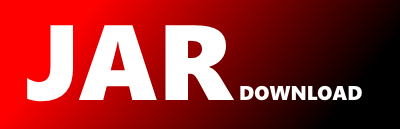
com.pulumi.gcp.compute.kotlin.RouterInterfaceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.RouterInterfaceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Manages a Cloud Router interface. For more information see
* [the official documentation](https://cloud.google.com/compute/docs/cloudrouter)
* and
* [API](https://cloud.google.com/compute/docs/reference/latest/routers).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const foobar = new gcp.compute.RouterInterface("foobar", {
* name: "interface-1",
* router: "router-1",
* region: "us-central1",
* ipRange: "169.254.1.1/30",
* vpnTunnel: "tunnel-1",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* foobar = gcp.compute.RouterInterface("foobar",
* name="interface-1",
* router="router-1",
* region="us-central1",
* ip_range="169.254.1.1/30",
* vpn_tunnel="tunnel-1")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var foobar = new Gcp.Compute.RouterInterface("foobar", new()
* {
* Name = "interface-1",
* Router = "router-1",
* Region = "us-central1",
* IpRange = "169.254.1.1/30",
* VpnTunnel = "tunnel-1",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewRouterInterface(ctx, "foobar", &compute.RouterInterfaceArgs{
* Name: pulumi.String("interface-1"),
* Router: pulumi.String("router-1"),
* Region: pulumi.String("us-central1"),
* IpRange: pulumi.String("169.254.1.1/30"),
* VpnTunnel: pulumi.String("tunnel-1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.RouterInterface;
* import com.pulumi.gcp.compute.RouterInterfaceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var foobar = new RouterInterface("foobar", RouterInterfaceArgs.builder()
* .name("interface-1")
* .router("router-1")
* .region("us-central1")
* .ipRange("169.254.1.1/30")
* .vpnTunnel("tunnel-1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* foobar:
* type: gcp:compute:RouterInterface
* properties:
* name: interface-1
* router: router-1
* region: us-central1
* ipRange: 169.254.1.1/30
* vpnTunnel: tunnel-1
* ```
*
* ## Import
* Router interfaces can be imported using the `project` (optional), `region`, `router`, and `name`, e.g.
* * `{{project_id}}/{{region}}/{{router}}/{{name}}`
* * `{{region}}/{{router}}/{{name}}`
* When using the `pulumi import` command, router interfaces can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/routerInterface:RouterInterface default {{project_id}}/{{region}}/{{router}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/routerInterface:RouterInterface default {{region}}/{{router}}/{{name}}
* ```
* @property interconnectAttachment The name or resource link to the
* VLAN interconnect for this interface. Changing this forces a new interface to
* be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
* @property ipRange IP address and range of the interface. The IP range must be
* in the RFC3927 link-local IP space. Changing this forces a new interface to be created.
* @property ipVersion IP version of this interface. Can be either IPV4 or IPV6.
* @property name A unique name for the interface, required by GCE. Changing
* this forces a new interface to be created.
* @property privateIpAddress The regional private internal IP address that is used
* to establish BGP sessions to a VM instance acting as a third-party Router Appliance. Changing this forces a new interface to be created.
* @property project The ID of the project in which this interface's routerbelongs.
* If it is not provided, the provider project is used. Changing this forces a new interface to be created.
* @property redundantInterface The name of the interface that is redundant to
* this interface. Changing this forces a new interface to be created.
* @property region The region this interface's router sits in.
* If not specified, the project region will be used. Changing this forces a new interface to be created.
* @property router The name of the router this interface will be attached to.
* Changing this forces a new interface to be created.
* In addition to the above required fields, a router interface must have specified either `ip_range` or exactly one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork`, or both.
* - - -
* @property subnetwork The URI of the subnetwork resource that this interface
* belongs to, which must be in the same region as the Cloud Router. When you establish a BGP session to a VM instance using this interface, the VM instance must belong to the same subnetwork as the subnetwork specified here. Changing this forces a new interface to be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
* @property vpnTunnel The name or resource link to the VPN tunnel this
* interface will be linked to. Changing this forces a new interface to be created. Only
* one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
public data class RouterInterfaceArgs(
public val interconnectAttachment: Output? = null,
public val ipRange: Output? = null,
public val ipVersion: Output? = null,
public val name: Output? = null,
public val privateIpAddress: Output? = null,
public val project: Output? = null,
public val redundantInterface: Output? = null,
public val region: Output? = null,
public val router: Output? = null,
public val subnetwork: Output? = null,
public val vpnTunnel: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.RouterInterfaceArgs =
com.pulumi.gcp.compute.RouterInterfaceArgs.builder()
.interconnectAttachment(interconnectAttachment?.applyValue({ args0 -> args0 }))
.ipRange(ipRange?.applyValue({ args0 -> args0 }))
.ipVersion(ipVersion?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.privateIpAddress(privateIpAddress?.applyValue({ args0 -> args0 }))
.project(project?.applyValue({ args0 -> args0 }))
.redundantInterface(redundantInterface?.applyValue({ args0 -> args0 }))
.region(region?.applyValue({ args0 -> args0 }))
.router(router?.applyValue({ args0 -> args0 }))
.subnetwork(subnetwork?.applyValue({ args0 -> args0 }))
.vpnTunnel(vpnTunnel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RouterInterfaceArgs].
*/
@PulumiTagMarker
public class RouterInterfaceArgsBuilder internal constructor() {
private var interconnectAttachment: Output? = null
private var ipRange: Output? = null
private var ipVersion: Output? = null
private var name: Output? = null
private var privateIpAddress: Output? = null
private var project: Output? = null
private var redundantInterface: Output? = null
private var region: Output? = null
private var router: Output? = null
private var subnetwork: Output? = null
private var vpnTunnel: Output? = null
/**
* @param value The name or resource link to the
* VLAN interconnect for this interface. Changing this forces a new interface to
* be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("opiyfsprrqtyvqig")
public suspend fun interconnectAttachment(`value`: Output) {
this.interconnectAttachment = value
}
/**
* @param value IP address and range of the interface. The IP range must be
* in the RFC3927 link-local IP space. Changing this forces a new interface to be created.
*/
@JvmName("vbjeaeplcicsoshn")
public suspend fun ipRange(`value`: Output) {
this.ipRange = value
}
/**
* @param value IP version of this interface. Can be either IPV4 or IPV6.
*/
@JvmName("wpntgkryttobvjli")
public suspend fun ipVersion(`value`: Output) {
this.ipVersion = value
}
/**
* @param value A unique name for the interface, required by GCE. Changing
* this forces a new interface to be created.
*/
@JvmName("jveufflgjcfdjnjk")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The regional private internal IP address that is used
* to establish BGP sessions to a VM instance acting as a third-party Router Appliance. Changing this forces a new interface to be created.
*/
@JvmName("cjtadtprxhbnmwjl")
public suspend fun privateIpAddress(`value`: Output) {
this.privateIpAddress = value
}
/**
* @param value The ID of the project in which this interface's routerbelongs.
* If it is not provided, the provider project is used. Changing this forces a new interface to be created.
*/
@JvmName("meejamxpsydodwfw")
public suspend fun project(`value`: Output) {
this.project = value
}
/**
* @param value The name of the interface that is redundant to
* this interface. Changing this forces a new interface to be created.
*/
@JvmName("kjawvdeahgbeaqnu")
public suspend fun redundantInterface(`value`: Output) {
this.redundantInterface = value
}
/**
* @param value The region this interface's router sits in.
* If not specified, the project region will be used. Changing this forces a new interface to be created.
*/
@JvmName("duncmnholwehhcsa")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value The name of the router this interface will be attached to.
* Changing this forces a new interface to be created.
* In addition to the above required fields, a router interface must have specified either `ip_range` or exactly one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork`, or both.
* - - -
*/
@JvmName("hjaefddbwbcwjfmh")
public suspend fun router(`value`: Output) {
this.router = value
}
/**
* @param value The URI of the subnetwork resource that this interface
* belongs to, which must be in the same region as the Cloud Router. When you establish a BGP session to a VM instance using this interface, the VM instance must belong to the same subnetwork as the subnetwork specified here. Changing this forces a new interface to be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("esbehicugtesotvd")
public suspend fun subnetwork(`value`: Output) {
this.subnetwork = value
}
/**
* @param value The name or resource link to the VPN tunnel this
* interface will be linked to. Changing this forces a new interface to be created. Only
* one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("yuembyuipoaubjcc")
public suspend fun vpnTunnel(`value`: Output) {
this.vpnTunnel = value
}
/**
* @param value The name or resource link to the
* VLAN interconnect for this interface. Changing this forces a new interface to
* be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("slajlxsjcyixdbnd")
public suspend fun interconnectAttachment(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.interconnectAttachment = mapped
}
/**
* @param value IP address and range of the interface. The IP range must be
* in the RFC3927 link-local IP space. Changing this forces a new interface to be created.
*/
@JvmName("xgoprgsgfgslngfh")
public suspend fun ipRange(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipRange = mapped
}
/**
* @param value IP version of this interface. Can be either IPV4 or IPV6.
*/
@JvmName("gqpnyxaiilmbrtna")
public suspend fun ipVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipVersion = mapped
}
/**
* @param value A unique name for the interface, required by GCE. Changing
* this forces a new interface to be created.
*/
@JvmName("jcilgpyyihpfegxp")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The regional private internal IP address that is used
* to establish BGP sessions to a VM instance acting as a third-party Router Appliance. Changing this forces a new interface to be created.
*/
@JvmName("ykbkuhnifeebprjr")
public suspend fun privateIpAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.privateIpAddress = mapped
}
/**
* @param value The ID of the project in which this interface's routerbelongs.
* If it is not provided, the provider project is used. Changing this forces a new interface to be created.
*/
@JvmName("rtokkflslaxrpsdo")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.project = mapped
}
/**
* @param value The name of the interface that is redundant to
* this interface. Changing this forces a new interface to be created.
*/
@JvmName("xoxeamrmjxjwaxcx")
public suspend fun redundantInterface(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redundantInterface = mapped
}
/**
* @param value The region this interface's router sits in.
* If not specified, the project region will be used. Changing this forces a new interface to be created.
*/
@JvmName("jwmyjwwycqkxyyyn")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value The name of the router this interface will be attached to.
* Changing this forces a new interface to be created.
* In addition to the above required fields, a router interface must have specified either `ip_range` or exactly one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork`, or both.
* - - -
*/
@JvmName("vgxqxamobhbclymi")
public suspend fun router(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.router = mapped
}
/**
* @param value The URI of the subnetwork resource that this interface
* belongs to, which must be in the same region as the Cloud Router. When you establish a BGP session to a VM instance using this interface, the VM instance must belong to the same subnetwork as the subnetwork specified here. Changing this forces a new interface to be created. Only one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("mdodnarlshavhfhg")
public suspend fun subnetwork(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subnetwork = mapped
}
/**
* @param value The name or resource link to the VPN tunnel this
* interface will be linked to. Changing this forces a new interface to be created. Only
* one of `vpn_tunnel`, `interconnect_attachment` or `subnetwork` can be specified.
*/
@JvmName("cwuvfxabaqxvhhbi")
public suspend fun vpnTunnel(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.vpnTunnel = mapped
}
internal fun build(): RouterInterfaceArgs = RouterInterfaceArgs(
interconnectAttachment = interconnectAttachment,
ipRange = ipRange,
ipVersion = ipVersion,
name = name,
privateIpAddress = privateIpAddress,
project = project,
redundantInterface = redundantInterface,
region = region,
router = router,
subnetwork = subnetwork,
vpnTunnel = vpnTunnel,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy