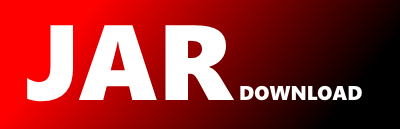
com.pulumi.gcp.compute.kotlin.InstanceGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.InstanceGroupArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.InstanceGroupNamedPortArgs
import com.pulumi.gcp.compute.kotlin.inputs.InstanceGroupNamedPortArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Creates a group of dissimilar Compute Engine virtual machine instances.
* For more information, see [the official documentation](https://cloud.google.com/compute/docs/instance-groups/#unmanaged_instance_groups)
* and [API](https://cloud.google.com/compute/docs/reference/latest/instanceGroups)
* ## Example Usage
* ### Empty Instance Group
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const test = new gcp.compute.InstanceGroup("test", {
* name: "test",
* description: "Test instance group",
* zone: "us-central1-a",
* network: _default.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* test = gcp.compute.InstanceGroup("test",
* name="test",
* description="Test instance group",
* zone="us-central1-a",
* network=default["id"])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var test = new Gcp.Compute.InstanceGroup("test", new()
* {
* Name = "test",
* Description = "Test instance group",
* Zone = "us-central1-a",
* Network = @default.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewInstanceGroup(ctx, "test", &compute.InstanceGroupArgs{
* Name: pulumi.String("test"),
* Description: pulumi.String("Test instance group"),
* Zone: pulumi.String("us-central1-a"),
* Network: pulumi.Any(_default.Id),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.InstanceGroup;
* import com.pulumi.gcp.compute.InstanceGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var test = new InstanceGroup("test", InstanceGroupArgs.builder()
* .name("test")
* .description("Test instance group")
* .zone("us-central1-a")
* .network(default_.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* test:
* type: gcp:compute:InstanceGroup
* properties:
* name: test
* description: Test instance group
* zone: us-central1-a
* network: ${default.id}
* ```
*
* ### Example Usage - With instances and named ports
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const webservers = new gcp.compute.InstanceGroup("webservers", {
* name: "webservers",
* description: "Test instance group",
* instances: [
* test.id,
* test2.id,
* ],
* namedPorts: [
* {
* name: "http",
* port: 8080,
* },
* {
* name: "https",
* port: 8443,
* },
* ],
* zone: "us-central1-a",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* webservers = gcp.compute.InstanceGroup("webservers",
* name="webservers",
* description="Test instance group",
* instances=[
* test["id"],
* test2["id"],
* ],
* named_ports=[
* gcp.compute.InstanceGroupNamedPortArgs(
* name="http",
* port=8080,
* ),
* gcp.compute.InstanceGroupNamedPortArgs(
* name="https",
* port=8443,
* ),
* ],
* zone="us-central1-a")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var webservers = new Gcp.Compute.InstanceGroup("webservers", new()
* {
* Name = "webservers",
* Description = "Test instance group",
* Instances = new[]
* {
* test.Id,
* test2.Id,
* },
* NamedPorts = new[]
* {
* new Gcp.Compute.Inputs.InstanceGroupNamedPortArgs
* {
* Name = "http",
* Port = 8080,
* },
* new Gcp.Compute.Inputs.InstanceGroupNamedPortArgs
* {
* Name = "https",
* Port = 8443,
* },
* },
* Zone = "us-central1-a",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewInstanceGroup(ctx, "webservers", &compute.InstanceGroupArgs{
* Name: pulumi.String("webservers"),
* Description: pulumi.String("Test instance group"),
* Instances: pulumi.StringArray{
* test.Id,
* test2.Id,
* },
* NamedPorts: compute.InstanceGroupNamedPortTypeArray{
* &compute.InstanceGroupNamedPortTypeArgs{
* Name: pulumi.String("http"),
* Port: pulumi.Int(8080),
* },
* &compute.InstanceGroupNamedPortTypeArgs{
* Name: pulumi.String("https"),
* Port: pulumi.Int(8443),
* },
* },
* Zone: pulumi.String("us-central1-a"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.InstanceGroup;
* import com.pulumi.gcp.compute.InstanceGroupArgs;
* import com.pulumi.gcp.compute.inputs.InstanceGroupNamedPortArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var webservers = new InstanceGroup("webservers", InstanceGroupArgs.builder()
* .name("webservers")
* .description("Test instance group")
* .instances(
* test.id(),
* test2.id())
* .namedPorts(
* InstanceGroupNamedPortArgs.builder()
* .name("http")
* .port("8080")
* .build(),
* InstanceGroupNamedPortArgs.builder()
* .name("https")
* .port("8443")
* .build())
* .zone("us-central1-a")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* webservers:
* type: gcp:compute:InstanceGroup
* properties:
* name: webservers
* description: Test instance group
* instances:
* - ${test.id}
* - ${test2.id}
* namedPorts:
* - name: http
* port: '8080'
* - name: https
* port: '8443'
* zone: us-central1-a
* ```
*
* ### Example Usage - Recreating an instance group in use
* Recreating an instance group that's in use by another resource will give a
* `resourceInUseByAnotherResource` error. Use `lifecycle.create_before_destroy`
* as shown in this example to avoid this type of error.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const debianImage = gcp.compute.getImage({
* family: "debian-11",
* project: "debian-cloud",
* });
* const stagingVm = new gcp.compute.Instance("staging_vm", {
* name: "staging-vm",
* machineType: "e2-medium",
* zone: "us-central1-c",
* bootDisk: {
* initializeParams: {
* image: debianImage.then(debianImage => debianImage.selfLink),
* },
* },
* networkInterfaces: [{
* network: "default",
* }],
* });
* const stagingGroup = new gcp.compute.InstanceGroup("staging_group", {
* name: "staging-instance-group",
* zone: "us-central1-c",
* instances: [stagingVm.id],
* namedPorts: [
* {
* name: "http",
* port: 8080,
* },
* {
* name: "https",
* port: 8443,
* },
* ],
* });
* const stagingHealth = new gcp.compute.HttpsHealthCheck("staging_health", {
* name: "staging-health",
* requestPath: "/health_check",
* });
* const stagingService = new gcp.compute.BackendService("staging_service", {
* name: "staging-service",
* portName: "https",
* protocol: "HTTPS",
* backends: [{
* group: stagingGroup.id,
* }],
* healthChecks: stagingHealth.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* debian_image = gcp.compute.get_image(family="debian-11",
* project="debian-cloud")
* staging_vm = gcp.compute.Instance("staging_vm",
* name="staging-vm",
* machine_type="e2-medium",
* zone="us-central1-c",
* boot_disk=gcp.compute.InstanceBootDiskArgs(
* initialize_params=gcp.compute.InstanceBootDiskInitializeParamsArgs(
* image=debian_image.self_link,
* ),
* ),
* network_interfaces=[gcp.compute.InstanceNetworkInterfaceArgs(
* network="default",
* )])
* staging_group = gcp.compute.InstanceGroup("staging_group",
* name="staging-instance-group",
* zone="us-central1-c",
* instances=[staging_vm.id],
* named_ports=[
* gcp.compute.InstanceGroupNamedPortArgs(
* name="http",
* port=8080,
* ),
* gcp.compute.InstanceGroupNamedPortArgs(
* name="https",
* port=8443,
* ),
* ])
* staging_health = gcp.compute.HttpsHealthCheck("staging_health",
* name="staging-health",
* request_path="/health_check")
* staging_service = gcp.compute.BackendService("staging_service",
* name="staging-service",
* port_name="https",
* protocol="HTTPS",
* backends=[gcp.compute.BackendServiceBackendArgs(
* group=staging_group.id,
* )],
* health_checks=staging_health.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var debianImage = Gcp.Compute.GetImage.Invoke(new()
* {
* Family = "debian-11",
* Project = "debian-cloud",
* });
* var stagingVm = new Gcp.Compute.Instance("staging_vm", new()
* {
* Name = "staging-vm",
* MachineType = "e2-medium",
* Zone = "us-central1-c",
* BootDisk = new Gcp.Compute.Inputs.InstanceBootDiskArgs
* {
* InitializeParams = new Gcp.Compute.Inputs.InstanceBootDiskInitializeParamsArgs
* {
* Image = debianImage.Apply(getImageResult => getImageResult.SelfLink),
* },
* },
* NetworkInterfaces = new[]
* {
* new Gcp.Compute.Inputs.InstanceNetworkInterfaceArgs
* {
* Network = "default",
* },
* },
* });
* var stagingGroup = new Gcp.Compute.InstanceGroup("staging_group", new()
* {
* Name = "staging-instance-group",
* Zone = "us-central1-c",
* Instances = new[]
* {
* stagingVm.Id,
* },
* NamedPorts = new[]
* {
* new Gcp.Compute.Inputs.InstanceGroupNamedPortArgs
* {
* Name = "http",
* Port = 8080,
* },
* new Gcp.Compute.Inputs.InstanceGroupNamedPortArgs
* {
* Name = "https",
* Port = 8443,
* },
* },
* });
* var stagingHealth = new Gcp.Compute.HttpsHealthCheck("staging_health", new()
* {
* Name = "staging-health",
* RequestPath = "/health_check",
* });
* var stagingService = new Gcp.Compute.BackendService("staging_service", new()
* {
* Name = "staging-service",
* PortName = "https",
* Protocol = "HTTPS",
* Backends = new[]
* {
* new Gcp.Compute.Inputs.BackendServiceBackendArgs
* {
* Group = stagingGroup.Id,
* },
* },
* HealthChecks = stagingHealth.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* debianImage, err := compute.LookupImage(ctx, &compute.LookupImageArgs{
* Family: pulumi.StringRef("debian-11"),
* Project: pulumi.StringRef("debian-cloud"),
* }, nil)
* if err != nil {
* return err
* }
* stagingVm, err := compute.NewInstance(ctx, "staging_vm", &compute.InstanceArgs{
* Name: pulumi.String("staging-vm"),
* MachineType: pulumi.String("e2-medium"),
* Zone: pulumi.String("us-central1-c"),
* BootDisk: &compute.InstanceBootDiskArgs{
* InitializeParams: &compute.InstanceBootDiskInitializeParamsArgs{
* Image: pulumi.String(debianImage.SelfLink),
* },
* },
* NetworkInterfaces: compute.InstanceNetworkInterfaceArray{
* &compute.InstanceNetworkInterfaceArgs{
* Network: pulumi.String("default"),
* },
* },
* })
* if err != nil {
* return err
* }
* stagingGroup, err := compute.NewInstanceGroup(ctx, "staging_group", &compute.InstanceGroupArgs{
* Name: pulumi.String("staging-instance-group"),
* Zone: pulumi.String("us-central1-c"),
* Instances: pulumi.StringArray{
* stagingVm.ID(),
* },
* NamedPorts: compute.InstanceGroupNamedPortTypeArray{
* &compute.InstanceGroupNamedPortTypeArgs{
* Name: pulumi.String("http"),
* Port: pulumi.Int(8080),
* },
* &compute.InstanceGroupNamedPortTypeArgs{
* Name: pulumi.String("https"),
* Port: pulumi.Int(8443),
* },
* },
* })
* if err != nil {
* return err
* }
* stagingHealth, err := compute.NewHttpsHealthCheck(ctx, "staging_health", &compute.HttpsHealthCheckArgs{
* Name: pulumi.String("staging-health"),
* RequestPath: pulumi.String("/health_check"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "staging_service", &compute.BackendServiceArgs{
* Name: pulumi.String("staging-service"),
* PortName: pulumi.String("https"),
* Protocol: pulumi.String("HTTPS"),
* Backends: compute.BackendServiceBackendArray{
* &compute.BackendServiceBackendArgs{
* Group: stagingGroup.ID(),
* },
* },
* HealthChecks: stagingHealth.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.ComputeFunctions;
* import com.pulumi.gcp.compute.inputs.GetImageArgs;
* import com.pulumi.gcp.compute.Instance;
* import com.pulumi.gcp.compute.InstanceArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskArgs;
* import com.pulumi.gcp.compute.inputs.InstanceBootDiskInitializeParamsArgs;
* import com.pulumi.gcp.compute.inputs.InstanceNetworkInterfaceArgs;
* import com.pulumi.gcp.compute.InstanceGroup;
* import com.pulumi.gcp.compute.InstanceGroupArgs;
* import com.pulumi.gcp.compute.inputs.InstanceGroupNamedPortArgs;
* import com.pulumi.gcp.compute.HttpsHealthCheck;
* import com.pulumi.gcp.compute.HttpsHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.inputs.BackendServiceBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var debianImage = ComputeFunctions.getImage(GetImageArgs.builder()
* .family("debian-11")
* .project("debian-cloud")
* .build());
* var stagingVm = new Instance("stagingVm", InstanceArgs.builder()
* .name("staging-vm")
* .machineType("e2-medium")
* .zone("us-central1-c")
* .bootDisk(InstanceBootDiskArgs.builder()
* .initializeParams(InstanceBootDiskInitializeParamsArgs.builder()
* .image(debianImage.applyValue(getImageResult -> getImageResult.selfLink()))
* .build())
* .build())
* .networkInterfaces(InstanceNetworkInterfaceArgs.builder()
* .network("default")
* .build())
* .build());
* var stagingGroup = new InstanceGroup("stagingGroup", InstanceGroupArgs.builder()
* .name("staging-instance-group")
* .zone("us-central1-c")
* .instances(stagingVm.id())
* .namedPorts(
* InstanceGroupNamedPortArgs.builder()
* .name("http")
* .port("8080")
* .build(),
* InstanceGroupNamedPortArgs.builder()
* .name("https")
* .port("8443")
* .build())
* .build());
* var stagingHealth = new HttpsHealthCheck("stagingHealth", HttpsHealthCheckArgs.builder()
* .name("staging-health")
* .requestPath("/health_check")
* .build());
* var stagingService = new BackendService("stagingService", BackendServiceArgs.builder()
* .name("staging-service")
* .portName("https")
* .protocol("HTTPS")
* .backends(BackendServiceBackendArgs.builder()
* .group(stagingGroup.id())
* .build())
* .healthChecks(stagingHealth.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* stagingGroup:
* type: gcp:compute:InstanceGroup
* name: staging_group
* properties:
* name: staging-instance-group
* zone: us-central1-c
* instances:
* - ${stagingVm.id}
* namedPorts:
* - name: http
* port: '8080'
* - name: https
* port: '8443'
* stagingVm:
* type: gcp:compute:Instance
* name: staging_vm
* properties:
* name: staging-vm
* machineType: e2-medium
* zone: us-central1-c
* bootDisk:
* initializeParams:
* image: ${debianImage.selfLink}
* networkInterfaces:
* - network: default
* stagingService:
* type: gcp:compute:BackendService
* name: staging_service
* properties:
* name: staging-service
* portName: https
* protocol: HTTPS
* backends:
* - group: ${stagingGroup.id}
* healthChecks: ${stagingHealth.id}
* stagingHealth:
* type: gcp:compute:HttpsHealthCheck
* name: staging_health
* properties:
* name: staging-health
* requestPath: /health_check
* variables:
* debianImage:
* fn::invoke:
* Function: gcp:compute:getImage
* Arguments:
* family: debian-11
* project: debian-cloud
* ```
*
* ## Import
* Instance groups can be imported using the `zone` and `name` with an optional `project`, e.g.
* * `projects/{{project_id}}/zones/{{zone}}/instanceGroups/{{instance_group_id}}`
* * `{{project_id}}/{{zone}}/{{instance_group_id}}`
* * `{{zone}}/{{instance_group_id}}`
* When using the `pulumi import` command, instance groups can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/instanceGroup:InstanceGroup default {{zone}}/{{instance_group_id}}
* ```
* ```sh
* $ pulumi import gcp:compute/instanceGroup:InstanceGroup default {{project_id}}/{{zone}}/{{instance_group_id}}
* ```
* ```sh
* $ pulumi import gcp:compute/instanceGroup:InstanceGroup default projects/{{project_id}}/zones/{{zone}}/instanceGroups/{{instance_group_id}}
* ```
* @property description An optional textual description of the instance
* group.
* @property instances The list of instances in the group, in `self_link` format.
* When adding instances they must all be in the same network and zone as the instance group.
* @property name The name of the instance group. Must be 1-63
* characters long and comply with
* [RFC1035](https://www.ietf.org/rfc/rfc1035.txt). Supported characters
* include lowercase letters, numbers, and hyphens.
* @property namedPorts The named port configuration. See the section below
* for details on configuration. Structure is documented below.
* @property network The URL of the network the instance group is in. If
* this is different from the network where the instances are in, the creation
* fails. Defaults to the network where the instances are in (if neither
* `network` nor `instances` is specified, this field will be blank).
* @property project The ID of the project in which the resource belongs. If it
* is not provided, the provider project is used.
* @property zone The zone that this instance group should be created in.
* - - -
*/
public data class InstanceGroupArgs(
public val description: Output? = null,
public val instances: Output>? = null,
public val name: Output? = null,
public val namedPorts: Output>? = null,
public val network: Output? = null,
public val project: Output? = null,
public val zone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.InstanceGroupArgs =
com.pulumi.gcp.compute.InstanceGroupArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.instances(instances?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.name(name?.applyValue({ args0 -> args0 }))
.namedPorts(
namedPorts?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.network(network?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.zone(zone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceGroupArgs].
*/
@PulumiTagMarker
public class InstanceGroupArgsBuilder internal constructor() {
private var description: Output? = null
private var instances: Output>? = null
private var name: Output? = null
private var namedPorts: Output>? = null
private var network: Output? = null
private var project: Output? = null
private var zone: Output? = null
/**
* @param value An optional textual description of the instance
* group.
*/
@JvmName("enlhfhanjvworxxn")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The list of instances in the group, in `self_link` format.
* When adding instances they must all be in the same network and zone as the instance group.
*/
@JvmName("lvbhhxsqhqseaclf")
public suspend fun instances(`value`: Output>) {
this.instances = value
}
@JvmName("holvouxqyybnmona")
public suspend fun instances(vararg values: Output) {
this.instances = Output.all(values.asList())
}
/**
* @param values The list of instances in the group, in `self_link` format.
* When adding instances they must all be in the same network and zone as the instance group.
*/
@JvmName("bqkywxsntqtdartw")
public suspend fun instances(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy