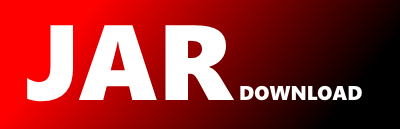
com.pulumi.gcp.compute.kotlin.ReservationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.ReservationArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.ReservationShareSettingsArgs
import com.pulumi.gcp.compute.kotlin.inputs.ReservationShareSettingsArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.ReservationSpecificReservationArgs
import com.pulumi.gcp.compute.kotlin.inputs.ReservationSpecificReservationArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Represents a reservation resource. A reservation ensures that capacity is
* held in a specific zone even if the reserved VMs are not running.
* Reservations apply only to Compute Engine, Cloud Dataproc, and Google
* Kubernetes Engine VM usage.Reservations do not apply to `f1-micro` or
* `g1-small` machine types, preemptible VMs, sole tenant nodes, or other
* services not listed above
* like Cloud SQL and Dataflow.
* To get more information about Reservation, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/rest/v1/reservations)
* * How-to Guides
* * [Reserving zonal resources](https://cloud.google.com/compute/docs/instances/reserving-zonal-resources)
* ## Example Usage
* ### Reservation Basic
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const gceReservation = new gcp.compute.Reservation("gce_reservation", {
* name: "gce-reservation",
* zone: "us-central1-a",
* specificReservation: {
* count: 1,
* instanceProperties: {
* minCpuPlatform: "Intel Cascade Lake",
* machineType: "n2-standard-2",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* gce_reservation = gcp.compute.Reservation("gce_reservation",
* name="gce-reservation",
* zone="us-central1-a",
* specific_reservation=gcp.compute.ReservationSpecificReservationArgs(
* count=1,
* instance_properties=gcp.compute.ReservationSpecificReservationInstancePropertiesArgs(
* min_cpu_platform="Intel Cascade Lake",
* machine_type="n2-standard-2",
* ),
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var gceReservation = new Gcp.Compute.Reservation("gce_reservation", new()
* {
* Name = "gce-reservation",
* Zone = "us-central1-a",
* SpecificReservation = new Gcp.Compute.Inputs.ReservationSpecificReservationArgs
* {
* Count = 1,
* InstanceProperties = new Gcp.Compute.Inputs.ReservationSpecificReservationInstancePropertiesArgs
* {
* MinCpuPlatform = "Intel Cascade Lake",
* MachineType = "n2-standard-2",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewReservation(ctx, "gce_reservation", &compute.ReservationArgs{
* Name: pulumi.String("gce-reservation"),
* Zone: pulumi.String("us-central1-a"),
* SpecificReservation: &compute.ReservationSpecificReservationArgs{
* Count: pulumi.Int(1),
* InstanceProperties: &compute.ReservationSpecificReservationInstancePropertiesArgs{
* MinCpuPlatform: pulumi.String("Intel Cascade Lake"),
* MachineType: pulumi.String("n2-standard-2"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.Reservation;
* import com.pulumi.gcp.compute.ReservationArgs;
* import com.pulumi.gcp.compute.inputs.ReservationSpecificReservationArgs;
* import com.pulumi.gcp.compute.inputs.ReservationSpecificReservationInstancePropertiesArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var gceReservation = new Reservation("gceReservation", ReservationArgs.builder()
* .name("gce-reservation")
* .zone("us-central1-a")
* .specificReservation(ReservationSpecificReservationArgs.builder()
* .count(1)
* .instanceProperties(ReservationSpecificReservationInstancePropertiesArgs.builder()
* .minCpuPlatform("Intel Cascade Lake")
* .machineType("n2-standard-2")
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* gceReservation:
* type: gcp:compute:Reservation
* name: gce_reservation
* properties:
* name: gce-reservation
* zone: us-central1-a
* specificReservation:
* count: 1
* instanceProperties:
* minCpuPlatform: Intel Cascade Lake
* machineType: n2-standard-2
* ```
*
* ## Import
* Reservation can be imported using any of these accepted formats:
* * `projects/{{project}}/zones/{{zone}}/reservations/{{name}}`
* * `{{project}}/{{zone}}/{{name}}`
* * `{{zone}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, Reservation can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/reservation:Reservation default projects/{{project}}/zones/{{zone}}/reservations/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/reservation:Reservation default {{project}}/{{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/reservation:Reservation default {{zone}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/reservation:Reservation default {{name}}
* ```
* @property description An optional description of this resource.
* @property name Name of the resource. Provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and match
* the regular expression `a-z?` which means the
* first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the last
* character, which cannot be a dash.
* @property project
* @property shareSettings The share setting for reservations.
* @property specificReservation Reservation for instances with specific machine shapes.
* Structure is documented below.
* @property specificReservationRequired When set to true, only VMs that target this reservation by name can consume this reservation. Otherwise, it can be
* consumed by VMs with affinity for any reservation. Defaults to false.
* @property zone The zone where the reservation is made.
*/
public data class ReservationArgs(
public val description: Output? = null,
public val name: Output? = null,
public val project: Output? = null,
public val shareSettings: Output? = null,
public val specificReservation: Output? = null,
public val specificReservationRequired: Output? = null,
public val zone: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.ReservationArgs =
com.pulumi.gcp.compute.ReservationArgs.builder()
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.shareSettings(shareSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.specificReservation(
specificReservation?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.specificReservationRequired(specificReservationRequired?.applyValue({ args0 -> args0 }))
.zone(zone?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ReservationArgs].
*/
@PulumiTagMarker
public class ReservationArgsBuilder internal constructor() {
private var description: Output? = null
private var name: Output? = null
private var project: Output? = null
private var shareSettings: Output? = null
private var specificReservation: Output? = null
private var specificReservationRequired: Output? = null
private var zone: Output? = null
/**
* @param value An optional description of this resource.
*/
@JvmName("vqtujesddpeplkfk")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Name of the resource. Provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and match
* the regular expression `a-z?` which means the
* first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the last
* character, which cannot be a dash.
*/
@JvmName("snjgatveindgbkfn")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value
*/
@JvmName("gogvbxbmeahivqad")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The share setting for reservations.
*/
@JvmName("bgichljwjyedhrob")
public suspend fun shareSettings(`value`: Output) {
this.shareSettings = value
}
/**
* @param value Reservation for instances with specific machine shapes.
* Structure is documented below.
*/
@JvmName("floqlosxyvunajaq")
public suspend fun specificReservation(`value`: Output) {
this.specificReservation = value
}
/**
* @param value When set to true, only VMs that target this reservation by name can consume this reservation. Otherwise, it can be
* consumed by VMs with affinity for any reservation. Defaults to false.
*/
@JvmName("ylqrcjolyrpvweww")
public suspend fun specificReservationRequired(`value`: Output) {
this.specificReservationRequired = value
}
/**
* @param value The zone where the reservation is made.
*/
@JvmName("sveaadorvatdxkyk")
public suspend fun zone(`value`: Output) {
this.zone = value
}
/**
* @param value An optional description of this resource.
*/
@JvmName("bvvovwbutumvxuaf")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value Name of the resource. Provided by the client when the resource is
* created. The name must be 1-63 characters long, and comply with
* RFC1035. Specifically, the name must be 1-63 characters long and match
* the regular expression `a-z?` which means the
* first character must be a lowercase letter, and all following
* characters must be a dash, lowercase letter, or digit, except the last
* character, which cannot be a dash.
*/
@JvmName("knsmtfsoglbcxibn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value
*/
@JvmName("lwuwbqqgbbsmalvc")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The share setting for reservations.
*/
@JvmName("fwbjuvkjqsmhowia")
public suspend fun shareSettings(`value`: ReservationShareSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shareSettings = mapped
}
/**
* @param argument The share setting for reservations.
*/
@JvmName("qvnsnmrqvuoonlvk")
public suspend fun shareSettings(argument: suspend ReservationShareSettingsArgsBuilder.() -> Unit) {
val toBeMapped = ReservationShareSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.shareSettings = mapped
}
/**
* @param value Reservation for instances with specific machine shapes.
* Structure is documented below.
*/
@JvmName("dldammiefilhpeaw")
public suspend fun specificReservation(`value`: ReservationSpecificReservationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.specificReservation = mapped
}
/**
* @param argument Reservation for instances with specific machine shapes.
* Structure is documented below.
*/
@JvmName("umulrgmxfafslrxu")
public suspend fun specificReservation(argument: suspend ReservationSpecificReservationArgsBuilder.() -> Unit) {
val toBeMapped = ReservationSpecificReservationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.specificReservation = mapped
}
/**
* @param value When set to true, only VMs that target this reservation by name can consume this reservation. Otherwise, it can be
* consumed by VMs with affinity for any reservation. Defaults to false.
*/
@JvmName("oxxptvmwhyiherit")
public suspend fun specificReservationRequired(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.specificReservationRequired = mapped
}
/**
* @param value The zone where the reservation is made.
*/
@JvmName("ehrnnvmuhptoanop")
public suspend fun zone(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.zone = mapped
}
internal fun build(): ReservationArgs = ReservationArgs(
description = description,
name = name,
project = project,
shareSettings = shareSettings,
specificReservation = specificReservation,
specificReservationRequired = specificReservationRequired,
zone = zone,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy