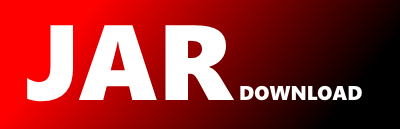
com.pulumi.gcp.compute.kotlin.BackendService.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceBackend
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceCdnPolicy
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceCircuitBreakers
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceConsistentHash
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceIap
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceLocalityLbPolicy
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceLogConfig
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceOutlierDetection
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceSecuritySettings
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceBackend.Companion.toKotlin as backendServiceBackendToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceCdnPolicy.Companion.toKotlin as backendServiceCdnPolicyToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceCircuitBreakers.Companion.toKotlin as backendServiceCircuitBreakersToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceConsistentHash.Companion.toKotlin as backendServiceConsistentHashToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceIap.Companion.toKotlin as backendServiceIapToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceLocalityLbPolicy.Companion.toKotlin as backendServiceLocalityLbPolicyToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceLogConfig.Companion.toKotlin as backendServiceLogConfigToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceOutlierDetection.Companion.toKotlin as backendServiceOutlierDetectionToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.BackendServiceSecuritySettings.Companion.toKotlin as backendServiceSecuritySettingsToKotlin
/**
* Builder for [BackendService].
*/
@PulumiTagMarker
public class BackendServiceResourceBuilder internal constructor() {
public var name: String? = null
public var args: BackendServiceArgs = BackendServiceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend BackendServiceArgsBuilder.() -> Unit) {
val builder = BackendServiceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): BackendService {
val builtJavaResource = com.pulumi.gcp.compute.BackendService(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return BackendService(builtJavaResource)
}
}
/**
* A Backend Service defines a group of virtual machines that will serve
* traffic for load balancing. This resource is a global backend service,
* appropriate for external load balancing or self-managed internal load balancing.
* For managed internal load balancing, use a regional backend service instead.
* Currently self-managed internal load balancing is only available in beta.
* To get more information about BackendService, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/v1/backendServices)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/compute/docs/load-balancing/http/backend-service)
* ## Example Usage
* ### Backend Service Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: defaultHttpHealthCheck.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=default_http_health_check.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${defaultHttpHealthCheck.id}
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Backend Service External Iap
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.BackendService("default", {
* name: "tf-test-backend-service-external",
* protocol: "HTTP",
* loadBalancingScheme: "EXTERNAL",
* iap: {
* oauth2ClientId: "abc",
* oauth2ClientSecret: "xyz",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.BackendService("default",
* name="tf-test-backend-service-external",
* protocol="HTTP",
* load_balancing_scheme="EXTERNAL",
* iap=gcp.compute.BackendServiceIapArgs(
* oauth2_client_id="abc",
* oauth2_client_secret="xyz",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "tf-test-backend-service-external",
* Protocol = "HTTP",
* LoadBalancingScheme = "EXTERNAL",
* Iap = new Gcp.Compute.Inputs.BackendServiceIapArgs
* {
* Oauth2ClientId = "abc",
* Oauth2ClientSecret = "xyz",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("tf-test-backend-service-external"),
* Protocol: pulumi.String("HTTP"),
* LoadBalancingScheme: pulumi.String("EXTERNAL"),
* Iap: &compute.BackendServiceIapArgs{
* Oauth2ClientId: pulumi.String("abc"),
* Oauth2ClientSecret: pulumi.String("xyz"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceIapArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("tf-test-backend-service-external")
* .protocol("HTTP")
* .loadBalancingScheme("EXTERNAL")
* .iap(BackendServiceIapArgs.builder()
* .oauth2ClientId("abc")
* .oauth2ClientSecret("xyz")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: tf-test-backend-service-external
* protocol: HTTP
* loadBalancingScheme: EXTERNAL
* iap:
* oauth2ClientId: abc
* oauth2ClientSecret: xyz
* ```
*
* ### Backend Service Cache Simple
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: defaultHttpHealthCheck.id,
* enableCdn: true,
* cdnPolicy: {
* signedUrlCacheMaxAgeSec: 7200,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=default_http_health_check.id,
* enable_cdn=True,
* cdn_policy=gcp.compute.BackendServiceCdnPolicyArgs(
* signed_url_cache_max_age_sec=7200,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = defaultHttpHealthCheck.Id,
* EnableCdn = true,
* CdnPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyArgs
* {
* SignedUrlCacheMaxAgeSec = 7200,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: defaultHttpHealthCheck.ID(),
* EnableCdn: pulumi.Bool(true),
* CdnPolicy: &compute.BackendServiceCdnPolicyArgs{
* SignedUrlCacheMaxAgeSec: pulumi.Int(7200),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(defaultHttpHealthCheck.id())
* .enableCdn(true)
* .cdnPolicy(BackendServiceCdnPolicyArgs.builder()
* .signedUrlCacheMaxAgeSec(7200)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${defaultHttpHealthCheck.id}
* enableCdn: true
* cdnPolicy:
* signedUrlCacheMaxAgeSec: 7200
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Backend Service Cache Include Http Headers
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* enableCdn: true,
* cdnPolicy: {
* cacheMode: "USE_ORIGIN_HEADERS",
* cacheKeyPolicy: {
* includeHost: true,
* includeProtocol: true,
* includeQueryString: true,
* includeHttpHeaders: ["X-My-Header-Field"],
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.BackendService("default",
* name="backend-service",
* enable_cdn=True,
* cdn_policy=gcp.compute.BackendServiceCdnPolicyArgs(
* cache_mode="USE_ORIGIN_HEADERS",
* cache_key_policy=gcp.compute.BackendServiceCdnPolicyCacheKeyPolicyArgs(
* include_host=True,
* include_protocol=True,
* include_query_string=True,
* include_http_headers=["X-My-Header-Field"],
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* EnableCdn = true,
* CdnPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyArgs
* {
* CacheMode = "USE_ORIGIN_HEADERS",
* CacheKeyPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyCacheKeyPolicyArgs
* {
* IncludeHost = true,
* IncludeProtocol = true,
* IncludeQueryString = true,
* IncludeHttpHeaders = new[]
* {
* "X-My-Header-Field",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* EnableCdn: pulumi.Bool(true),
* CdnPolicy: &compute.BackendServiceCdnPolicyArgs{
* CacheMode: pulumi.String("USE_ORIGIN_HEADERS"),
* CacheKeyPolicy: &compute.BackendServiceCdnPolicyCacheKeyPolicyArgs{
* IncludeHost: pulumi.Bool(true),
* IncludeProtocol: pulumi.Bool(true),
* IncludeQueryString: pulumi.Bool(true),
* IncludeHttpHeaders: pulumi.StringArray{
* pulumi.String("X-My-Header-Field"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyCacheKeyPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .enableCdn(true)
* .cdnPolicy(BackendServiceCdnPolicyArgs.builder()
* .cacheMode("USE_ORIGIN_HEADERS")
* .cacheKeyPolicy(BackendServiceCdnPolicyCacheKeyPolicyArgs.builder()
* .includeHost(true)
* .includeProtocol(true)
* .includeQueryString(true)
* .includeHttpHeaders("X-My-Header-Field")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* enableCdn: true
* cdnPolicy:
* cacheMode: USE_ORIGIN_HEADERS
* cacheKeyPolicy:
* includeHost: true
* includeProtocol: true
* includeQueryString: true
* includeHttpHeaders:
* - X-My-Header-Field
* ```
*
* ### Backend Service Cache Include Named Cookies
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* enableCdn: true,
* cdnPolicy: {
* cacheMode: "CACHE_ALL_STATIC",
* defaultTtl: 3600,
* clientTtl: 7200,
* maxTtl: 10800,
* cacheKeyPolicy: {
* includeHost: true,
* includeProtocol: true,
* includeQueryString: true,
* includeNamedCookies: [
* "__next_preview_data",
* "__prerender_bypass",
* ],
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.BackendService("default",
* name="backend-service",
* enable_cdn=True,
* cdn_policy=gcp.compute.BackendServiceCdnPolicyArgs(
* cache_mode="CACHE_ALL_STATIC",
* default_ttl=3600,
* client_ttl=7200,
* max_ttl=10800,
* cache_key_policy=gcp.compute.BackendServiceCdnPolicyCacheKeyPolicyArgs(
* include_host=True,
* include_protocol=True,
* include_query_string=True,
* include_named_cookies=[
* "__next_preview_data",
* "__prerender_bypass",
* ],
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* EnableCdn = true,
* CdnPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyArgs
* {
* CacheMode = "CACHE_ALL_STATIC",
* DefaultTtl = 3600,
* ClientTtl = 7200,
* MaxTtl = 10800,
* CacheKeyPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyCacheKeyPolicyArgs
* {
* IncludeHost = true,
* IncludeProtocol = true,
* IncludeQueryString = true,
* IncludeNamedCookies = new[]
* {
* "__next_preview_data",
* "__prerender_bypass",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* EnableCdn: pulumi.Bool(true),
* CdnPolicy: &compute.BackendServiceCdnPolicyArgs{
* CacheMode: pulumi.String("CACHE_ALL_STATIC"),
* DefaultTtl: pulumi.Int(3600),
* ClientTtl: pulumi.Int(7200),
* MaxTtl: pulumi.Int(10800),
* CacheKeyPolicy: &compute.BackendServiceCdnPolicyCacheKeyPolicyArgs{
* IncludeHost: pulumi.Bool(true),
* IncludeProtocol: pulumi.Bool(true),
* IncludeQueryString: pulumi.Bool(true),
* IncludeNamedCookies: pulumi.StringArray{
* pulumi.String("__next_preview_data"),
* pulumi.String("__prerender_bypass"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyCacheKeyPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .enableCdn(true)
* .cdnPolicy(BackendServiceCdnPolicyArgs.builder()
* .cacheMode("CACHE_ALL_STATIC")
* .defaultTtl(3600)
* .clientTtl(7200)
* .maxTtl(10800)
* .cacheKeyPolicy(BackendServiceCdnPolicyCacheKeyPolicyArgs.builder()
* .includeHost(true)
* .includeProtocol(true)
* .includeQueryString(true)
* .includeNamedCookies(
* "__next_preview_data",
* "__prerender_bypass")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* enableCdn: true
* cdnPolicy:
* cacheMode: CACHE_ALL_STATIC
* defaultTtl: 3600
* clientTtl: 7200
* maxTtl: 10800
* cacheKeyPolicy:
* includeHost: true
* includeProtocol: true
* includeQueryString: true
* includeNamedCookies:
* - __next_preview_data
* - __prerender_bypass
* ```
*
* ### Backend Service Cache
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: defaultHttpHealthCheck.id,
* enableCdn: true,
* cdnPolicy: {
* cacheMode: "CACHE_ALL_STATIC",
* defaultTtl: 3600,
* clientTtl: 7200,
* maxTtl: 10800,
* negativeCaching: true,
* signedUrlCacheMaxAgeSec: 7200,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=default_http_health_check.id,
* enable_cdn=True,
* cdn_policy=gcp.compute.BackendServiceCdnPolicyArgs(
* cache_mode="CACHE_ALL_STATIC",
* default_ttl=3600,
* client_ttl=7200,
* max_ttl=10800,
* negative_caching=True,
* signed_url_cache_max_age_sec=7200,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = defaultHttpHealthCheck.Id,
* EnableCdn = true,
* CdnPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyArgs
* {
* CacheMode = "CACHE_ALL_STATIC",
* DefaultTtl = 3600,
* ClientTtl = 7200,
* MaxTtl = 10800,
* NegativeCaching = true,
* SignedUrlCacheMaxAgeSec = 7200,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: defaultHttpHealthCheck.ID(),
* EnableCdn: pulumi.Bool(true),
* CdnPolicy: &compute.BackendServiceCdnPolicyArgs{
* CacheMode: pulumi.String("CACHE_ALL_STATIC"),
* DefaultTtl: pulumi.Int(3600),
* ClientTtl: pulumi.Int(7200),
* MaxTtl: pulumi.Int(10800),
* NegativeCaching: pulumi.Bool(true),
* SignedUrlCacheMaxAgeSec: pulumi.Int(7200),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(defaultHttpHealthCheck.id())
* .enableCdn(true)
* .cdnPolicy(BackendServiceCdnPolicyArgs.builder()
* .cacheMode("CACHE_ALL_STATIC")
* .defaultTtl(3600)
* .clientTtl(7200)
* .maxTtl(10800)
* .negativeCaching(true)
* .signedUrlCacheMaxAgeSec(7200)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${defaultHttpHealthCheck.id}
* enableCdn: true
* cdnPolicy:
* cacheMode: CACHE_ALL_STATIC
* defaultTtl: 3600
* clientTtl: 7200
* maxTtl: 10800
* negativeCaching: true
* signedUrlCacheMaxAgeSec: 7200
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Backend Service Cache Bypass Cache On Request Headers
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: defaultHttpHealthCheck.id,
* enableCdn: true,
* cdnPolicy: {
* cacheMode: "CACHE_ALL_STATIC",
* defaultTtl: 3600,
* clientTtl: 7200,
* maxTtl: 10800,
* negativeCaching: true,
* signedUrlCacheMaxAgeSec: 7200,
* bypassCacheOnRequestHeaders: [
* {
* headerName: "Authorization",
* },
* {
* headerName: "Proxy-Authorization",
* },
* ],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=default_http_health_check.id,
* enable_cdn=True,
* cdn_policy=gcp.compute.BackendServiceCdnPolicyArgs(
* cache_mode="CACHE_ALL_STATIC",
* default_ttl=3600,
* client_ttl=7200,
* max_ttl=10800,
* negative_caching=True,
* signed_url_cache_max_age_sec=7200,
* bypass_cache_on_request_headers=[
* gcp.compute.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs(
* header_name="Authorization",
* ),
* gcp.compute.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs(
* header_name="Proxy-Authorization",
* ),
* ],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = defaultHttpHealthCheck.Id,
* EnableCdn = true,
* CdnPolicy = new Gcp.Compute.Inputs.BackendServiceCdnPolicyArgs
* {
* CacheMode = "CACHE_ALL_STATIC",
* DefaultTtl = 3600,
* ClientTtl = 7200,
* MaxTtl = 10800,
* NegativeCaching = true,
* SignedUrlCacheMaxAgeSec = 7200,
* BypassCacheOnRequestHeaders = new[]
* {
* new Gcp.Compute.Inputs.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs
* {
* HeaderName = "Authorization",
* },
* new Gcp.Compute.Inputs.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs
* {
* HeaderName = "Proxy-Authorization",
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: defaultHttpHealthCheck.ID(),
* EnableCdn: pulumi.Bool(true),
* CdnPolicy: &compute.BackendServiceCdnPolicyArgs{
* CacheMode: pulumi.String("CACHE_ALL_STATIC"),
* DefaultTtl: pulumi.Int(3600),
* ClientTtl: pulumi.Int(7200),
* MaxTtl: pulumi.Int(10800),
* NegativeCaching: pulumi.Bool(true),
* SignedUrlCacheMaxAgeSec: pulumi.Int(7200),
* BypassCacheOnRequestHeaders: compute.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArray{
* &compute.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs{
* HeaderName: pulumi.String("Authorization"),
* },
* &compute.BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs{
* HeaderName: pulumi.String("Proxy-Authorization"),
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCdnPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(defaultHttpHealthCheck.id())
* .enableCdn(true)
* .cdnPolicy(BackendServiceCdnPolicyArgs.builder()
* .cacheMode("CACHE_ALL_STATIC")
* .defaultTtl(3600)
* .clientTtl(7200)
* .maxTtl(10800)
* .negativeCaching(true)
* .signedUrlCacheMaxAgeSec(7200)
* .bypassCacheOnRequestHeaders(
* BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs.builder()
* .headerName("Authorization")
* .build(),
* BackendServiceCdnPolicyBypassCacheOnRequestHeaderArgs.builder()
* .headerName("Proxy-Authorization")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${defaultHttpHealthCheck.id}
* enableCdn: true
* cdnPolicy:
* cacheMode: CACHE_ALL_STATIC
* defaultTtl: 3600
* clientTtl: 7200
* maxTtl: 10800
* negativeCaching: true
* signedUrlCacheMaxAgeSec: 7200
* bypassCacheOnRequestHeaders:
* - headerName: Authorization
* - headerName: Proxy-Authorization
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Backend Service Traffic Director Round Robin
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const healthCheck = new gcp.compute.HealthCheck("health_check", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: healthCheck.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* localityLbPolicy: "ROUND_ROBIN",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* health_check = gcp.compute.HealthCheck("health_check",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=health_check.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED",
* locality_lb_policy="ROUND_ROBIN")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var healthCheck = new Gcp.Compute.HealthCheck("health_check", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = healthCheck.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* LocalityLbPolicy = "ROUND_ROBIN",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* healthCheck, err := compute.NewHealthCheck(ctx, "health_check", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: healthCheck.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* LocalityLbPolicy: pulumi.String("ROUND_ROBIN"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var healthCheck = new HealthCheck("healthCheck", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(healthCheck.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .localityLbPolicy("ROUND_ROBIN")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${healthCheck.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* localityLbPolicy: ROUND_ROBIN
* healthCheck:
* type: gcp:compute:HealthCheck
* name: health_check
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Backend Service Traffic Director Ring Hash
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const healthCheck = new gcp.compute.HealthCheck("health_check", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: healthCheck.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* localityLbPolicy: "RING_HASH",
* sessionAffinity: "HTTP_COOKIE",
* circuitBreakers: {
* maxConnections: 10,
* },
* consistentHash: {
* httpCookie: {
* ttl: {
* seconds: 11,
* nanos: 1111,
* },
* name: "mycookie",
* },
* },
* outlierDetection: {
* consecutiveErrors: 2,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* health_check = gcp.compute.HealthCheck("health_check",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=health_check.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED",
* locality_lb_policy="RING_HASH",
* session_affinity="HTTP_COOKIE",
* circuit_breakers=gcp.compute.BackendServiceCircuitBreakersArgs(
* max_connections=10,
* ),
* consistent_hash=gcp.compute.BackendServiceConsistentHashArgs(
* http_cookie=gcp.compute.BackendServiceConsistentHashHttpCookieArgs(
* ttl=gcp.compute.BackendServiceConsistentHashHttpCookieTtlArgs(
* seconds=11,
* nanos=1111,
* ),
* name="mycookie",
* ),
* ),
* outlier_detection=gcp.compute.BackendServiceOutlierDetectionArgs(
* consecutive_errors=2,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var healthCheck = new Gcp.Compute.HealthCheck("health_check", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = healthCheck.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* LocalityLbPolicy = "RING_HASH",
* SessionAffinity = "HTTP_COOKIE",
* CircuitBreakers = new Gcp.Compute.Inputs.BackendServiceCircuitBreakersArgs
* {
* MaxConnections = 10,
* },
* ConsistentHash = new Gcp.Compute.Inputs.BackendServiceConsistentHashArgs
* {
* HttpCookie = new Gcp.Compute.Inputs.BackendServiceConsistentHashHttpCookieArgs
* {
* Ttl = new Gcp.Compute.Inputs.BackendServiceConsistentHashHttpCookieTtlArgs
* {
* Seconds = 11,
* Nanos = 1111,
* },
* Name = "mycookie",
* },
* },
* OutlierDetection = new Gcp.Compute.Inputs.BackendServiceOutlierDetectionArgs
* {
* ConsecutiveErrors = 2,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* healthCheck, err := compute.NewHealthCheck(ctx, "health_check", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: healthCheck.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* LocalityLbPolicy: pulumi.String("RING_HASH"),
* SessionAffinity: pulumi.String("HTTP_COOKIE"),
* CircuitBreakers: &compute.BackendServiceCircuitBreakersArgs{
* MaxConnections: pulumi.Int(10),
* },
* ConsistentHash: &compute.BackendServiceConsistentHashArgs{
* HttpCookie: &compute.BackendServiceConsistentHashHttpCookieArgs{
* Ttl: &compute.BackendServiceConsistentHashHttpCookieTtlArgs{
* Seconds: pulumi.Int(11),
* Nanos: pulumi.Int(1111),
* },
* Name: pulumi.String("mycookie"),
* },
* },
* OutlierDetection: &compute.BackendServiceOutlierDetectionArgs{
* ConsecutiveErrors: pulumi.Int(2),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceCircuitBreakersArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceConsistentHashArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceConsistentHashHttpCookieArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceConsistentHashHttpCookieTtlArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceOutlierDetectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var healthCheck = new HealthCheck("healthCheck", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(healthCheck.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .localityLbPolicy("RING_HASH")
* .sessionAffinity("HTTP_COOKIE")
* .circuitBreakers(BackendServiceCircuitBreakersArgs.builder()
* .maxConnections(10)
* .build())
* .consistentHash(BackendServiceConsistentHashArgs.builder()
* .httpCookie(BackendServiceConsistentHashHttpCookieArgs.builder()
* .ttl(BackendServiceConsistentHashHttpCookieTtlArgs.builder()
* .seconds(11)
* .nanos(1111)
* .build())
* .name("mycookie")
* .build())
* .build())
* .outlierDetection(BackendServiceOutlierDetectionArgs.builder()
* .consecutiveErrors(2)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${healthCheck.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* localityLbPolicy: RING_HASH
* sessionAffinity: HTTP_COOKIE
* circuitBreakers:
* maxConnections: 10
* consistentHash:
* httpCookie:
* ttl:
* seconds: 11
* nanos: 1111
* name: mycookie
* outlierDetection:
* consecutiveErrors: 2
* healthCheck:
* type: gcp:compute:HealthCheck
* name: health_check
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Backend Service Network Endpoint
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const externalProxy = new gcp.compute.GlobalNetworkEndpointGroup("external_proxy", {
* name: "network-endpoint",
* networkEndpointType: "INTERNET_FQDN_PORT",
* defaultPort: 443,
* });
* const proxy = new gcp.compute.GlobalNetworkEndpoint("proxy", {
* globalNetworkEndpointGroup: externalProxy.id,
* fqdn: "test.example.com",
* port: externalProxy.defaultPort,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* enableCdn: true,
* timeoutSec: 10,
* connectionDrainingTimeoutSec: 10,
* customRequestHeaders: [proxy.fqdn.apply(fqdn => `host: ${fqdn}`)],
* customResponseHeaders: ["X-Cache-Hit: {cdn_cache_status}"],
* backends: [{
* group: externalProxy.id,
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* external_proxy = gcp.compute.GlobalNetworkEndpointGroup("external_proxy",
* name="network-endpoint",
* network_endpoint_type="INTERNET_FQDN_PORT",
* default_port=443)
* proxy = gcp.compute.GlobalNetworkEndpoint("proxy",
* global_network_endpoint_group=external_proxy.id,
* fqdn="test.example.com",
* port=external_proxy.default_port)
* default = gcp.compute.BackendService("default",
* name="backend-service",
* enable_cdn=True,
* timeout_sec=10,
* connection_draining_timeout_sec=10,
* custom_request_headers=[proxy.fqdn.apply(lambda fqdn: f"host: {fqdn}")],
* custom_response_headers=["X-Cache-Hit: {cdn_cache_status}"],
* backends=[gcp.compute.BackendServiceBackendArgs(
* group=external_proxy.id,
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var externalProxy = new Gcp.Compute.GlobalNetworkEndpointGroup("external_proxy", new()
* {
* Name = "network-endpoint",
* NetworkEndpointType = "INTERNET_FQDN_PORT",
* DefaultPort = 443,
* });
* var proxy = new Gcp.Compute.GlobalNetworkEndpoint("proxy", new()
* {
* GlobalNetworkEndpointGroup = externalProxy.Id,
* Fqdn = "test.example.com",
* Port = externalProxy.DefaultPort,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* EnableCdn = true,
* TimeoutSec = 10,
* ConnectionDrainingTimeoutSec = 10,
* CustomRequestHeaders = new[]
* {
* proxy.Fqdn.Apply(fqdn => $"host: {fqdn}"),
* },
* CustomResponseHeaders = new[]
* {
* "X-Cache-Hit: {cdn_cache_status}",
* },
* Backends = new[]
* {
* new Gcp.Compute.Inputs.BackendServiceBackendArgs
* {
* Group = externalProxy.Id,
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* externalProxy, err := compute.NewGlobalNetworkEndpointGroup(ctx, "external_proxy", &compute.GlobalNetworkEndpointGroupArgs{
* Name: pulumi.String("network-endpoint"),
* NetworkEndpointType: pulumi.String("INTERNET_FQDN_PORT"),
* DefaultPort: pulumi.Int(443),
* })
* if err != nil {
* return err
* }
* proxy, err := compute.NewGlobalNetworkEndpoint(ctx, "proxy", &compute.GlobalNetworkEndpointArgs{
* GlobalNetworkEndpointGroup: externalProxy.ID(),
* Fqdn: pulumi.String("test.example.com"),
* Port: externalProxy.DefaultPort,
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* EnableCdn: pulumi.Bool(true),
* TimeoutSec: pulumi.Int(10),
* ConnectionDrainingTimeoutSec: pulumi.Int(10),
* CustomRequestHeaders: pulumi.StringArray{
* proxy.Fqdn.ApplyT(func(fqdn *string) (string, error) {
* return fmt.Sprintf("host: %v", fqdn), nil
* }).(pulumi.StringOutput),
* },
* CustomResponseHeaders: pulumi.StringArray{
* pulumi.String("X-Cache-Hit: {cdn_cache_status}"),
* },
* Backends: compute.BackendServiceBackendArray{
* &compute.BackendServiceBackendArgs{
* Group: externalProxy.ID(),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.GlobalNetworkEndpointGroup;
* import com.pulumi.gcp.compute.GlobalNetworkEndpointGroupArgs;
* import com.pulumi.gcp.compute.GlobalNetworkEndpoint;
* import com.pulumi.gcp.compute.GlobalNetworkEndpointArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var externalProxy = new GlobalNetworkEndpointGroup("externalProxy", GlobalNetworkEndpointGroupArgs.builder()
* .name("network-endpoint")
* .networkEndpointType("INTERNET_FQDN_PORT")
* .defaultPort("443")
* .build());
* var proxy = new GlobalNetworkEndpoint("proxy", GlobalNetworkEndpointArgs.builder()
* .globalNetworkEndpointGroup(externalProxy.id())
* .fqdn("test.example.com")
* .port(externalProxy.defaultPort())
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .enableCdn(true)
* .timeoutSec(10)
* .connectionDrainingTimeoutSec(10)
* .customRequestHeaders(proxy.fqdn().applyValue(fqdn -> String.format("host: %s", fqdn)))
* .customResponseHeaders("X-Cache-Hit: {cdn_cache_status}")
* .backends(BackendServiceBackendArgs.builder()
* .group(externalProxy.id())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* externalProxy:
* type: gcp:compute:GlobalNetworkEndpointGroup
* name: external_proxy
* properties:
* name: network-endpoint
* networkEndpointType: INTERNET_FQDN_PORT
* defaultPort: '443'
* proxy:
* type: gcp:compute:GlobalNetworkEndpoint
* properties:
* globalNetworkEndpointGroup: ${externalProxy.id}
* fqdn: test.example.com
* port: ${externalProxy.defaultPort}
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* enableCdn: true
* timeoutSec: 10
* connectionDrainingTimeoutSec: 10
* customRequestHeaders:
* - 'host: ${proxy.fqdn}'
* customResponseHeaders:
* - 'X-Cache-Hit: {cdn_cache_status}'
* backends:
* - group: ${externalProxy.id}
* ```
*
* ### Backend Service External Managed
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHealthCheck = new gcp.compute.HealthCheck("default", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "backend-service",
* healthChecks: defaultHealthCheck.id,
* loadBalancingScheme: "EXTERNAL_MANAGED",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_health_check = gcp.compute.HealthCheck("default",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* default = gcp.compute.BackendService("default",
* name="backend-service",
* health_checks=default_health_check.id,
* load_balancing_scheme="EXTERNAL_MANAGED")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHealthCheck = new Gcp.Compute.HealthCheck("default", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "backend-service",
* HealthChecks = defaultHealthCheck.Id,
* LoadBalancingScheme = "EXTERNAL_MANAGED",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHealthCheck, err := compute.NewHealthCheck(ctx, "default", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("backend-service"),
* HealthChecks: defaultHealthCheck.ID(),
* LoadBalancingScheme: pulumi.String("EXTERNAL_MANAGED"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHealthCheck = new HealthCheck("defaultHealthCheck", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("backend-service")
* .healthChecks(defaultHealthCheck.id())
* .loadBalancingScheme("EXTERNAL_MANAGED")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:compute:BackendService
* properties:
* name: backend-service
* healthChecks: ${defaultHealthCheck.id}
* loadBalancingScheme: EXTERNAL_MANAGED
* defaultHealthCheck:
* type: gcp:compute:HealthCheck
* name: default
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ## Import
* BackendService can be imported using any of these accepted formats:
* * `projects/{{project}}/global/backendServices/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, BackendService can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/backendService:BackendService default projects/{{project}}/global/backendServices/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/backendService:BackendService default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/backendService:BackendService default {{name}}
* ```
*/
public class BackendService internal constructor(
override val javaResource: com.pulumi.gcp.compute.BackendService,
) : KotlinCustomResource(javaResource, BackendServiceMapper) {
/**
* Lifetime of cookies in seconds if session_affinity is
* GENERATED_COOKIE. If set to 0, the cookie is non-persistent and lasts
* only until the end of the browser session (or equivalent). The
* maximum allowed value for TTL is one day.
* When the load balancing scheme is INTERNAL, this field is not used.
*/
public val affinityCookieTtlSec: Output?
get() = javaResource.affinityCookieTtlSec().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The set of backends that serve this BackendService.
* Structure is documented below.
*/
public val backends: Output>?
get() = javaResource.backends().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> backendServiceBackendToKotlin(args0) })
})
}).orElse(null)
})
/**
* Cloud CDN configuration for this BackendService.
* Structure is documented below.
*/
public val cdnPolicy: Output
get() = javaResource.cdnPolicy().applyValue({ args0 ->
args0.let({ args0 ->
backendServiceCdnPolicyToKotlin(args0)
})
})
/**
* Settings controlling the volume of connections to a backend service. This field
* is applicable only when the load_balancing_scheme is set to INTERNAL_SELF_MANAGED.
* Structure is documented below.
*/
public val circuitBreakers: Output?
get() = javaResource.circuitBreakers().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> backendServiceCircuitBreakersToKotlin(args0) })
}).orElse(null)
})
/**
* Compress text responses using Brotli or gzip compression, based on the client's Accept-Encoding header.
* Possible values are: `AUTOMATIC`, `DISABLED`.
*/
public val compressionMode: Output?
get() = javaResource.compressionMode().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Time for which instance will be drained (not accept new
* connections, but still work to finish started).
*/
public val connectionDrainingTimeoutSec: Output?
get() = javaResource.connectionDrainingTimeoutSec().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Consistent Hash-based load balancing can be used to provide soft session
* affinity based on HTTP headers, cookies or other properties. This load balancing
* policy is applicable only for HTTP connections. The affinity to a particular
* destination host will be lost when one or more hosts are added/removed from the
* destination service. This field specifies parameters that control consistent
* hashing. This field only applies if the load_balancing_scheme is set to
* INTERNAL_SELF_MANAGED. This field is only applicable when locality_lb_policy is
* set to MAGLEV or RING_HASH.
* Structure is documented below.
*/
public val consistentHash: Output?
get() = javaResource.consistentHash().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> backendServiceConsistentHashToKotlin(args0) })
}).orElse(null)
})
/**
* Creation timestamp in RFC3339 text format.
*/
public val creationTimestamp: Output
get() = javaResource.creationTimestamp().applyValue({ args0 -> args0 })
/**
* Headers that the HTTP/S load balancer should add to proxied
* requests.
*/
public val customRequestHeaders: Output>?
get() = javaResource.customRequestHeaders().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Headers that the HTTP/S load balancer should add to proxied
* responses.
*/
public val customResponseHeaders: Output>?
get() = javaResource.customResponseHeaders().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* An optional description of this resource.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The resource URL for the edge security policy associated with this backend service.
*/
public val edgeSecurityPolicy: Output?
get() = javaResource.edgeSecurityPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If true, enable Cloud CDN for this BackendService.
*/
public val enableCdn: Output?
get() = javaResource.enableCdn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Fingerprint of this resource. A hash of the contents stored in this
* object. This field is used in optimistic locking.
*/
public val fingerprint: Output
get() = javaResource.fingerprint().applyValue({ args0 -> args0 })
/**
* The unique identifier for the resource. This identifier is defined by the server.
*/
public val generatedId: Output
get() = javaResource.generatedId().applyValue({ args0 -> args0 })
/**
* The set of URLs to the HttpHealthCheck or HttpsHealthCheck resource
* for health checking this BackendService. Currently at most one health
* check can be specified.
* A health check must be specified unless the backend service uses an internet
* or serverless NEG as a backend.
* For internal load balancing, a URL to a HealthCheck resource must be specified instead.
*/
public val healthChecks: Output?
get() = javaResource.healthChecks().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Settings for enabling Cloud Identity Aware Proxy
* Structure is documented below.
*/
public val iap: Output?
get() = javaResource.iap().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
backendServiceIapToKotlin(args0)
})
}).orElse(null)
})
/**
* Indicates whether the backend service will be used with internal or
* external load balancing. A backend service created for one type of
* load balancing cannot be used with the other. For more information, refer to
* [Choosing a load balancer](https://cloud.google.com/load-balancing/docs/backend-service).
* Default value is `EXTERNAL`.
* Possible values are: `EXTERNAL`, `INTERNAL_SELF_MANAGED`, `INTERNAL_MANAGED`, `EXTERNAL_MANAGED`.
*/
public val loadBalancingScheme: Output?
get() = javaResource.loadBalancingScheme().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A list of locality load balancing policies to be used in order of
* preference. Either the policy or the customPolicy field should be set.
* Overrides any value set in the localityLbPolicy field.
* localityLbPolicies is only supported when the BackendService is referenced
* by a URL Map that is referenced by a target gRPC proxy that has the
* validateForProxyless field set to true.
* Structure is documented below.
*/
public val localityLbPolicies: Output>?
get() = javaResource.localityLbPolicies().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
backendServiceLocalityLbPolicyToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The load balancing algorithm used within the scope of the locality.
* The possible values are:
* * `ROUND_ROBIN`: This is a simple policy in which each healthy backend
* is selected in round robin order.
* * `LEAST_REQUEST`: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests.
* * `RING_HASH`: The ring/modulo hash load balancer implements consistent
* hashing to backends. The algorithm has the property that the
* addition/removal of a host from a set of N hosts only affects
* 1/N of the requests.
* * `RANDOM`: The load balancer selects a random healthy host.
* * `ORIGINAL_DESTINATION`: Backend host is selected based on the client
* connection metadata, i.e., connections are opened
* to the same address as the destination address of
* the incoming connection before the connection
* was redirected to the load balancer.
* * `MAGLEV`: used as a drop in replacement for the ring hash load balancer.
* Maglev is not as stable as ring hash but has faster table lookup
* build times and host selection times. For more information about
* Maglev, refer to https://ai.google/research/pubs/pub44824
* * `WEIGHTED_MAGLEV`: Per-instance weighted Load Balancing via health check
* reported weights. If set, the Backend Service must
* configure a non legacy HTTP-based Health Check, and
* health check replies are expected to contain
* non-standard HTTP response header field
* X-Load-Balancing-Endpoint-Weight to specify the
* per-instance weights. If set, Load Balancing is weight
* based on the per-instance weights reported in the last
* processed health check replies, as long as every
* instance either reported a valid weight or had
* UNAVAILABLE_WEIGHT. Otherwise, Load Balancing remains
* equal-weight.
* This field is applicable to either:
* * A regional backend service with the service_protocol set to HTTP, HTTPS, or HTTP2,
* and loadBalancingScheme set to INTERNAL_MANAGED.
* * A global backend service with the load_balancing_scheme set to INTERNAL_SELF_MANAGED.
* * A regional backend service with loadBalancingScheme set to EXTERNAL (External Network
* Load Balancing). Only MAGLEV and WEIGHTED_MAGLEV values are possible for External
* Network Load Balancing. The default is MAGLEV.
* If session_affinity is not NONE, and this field is not set to MAGLEV, WEIGHTED_MAGLEV,
* or RING_HASH, session affinity settings will not take effect.
* Only ROUND_ROBIN and RING_HASH are supported when the backend service is referenced
* by a URL map that is bound to target gRPC proxy that has validate_for_proxyless
* field set to true.
* Possible values are: `ROUND_ROBIN`, `LEAST_REQUEST`, `RING_HASH`, `RANDOM`, `ORIGINAL_DESTINATION`, `MAGLEV`, `WEIGHTED_MAGLEV`.
*/
public val localityLbPolicy: Output?
get() = javaResource.localityLbPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* This field denotes the logging options for the load balancer traffic served by this backend service.
* If logging is enabled, logs will be exported to Stackdriver.
* Structure is documented below.
*/
public val logConfig: Output
get() = javaResource.logConfig().applyValue({ args0 ->
args0.let({ args0 ->
backendServiceLogConfigToKotlin(args0)
})
})
/**
* Name of the resource. Provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and match
* the regular expression `a-z?` which means the
* first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the last
* character, which cannot be a dash.
* - - -
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* Settings controlling eviction of unhealthy hosts from the load balancing pool.
* Applicable backend service types can be a global backend service with the
* loadBalancingScheme set to INTERNAL_SELF_MANAGED or EXTERNAL_MANAGED.
* Structure is documented below.
*/
public val outlierDetection: Output?
get() = javaResource.outlierDetection().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> backendServiceOutlierDetectionToKotlin(args0) })
}).orElse(null)
})
/**
* Name of backend port. The same name should appear in the instance
* groups referenced by this service. Required when the load balancing
* scheme is EXTERNAL.
*/
public val portName: Output
get() = javaResource.portName().applyValue({ args0 -> args0 })
/**
* The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* The protocol this BackendService uses to communicate with backends.
* The default is HTTP. **NOTE**: HTTP2 is only valid for beta HTTP/2 load balancer
* types and may result in errors if used with the GA API. **NOTE**: With protocol “UNSPECIFIED”,
* the backend service can be used by Layer 4 Internal Load Balancing or Network Load Balancing
* with TCP/UDP/L3_DEFAULT Forwarding Rule protocol.
* Possible values are: `HTTP`, `HTTPS`, `HTTP2`, `TCP`, `SSL`, `GRPC`, `UNSPECIFIED`.
*/
public val protocol: Output
get() = javaResource.protocol().applyValue({ args0 -> args0 })
/**
* The security policy associated with this backend service.
*/
public val securityPolicy: Output?
get() = javaResource.securityPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The security settings that apply to this backend service. This field is applicable to either
* a regional backend service with the service_protocol set to HTTP, HTTPS, or HTTP2, and
* load_balancing_scheme set to INTERNAL_MANAGED; or a global backend service with the
* load_balancing_scheme set to INTERNAL_SELF_MANAGED.
* Structure is documented below.
*/
public val securitySettings: Output?
get() = javaResource.securitySettings().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> backendServiceSecuritySettingsToKotlin(args0) })
}).orElse(null)
})
/**
* The URI of the created resource.
*/
public val selfLink: Output
get() = javaResource.selfLink().applyValue({ args0 -> args0 })
/**
* Type of session affinity to use. The default is NONE. Session affinity is
* not applicable if the protocol is UDP.
* Possible values are: `NONE`, `CLIENT_IP`, `CLIENT_IP_PORT_PROTO`, `CLIENT_IP_PROTO`, `GENERATED_COOKIE`, `HEADER_FIELD`, `HTTP_COOKIE`.
*/
public val sessionAffinity: Output
get() = javaResource.sessionAffinity().applyValue({ args0 -> args0 })
/**
* How many seconds to wait for the backend before considering it a
* failed request. Default is 30 seconds. Valid range is [1, 86400].
*/
public val timeoutSec: Output
get() = javaResource.timeoutSec().applyValue({ args0 -> args0 })
}
public object BackendServiceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.gcp.compute.BackendService::class == javaResource::class
override fun map(javaResource: Resource): BackendService = BackendService(
javaResource as
com.pulumi.gcp.compute.BackendService,
)
}
/**
* @see [BackendService].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [BackendService].
*/
public suspend fun backendService(
name: String,
block: suspend BackendServiceResourceBuilder.() -> Unit,
): BackendService {
val builder = BackendServiceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [BackendService].
* @param name The _unique_ name of the resulting resource.
*/
public fun backendService(name: String): BackendService {
val builder = BackendServiceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy