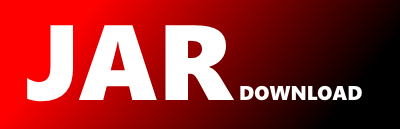
com.pulumi.gcp.compute.kotlin.ImageArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.ImageArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.ImageGuestOsFeatureArgs
import com.pulumi.gcp.compute.kotlin.inputs.ImageGuestOsFeatureArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.ImageImageEncryptionKeyArgs
import com.pulumi.gcp.compute.kotlin.inputs.ImageImageEncryptionKeyArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.ImageRawDiskArgs
import com.pulumi.gcp.compute.kotlin.inputs.ImageRawDiskArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Represents an Image resource.
* Google Compute Engine uses operating system images to create the root
* persistent disks for your instances. You specify an image when you create
* an instance. Images contain a boot loader, an operating system, and a
* root file system. Linux operating system images are also capable of
* running containers on Compute Engine.
* Images can be either public or custom.
* Public images are provided and maintained by Google, open-source
* communities, and third-party vendors. By default, all projects have
* access to these images and can use them to create instances. Custom
* images are available only to your project. You can create a custom image
* from root persistent disks and other images. Then, use the custom image
* to create an instance.
* To get more information about Image, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/v1/images)
* * How-to Guides
* * [Official Documentation](https://cloud.google.com/compute/docs/images)
* ## Example Usage
* ### Image Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.compute.Image("example", {
* name: "example-image",
* rawDisk: {
* source: "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.compute.Image("example",
* name="example-image",
* raw_disk=gcp.compute.ImageRawDiskArgs(
* source="https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.Compute.Image("example", new()
* {
* Name = "example-image",
* RawDisk = new Gcp.Compute.Inputs.ImageRawDiskArgs
* {
* Source = "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewImage(ctx, "example", &compute.ImageArgs{
* Name: pulumi.String("example-image"),
* RawDisk: &compute.ImageRawDiskArgs{
* Source: pulumi.String("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Image;
* import com.pulumi.gcp.compute.ImageArgs;
* import com.pulumi.gcp.compute.inputs.ImageRawDiskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Image("example", ImageArgs.builder()
* .name("example-image")
* .rawDisk(ImageRawDiskArgs.builder()
* .source("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:compute:Image
* properties:
* name: example-image
* rawDisk:
* source: https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz
* ```
*
* ### Image Guest Os
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.compute.Image("example", {
* name: "example-image",
* rawDisk: {
* source: "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* guestOsFeatures: [
* {
* type: "SECURE_BOOT",
* },
* {
* type: "MULTI_IP_SUBNET",
* },
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.compute.Image("example",
* name="example-image",
* raw_disk=gcp.compute.ImageRawDiskArgs(
* source="https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* ),
* guest_os_features=[
* gcp.compute.ImageGuestOsFeatureArgs(
* type="SECURE_BOOT",
* ),
* gcp.compute.ImageGuestOsFeatureArgs(
* type="MULTI_IP_SUBNET",
* ),
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.Compute.Image("example", new()
* {
* Name = "example-image",
* RawDisk = new Gcp.Compute.Inputs.ImageRawDiskArgs
* {
* Source = "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* GuestOsFeatures = new[]
* {
* new Gcp.Compute.Inputs.ImageGuestOsFeatureArgs
* {
* Type = "SECURE_BOOT",
* },
* new Gcp.Compute.Inputs.ImageGuestOsFeatureArgs
* {
* Type = "MULTI_IP_SUBNET",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewImage(ctx, "example", &compute.ImageArgs{
* Name: pulumi.String("example-image"),
* RawDisk: &compute.ImageRawDiskArgs{
* Source: pulumi.String("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz"),
* },
* GuestOsFeatures: compute.ImageGuestOsFeatureArray{
* &compute.ImageGuestOsFeatureArgs{
* Type: pulumi.String("SECURE_BOOT"),
* },
* &compute.ImageGuestOsFeatureArgs{
* Type: pulumi.String("MULTI_IP_SUBNET"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Image;
* import com.pulumi.gcp.compute.ImageArgs;
* import com.pulumi.gcp.compute.inputs.ImageRawDiskArgs;
* import com.pulumi.gcp.compute.inputs.ImageGuestOsFeatureArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Image("example", ImageArgs.builder()
* .name("example-image")
* .rawDisk(ImageRawDiskArgs.builder()
* .source("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz")
* .build())
* .guestOsFeatures(
* ImageGuestOsFeatureArgs.builder()
* .type("SECURE_BOOT")
* .build(),
* ImageGuestOsFeatureArgs.builder()
* .type("MULTI_IP_SUBNET")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:compute:Image
* properties:
* name: example-image
* rawDisk:
* source: https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz
* guestOsFeatures:
* - type: SECURE_BOOT
* - type: MULTI_IP_SUBNET
* ```
*
* ### Image Basic Storage Location
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const example = new gcp.compute.Image("example", {
* name: "example-sl-image",
* rawDisk: {
* source: "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* storageLocations: ["us-central1"],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* example = gcp.compute.Image("example",
* name="example-sl-image",
* raw_disk=gcp.compute.ImageRawDiskArgs(
* source="https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* ),
* storage_locations=["us-central1"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var example = new Gcp.Compute.Image("example", new()
* {
* Name = "example-sl-image",
* RawDisk = new Gcp.Compute.Inputs.ImageRawDiskArgs
* {
* Source = "https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz",
* },
* StorageLocations = new[]
* {
* "us-central1",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewImage(ctx, "example", &compute.ImageArgs{
* Name: pulumi.String("example-sl-image"),
* RawDisk: &compute.ImageRawDiskArgs{
* Source: pulumi.String("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz"),
* },
* StorageLocations: pulumi.StringArray{
* pulumi.String("us-central1"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Image;
* import com.pulumi.gcp.compute.ImageArgs;
* import com.pulumi.gcp.compute.inputs.ImageRawDiskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new Image("example", ImageArgs.builder()
* .name("example-sl-image")
* .rawDisk(ImageRawDiskArgs.builder()
* .source("https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz")
* .build())
* .storageLocations("us-central1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: gcp:compute:Image
* properties:
* name: example-sl-image
* rawDisk:
* source: https://storage.googleapis.com/bosh-gce-raw-stemcells/bosh-stemcell-97.98-google-kvm-ubuntu-xenial-go_agent-raw-1557960142.tar.gz
* storageLocations:
* - us-central1
* ```
*
* ## Import
* Image can be imported using any of these accepted formats:
* * `projects/{{project}}/global/images/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Image can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/image:Image default projects/{{project}}/global/images/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/image:Image default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/image:Image default {{name}}
* ```
* @property description An optional description of this resource. Provide this property when
* you create the resource.
* @property diskSizeGb Size of the image when restored onto a persistent disk (in GB).
* @property family The name of the image family to which this image belongs. You can
* create disks by specifying an image family instead of a specific
* image name. The image family always returns its latest image that is
* not deprecated. The name of the image family must comply with
* RFC1035.
* @property guestOsFeatures A list of features to enable on the guest operating system.
* Applicable only for bootable images.
* Structure is documented below.
* @property imageEncryptionKey Encrypts the image using a customer-supplied encryption key.
* After you encrypt an image with a customer-supplied key, you must
* provide the same key if you use the image later (e.g. to create a
* disk from the image)
* Structure is documented below.
* @property labels Labels to apply to this Image.
* **Note**: This field is non-authoritative, and will only manage the labels present in your configuration.
* Please refer to the field `effective_labels` for all of the labels present on the resource.
* @property licenses Any applicable license URI.
* @property name Name of the resource; provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and
* match the regular expression `a-z?` which means
* the first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* - - -
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property rawDisk The parameters of the raw disk image.
* Structure is documented below.
* @property sourceDisk The source disk to create this image based on.
* You must provide either this property or the
* rawDisk.source property but not both to create an image.
* @property sourceImage URL of the source image used to create this image. In order to create an image, you must provide the full or partial
* URL of one of the following:
* * The selfLink URL
* * This property
* * The rawDisk.source URL
* * The sourceDisk URL
* @property sourceSnapshot URL of the source snapshot used to create this image.
* In order to create an image, you must provide the full or partial URL of one of the following:
* * The selfLink URL
* * This property
* * The sourceImage URL
* * The rawDisk.source URL
* * The sourceDisk URL
* @property storageLocations Cloud Storage bucket storage location of the image
* (regional or multi-regional).
* Reference link: https://cloud.google.com/compute/docs/reference/rest/v1/images
*/
public data class ImageArgs(
public val description: Output? = null,
public val diskSizeGb: Output? = null,
public val family: Output? = null,
public val guestOsFeatures: Output>? = null,
public val imageEncryptionKey: Output? = null,
public val labels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy