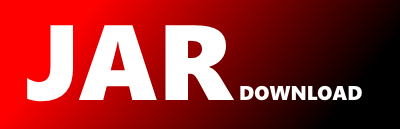
com.pulumi.gcp.compute.kotlin.Instance.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.gcp.compute.kotlin.outputs.InstanceAdvancedMachineFeatures
import com.pulumi.gcp.compute.kotlin.outputs.InstanceAttachedDisk
import com.pulumi.gcp.compute.kotlin.outputs.InstanceBootDisk
import com.pulumi.gcp.compute.kotlin.outputs.InstanceConfidentialInstanceConfig
import com.pulumi.gcp.compute.kotlin.outputs.InstanceGuestAccelerator
import com.pulumi.gcp.compute.kotlin.outputs.InstanceNetworkInterface
import com.pulumi.gcp.compute.kotlin.outputs.InstanceNetworkPerformanceConfig
import com.pulumi.gcp.compute.kotlin.outputs.InstanceParams
import com.pulumi.gcp.compute.kotlin.outputs.InstanceReservationAffinity
import com.pulumi.gcp.compute.kotlin.outputs.InstanceScheduling
import com.pulumi.gcp.compute.kotlin.outputs.InstanceScratchDisk
import com.pulumi.gcp.compute.kotlin.outputs.InstanceServiceAccount
import com.pulumi.gcp.compute.kotlin.outputs.InstanceShieldedInstanceConfig
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.gcp.compute.kotlin.outputs.InstanceAdvancedMachineFeatures.Companion.toKotlin as instanceAdvancedMachineFeaturesToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceAttachedDisk.Companion.toKotlin as instanceAttachedDiskToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceBootDisk.Companion.toKotlin as instanceBootDiskToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceConfidentialInstanceConfig.Companion.toKotlin as instanceConfidentialInstanceConfigToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceGuestAccelerator.Companion.toKotlin as instanceGuestAcceleratorToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceNetworkInterface.Companion.toKotlin as instanceNetworkInterfaceToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceNetworkPerformanceConfig.Companion.toKotlin as instanceNetworkPerformanceConfigToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceParams.Companion.toKotlin as instanceParamsToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceReservationAffinity.Companion.toKotlin as instanceReservationAffinityToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceScheduling.Companion.toKotlin as instanceSchedulingToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceScratchDisk.Companion.toKotlin as instanceScratchDiskToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceServiceAccount.Companion.toKotlin as instanceServiceAccountToKotlin
import com.pulumi.gcp.compute.kotlin.outputs.InstanceShieldedInstanceConfig.Companion.toKotlin as instanceShieldedInstanceConfigToKotlin
/**
* Builder for [Instance].
*/
@PulumiTagMarker
public class InstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InstanceArgs = InstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InstanceArgsBuilder.() -> Unit) {
val builder = InstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Instance {
val builtJavaResource = com.pulumi.gcp.compute.Instance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Instance(builtJavaResource)
}
}
/**
* Manages a VM instance resource within GCE. For more information see
* [the official documentation](https://cloud.google.com/compute/docs/instances)
* and
* [API](https://cloud.google.com/compute/docs/reference/latest/instances).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.serviceaccount.Account("default", {
* accountId: "my-custom-sa",
* displayName: "Custom SA for VM Instance",
* });
* const defaultInstance = new gcp.compute.Instance("default", {
* networkInterfaces: [{
* accessConfigs: [{}],
* network: "default",
* }],
* name: "my-instance",
* machineType: "n2-standard-2",
* zone: "us-central1-a",
* tags: [
* "foo",
* "bar",
* ],
* bootDisk: {
* initializeParams: {
* image: "debian-cloud/debian-11",
* labels: {
* my_label: "value",
* },
* },
* },
* scratchDisks: [{
* "interface": "NVME",
* }],
* metadata: {
* foo: "bar",
* },
* metadataStartupScript: "echo hi > /test.txt",
* serviceAccount: {
* email: _default.email,
* scopes: ["cloud-platform"],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.serviceaccount.Account("default",
* account_id="my-custom-sa",
* display_name="Custom SA for VM Instance")
* default_instance = gcp.compute.Instance("default",
* network_interfaces=[gcp.compute.InstanceNetworkInterfaceArgs(
* access_configs=[gcp.compute.InstanceNetworkInterfaceAccessConfigArgs()],
* network="default",
* )],
* name="my-instance",
* machine_type="n2-standard-2",
* zone="us-central1-a",
* tags=[
* "foo",
* "bar",
* ],
* boot_disk=gcp.compute.InstanceBootDiskArgs(
* initialize_params=gcp.compute.InstanceBootDiskInitializeParamsArgs(
* image="debian-cloud/debian-11",
* labels={
* "my_label": "value",
* },
* ),
* ),
* scratch_disks=[gcp.compute.InstanceScratchDiskArgs(
* interface="NVME",
* )],
* metadata={
* "foo": "bar",
* },
* metadata_startup_script="echo hi > /test.txt",
* service_account=gcp.compute.InstanceServiceAccountArgs(
* email=default.email,
* scopes=["cloud-platform"],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.ServiceAccount.Account("default", new()
* {
* AccountId = "my-custom-sa",
* DisplayName = "Custom SA for VM Instance",
* });
* var defaultInstance = new Gcp.Compute.Instance("default", new()
* {
* NetworkInterfaces = new[]
* {
* new Gcp.Compute.Inputs.InstanceNetworkInterfaceArgs
* {
* AccessConfigs = new[]
* {
* null,
* },
* Network = "default",
* },
* },
* Name = "my-instance",
* MachineType = "n2-standard-2",
* Zone = "us-central1-a",
* Tags = new[]
* {
* "foo",
* "bar",
* },
* BootDisk = new Gcp.Compute.Inputs.InstanceBootDiskArgs
* {
* InitializeParams = new Gcp.Compute.Inputs.InstanceBootDiskInitializeParamsArgs
* {
* Image = "debian-cloud/debian-11",
* Labels =
* {
* { "my_label", "value" },
* },
* },
* },
* ScratchDisks = new[]
* {
* new Gcp.Compute.Inputs.InstanceScratchDiskArgs
* {
* Interface = "NVME",
* },
* },
* Metadata =
* {
* { "foo", "bar" },
* },
* MetadataStartupScript = "echo hi > /test.txt",
* ServiceAccount = new Gcp.Compute.Inputs.InstanceServiceAccountArgs
* {
* Email = @default.Email,
* Scopes = new[]
* {
* "cloud-platform",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/serviceaccount"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := serviceaccount.NewAccount(ctx, "default", &serviceaccount.AccountArgs{
* AccountId: pulumi.String("my-custom-sa"),
* DisplayName: pulumi.String("Custom SA for VM Instance"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewInstance(ctx, "default", &compute.InstanceArgs{
* NetworkInterfaces: compute.InstanceNetworkInterfaceArray{
* &compute.InstanceNetworkInterfaceArgs{
* AccessConfigs: compute.InstanceNetworkInterfaceAccessConfigArray{
* nil,
* },
* Network: pulumi.String("default"),
* },
* },
* Name: pulumi.String("my-instance"),
* MachineType: pulumi.String("n2-standard-2"),
* Zone: pulumi.String("us-central1-a"),
* Tags: pulumi.StringArray{
* pulumi.String("foo"),
* pulumi.String("bar"),
* },
* BootDisk: &compute.InstanceBootDiskArgs{
* InitializeParams: &compute.InstanceBootDiskInitializeParamsArgs{
* Image: pulumi.String("debian-cloud/debian-11"),
* Labels: pulumi.Map{
* "my_label": pulumi.Any("value"),
* },
* },
* },
* ScratchDisks: compute.InstanceScratchDiskArray{
* &compute.InstanceScratchDiskArgs{
* Interface: pulumi.String("NVME"),
* },
* },
* Metadata: pulumi.StringMap{
* "foo": pulumi.String("bar"),
* },
* MetadataStartupScript: pulumi.String("echo hi > /test.txt"),
* ServiceAccount: &compute.InstanceServiceAccountArgs{
* Email: _default.Email,
* Scopes: pulumi.StringArray{
* pulumi.String("cloud-platform"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.serviceaccount.Account;
* import com.pulumi.gcp.serviceaccount.AccountArgs;
* import com.pulumi.gcp.compute.Instance;
* import com.pulumi.gcp.compute.InstanceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceNetworkInterfaceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskInitializeParamsArgs;
* import com.pulumi.gcp.compute.inputs.InstanceScratchDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceServiceAccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Account("default", AccountArgs.builder()
* .accountId("my-custom-sa")
* .displayName("Custom SA for VM Instance")
* .build());
* var defaultInstance = new Instance("defaultInstance", InstanceArgs.builder()
* .networkInterfaces(InstanceNetworkInterfaceArgs.builder()
* .accessConfigs()
* .network("default")
* .build())
* .name("my-instance")
* .machineType("n2-standard-2")
* .zone("us-central1-a")
* .tags(
* "foo",
* "bar")
* .bootDisk(InstanceBootDiskArgs.builder()
* .initializeParams(InstanceBootDiskInitializeParamsArgs.builder()
* .image("debian-cloud/debian-11")
* .labels(Map.of("my_label", "value"))
* .build())
* .build())
* .scratchDisks(InstanceScratchDiskArgs.builder()
* .interface_("NVME")
* .build())
* .metadata(Map.of("foo", "bar"))
* .metadataStartupScript("echo hi > /test.txt")
* .serviceAccount(InstanceServiceAccountArgs.builder()
* .email(default_.email())
* .scopes("cloud-platform")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* default:
* type: gcp:serviceaccount:Account
* properties:
* accountId: my-custom-sa
* displayName: Custom SA for VM Instance
* defaultInstance:
* type: gcp:compute:Instance
* name: default
* properties:
* networkInterfaces:
* - accessConfigs:
* - {}
* network: default
* name: my-instance
* machineType: n2-standard-2
* zone: us-central1-a
* tags:
* - foo
* - bar
* bootDisk:
* initializeParams:
* image: debian-cloud/debian-11
* labels:
* my_label: value
* scratchDisks:
* - interface: NVME
* metadata:
* foo: bar
* metadataStartupScript: echo hi > /test.txt
* serviceAccount:
* email: ${default.email}
* scopes:
* - cloud-platform
* ```
*
* ## Import
* Instances can be imported using any of these accepted formats:
* * `projects/{{project}}/zones/{{zone}}/instances/{{name}}`
* * `{{project}}/{{zone}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, instances can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/instance:Instance default projects/{{project}}/zones/{{zone}}/instances/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/instance:Instance default {{project}}/{{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/instance:Instance default {{name}}
* ```
*/
public class Instance internal constructor(
override val javaResource: com.pulumi.gcp.compute.Instance,
) : KotlinCustomResource(javaResource, InstanceMapper) {
/**
* Configure Nested Virtualisation and Simultaneous Hyper Threading on this VM. Structure is documented below
*/
public val advancedMachineFeatures: Output?
get() = javaResource.advancedMachineFeatures().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> instanceAdvancedMachineFeaturesToKotlin(args0) })
}).orElse(null)
})
/**
* If true, allows this prvider to stop the instance to update its properties.
* If you try to update a property that requires stopping the instance without setting this field, the update will fail.
*/
public val allowStoppingForUpdate: Output?
get() = javaResource.allowStoppingForUpdate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Additional disks to attach to the instance. Can be repeated multiple times for multiple disks. Structure is documented below.
*/
public val attachedDisks: Output>?
get() = javaResource.attachedDisks().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
instanceAttachedDiskToKotlin(args0)
})
})
}).orElse(null)
})
/**
* The boot disk for the instance.
* Structure is documented below.
*/
public val bootDisk: Output
get() = javaResource.bootDisk().applyValue({ args0 ->
args0.let({ args0 ->
instanceBootDiskToKotlin(args0)
})
})
/**
* Whether to allow sending and receiving of
* packets with non-matching source or destination IPs.
* This defaults to false.
*/
public val canIpForward: Output?
get() = javaResource.canIpForward().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Enable [Confidential Mode](https://cloud.google.com/compute/confidential-vm/docs/about-cvm) on this VM. Structure is documented below
*/
public val confidentialInstanceConfig: Output
get() = javaResource.confidentialInstanceConfig().applyValue({ args0 ->
args0.let({ args0 ->
instanceConfidentialInstanceConfigToKotlin(args0)
})
})
/**
* The CPU platform used by this instance.
*/
public val cpuPlatform: Output
get() = javaResource.cpuPlatform().applyValue({ args0 -> args0 })
/**
* The current status of the instance. This could be one of the following values: PROVISIONING, STAGING, RUNNING, STOPPING, SUSPENDING, SUSPENDED, REPAIRING, and TERMINATED. For more information about the status of the instance, see [Instance life cycle](https://cloud.google.com/compute/docs/instances/instance-life-cycle).`,
*/
public val currentStatus: Output
get() = javaResource.currentStatus().applyValue({ args0 -> args0 })
/**
* Enable deletion protection on this instance. Defaults to false.
* **Note:** you must disable deletion protection before removing the resource (e.g., via `pulumi destroy`), or the instance cannot be deleted and the provider run will not complete successfully.
*/
public val deletionProtection: Output?
get() = javaResource.deletionProtection().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* A brief description of this resource.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Desired status of the instance. Either
* `"RUNNING"` or `"TERMINATED"`.
*/
public val desiredStatus: Output?
get() = javaResource.desiredStatus().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
*/
public val effectiveLabels: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy