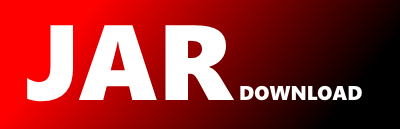
com.pulumi.gcp.compute.kotlin.NetworkPeeringArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.NetworkPeeringArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a network peering within GCE. For more information see
* [the official documentation](https://cloud.google.com/compute/docs/vpc/vpc-peering)
* and
* [API](https://cloud.google.com/compute/docs/reference/latest/networks).
* > Both networks must create a peering with each other for the peering
* to be functional.
* > Subnets IP ranges across peered VPC networks cannot overlap.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.Network("default", {
* name: "foobar",
* autoCreateSubnetworks: false,
* });
* const other = new gcp.compute.Network("other", {
* name: "other",
* autoCreateSubnetworks: false,
* });
* const peering1 = new gcp.compute.NetworkPeering("peering1", {
* name: "peering1",
* network: _default.selfLink,
* peerNetwork: other.selfLink,
* });
* const peering2 = new gcp.compute.NetworkPeering("peering2", {
* name: "peering2",
* network: other.selfLink,
* peerNetwork: _default.selfLink,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.Network("default",
* name="foobar",
* auto_create_subnetworks=False)
* other = gcp.compute.Network("other",
* name="other",
* auto_create_subnetworks=False)
* peering1 = gcp.compute.NetworkPeering("peering1",
* name="peering1",
* network=default.self_link,
* peer_network=other.self_link)
* peering2 = gcp.compute.NetworkPeering("peering2",
* name="peering2",
* network=other.self_link,
* peer_network=default.self_link)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.Network("default", new()
* {
* Name = "foobar",
* AutoCreateSubnetworks = false,
* });
* var other = new Gcp.Compute.Network("other", new()
* {
* Name = "other",
* AutoCreateSubnetworks = false,
* });
* var peering1 = new Gcp.Compute.NetworkPeering("peering1", new()
* {
* Name = "peering1",
* Network = @default.SelfLink,
* PeerNetwork = other.SelfLink,
* });
* var peering2 = new Gcp.Compute.NetworkPeering("peering2", new()
* {
* Name = "peering2",
* Network = other.SelfLink,
* PeerNetwork = @default.SelfLink,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNetwork(ctx, "default", &compute.NetworkArgs{
* Name: pulumi.String("foobar"),
* AutoCreateSubnetworks: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* other, err := compute.NewNetwork(ctx, "other", &compute.NetworkArgs{
* Name: pulumi.String("other"),
* AutoCreateSubnetworks: pulumi.Bool(false),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNetworkPeering(ctx, "peering1", &compute.NetworkPeeringArgs{
* Name: pulumi.String("peering1"),
* Network: _default.SelfLink,
* PeerNetwork: other.SelfLink,
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNetworkPeering(ctx, "peering2", &compute.NetworkPeeringArgs{
* Name: pulumi.String("peering2"),
* Network: other.SelfLink,
* PeerNetwork: _default.SelfLink,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Network;
* import com.pulumi.gcp.compute.NetworkArgs;
* import com.pulumi.gcp.compute.NetworkPeering;
* import com.pulumi.gcp.compute.NetworkPeeringArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new Network("default", NetworkArgs.builder()
* .name("foobar")
* .autoCreateSubnetworks("false")
* .build());
* var other = new Network("other", NetworkArgs.builder()
* .name("other")
* .autoCreateSubnetworks("false")
* .build());
* var peering1 = new NetworkPeering("peering1", NetworkPeeringArgs.builder()
* .name("peering1")
* .network(default_.selfLink())
* .peerNetwork(other.selfLink())
* .build());
* var peering2 = new NetworkPeering("peering2", NetworkPeeringArgs.builder()
* .name("peering2")
* .network(other.selfLink())
* .peerNetwork(default_.selfLink())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* peering1:
* type: gcp:compute:NetworkPeering
* properties:
* name: peering1
* network: ${default.selfLink}
* peerNetwork: ${other.selfLink}
* peering2:
* type: gcp:compute:NetworkPeering
* properties:
* name: peering2
* network: ${other.selfLink}
* peerNetwork: ${default.selfLink}
* default:
* type: gcp:compute:Network
* properties:
* name: foobar
* autoCreateSubnetworks: 'false'
* other:
* type: gcp:compute:Network
* properties:
* name: other
* autoCreateSubnetworks: 'false'
* ```
*
* ## Import
* VPC network peerings can be imported using the name and project of the primary network the peering exists in and the name of the network peering
* * `{{project_id}}/{{network_id}}/{{peering_id}}`
* When using the `pulumi import` command, VPC network peerings can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/networkPeering:NetworkPeering default {{project_id}}/{{network_id}}/{{peering_id}}
* ```
* @property exportCustomRoutes Whether to export the custom routes to the peer network. Defaults to `false`.
* @property exportSubnetRoutesWithPublicIp Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not controlled by this field.
* @property importCustomRoutes Whether to import the custom routes from the peer network. Defaults to `false`.
* @property importSubnetRoutesWithPublicIp Whether subnet routes with public IP range are imported. The default value is false. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported from peers and are not controlled by this field.
* @property name Name of the peering.
* @property network The primary network of the peering.
* @property peerNetwork The peer network in the peering. The peer network
* may belong to a different project.
* @property stackType Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY. Possible values: ["IPV4_ONLY", "IPV4_IPV6"].
*/
public data class NetworkPeeringArgs(
public val exportCustomRoutes: Output? = null,
public val exportSubnetRoutesWithPublicIp: Output? = null,
public val importCustomRoutes: Output? = null,
public val importSubnetRoutesWithPublicIp: Output? = null,
public val name: Output? = null,
public val network: Output? = null,
public val peerNetwork: Output? = null,
public val stackType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.NetworkPeeringArgs =
com.pulumi.gcp.compute.NetworkPeeringArgs.builder()
.exportCustomRoutes(exportCustomRoutes?.applyValue({ args0 -> args0 }))
.exportSubnetRoutesWithPublicIp(exportSubnetRoutesWithPublicIp?.applyValue({ args0 -> args0 }))
.importCustomRoutes(importCustomRoutes?.applyValue({ args0 -> args0 }))
.importSubnetRoutesWithPublicIp(importSubnetRoutesWithPublicIp?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.network(network?.applyValue({ args0 -> args0 }))
.peerNetwork(peerNetwork?.applyValue({ args0 -> args0 }))
.stackType(stackType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NetworkPeeringArgs].
*/
@PulumiTagMarker
public class NetworkPeeringArgsBuilder internal constructor() {
private var exportCustomRoutes: Output? = null
private var exportSubnetRoutesWithPublicIp: Output? = null
private var importCustomRoutes: Output? = null
private var importSubnetRoutesWithPublicIp: Output? = null
private var name: Output? = null
private var network: Output? = null
private var peerNetwork: Output? = null
private var stackType: Output? = null
/**
* @param value Whether to export the custom routes to the peer network. Defaults to `false`.
*/
@JvmName("qhyjpulmeqmbyrpj")
public suspend fun exportCustomRoutes(`value`: Output) {
this.exportCustomRoutes = value
}
/**
* @param value Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not controlled by this field.
*/
@JvmName("sqtrvhekwyermfpu")
public suspend fun exportSubnetRoutesWithPublicIp(`value`: Output) {
this.exportSubnetRoutesWithPublicIp = value
}
/**
* @param value Whether to import the custom routes from the peer network. Defaults to `false`.
*/
@JvmName("oiwbuqolabcpgngj")
public suspend fun importCustomRoutes(`value`: Output) {
this.importCustomRoutes = value
}
/**
* @param value Whether subnet routes with public IP range are imported. The default value is false. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported from peers and are not controlled by this field.
*/
@JvmName("qtatywdhamfvivca")
public suspend fun importSubnetRoutesWithPublicIp(`value`: Output) {
this.importSubnetRoutesWithPublicIp = value
}
/**
* @param value Name of the peering.
*/
@JvmName("luydwnjawxlintbi")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The primary network of the peering.
*/
@JvmName("wsytrhopdaytwlvy")
public suspend fun network(`value`: Output) {
this.network = value
}
/**
* @param value The peer network in the peering. The peer network
* may belong to a different project.
*/
@JvmName("hwtbctynhfpwssef")
public suspend fun peerNetwork(`value`: Output) {
this.peerNetwork = value
}
/**
* @param value Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY. Possible values: ["IPV4_ONLY", "IPV4_IPV6"].
*/
@JvmName("nujoatckwpgclpld")
public suspend fun stackType(`value`: Output) {
this.stackType = value
}
/**
* @param value Whether to export the custom routes to the peer network. Defaults to `false`.
*/
@JvmName("fuonyathesiejynf")
public suspend fun exportCustomRoutes(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exportCustomRoutes = mapped
}
/**
* @param value Whether subnet routes with public IP range are exported. The default value is true, all subnet routes are exported. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always exported to peers and are not controlled by this field.
*/
@JvmName("hkbwcvtnolkhxrpp")
public suspend fun exportSubnetRoutesWithPublicIp(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.exportSubnetRoutesWithPublicIp = mapped
}
/**
* @param value Whether to import the custom routes from the peer network. Defaults to `false`.
*/
@JvmName("ljqueylmvsbfynsa")
public suspend fun importCustomRoutes(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.importCustomRoutes = mapped
}
/**
* @param value Whether subnet routes with public IP range are imported. The default value is false. The IPv4 special-use ranges (https://en.wikipedia.org/wiki/IPv4#Special_addresses) are always imported from peers and are not controlled by this field.
*/
@JvmName("qwfdwvkyioqhwdad")
public suspend fun importSubnetRoutesWithPublicIp(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.importSubnetRoutesWithPublicIp = mapped
}
/**
* @param value Name of the peering.
*/
@JvmName("oaytndqykwqospuo")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The primary network of the peering.
*/
@JvmName("cunpgxeiuenawcyi")
public suspend fun network(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.network = mapped
}
/**
* @param value The peer network in the peering. The peer network
* may belong to a different project.
*/
@JvmName("vtfnosnfyccxubbq")
public suspend fun peerNetwork(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.peerNetwork = mapped
}
/**
* @param value Which IP version(s) of traffic and routes are allowed to be imported or exported between peer networks. The default value is IPV4_ONLY. Possible values: ["IPV4_ONLY", "IPV4_IPV6"].
*/
@JvmName("ewnssylvsbdcjrga")
public suspend fun stackType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stackType = mapped
}
internal fun build(): NetworkPeeringArgs = NetworkPeeringArgs(
exportCustomRoutes = exportCustomRoutes,
exportSubnetRoutesWithPublicIp = exportSubnetRoutesWithPublicIp,
importCustomRoutes = importCustomRoutes,
importSubnetRoutesWithPublicIp = importSubnetRoutesWithPublicIp,
name = name,
network = network,
peerNetwork = peerNetwork,
stackType = stackType,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy