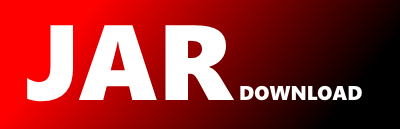
com.pulumi.gcp.compute.kotlin.NodeGroupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.NodeGroupArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupAutoscalingPolicyArgs
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupAutoscalingPolicyArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupMaintenanceWindowArgs
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupMaintenanceWindowArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupShareSettingsArgs
import com.pulumi.gcp.compute.kotlin.inputs.NodeGroupShareSettingsArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents a NodeGroup resource to manage a group of sole-tenant nodes.
* To get more information about NodeGroup, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/rest/v1/nodeGroups)
* * How-to Guides
* * [Sole-Tenant Nodes](https://cloud.google.com/compute/docs/nodes/)
* > **Warning:** Due to limitations of the API, this provider cannot update the
* number of nodes in a node group and changes to node group size either
* through provider config or through external changes will cause
* the provider to delete and recreate the node group.
* ## Example Usage
* ### Node Group Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const soletenant_tmpl = new gcp.compute.NodeTemplate("soletenant-tmpl", {
* name: "soletenant-tmpl",
* region: "us-central1",
* nodeType: "n1-node-96-624",
* });
* const nodes = new gcp.compute.NodeGroup("nodes", {
* name: "soletenant-group",
* zone: "us-central1-a",
* description: "example google_compute_node_group for the Google Provider",
* initialSize: 1,
* nodeTemplate: soletenant_tmpl.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* soletenant_tmpl = gcp.compute.NodeTemplate("soletenant-tmpl",
* name="soletenant-tmpl",
* region="us-central1",
* node_type="n1-node-96-624")
* nodes = gcp.compute.NodeGroup("nodes",
* name="soletenant-group",
* zone="us-central1-a",
* description="example google_compute_node_group for the Google Provider",
* initial_size=1,
* node_template=soletenant_tmpl.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var soletenant_tmpl = new Gcp.Compute.NodeTemplate("soletenant-tmpl", new()
* {
* Name = "soletenant-tmpl",
* Region = "us-central1",
* NodeType = "n1-node-96-624",
* });
* var nodes = new Gcp.Compute.NodeGroup("nodes", new()
* {
* Name = "soletenant-group",
* Zone = "us-central1-a",
* Description = "example google_compute_node_group for the Google Provider",
* InitialSize = 1,
* NodeTemplate = soletenant_tmpl.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNodeTemplate(ctx, "soletenant-tmpl", &compute.NodeTemplateArgs{
* Name: pulumi.String("soletenant-tmpl"),
* Region: pulumi.String("us-central1"),
* NodeType: pulumi.String("n1-node-96-624"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNodeGroup(ctx, "nodes", &compute.NodeGroupArgs{
* Name: pulumi.String("soletenant-group"),
* Zone: pulumi.String("us-central1-a"),
* Description: pulumi.String("example google_compute_node_group for the Google Provider"),
* InitialSize: pulumi.Int(1),
* NodeTemplate: soletenant_tmpl.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.NodeTemplate;
* import com.pulumi.gcp.compute.NodeTemplateArgs;
* import com.pulumi.gcp.compute.NodeGroup;
* import com.pulumi.gcp.compute.NodeGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var soletenant_tmpl = new NodeTemplate("soletenant-tmpl", NodeTemplateArgs.builder()
* .name("soletenant-tmpl")
* .region("us-central1")
* .nodeType("n1-node-96-624")
* .build());
* var nodes = new NodeGroup("nodes", NodeGroupArgs.builder()
* .name("soletenant-group")
* .zone("us-central1-a")
* .description("example google_compute_node_group for the Google Provider")
* .initialSize(1)
* .nodeTemplate(soletenant_tmpl.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* soletenant-tmpl:
* type: gcp:compute:NodeTemplate
* properties:
* name: soletenant-tmpl
* region: us-central1
* nodeType: n1-node-96-624
* nodes:
* type: gcp:compute:NodeGroup
* properties:
* name: soletenant-group
* zone: us-central1-a
* description: example google_compute_node_group for the Google Provider
* initialSize: 1
* nodeTemplate: ${["soletenant-tmpl"].id}
* ```
*
* ### Node Group Maintenance Interval
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const soletenant_tmpl = new gcp.compute.NodeTemplate("soletenant-tmpl", {
* name: "soletenant-tmpl",
* region: "us-central1",
* nodeType: "c2-node-60-240",
* });
* const nodes = new gcp.compute.NodeGroup("nodes", {
* name: "soletenant-group",
* zone: "us-central1-a",
* description: "example google_compute_node_group for Terraform Google Provider",
* initialSize: 1,
* nodeTemplate: soletenant_tmpl.id,
* maintenanceInterval: "RECURRENT",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* soletenant_tmpl = gcp.compute.NodeTemplate("soletenant-tmpl",
* name="soletenant-tmpl",
* region="us-central1",
* node_type="c2-node-60-240")
* nodes = gcp.compute.NodeGroup("nodes",
* name="soletenant-group",
* zone="us-central1-a",
* description="example google_compute_node_group for Terraform Google Provider",
* initial_size=1,
* node_template=soletenant_tmpl.id,
* maintenance_interval="RECURRENT")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var soletenant_tmpl = new Gcp.Compute.NodeTemplate("soletenant-tmpl", new()
* {
* Name = "soletenant-tmpl",
* Region = "us-central1",
* NodeType = "c2-node-60-240",
* });
* var nodes = new Gcp.Compute.NodeGroup("nodes", new()
* {
* Name = "soletenant-group",
* Zone = "us-central1-a",
* Description = "example google_compute_node_group for Terraform Google Provider",
* InitialSize = 1,
* NodeTemplate = soletenant_tmpl.Id,
* MaintenanceInterval = "RECURRENT",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNodeTemplate(ctx, "soletenant-tmpl", &compute.NodeTemplateArgs{
* Name: pulumi.String("soletenant-tmpl"),
* Region: pulumi.String("us-central1"),
* NodeType: pulumi.String("c2-node-60-240"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNodeGroup(ctx, "nodes", &compute.NodeGroupArgs{
* Name: pulumi.String("soletenant-group"),
* Zone: pulumi.String("us-central1-a"),
* Description: pulumi.String("example google_compute_node_group for Terraform Google Provider"),
* InitialSize: pulumi.Int(1),
* NodeTemplate: soletenant_tmpl.ID(),
* MaintenanceInterval: pulumi.String("RECURRENT"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.NodeTemplate;
* import com.pulumi.gcp.compute.NodeTemplateArgs;
* import com.pulumi.gcp.compute.NodeGroup;
* import com.pulumi.gcp.compute.NodeGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var soletenant_tmpl = new NodeTemplate("soletenant-tmpl", NodeTemplateArgs.builder()
* .name("soletenant-tmpl")
* .region("us-central1")
* .nodeType("c2-node-60-240")
* .build());
* var nodes = new NodeGroup("nodes", NodeGroupArgs.builder()
* .name("soletenant-group")
* .zone("us-central1-a")
* .description("example google_compute_node_group for Terraform Google Provider")
* .initialSize(1)
* .nodeTemplate(soletenant_tmpl.id())
* .maintenanceInterval("RECURRENT")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* soletenant-tmpl:
* type: gcp:compute:NodeTemplate
* properties:
* name: soletenant-tmpl
* region: us-central1
* nodeType: c2-node-60-240
* nodes:
* type: gcp:compute:NodeGroup
* properties:
* name: soletenant-group
* zone: us-central1-a
* description: example google_compute_node_group for Terraform Google Provider
* initialSize: 1
* nodeTemplate: ${["soletenant-tmpl"].id}
* maintenanceInterval: RECURRENT
* ```
*
* ### Node Group Autoscaling Policy
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const soletenant_tmpl = new gcp.compute.NodeTemplate("soletenant-tmpl", {
* name: "soletenant-tmpl",
* region: "us-central1",
* nodeType: "n1-node-96-624",
* });
* const nodes = new gcp.compute.NodeGroup("nodes", {
* name: "soletenant-group",
* zone: "us-central1-a",
* description: "example google_compute_node_group for Google Provider",
* maintenancePolicy: "RESTART_IN_PLACE",
* maintenanceWindow: {
* startTime: "08:00",
* },
* initialSize: 1,
* nodeTemplate: soletenant_tmpl.id,
* autoscalingPolicy: {
* mode: "ONLY_SCALE_OUT",
* minNodes: 1,
* maxNodes: 10,
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* soletenant_tmpl = gcp.compute.NodeTemplate("soletenant-tmpl",
* name="soletenant-tmpl",
* region="us-central1",
* node_type="n1-node-96-624")
* nodes = gcp.compute.NodeGroup("nodes",
* name="soletenant-group",
* zone="us-central1-a",
* description="example google_compute_node_group for Google Provider",
* maintenance_policy="RESTART_IN_PLACE",
* maintenance_window=gcp.compute.NodeGroupMaintenanceWindowArgs(
* start_time="08:00",
* ),
* initial_size=1,
* node_template=soletenant_tmpl.id,
* autoscaling_policy=gcp.compute.NodeGroupAutoscalingPolicyArgs(
* mode="ONLY_SCALE_OUT",
* min_nodes=1,
* max_nodes=10,
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var soletenant_tmpl = new Gcp.Compute.NodeTemplate("soletenant-tmpl", new()
* {
* Name = "soletenant-tmpl",
* Region = "us-central1",
* NodeType = "n1-node-96-624",
* });
* var nodes = new Gcp.Compute.NodeGroup("nodes", new()
* {
* Name = "soletenant-group",
* Zone = "us-central1-a",
* Description = "example google_compute_node_group for Google Provider",
* MaintenancePolicy = "RESTART_IN_PLACE",
* MaintenanceWindow = new Gcp.Compute.Inputs.NodeGroupMaintenanceWindowArgs
* {
* StartTime = "08:00",
* },
* InitialSize = 1,
* NodeTemplate = soletenant_tmpl.Id,
* AutoscalingPolicy = new Gcp.Compute.Inputs.NodeGroupAutoscalingPolicyArgs
* {
* Mode = "ONLY_SCALE_OUT",
* MinNodes = 1,
* MaxNodes = 10,
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewNodeTemplate(ctx, "soletenant-tmpl", &compute.NodeTemplateArgs{
* Name: pulumi.String("soletenant-tmpl"),
* Region: pulumi.String("us-central1"),
* NodeType: pulumi.String("n1-node-96-624"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNodeGroup(ctx, "nodes", &compute.NodeGroupArgs{
* Name: pulumi.String("soletenant-group"),
* Zone: pulumi.String("us-central1-a"),
* Description: pulumi.String("example google_compute_node_group for Google Provider"),
* MaintenancePolicy: pulumi.String("RESTART_IN_PLACE"),
* MaintenanceWindow: &compute.NodeGroupMaintenanceWindowArgs{
* StartTime: pulumi.String("08:00"),
* },
* InitialSize: pulumi.Int(1),
* NodeTemplate: soletenant_tmpl.ID(),
* AutoscalingPolicy: &compute.NodeGroupAutoscalingPolicyArgs{
* Mode: pulumi.String("ONLY_SCALE_OUT"),
* MinNodes: pulumi.Int(1),
* MaxNodes: pulumi.Int(10),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.NodeTemplate;
* import com.pulumi.gcp.compute.NodeTemplateArgs;
* import com.pulumi.gcp.compute.NodeGroup;
* import com.pulumi.gcp.compute.NodeGroupArgs;
* import com.pulumi.gcp.compute.inputs.NodeGroupMaintenanceWindowArgs;
* import com.pulumi.gcp.compute.inputs.NodeGroupAutoscalingPolicyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var soletenant_tmpl = new NodeTemplate("soletenant-tmpl", NodeTemplateArgs.builder()
* .name("soletenant-tmpl")
* .region("us-central1")
* .nodeType("n1-node-96-624")
* .build());
* var nodes = new NodeGroup("nodes", NodeGroupArgs.builder()
* .name("soletenant-group")
* .zone("us-central1-a")
* .description("example google_compute_node_group for Google Provider")
* .maintenancePolicy("RESTART_IN_PLACE")
* .maintenanceWindow(NodeGroupMaintenanceWindowArgs.builder()
* .startTime("08:00")
* .build())
* .initialSize(1)
* .nodeTemplate(soletenant_tmpl.id())
* .autoscalingPolicy(NodeGroupAutoscalingPolicyArgs.builder()
* .mode("ONLY_SCALE_OUT")
* .minNodes(1)
* .maxNodes(10)
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* soletenant-tmpl:
* type: gcp:compute:NodeTemplate
* properties:
* name: soletenant-tmpl
* region: us-central1
* nodeType: n1-node-96-624
* nodes:
* type: gcp:compute:NodeGroup
* properties:
* name: soletenant-group
* zone: us-central1-a
* description: example google_compute_node_group for Google Provider
* maintenancePolicy: RESTART_IN_PLACE
* maintenanceWindow:
* startTime: 08:00
* initialSize: 1
* nodeTemplate: ${["soletenant-tmpl"].id}
* autoscalingPolicy:
* mode: ONLY_SCALE_OUT
* minNodes: 1
* maxNodes: 10
* ```
*
* ### Node Group Share Settings
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const guestProject = new gcp.organizations.Project("guest_project", {
* projectId: "project-id",
* name: "project-name",
* orgId: "123456789",
* });
* const soletenant_tmpl = new gcp.compute.NodeTemplate("soletenant-tmpl", {
* name: "soletenant-tmpl",
* region: "us-central1",
* nodeType: "n1-node-96-624",
* });
* const nodes = new gcp.compute.NodeGroup("nodes", {
* name: "soletenant-group",
* zone: "us-central1-f",
* description: "example google_compute_node_group for Terraform Google Provider",
* initialSize: 1,
* nodeTemplate: soletenant_tmpl.id,
* shareSettings: {
* shareType: "SPECIFIC_PROJECTS",
* projectMaps: [{
* id: guestProject.projectId,
* projectId: guestProject.projectId,
* }],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* guest_project = gcp.organizations.Project("guest_project",
* project_id="project-id",
* name="project-name",
* org_id="123456789")
* soletenant_tmpl = gcp.compute.NodeTemplate("soletenant-tmpl",
* name="soletenant-tmpl",
* region="us-central1",
* node_type="n1-node-96-624")
* nodes = gcp.compute.NodeGroup("nodes",
* name="soletenant-group",
* zone="us-central1-f",
* description="example google_compute_node_group for Terraform Google Provider",
* initial_size=1,
* node_template=soletenant_tmpl.id,
* share_settings=gcp.compute.NodeGroupShareSettingsArgs(
* share_type="SPECIFIC_PROJECTS",
* project_maps=[gcp.compute.NodeGroupShareSettingsProjectMapArgs(
* id=guest_project.project_id,
* project_id=guest_project.project_id,
* )],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var guestProject = new Gcp.Organizations.Project("guest_project", new()
* {
* ProjectId = "project-id",
* Name = "project-name",
* OrgId = "123456789",
* });
* var soletenant_tmpl = new Gcp.Compute.NodeTemplate("soletenant-tmpl", new()
* {
* Name = "soletenant-tmpl",
* Region = "us-central1",
* NodeType = "n1-node-96-624",
* });
* var nodes = new Gcp.Compute.NodeGroup("nodes", new()
* {
* Name = "soletenant-group",
* Zone = "us-central1-f",
* Description = "example google_compute_node_group for Terraform Google Provider",
* InitialSize = 1,
* NodeTemplate = soletenant_tmpl.Id,
* ShareSettings = new Gcp.Compute.Inputs.NodeGroupShareSettingsArgs
* {
* ShareType = "SPECIFIC_PROJECTS",
* ProjectMaps = new[]
* {
* new Gcp.Compute.Inputs.NodeGroupShareSettingsProjectMapArgs
* {
* Id = guestProject.ProjectId,
* ProjectId = guestProject.ProjectId,
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/organizations"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* guestProject, err := organizations.NewProject(ctx, "guest_project", &organizations.ProjectArgs{
* ProjectId: pulumi.String("project-id"),
* Name: pulumi.String("project-name"),
* OrgId: pulumi.String("123456789"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNodeTemplate(ctx, "soletenant-tmpl", &compute.NodeTemplateArgs{
* Name: pulumi.String("soletenant-tmpl"),
* Region: pulumi.String("us-central1"),
* NodeType: pulumi.String("n1-node-96-624"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewNodeGroup(ctx, "nodes", &compute.NodeGroupArgs{
* Name: pulumi.String("soletenant-group"),
* Zone: pulumi.String("us-central1-f"),
* Description: pulumi.String("example google_compute_node_group for Terraform Google Provider"),
* InitialSize: pulumi.Int(1),
* NodeTemplate: soletenant_tmpl.ID(),
* ShareSettings: &compute.NodeGroupShareSettingsArgs{
* ShareType: pulumi.String("SPECIFIC_PROJECTS"),
* ProjectMaps: compute.NodeGroupShareSettingsProjectMapArray{
* &compute.NodeGroupShareSettingsProjectMapArgs{
* Id: guestProject.ProjectId,
* ProjectId: guestProject.ProjectId,
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.organizations.Project;
* import com.pulumi.gcp.organizations.ProjectArgs;
* import com.pulumi.gcp.compute.NodeTemplate;
* import com.pulumi.gcp.compute.NodeTemplateArgs;
* import com.pulumi.gcp.compute.NodeGroup;
* import com.pulumi.gcp.compute.NodeGroupArgs;
* import com.pulumi.gcp.compute.inputs.NodeGroupShareSettingsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var guestProject = new Project("guestProject", ProjectArgs.builder()
* .projectId("project-id")
* .name("project-name")
* .orgId("123456789")
* .build());
* var soletenant_tmpl = new NodeTemplate("soletenant-tmpl", NodeTemplateArgs.builder()
* .name("soletenant-tmpl")
* .region("us-central1")
* .nodeType("n1-node-96-624")
* .build());
* var nodes = new NodeGroup("nodes", NodeGroupArgs.builder()
* .name("soletenant-group")
* .zone("us-central1-f")
* .description("example google_compute_node_group for Terraform Google Provider")
* .initialSize(1)
* .nodeTemplate(soletenant_tmpl.id())
* .shareSettings(NodeGroupShareSettingsArgs.builder()
* .shareType("SPECIFIC_PROJECTS")
* .projectMaps(NodeGroupShareSettingsProjectMapArgs.builder()
* .id(guestProject.projectId())
* .projectId(guestProject.projectId())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* guestProject:
* type: gcp:organizations:Project
* name: guest_project
* properties:
* projectId: project-id
* name: project-name
* orgId: '123456789'
* soletenant-tmpl:
* type: gcp:compute:NodeTemplate
* properties:
* name: soletenant-tmpl
* region: us-central1
* nodeType: n1-node-96-624
* nodes:
* type: gcp:compute:NodeGroup
* properties:
* name: soletenant-group
* zone: us-central1-f
* description: example google_compute_node_group for Terraform Google Provider
* initialSize: 1
* nodeTemplate: ${["soletenant-tmpl"].id}
* shareSettings:
* shareType: SPECIFIC_PROJECTS
* projectMaps:
* - id: ${guestProject.projectId}
* projectId: ${guestProject.projectId}
* ```
*
* ## Import
* NodeGroup can be imported using any of these accepted formats:
* * `projects/{{project}}/zones/{{zone}}/nodeGroups/{{name}}`
* * `{{project}}/{{zone}}/{{name}}`
* * `{{zone}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, NodeGroup can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/nodeGroup:NodeGroup default projects/{{project}}/zones/{{zone}}/nodeGroups/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/nodeGroup:NodeGroup default {{project}}/{{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/nodeGroup:NodeGroup default {{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/nodeGroup:NodeGroup default {{name}}
* ```
* @property autoscalingPolicy If you use sole-tenant nodes for your workloads, you can use the node
* group autoscaler to automatically manage the sizes of your node groups.
* One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
* Structure is documented below.
* @property description An optional textual description of the resource.
* @property initialSize The initial number of nodes in the node group. One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
* @property maintenanceInterval Specifies the frequency of planned maintenance events. Set to one of the following:
* - AS_NEEDED: Hosts are eligible to receive infrastructure and hypervisor updates as they become available.
* - RECURRENT: Hosts receive planned infrastructure and hypervisor updates on a periodic basis, but not more frequently than every 28 days. This minimizes the number of planned maintenance operations on individual hosts and reduces the frequency of disruptions, both live migrations and terminations, on individual VMs.
* Possible values are: `AS_NEEDED`, `RECURRENT`.
* @property maintenancePolicy Specifies how to handle instances when a node in the group undergoes maintenance. Set to one of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT.
* @property maintenanceWindow contains properties for the timeframe of maintenance
* Structure is documented below.
* @property name Name of the resource.
* @property nodeTemplate The URL of the node template to which this node group belongs.
* - - -
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property shareSettings Share settings for the node group.
* Structure is documented below.
* @property zone Zone where this node group is located
*/
public data class NodeGroupArgs(
public val autoscalingPolicy: Output? = null,
public val description: Output? = null,
public val initialSize: Output? = null,
public val maintenanceInterval: Output? = null,
public val maintenancePolicy: Output? = null,
public val maintenanceWindow: Output? = null,
public val name: Output? = null,
public val nodeTemplate: Output? = null,
public val project: Output? = null,
public val shareSettings: Output? = null,
public val zone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.NodeGroupArgs =
com.pulumi.gcp.compute.NodeGroupArgs.builder()
.autoscalingPolicy(autoscalingPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.initialSize(initialSize?.applyValue({ args0 -> args0 }))
.maintenanceInterval(maintenanceInterval?.applyValue({ args0 -> args0 }))
.maintenancePolicy(maintenancePolicy?.applyValue({ args0 -> args0 }))
.maintenanceWindow(maintenanceWindow?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name?.applyValue({ args0 -> args0 }))
.nodeTemplate(nodeTemplate?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.shareSettings(shareSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.zone(zone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NodeGroupArgs].
*/
@PulumiTagMarker
public class NodeGroupArgsBuilder internal constructor() {
private var autoscalingPolicy: Output? = null
private var description: Output? = null
private var initialSize: Output? = null
private var maintenanceInterval: Output? = null
private var maintenancePolicy: Output? = null
private var maintenanceWindow: Output? = null
private var name: Output? = null
private var nodeTemplate: Output? = null
private var project: Output? = null
private var shareSettings: Output? = null
private var zone: Output? = null
/**
* @param value If you use sole-tenant nodes for your workloads, you can use the node
* group autoscaler to automatically manage the sizes of your node groups.
* One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
* Structure is documented below.
*/
@JvmName("ajdnkfibxmbwprys")
public suspend fun autoscalingPolicy(`value`: Output) {
this.autoscalingPolicy = value
}
/**
* @param value An optional textual description of the resource.
*/
@JvmName("owekswinmdnvhrbj")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The initial number of nodes in the node group. One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
*/
@JvmName("lkuktqgrutxqcgwm")
public suspend fun initialSize(`value`: Output) {
this.initialSize = value
}
/**
* @param value Specifies the frequency of planned maintenance events. Set to one of the following:
* - AS_NEEDED: Hosts are eligible to receive infrastructure and hypervisor updates as they become available.
* - RECURRENT: Hosts receive planned infrastructure and hypervisor updates on a periodic basis, but not more frequently than every 28 days. This minimizes the number of planned maintenance operations on individual hosts and reduces the frequency of disruptions, both live migrations and terminations, on individual VMs.
* Possible values are: `AS_NEEDED`, `RECURRENT`.
*/
@JvmName("peaqbemnuqhtatps")
public suspend fun maintenanceInterval(`value`: Output) {
this.maintenanceInterval = value
}
/**
* @param value Specifies how to handle instances when a node in the group undergoes maintenance. Set to one of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT.
*/
@JvmName("ddmkeuuaajfltdoa")
public suspend fun maintenancePolicy(`value`: Output) {
this.maintenancePolicy = value
}
/**
* @param value contains properties for the timeframe of maintenance
* Structure is documented below.
*/
@JvmName("tpssnmbthvmavhhr")
public suspend fun maintenanceWindow(`value`: Output) {
this.maintenanceWindow = value
}
/**
* @param value Name of the resource.
*/
@JvmName("bgqvruymndkxtgkx")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The URL of the node template to which this node group belongs.
* - - -
*/
@JvmName("hxyxdmlatlthpfwu")
public suspend fun nodeTemplate(`value`: Output) {
this.nodeTemplate = value
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("jjpabroccjyeibbh")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value Share settings for the node group.
* Structure is documented below.
*/
@JvmName("dxuitdgipkuaqnfj")
public suspend fun shareSettings(`value`: Output) {
this.shareSettings = value
}
/**
* @param value Zone where this node group is located
*/
@JvmName("tnbkvdjpjheguqri")
public suspend fun zone(`value`: Output) {
this.zone = value
}
/**
* @param value If you use sole-tenant nodes for your workloads, you can use the node
* group autoscaler to automatically manage the sizes of your node groups.
* One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
* Structure is documented below.
*/
@JvmName("kcblnvueybwimtge")
public suspend fun autoscalingPolicy(`value`: NodeGroupAutoscalingPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoscalingPolicy = mapped
}
/**
* @param argument If you use sole-tenant nodes for your workloads, you can use the node
* group autoscaler to automatically manage the sizes of your node groups.
* One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
* Structure is documented below.
*/
@JvmName("dvryjwotddddypcr")
public suspend fun autoscalingPolicy(argument: suspend NodeGroupAutoscalingPolicyArgsBuilder.() -> Unit) {
val toBeMapped = NodeGroupAutoscalingPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.autoscalingPolicy = mapped
}
/**
* @param value An optional textual description of the resource.
*/
@JvmName("qwbbbrlksscbeatj")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The initial number of nodes in the node group. One of `initial_size` or `autoscaling_policy` must be configured on resource creation.
*/
@JvmName("yvdoccctqmostlxt")
public suspend fun initialSize(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.initialSize = mapped
}
/**
* @param value Specifies the frequency of planned maintenance events. Set to one of the following:
* - AS_NEEDED: Hosts are eligible to receive infrastructure and hypervisor updates as they become available.
* - RECURRENT: Hosts receive planned infrastructure and hypervisor updates on a periodic basis, but not more frequently than every 28 days. This minimizes the number of planned maintenance operations on individual hosts and reduces the frequency of disruptions, both live migrations and terminations, on individual VMs.
* Possible values are: `AS_NEEDED`, `RECURRENT`.
*/
@JvmName("kkgxhhnqsphxhdmn")
public suspend fun maintenanceInterval(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maintenanceInterval = mapped
}
/**
* @param value Specifies how to handle instances when a node in the group undergoes maintenance. Set to one of: DEFAULT, RESTART_IN_PLACE, or MIGRATE_WITHIN_NODE_GROUP. The default value is DEFAULT.
*/
@JvmName("fsetwebbqxxisjsw")
public suspend fun maintenancePolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maintenancePolicy = mapped
}
/**
* @param value contains properties for the timeframe of maintenance
* Structure is documented below.
*/
@JvmName("xibyxfwqewnhmfkp")
public suspend fun maintenanceWindow(`value`: NodeGroupMaintenanceWindowArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maintenanceWindow = mapped
}
/**
* @param argument contains properties for the timeframe of maintenance
* Structure is documented below.
*/
@JvmName("ygkbgnhntntapcql")
public suspend fun maintenanceWindow(argument: suspend NodeGroupMaintenanceWindowArgsBuilder.() -> Unit) {
val toBeMapped = NodeGroupMaintenanceWindowArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.maintenanceWindow = mapped
}
/**
* @param value Name of the resource.
*/
@JvmName("mlhxsuktamtoaitn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The URL of the node template to which this node group belongs.
* - - -
*/
@JvmName("ociqlglwplysfnni")
public suspend fun nodeTemplate(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.nodeTemplate = mapped
}
/**
* @param value The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
*/
@JvmName("tiiwntvloodmqhsu")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value Share settings for the node group.
* Structure is documented below.
*/
@JvmName("lthenakjwgharcuc")
public suspend fun shareSettings(`value`: NodeGroupShareSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shareSettings = mapped
}
/**
* @param argument Share settings for the node group.
* Structure is documented below.
*/
@JvmName("fiitiaollukhaqye")
public suspend fun shareSettings(argument: suspend NodeGroupShareSettingsArgsBuilder.() -> Unit) {
val toBeMapped = NodeGroupShareSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.shareSettings = mapped
}
/**
* @param value Zone where this node group is located
*/
@JvmName("vmvjnanhitiktnst")
public suspend fun zone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zone = mapped
}
internal fun build(): NodeGroupArgs = NodeGroupArgs(
autoscalingPolicy = autoscalingPolicy,
description = description,
initialSize = initialSize,
maintenanceInterval = maintenanceInterval,
maintenancePolicy = maintenancePolicy,
maintenanceWindow = maintenanceWindow,
name = name,
nodeTemplate = nodeTemplate,
project = project,
shareSettings = shareSettings,
zone = zone,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy