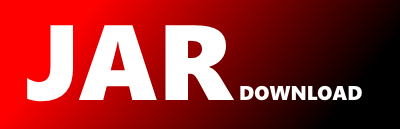
com.pulumi.gcp.compute.kotlin.FirewallPolicyRuleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.FirewallPolicyRuleArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.FirewallPolicyRuleMatchArgs
import com.pulumi.gcp.compute.kotlin.inputs.FirewallPolicyRuleMatchArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* The Compute FirewallPolicyRule resource
* ## Example Usage
* ### Basic_fir_sec_rule
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const basicGlobalNetworksecurityAddressGroup = new gcp.networksecurity.AddressGroup("basic_global_networksecurity_address_group", {
* name: "policy",
* parent: "organizations/123456789",
* description: "Sample global networksecurity_address_group",
* location: "global",
* items: ["208.80.154.224/32"],
* type: "IPV4",
* capacity: 100,
* });
* const folder = new gcp.organizations.Folder("folder", {
* displayName: "policy",
* parent: "organizations/123456789",
* });
* const _default = new gcp.compute.FirewallPolicy("default", {
* parent: folder.id,
* shortName: "policy",
* description: "Resource created for Terraform acceptance testing",
* });
* const primary = new gcp.compute.FirewallPolicyRule("primary", {
* firewallPolicy: _default.name,
* description: "Resource created for Terraform acceptance testing",
* priority: 9000,
* enableLogging: true,
* action: "allow",
* direction: "EGRESS",
* disabled: false,
* match: {
* layer4Configs: [
* {
* ipProtocol: "tcp",
* ports: ["8080"],
* },
* {
* ipProtocol: "udp",
* ports: ["22"],
* },
* ],
* destIpRanges: ["11.100.0.1/32"],
* destFqdns: [],
* destRegionCodes: ["US"],
* destThreatIntelligences: ["iplist-known-malicious-ips"],
* srcAddressGroups: [],
* destAddressGroups: [basicGlobalNetworksecurityAddressGroup.id],
* },
* targetServiceAccounts: ["my@service-account.com"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* basic_global_networksecurity_address_group = gcp.networksecurity.AddressGroup("basic_global_networksecurity_address_group",
* name="policy",
* parent="organizations/123456789",
* description="Sample global networksecurity_address_group",
* location="global",
* items=["208.80.154.224/32"],
* type="IPV4",
* capacity=100)
* folder = gcp.organizations.Folder("folder",
* display_name="policy",
* parent="organizations/123456789")
* default = gcp.compute.FirewallPolicy("default",
* parent=folder.id,
* short_name="policy",
* description="Resource created for Terraform acceptance testing")
* primary = gcp.compute.FirewallPolicyRule("primary",
* firewall_policy=default.name,
* description="Resource created for Terraform acceptance testing",
* priority=9000,
* enable_logging=True,
* action="allow",
* direction="EGRESS",
* disabled=False,
* match=gcp.compute.FirewallPolicyRuleMatchArgs(
* layer4_configs=[
* gcp.compute.FirewallPolicyRuleMatchLayer4ConfigArgs(
* ip_protocol="tcp",
* ports=["8080"],
* ),
* gcp.compute.FirewallPolicyRuleMatchLayer4ConfigArgs(
* ip_protocol="udp",
* ports=["22"],
* ),
* ],
* dest_ip_ranges=["11.100.0.1/32"],
* dest_fqdns=[],
* dest_region_codes=["US"],
* dest_threat_intelligences=["iplist-known-malicious-ips"],
* src_address_groups=[],
* dest_address_groups=[basic_global_networksecurity_address_group.id],
* ),
* target_service_accounts=["my@service-account.com"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var basicGlobalNetworksecurityAddressGroup = new Gcp.NetworkSecurity.AddressGroup("basic_global_networksecurity_address_group", new()
* {
* Name = "policy",
* Parent = "organizations/123456789",
* Description = "Sample global networksecurity_address_group",
* Location = "global",
* Items = new[]
* {
* "208.80.154.224/32",
* },
* Type = "IPV4",
* Capacity = 100,
* });
* var folder = new Gcp.Organizations.Folder("folder", new()
* {
* DisplayName = "policy",
* Parent = "organizations/123456789",
* });
* var @default = new Gcp.Compute.FirewallPolicy("default", new()
* {
* Parent = folder.Id,
* ShortName = "policy",
* Description = "Resource created for Terraform acceptance testing",
* });
* var primary = new Gcp.Compute.FirewallPolicyRule("primary", new()
* {
* FirewallPolicy = @default.Name,
* Description = "Resource created for Terraform acceptance testing",
* Priority = 9000,
* EnableLogging = true,
* Action = "allow",
* Direction = "EGRESS",
* Disabled = false,
* Match = new Gcp.Compute.Inputs.FirewallPolicyRuleMatchArgs
* {
* Layer4Configs = new[]
* {
* new Gcp.Compute.Inputs.FirewallPolicyRuleMatchLayer4ConfigArgs
* {
* IpProtocol = "tcp",
* Ports = new[]
* {
* "8080",
* },
* },
* new Gcp.Compute.Inputs.FirewallPolicyRuleMatchLayer4ConfigArgs
* {
* IpProtocol = "udp",
* Ports = new[]
* {
* "22",
* },
* },
* },
* DestIpRanges = new[]
* {
* "11.100.0.1/32",
* },
* DestFqdns = new() { },
* DestRegionCodes = new[]
* {
* "US",
* },
* DestThreatIntelligences = new[]
* {
* "iplist-known-malicious-ips",
* },
* SrcAddressGroups = new() { },
* DestAddressGroups = new[]
* {
* basicGlobalNetworksecurityAddressGroup.Id,
* },
* },
* TargetServiceAccounts = new[]
* {
* "[email protected]",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/networksecurity"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* basicGlobalNetworksecurityAddressGroup, err := networksecurity.NewAddressGroup(ctx, "basic_global_networksecurity_address_group", &networksecurity.AddressGroupArgs{
* Name: pulumi.String("policy"),
* Parent: pulumi.String("organizations/123456789"),
* Description: pulumi.String("Sample global networksecurity_address_group"),
* Location: pulumi.String("global"),
* Items: pulumi.StringArray{
* pulumi.String("208.80.154.224/32"),
* },
* Type: pulumi.String("IPV4"),
* Capacity: pulumi.Int(100),
* })
* if err != nil {
* return err
* }
* folder, err := organizations.NewFolder(ctx, "folder", &organizations.FolderArgs{
* DisplayName: pulumi.String("policy"),
* Parent: pulumi.String("organizations/123456789"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewFirewallPolicy(ctx, "default", &compute.FirewallPolicyArgs{
* Parent: folder.ID(),
* ShortName: pulumi.String("policy"),
* Description: pulumi.String("Resource created for Terraform acceptance testing"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewFirewallPolicyRule(ctx, "primary", &compute.FirewallPolicyRuleArgs{
* FirewallPolicy: _default.Name,
* Description: pulumi.String("Resource created for Terraform acceptance testing"),
* Priority: pulumi.Int(9000),
* EnableLogging: pulumi.Bool(true),
* Action: pulumi.String("allow"),
* Direction: pulumi.String("EGRESS"),
* Disabled: pulumi.Bool(false),
* Match: &compute.FirewallPolicyRuleMatchArgs{
* Layer4Configs: compute.FirewallPolicyRuleMatchLayer4ConfigArray{
* &compute.FirewallPolicyRuleMatchLayer4ConfigArgs{
* IpProtocol: pulumi.String("tcp"),
* Ports: pulumi.StringArray{
* pulumi.String("8080"),
* },
* },
* &compute.FirewallPolicyRuleMatchLayer4ConfigArgs{
* IpProtocol: pulumi.String("udp"),
* Ports: pulumi.StringArray{
* pulumi.String("22"),
* },
* },
* },
* DestIpRanges: pulumi.StringArray{
* pulumi.String("11.100.0.1/32"),
* },
* DestFqdns: pulumi.StringArray{},
* DestRegionCodes: pulumi.StringArray{
* pulumi.String("US"),
* },
* DestThreatIntelligences: pulumi.StringArray{
* pulumi.String("iplist-known-malicious-ips"),
* },
* SrcAddressGroups: pulumi.StringArray{},
* DestAddressGroups: pulumi.StringArray{
* basicGlobalNetworksecurityAddressGroup.ID(),
* },
* },
* TargetServiceAccounts: pulumi.StringArray{
* pulumi.String("[email protected]"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.networksecurity.AddressGroup;
* import com.pulumi.gcp.networksecurity.AddressGroupArgs;
* import com.pulumi.gcp.organizations.Folder;
* import com.pulumi.gcp.organizations.FolderArgs;
* import com.pulumi.gcp.compute.FirewallPolicy;
* import com.pulumi.gcp.compute.FirewallPolicyArgs;
* import com.pulumi.gcp.compute.FirewallPolicyRule;
* import com.pulumi.gcp.compute.FirewallPolicyRuleArgs;
* import com.pulumi.gcp.compute.inputs.FirewallPolicyRuleMatchArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var basicGlobalNetworksecurityAddressGroup = new AddressGroup("basicGlobalNetworksecurityAddressGroup", AddressGroupArgs.builder()
* .name("policy")
* .parent("organizations/123456789")
* .description("Sample global networksecurity_address_group")
* .location("global")
* .items("208.80.154.224/32")
* .type("IPV4")
* .capacity(100)
* .build());
* var folder = new Folder("folder", FolderArgs.builder()
* .displayName("policy")
* .parent("organizations/123456789")
* .build());
* var default_ = new FirewallPolicy("default", FirewallPolicyArgs.builder()
* .parent(folder.id())
* .shortName("policy")
* .description("Resource created for Terraform acceptance testing")
* .build());
* var primary = new FirewallPolicyRule("primary", FirewallPolicyRuleArgs.builder()
* .firewallPolicy(default_.name())
* .description("Resource created for Terraform acceptance testing")
* .priority(9000)
* .enableLogging(true)
* .action("allow")
* .direction("EGRESS")
* .disabled(false)
* .match(FirewallPolicyRuleMatchArgs.builder()
* .layer4Configs(
* FirewallPolicyRuleMatchLayer4ConfigArgs.builder()
* .ipProtocol("tcp")
* .ports(8080)
* .build(),
* FirewallPolicyRuleMatchLayer4ConfigArgs.builder()
* .ipProtocol("udp")
* .ports(22)
* .build())
* .destIpRanges("11.100.0.1/32")
* .destFqdns()
* .destRegionCodes("US")
* .destThreatIntelligences("iplist-known-malicious-ips")
* .srcAddressGroups()
* .destAddressGroups(basicGlobalNetworksecurityAddressGroup.id())
* .build())
* .targetServiceAccounts("[email protected]")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* basicGlobalNetworksecurityAddressGroup:
* type: gcp:networksecurity:AddressGroup
* name: basic_global_networksecurity_address_group
* properties:
* name: policy
* parent: organizations/123456789
* description: Sample global networksecurity_address_group
* location: global
* items:
* - 208.80.154.224/32
* type: IPV4
* capacity: 100
* folder:
* type: gcp:organizations:Folder
* properties:
* displayName: policy
* parent: organizations/123456789
* default:
* type: gcp:compute:FirewallPolicy
* properties:
* parent: ${folder.id}
* shortName: policy
* description: Resource created for Terraform acceptance testing
* primary:
* type: gcp:compute:FirewallPolicyRule
* properties:
* firewallPolicy: ${default.name}
* description: Resource created for Terraform acceptance testing
* priority: 9000
* enableLogging: true
* action: allow
* direction: EGRESS
* disabled: false
* match:
* layer4Configs:
* - ipProtocol: tcp
* ports:
* - 8080
* - ipProtocol: udp
* ports:
* - 22
* destIpRanges:
* - 11.100.0.1/32
* destFqdns: []
* destRegionCodes:
* - US
* destThreatIntelligences:
* - iplist-known-malicious-ips
* srcAddressGroups: []
* destAddressGroups:
* - ${basicGlobalNetworksecurityAddressGroup.id}
* targetServiceAccounts:
* - [email protected]
* ```
*
* ## Import
* FirewallPolicyRule can be imported using any of these accepted formats:
* * `locations/global/firewallPolicies/{{firewall_policy}}/rules/{{priority}}`
* * `{{firewall_policy}}/{{priority}}`
* When using the `pulumi import` command, FirewallPolicyRule can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/firewallPolicyRule:FirewallPolicyRule default locations/global/firewallPolicies/{{firewall_policy}}/rules/{{priority}}
* ```
* ```sh
* $ pulumi import gcp:compute/firewallPolicyRule:FirewallPolicyRule default {{firewall_policy}}/{{priority}}
* ```
* @property action The Action to perform when the client connection triggers the rule. Valid actions are "allow", "deny", "goto_next" and "apply_security_profile_group".
* @property description An optional description for this resource.
* @property direction The direction in which this rule applies. Possible values: INGRESS, EGRESS
* @property disabled Denotes whether the firewall policy rule is disabled. When set to true, the firewall policy rule is not enforced and
* traffic behaves as if it did not exist. If this is unspecified, the firewall policy rule will be enabled.
* @property enableLogging Denotes whether to enable logging for a particular rule. If logging is enabled, logs will be exported to the configured
* export destination in Stackdriver. Logs may be exported to BigQuery or Pub/Sub. Note: you cannot enable logging on
* "goto_next" rules.
* @property firewallPolicy The firewall policy of the resource.
* @property match A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
* @property priority An integer indicating the priority of a rule in the list. The priority must be a positive value between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the highest priority and 2147483647 is the lowest prority.
* @property securityProfileGroup A fully-qualified URL of a SecurityProfileGroup resource. Example:
* https://networksecurity.googleapis.com/v1/organizations/{organizationId}/locations/global/securityProfileGroups/my-security-profile-group.
* It must be specified if action = 'apply_security_profile_group' and cannot be specified for other actions.
* @property targetResources A list of network resource URLs to which this rule applies. This field allows you to control which network's VMs get
* this rule. If this field is left blank, all VMs within the organization will receive the rule.
* @property targetServiceAccounts A list of service accounts indicating the sets of instances that are applied with this rule.
* @property tlsInspect Boolean flag indicating if the traffic should be TLS decrypted. It can be set only if action =
* 'apply_security_profile_group' and cannot be set for other actions.
*/
public data class FirewallPolicyRuleArgs(
public val action: Output? = null,
public val description: Output? = null,
public val direction: Output? = null,
public val disabled: Output? = null,
public val enableLogging: Output? = null,
public val firewallPolicy: Output? = null,
public val match: Output? = null,
public val priority: Output? = null,
public val securityProfileGroup: Output? = null,
public val targetResources: Output>? = null,
public val targetServiceAccounts: Output>? = null,
public val tlsInspect: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.FirewallPolicyRuleArgs =
com.pulumi.gcp.compute.FirewallPolicyRuleArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.direction(direction?.applyValue({ args0 -> args0 }))
.disabled(disabled?.applyValue({ args0 -> args0 }))
.enableLogging(enableLogging?.applyValue({ args0 -> args0 }))
.firewallPolicy(firewallPolicy?.applyValue({ args0 -> args0 }))
.match(match?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.priority(priority?.applyValue({ args0 -> args0 }))
.securityProfileGroup(securityProfileGroup?.applyValue({ args0 -> args0 }))
.targetResources(targetResources?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.targetServiceAccounts(targetServiceAccounts?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tlsInspect(tlsInspect?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FirewallPolicyRuleArgs].
*/
@PulumiTagMarker
public class FirewallPolicyRuleArgsBuilder internal constructor() {
private var action: Output? = null
private var description: Output? = null
private var direction: Output? = null
private var disabled: Output? = null
private var enableLogging: Output? = null
private var firewallPolicy: Output? = null
private var match: Output? = null
private var priority: Output? = null
private var securityProfileGroup: Output? = null
private var targetResources: Output>? = null
private var targetServiceAccounts: Output>? = null
private var tlsInspect: Output? = null
/**
* @param value The Action to perform when the client connection triggers the rule. Valid actions are "allow", "deny", "goto_next" and "apply_security_profile_group".
*/
@JvmName("ypwpcigekwgskvwl")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value An optional description for this resource.
*/
@JvmName("trshnwgbbnxybfio")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The direction in which this rule applies. Possible values: INGRESS, EGRESS
*/
@JvmName("qvcyuyjjrtmbnisy")
public suspend fun direction(`value`: Output) {
this.direction = value
}
/**
* @param value Denotes whether the firewall policy rule is disabled. When set to true, the firewall policy rule is not enforced and
* traffic behaves as if it did not exist. If this is unspecified, the firewall policy rule will be enabled.
*/
@JvmName("girwsyfaktulmxyo")
public suspend fun disabled(`value`: Output) {
this.disabled = value
}
/**
* @param value Denotes whether to enable logging for a particular rule. If logging is enabled, logs will be exported to the configured
* export destination in Stackdriver. Logs may be exported to BigQuery or Pub/Sub. Note: you cannot enable logging on
* "goto_next" rules.
*/
@JvmName("fuctcgsrhxqrdhck")
public suspend fun enableLogging(`value`: Output) {
this.enableLogging = value
}
/**
* @param value The firewall policy of the resource.
*/
@JvmName("wbjmxgkkpjhwwyyi")
public suspend fun firewallPolicy(`value`: Output) {
this.firewallPolicy = value
}
/**
* @param value A match condition that incoming traffic is evaluated against. If it evaluates to true, the corresponding 'action' is enforced.
*/
@JvmName("omeycifpnnnappru")
public suspend fun match(`value`: Output) {
this.match = value
}
/**
* @param value An integer indicating the priority of a rule in the list. The priority must be a positive value between 0 and 2147483647. Rules are evaluated from highest to lowest priority where 0 is the highest priority and 2147483647 is the lowest prority.
*/
@JvmName("ahgtibotqcflnywy")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value A fully-qualified URL of a SecurityProfileGroup resource. Example:
* https://networksecurity.googleapis.com/v1/organizations/{organizationId}/locations/global/securityProfileGroups/my-security-profile-group.
* It must be specified if action = 'apply_security_profile_group' and cannot be specified for other actions.
*/
@JvmName("tspvucghteghtrqa")
public suspend fun securityProfileGroup(`value`: Output) {
this.securityProfileGroup = value
}
/**
* @param value A list of network resource URLs to which this rule applies. This field allows you to control which network's VMs get
* this rule. If this field is left blank, all VMs within the organization will receive the rule.
*/
@JvmName("rkwnokukrhwqhkfg")
public suspend fun targetResources(`value`: Output>) {
this.targetResources = value
}
@JvmName("cbgnqqrmosxxyhgd")
public suspend fun targetResources(vararg values: Output) {
this.targetResources = Output.all(values.asList())
}
/**
* @param values A list of network resource URLs to which this rule applies. This field allows you to control which network's VMs get
* this rule. If this field is left blank, all VMs within the organization will receive the rule.
*/
@JvmName("ovqxybtmsravnyyd")
public suspend fun targetResources(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy