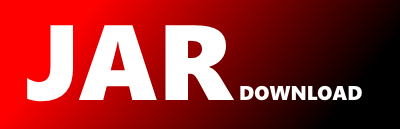
com.pulumi.gcp.compute.kotlin.SharedVPCServiceProjectArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.SharedVPCServiceProjectArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Enables the Google Compute Engine
* [Shared VPC](https://cloud.google.com/compute/docs/shared-vpc)
* feature for a project, assigning it as a Shared VPC service project associated
* with a given host project.
* For more information, see,
* [the Project API documentation](https://cloud.google.com/compute/docs/reference/latest/projects),
* where the Shared VPC feature is referred to by its former name "XPN".
* > **Note:** If Shared VPC Admin role is set at the folder level, use the google-beta provider. The google provider only supports this permission at project or organizational level currently. [[0]](https://cloud.google.com/vpc/docs/provisioning-shared-vpc#enable-shared-vpc-host)
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const service1 = new gcp.compute.SharedVPCServiceProject("service1", {
* hostProject: "host-project-id",
* serviceProject: "service-project-id-1",
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* service1 = gcp.compute.SharedVPCServiceProject("service1",
* host_project="host-project-id",
* service_project="service-project-id-1")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var service1 = new Gcp.Compute.SharedVPCServiceProject("service1", new()
* {
* HostProject = "host-project-id",
* ServiceProject = "service-project-id-1",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewSharedVPCServiceProject(ctx, "service1", &compute.SharedVPCServiceProjectArgs{
* HostProject: pulumi.String("host-project-id"),
* ServiceProject: pulumi.String("service-project-id-1"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.SharedVPCServiceProject;
* import com.pulumi.gcp.compute.SharedVPCServiceProjectArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var service1 = new SharedVPCServiceProject("service1", SharedVPCServiceProjectArgs.builder()
* .hostProject("host-project-id")
* .serviceProject("service-project-id-1")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* service1:
* type: gcp:compute:SharedVPCServiceProject
* properties:
* hostProject: host-project-id
* serviceProject: service-project-id-1
* ```
*
* For a complete Shared VPC example with both host and service projects, see
* [`gcp.compute.SharedVPCHostProject`](https://www.terraform.io/docs/providers/google/r/compute_shared_vpc_host_project.html).
* ## Import
* Google Compute Engine Shared VPC service project feature can be imported using the `host_project` and `service_project`, e.g.
* * `{{host_project}/{{service_project}}`
* When using the `pulumi import` command, Google Compute Engine Shared VPC service project can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/sharedVPCServiceProject:SharedVPCServiceProject default {{host_project}/{{service_project}}
* ```
* @property deletionPolicy The deletion policy for the shared VPC service. Setting ABANDON allows the resource to be abandoned rather than deleted. Possible values are: "ABANDON".
* @property hostProject The ID of a host project to associate.
* @property serviceProject The ID of the project that will serve as a Shared VPC service project.
*/
public data class SharedVPCServiceProjectArgs(
public val deletionPolicy: Output? = null,
public val hostProject: Output? = null,
public val serviceProject: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.SharedVPCServiceProjectArgs =
com.pulumi.gcp.compute.SharedVPCServiceProjectArgs.builder()
.deletionPolicy(deletionPolicy?.applyValue({ args0 -> args0 }))
.hostProject(hostProject?.applyValue({ args0 -> args0 }))
.serviceProject(serviceProject?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SharedVPCServiceProjectArgs].
*/
@PulumiTagMarker
public class SharedVPCServiceProjectArgsBuilder internal constructor() {
private var deletionPolicy: Output? = null
private var hostProject: Output? = null
private var serviceProject: Output? = null
/**
* @param value The deletion policy for the shared VPC service. Setting ABANDON allows the resource to be abandoned rather than deleted. Possible values are: "ABANDON".
*/
@JvmName("jkvfqjkhqbhaneyu")
public suspend fun deletionPolicy(`value`: Output) {
this.deletionPolicy = value
}
/**
* @param value The ID of a host project to associate.
*/
@JvmName("npmmtnewcdcmrdmc")
public suspend fun hostProject(`value`: Output) {
this.hostProject = value
}
/**
* @param value The ID of the project that will serve as a Shared VPC service project.
*/
@JvmName("fqfjkafuxijndush")
public suspend fun serviceProject(`value`: Output) {
this.serviceProject = value
}
/**
* @param value The deletion policy for the shared VPC service. Setting ABANDON allows the resource to be abandoned rather than deleted. Possible values are: "ABANDON".
*/
@JvmName("guvoldtcufgbvyoc")
public suspend fun deletionPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deletionPolicy = mapped
}
/**
* @param value The ID of a host project to associate.
*/
@JvmName("amhslrnissfhgosa")
public suspend fun hostProject(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostProject = mapped
}
/**
* @param value The ID of the project that will serve as a Shared VPC service project.
*/
@JvmName("cuecegghysgbelby")
public suspend fun serviceProject(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceProject = mapped
}
internal fun build(): SharedVPCServiceProjectArgs = SharedVPCServiceProjectArgs(
deletionPolicy = deletionPolicy,
hostProject = hostProject,
serviceProject = serviceProject,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy