv4CidrBlock = value
}
/**
* @param value The name of the services' secondary range used to allocate IP addresses to the cluster. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
*/
@JvmName("mtqlhlvbnqishpgg")
public suspend fun servicesSecondaryRangeName(`value`: Output) {
this.servicesSecondaryRangeName = value
}
/**
* @param value Whether or not to enable Alias IPs in the GKE cluster. If true, a VPC-native cluster is created. Defaults to true if the ip_allocation_policy block is present in config. This field is only supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*. Environments in newer versions always use VPC-native GKE clusters.
*/
@JvmName("ewtqdhknmoeahxvu")
public suspend fun useIpAliases(`value`: Output) {
this.useIpAliases = value
}
/**
* @param value The IP address range used to allocate IP addresses to pods in the cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both.
*/
@JvmName("olploycjejdgxrhh")
public suspend fun clusterIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterIpv4CidrBlock = mapped
}
/**
* @param value The name of the cluster's secondary range used to allocate IP addresses to pods. Specify either cluster_secondary_range_name or cluster_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
*/
@JvmName("kfbqplpecenikjwb")
public suspend fun clusterSecondaryRangeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterSecondaryRangeName = mapped
}
/**
* @param value The IP address range used to allocate IP addresses in this cluster. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true. Set to blank to have GKE choose a range with the default size. Set to /netmask (e.g. /14) to have GKE choose a range with a specific netmask. Set to a CIDR notation (e.g. 10.96.0.0/14) from the RFC-1918 private networks (e.g. 10.0.0.0/8, 172.16.0.0/12, 192.168.0.0/16) to pick a specific range to use. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both.
*/
@JvmName("plvsopwfifabcfhy")
public suspend fun servicesIpv4CidrBlock(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.servicesIpv4CidrBlock = mapped
}
/**
* @param value The name of the services' secondary range used to allocate IP addresses to the cluster. Specify either services_secondary_range_name or services_ipv4_cidr_block but not both. For Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*, this field is applicable only when use_ip_aliases is true.
*/
@JvmName("bpnndigdqpksbmwb")
public suspend fun servicesSecondaryRangeName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.servicesSecondaryRangeName = mapped
}
/**
* @param value Whether or not to enable Alias IPs in the GKE cluster. If true, a VPC-native cluster is created. Defaults to true if the ip_allocation_policy block is present in config. This field is only supported for Cloud Composer environments in versions composer-1.*.*-airflow-*.*.*. Environments in newer versions always use VPC-native GKE clusters.
*/
@JvmName("wxhejkpfsgvymosi")
public suspend fun useIpAliases(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useIpAliases = mapped
}
internal fun build(): EnvironmentConfigNodeConfigIpAllocationPolicyArgs =
EnvironmentConfigNodeConfigIpAllocationPolicyArgs(
clusterIpv4CidrBlock = clusterIpv4CidrBlock,
clusterSecondaryRangeName = clusterSecondaryRangeName,
servicesIpv4CidrBlock = servicesIpv4CidrBlock,
servicesSecondaryRangeName = servicesSecondaryRangeName,
useIpAliases = useIpAliases,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy