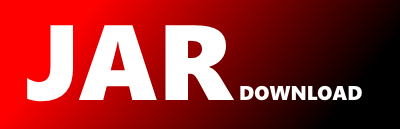
com.pulumi.gcp.compute.kotlin.URLMapArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.URLMapArgs.builder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapDefaultRouteActionArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapDefaultRouteActionArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapDefaultUrlRedirectArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapDefaultUrlRedirectArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapHeaderActionArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapHeaderActionArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapHostRuleArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapHostRuleArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapPathMatcherArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapPathMatcherArgsBuilder
import com.pulumi.gcp.compute.kotlin.inputs.URLMapTestArgs
import com.pulumi.gcp.compute.kotlin.inputs.URLMapTestArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* UrlMaps are used to route requests to a backend service based on rules
* that you define for the host and path of an incoming URL.
* To get more information about UrlMap, see:
* * [API documentation](https://cloud.google.com/compute/docs/reference/rest/v1/urlMaps)
* ## Example Usage
* ### Url Map Bucket And Service
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const login = new gcp.compute.BackendService("login", {
* name: "login",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: _default.id,
* });
* const staticBucket = new gcp.storage.Bucket("static", {
* name: "static-asset-bucket",
* location: "US",
* });
* const static = new gcp.compute.BackendBucket("static", {
* name: "static-asset-backend-bucket",
* bucketName: staticBucket.name,
* enableCdn: true,
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: static.id,
* hostRules: [
* {
* hosts: ["mysite.com"],
* pathMatcher: "mysite",
* },
* {
* hosts: ["myothersite.com"],
* pathMatcher: "otherpaths",
* },
* ],
* pathMatchers: [
* {
* name: "mysite",
* defaultService: static.id,
* pathRules: [
* {
* paths: ["/home"],
* service: static.id,
* },
* {
* paths: ["/login"],
* service: login.id,
* },
* {
* paths: ["/static"],
* service: static.id,
* },
* ],
* },
* {
* name: "otherpaths",
* defaultService: static.id,
* },
* ],
* tests: [{
* service: static.id,
* host: "example.com",
* path: "/home",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* login = gcp.compute.BackendService("login",
* name="login",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default.id)
* static_bucket = gcp.storage.Bucket("static",
* name="static-asset-bucket",
* location="US")
* static = gcp.compute.BackendBucket("static",
* name="static-asset-backend-bucket",
* bucket_name=static_bucket.name,
* enable_cdn=True)
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=static.id,
* host_rules=[
* gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="mysite",
* ),
* gcp.compute.URLMapHostRuleArgs(
* hosts=["myothersite.com"],
* path_matcher="otherpaths",
* ),
* ],
* path_matchers=[
* gcp.compute.URLMapPathMatcherArgs(
* name="mysite",
* default_service=static.id,
* path_rules=[
* gcp.compute.URLMapPathMatcherPathRuleArgs(
* paths=["/home"],
* service=static.id,
* ),
* gcp.compute.URLMapPathMatcherPathRuleArgs(
* paths=["/login"],
* service=login.id,
* ),
* gcp.compute.URLMapPathMatcherPathRuleArgs(
* paths=["/static"],
* service=static.id,
* ),
* ],
* ),
* gcp.compute.URLMapPathMatcherArgs(
* name="otherpaths",
* default_service=static.id,
* ),
* ],
* tests=[gcp.compute.URLMapTestArgs(
* service=static.id,
* host="example.com",
* path="/home",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var login = new Gcp.Compute.BackendService("login", new()
* {
* Name = "login",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = @default.Id,
* });
* var staticBucket = new Gcp.Storage.Bucket("static", new()
* {
* Name = "static-asset-bucket",
* Location = "US",
* });
* var @static = new Gcp.Compute.BackendBucket("static", new()
* {
* Name = "static-asset-backend-bucket",
* BucketName = staticBucket.Name,
* EnableCdn = true,
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = @static.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "mysite",
* },
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "myothersite.com",
* },
* PathMatcher = "otherpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "mysite",
* DefaultService = @static.Id,
* PathRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleArgs
* {
* Paths = new[]
* {
* "/home",
* },
* Service = @static.Id,
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleArgs
* {
* Paths = new[]
* {
* "/login",
* },
* Service = login.Id,
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleArgs
* {
* Paths = new[]
* {
* "/static",
* },
* Service = @static.Id,
* },
* },
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "otherpaths",
* DefaultService = @static.Id,
* },
* },
* Tests = new[]
* {
* new Gcp.Compute.Inputs.URLMapTestArgs
* {
* Service = @static.Id,
* Host = "example.com",
* Path = "/home",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* login, err := compute.NewBackendService(ctx, "login", &compute.BackendServiceArgs{
* Name: pulumi.String("login"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: _default.ID(),
* })
* if err != nil {
* return err
* }
* staticBucket, err := storage.NewBucket(ctx, "static", &storage.BucketArgs{
* Name: pulumi.String("static-asset-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* static, err := compute.NewBackendBucket(ctx, "static", &compute.BackendBucketArgs{
* Name: pulumi.String("static-asset-backend-bucket"),
* BucketName: staticBucket.Name,
* EnableCdn: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: static.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("mysite"),
* },
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("myothersite.com"),
* },
* PathMatcher: pulumi.String("otherpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("mysite"),
* DefaultService: static.ID(),
* PathRules: compute.URLMapPathMatcherPathRuleArray{
* &compute.URLMapPathMatcherPathRuleArgs{
* Paths: pulumi.StringArray{
* pulumi.String("/home"),
* },
* Service: static.ID(),
* },
* &compute.URLMapPathMatcherPathRuleArgs{
* Paths: pulumi.StringArray{
* pulumi.String("/login"),
* },
* Service: login.ID(),
* },
* &compute.URLMapPathMatcherPathRuleArgs{
* Paths: pulumi.StringArray{
* pulumi.String("/static"),
* },
* Service: static.ID(),
* },
* },
* },
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("otherpaths"),
* DefaultService: static.ID(),
* },
* },
* Tests: compute.URLMapTestArray{
* &compute.URLMapTestArgs{
* Service: static.ID(),
* Host: pulumi.String("example.com"),
* Path: pulumi.String("/home"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.compute.BackendBucket;
* import com.pulumi.gcp.compute.BackendBucketArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import com.pulumi.gcp.compute.inputs.URLMapTestArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HttpHealthCheck("default", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var login = new BackendService("login", BackendServiceArgs.builder()
* .name("login")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(default_.id())
* .build());
* var staticBucket = new Bucket("staticBucket", BucketArgs.builder()
* .name("static-asset-bucket")
* .location("US")
* .build());
* var static_ = new BackendBucket("static", BackendBucketArgs.builder()
* .name("static-asset-backend-bucket")
* .bucketName(staticBucket.name())
* .enableCdn(true)
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(static_.id())
* .hostRules(
* URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("mysite")
* .build(),
* URLMapHostRuleArgs.builder()
* .hosts("myothersite.com")
* .pathMatcher("otherpaths")
* .build())
* .pathMatchers(
* URLMapPathMatcherArgs.builder()
* .name("mysite")
* .defaultService(static_.id())
* .pathRules(
* URLMapPathMatcherPathRuleArgs.builder()
* .paths("/home")
* .service(static_.id())
* .build(),
* URLMapPathMatcherPathRuleArgs.builder()
* .paths("/login")
* .service(login.id())
* .build(),
* URLMapPathMatcherPathRuleArgs.builder()
* .paths("/static")
* .service(static_.id())
* .build())
* .build(),
* URLMapPathMatcherArgs.builder()
* .name("otherpaths")
* .defaultService(static_.id())
* .build())
* .tests(URLMapTestArgs.builder()
* .service(static_.id())
* .host("example.com")
* .path("/home")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${static.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: mysite
* - hosts:
* - myothersite.com
* pathMatcher: otherpaths
* pathMatchers:
* - name: mysite
* defaultService: ${static.id}
* pathRules:
* - paths:
* - /home
* service: ${static.id}
* - paths:
* - /login
* service: ${login.id}
* - paths:
* - /static
* service: ${static.id}
* - name: otherpaths
* defaultService: ${static.id}
* tests:
* - service: ${static.id}
* host: example.com
* path: /home
* login:
* type: gcp:compute:BackendService
* properties:
* name: login
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${default.id}
* default:
* type: gcp:compute:HttpHealthCheck
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* static:
* type: gcp:compute:BackendBucket
* properties:
* name: static-asset-backend-bucket
* bucketName: ${staticBucket.name}
* enableCdn: true
* staticBucket:
* type: gcp:storage:Bucket
* name: static
* properties:
* name: static-asset-bucket
* location: US
* ```
*
* ### Url Map Traffic Director Route
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HealthCheck("default", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const home = new gcp.compute.BackendService("home", {
* name: "home",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: _default.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: home.id,
* hostRules: [{
* hosts: ["mysite.com"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: home.id,
* routeRules: [{
* priority: 1,
* headerAction: {
* requestHeadersToRemoves: ["RemoveMe2"],
* requestHeadersToAdds: [{
* headerName: "AddSomethingElse",
* headerValue: "MyOtherValue",
* replace: true,
* }],
* responseHeadersToRemoves: ["RemoveMe3"],
* responseHeadersToAdds: [{
* headerName: "AddMe",
* headerValue: "MyValue",
* replace: false,
* }],
* },
* matchRules: [{
* fullPathMatch: "a full path",
* headerMatches: [{
* headerName: "someheader",
* exactMatch: "match this exactly",
* invertMatch: true,
* }],
* ignoreCase: true,
* metadataFilters: [{
* filterMatchCriteria: "MATCH_ANY",
* filterLabels: [{
* name: "PLANET",
* value: "MARS",
* }],
* }],
* queryParameterMatches: [{
* name: "a query parameter",
* presentMatch: true,
* }],
* }],
* urlRedirect: {
* hostRedirect: "A host",
* httpsRedirect: false,
* pathRedirect: "some/path",
* redirectResponseCode: "TEMPORARY_REDIRECT",
* stripQuery: true,
* },
* }],
* }],
* tests: [{
* service: home.id,
* host: "hi.com",
* path: "/home",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HealthCheck("default",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* home = gcp.compute.BackendService("home",
* name="home",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED")
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=home.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=home.id,
* route_rules=[gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=1,
* header_action=gcp.compute.URLMapPathMatcherRouteRuleHeaderActionArgs(
* request_headers_to_removes=["RemoveMe2"],
* request_headers_to_adds=[gcp.compute.URLMapPathMatcherRouteRuleHeaderActionRequestHeadersToAddArgs(
* header_name="AddSomethingElse",
* header_value="MyOtherValue",
* replace=True,
* )],
* response_headers_to_removes=["RemoveMe3"],
* response_headers_to_adds=[gcp.compute.URLMapPathMatcherRouteRuleHeaderActionResponseHeadersToAddArgs(
* header_name="AddMe",
* header_value="MyValue",
* replace=False,
* )],
* ),
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* full_path_match="a full path",
* header_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs(
* header_name="someheader",
* exact_match="match this exactly",
* invert_match=True,
* )],
* ignore_case=True,
* metadata_filters=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterArgs(
* filter_match_criteria="MATCH_ANY",
* filter_labels=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterFilterLabelArgs(
* name="PLANET",
* value="MARS",
* )],
* )],
* query_parameter_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs(
* name="a query parameter",
* present_match=True,
* )],
* )],
* url_redirect=gcp.compute.URLMapPathMatcherRouteRuleUrlRedirectArgs(
* host_redirect="A host",
* https_redirect=False,
* path_redirect="some/path",
* redirect_response_code="TEMPORARY_REDIRECT",
* strip_query=True,
* ),
* )],
* )],
* tests=[gcp.compute.URLMapTestArgs(
* service=home.id,
* host="hi.com",
* path="/home",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HealthCheck("default", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var home = new Gcp.Compute.BackendService("home", new()
* {
* Name = "home",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = @default.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = home.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = home.Id,
* RouteRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 1,
* HeaderAction = new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleHeaderActionArgs
* {
* RequestHeadersToRemoves = new[]
* {
* "RemoveMe2",
* },
* RequestHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleHeaderActionRequestHeadersToAddArgs
* {
* HeaderName = "AddSomethingElse",
* HeaderValue = "MyOtherValue",
* Replace = true,
* },
* },
* ResponseHeadersToRemoves = new[]
* {
* "RemoveMe3",
* },
* ResponseHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleHeaderActionResponseHeadersToAddArgs
* {
* HeaderName = "AddMe",
* HeaderValue = "MyValue",
* Replace = false,
* },
* },
* },
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* FullPathMatch = "a full path",
* HeaderMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs
* {
* HeaderName = "someheader",
* ExactMatch = "match this exactly",
* InvertMatch = true,
* },
* },
* IgnoreCase = true,
* MetadataFilters = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterArgs
* {
* FilterMatchCriteria = "MATCH_ANY",
* FilterLabels = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterFilterLabelArgs
* {
* Name = "PLANET",
* Value = "MARS",
* },
* },
* },
* },
* QueryParameterMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs
* {
* Name = "a query parameter",
* PresentMatch = true,
* },
* },
* },
* },
* UrlRedirect = new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleUrlRedirectArgs
* {
* HostRedirect = "A host",
* HttpsRedirect = false,
* PathRedirect = "some/path",
* RedirectResponseCode = "TEMPORARY_REDIRECT",
* StripQuery = true,
* },
* },
* },
* },
* },
* Tests = new[]
* {
* new Gcp.Compute.Inputs.URLMapTestArgs
* {
* Service = home.Id,
* Host = "hi.com",
* Path = "/home",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHealthCheck(ctx, "default", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* home, err := compute.NewBackendService(ctx, "home", &compute.BackendServiceArgs{
* Name: pulumi.String("home"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: _default.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: home.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: home.ID(),
* RouteRules: compute.URLMapPathMatcherRouteRuleArray{
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(1),
* HeaderAction: &compute.URLMapPathMatcherRouteRuleHeaderActionArgs{
* RequestHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe2"),
* },
* RequestHeadersToAdds: compute.URLMapPathMatcherRouteRuleHeaderActionRequestHeadersToAddArray{
* &compute.URLMapPathMatcherRouteRuleHeaderActionRequestHeadersToAddArgs{
* HeaderName: pulumi.String("AddSomethingElse"),
* HeaderValue: pulumi.String("MyOtherValue"),
* Replace: pulumi.Bool(true),
* },
* },
* ResponseHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe3"),
* },
* ResponseHeadersToAdds: compute.URLMapPathMatcherRouteRuleHeaderActionResponseHeadersToAddArray{
* &compute.URLMapPathMatcherRouteRuleHeaderActionResponseHeadersToAddArgs{
* HeaderName: pulumi.String("AddMe"),
* HeaderValue: pulumi.String("MyValue"),
* Replace: pulumi.Bool(false),
* },
* },
* },
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* FullPathMatch: pulumi.String("a full path"),
* HeaderMatches: compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs{
* HeaderName: pulumi.String("someheader"),
* ExactMatch: pulumi.String("match this exactly"),
* InvertMatch: pulumi.Bool(true),
* },
* },
* IgnoreCase: pulumi.Bool(true),
* MetadataFilters: compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterArgs{
* FilterMatchCriteria: pulumi.String("MATCH_ANY"),
* FilterLabels: compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterFilterLabelArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleMetadataFilterFilterLabelArgs{
* Name: pulumi.String("PLANET"),
* Value: pulumi.String("MARS"),
* },
* },
* },
* },
* QueryParameterMatches: compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs{
* Name: pulumi.String("a query parameter"),
* PresentMatch: pulumi.Bool(true),
* },
* },
* },
* },
* UrlRedirect: &compute.URLMapPathMatcherRouteRuleUrlRedirectArgs{
* HostRedirect: pulumi.String("A host"),
* HttpsRedirect: pulumi.Bool(false),
* PathRedirect: pulumi.String("some/path"),
* RedirectResponseCode: pulumi.String("TEMPORARY_REDIRECT"),
* StripQuery: pulumi.Bool(true),
* },
* },
* },
* },
* },
* Tests: compute.URLMapTestArray{
* &compute.URLMapTestArgs{
* Service: home.ID(),
* Host: pulumi.String("hi.com"),
* Path: pulumi.String("/home"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import com.pulumi.gcp.compute.inputs.URLMapTestArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HealthCheck("default", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var home = new BackendService("home", BackendServiceArgs.builder()
* .name("home")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(default_.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(home.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(home.id())
* .routeRules(URLMapPathMatcherRouteRuleArgs.builder()
* .priority(1)
* .headerAction(URLMapPathMatcherRouteRuleHeaderActionArgs.builder()
* .requestHeadersToRemoves("RemoveMe2")
* .requestHeadersToAdds(URLMapPathMatcherRouteRuleHeaderActionRequestHeadersToAddArgs.builder()
* .headerName("AddSomethingElse")
* .headerValue("MyOtherValue")
* .replace(true)
* .build())
* .responseHeadersToRemoves("RemoveMe3")
* .responseHeadersToAdds(URLMapPathMatcherRouteRuleHeaderActionResponseHeadersToAddArgs.builder()
* .headerName("AddMe")
* .headerValue("MyValue")
* .replace(false)
* .build())
* .build())
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .fullPathMatch("a full path")
* .headerMatches(URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs.builder()
* .headerName("someheader")
* .exactMatch("match this exactly")
* .invertMatch(true)
* .build())
* .ignoreCase(true)
* .metadataFilters(URLMapPathMatcherRouteRuleMatchRuleMetadataFilterArgs.builder()
* .filterMatchCriteria("MATCH_ANY")
* .filterLabels(URLMapPathMatcherRouteRuleMatchRuleMetadataFilterFilterLabelArgs.builder()
* .name("PLANET")
* .value("MARS")
* .build())
* .build())
* .queryParameterMatches(URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs.builder()
* .name("a query parameter")
* .presentMatch(true)
* .build())
* .build())
* .urlRedirect(URLMapPathMatcherRouteRuleUrlRedirectArgs.builder()
* .hostRedirect("A host")
* .httpsRedirect(false)
* .pathRedirect("some/path")
* .redirectResponseCode("TEMPORARY_REDIRECT")
* .stripQuery(true)
* .build())
* .build())
* .build())
* .tests(URLMapTestArgs.builder()
* .service(home.id())
* .host("hi.com")
* .path("/home")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${home.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${home.id}
* routeRules:
* - priority: 1
* headerAction:
* requestHeadersToRemoves:
* - RemoveMe2
* requestHeadersToAdds:
* - headerName: AddSomethingElse
* headerValue: MyOtherValue
* replace: true
* responseHeadersToRemoves:
* - RemoveMe3
* responseHeadersToAdds:
* - headerName: AddMe
* headerValue: MyValue
* replace: false
* matchRules:
* - fullPathMatch: a full path
* headerMatches:
* - headerName: someheader
* exactMatch: match this exactly
* invertMatch: true
* ignoreCase: true
* metadataFilters:
* - filterMatchCriteria: MATCH_ANY
* filterLabels:
* - name: PLANET
* value: MARS
* queryParameterMatches:
* - name: a query parameter
* presentMatch: true
* urlRedirect:
* hostRedirect: A host
* httpsRedirect: false
* pathRedirect: some/path
* redirectResponseCode: TEMPORARY_REDIRECT
* stripQuery: true
* tests:
* - service: ${home.id}
* host: hi.com
* path: /home
* home:
* type: gcp:compute:BackendService
* properties:
* name: home
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${default.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* default:
* type: gcp:compute:HealthCheck
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Url Map Traffic Director Route Partial
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HealthCheck("default", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const home = new gcp.compute.BackendService("home", {
* name: "home",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: _default.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: home.id,
* hostRules: [{
* hosts: ["mysite.com"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: home.id,
* routeRules: [{
* priority: 1,
* matchRules: [{
* prefixMatch: "/someprefix",
* headerMatches: [{
* headerName: "someheader",
* exactMatch: "match this exactly",
* invertMatch: true,
* }],
* }],
* urlRedirect: {
* pathRedirect: "some/path",
* redirectResponseCode: "TEMPORARY_REDIRECT",
* },
* }],
* }],
* tests: [{
* service: home.id,
* host: "hi.com",
* path: "/home",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HealthCheck("default",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* home = gcp.compute.BackendService("home",
* name="home",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED")
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=home.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=home.id,
* route_rules=[gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=1,
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* prefix_match="/someprefix",
* header_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs(
* header_name="someheader",
* exact_match="match this exactly",
* invert_match=True,
* )],
* )],
* url_redirect=gcp.compute.URLMapPathMatcherRouteRuleUrlRedirectArgs(
* path_redirect="some/path",
* redirect_response_code="TEMPORARY_REDIRECT",
* ),
* )],
* )],
* tests=[gcp.compute.URLMapTestArgs(
* service=home.id,
* host="hi.com",
* path="/home",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HealthCheck("default", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var home = new Gcp.Compute.BackendService("home", new()
* {
* Name = "home",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = @default.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = home.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = home.Id,
* RouteRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 1,
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* PrefixMatch = "/someprefix",
* HeaderMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs
* {
* HeaderName = "someheader",
* ExactMatch = "match this exactly",
* InvertMatch = true,
* },
* },
* },
* },
* UrlRedirect = new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleUrlRedirectArgs
* {
* PathRedirect = "some/path",
* RedirectResponseCode = "TEMPORARY_REDIRECT",
* },
* },
* },
* },
* },
* Tests = new[]
* {
* new Gcp.Compute.Inputs.URLMapTestArgs
* {
* Service = home.Id,
* Host = "hi.com",
* Path = "/home",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHealthCheck(ctx, "default", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* home, err := compute.NewBackendService(ctx, "home", &compute.BackendServiceArgs{
* Name: pulumi.String("home"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: _default.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: home.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: home.ID(),
* RouteRules: compute.URLMapPathMatcherRouteRuleArray{
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(1),
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* PrefixMatch: pulumi.String("/someprefix"),
* HeaderMatches: compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs{
* HeaderName: pulumi.String("someheader"),
* ExactMatch: pulumi.String("match this exactly"),
* InvertMatch: pulumi.Bool(true),
* },
* },
* },
* },
* UrlRedirect: &compute.URLMapPathMatcherRouteRuleUrlRedirectArgs{
* PathRedirect: pulumi.String("some/path"),
* RedirectResponseCode: pulumi.String("TEMPORARY_REDIRECT"),
* },
* },
* },
* },
* },
* Tests: compute.URLMapTestArray{
* &compute.URLMapTestArgs{
* Service: home.ID(),
* Host: pulumi.String("hi.com"),
* Path: pulumi.String("/home"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import com.pulumi.gcp.compute.inputs.URLMapTestArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HealthCheck("default", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var home = new BackendService("home", BackendServiceArgs.builder()
* .name("home")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(default_.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(home.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(home.id())
* .routeRules(URLMapPathMatcherRouteRuleArgs.builder()
* .priority(1)
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .prefixMatch("/someprefix")
* .headerMatches(URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs.builder()
* .headerName("someheader")
* .exactMatch("match this exactly")
* .invertMatch(true)
* .build())
* .build())
* .urlRedirect(URLMapPathMatcherRouteRuleUrlRedirectArgs.builder()
* .pathRedirect("some/path")
* .redirectResponseCode("TEMPORARY_REDIRECT")
* .build())
* .build())
* .build())
* .tests(URLMapTestArgs.builder()
* .service(home.id())
* .host("hi.com")
* .path("/home")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${home.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${home.id}
* routeRules:
* - priority: 1
* matchRules:
* - prefixMatch: /someprefix
* headerMatches:
* - headerName: someheader
* exactMatch: match this exactly
* invertMatch: true
* urlRedirect:
* pathRedirect: some/path
* redirectResponseCode: TEMPORARY_REDIRECT
* tests:
* - service: ${home.id}
* host: hi.com
* path: /home
* home:
* type: gcp:compute:BackendService
* properties:
* name: home
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${default.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* default:
* type: gcp:compute:HealthCheck
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Url Map Traffic Director Path
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HealthCheck("default", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const home = new gcp.compute.BackendService("home", {
* name: "home",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: _default.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: home.id,
* hostRules: [{
* hosts: ["mysite.com"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: home.id,
* pathRules: [{
* paths: ["/home"],
* routeAction: {
* corsPolicy: {
* allowCredentials: true,
* allowHeaders: ["Allowed content"],
* allowMethods: ["GET"],
* allowOriginRegexes: ["abc.*"],
* allowOrigins: ["Allowed origin"],
* exposeHeaders: ["Exposed header"],
* maxAge: 30,
* disabled: false,
* },
* faultInjectionPolicy: {
* abort: {
* httpStatus: 234,
* percentage: 5.6,
* },
* delay: {
* fixedDelay: {
* seconds: "0",
* nanos: 50000,
* },
* percentage: 7.8,
* },
* },
* requestMirrorPolicy: {
* backendService: home.id,
* },
* retryPolicy: {
* numRetries: 4,
* perTryTimeout: {
* seconds: "30",
* },
* retryConditions: [
* "5xx",
* "deadline-exceeded",
* ],
* },
* timeout: {
* seconds: "20",
* nanos: 750000000,
* },
* urlRewrite: {
* hostRewrite: "dev.example.com",
* pathPrefixRewrite: "/v1/api/",
* },
* weightedBackendServices: [{
* backendService: home.id,
* weight: 400,
* headerAction: {
* requestHeadersToRemoves: ["RemoveMe"],
* requestHeadersToAdds: [{
* headerName: "AddMe",
* headerValue: "MyValue",
* replace: true,
* }],
* responseHeadersToRemoves: ["RemoveMe"],
* responseHeadersToAdds: [{
* headerName: "AddMe",
* headerValue: "MyValue",
* replace: false,
* }],
* },
* }],
* },
* }],
* }],
* tests: [{
* service: home.id,
* host: "hi.com",
* path: "/home",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HealthCheck("default",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* home = gcp.compute.BackendService("home",
* name="home",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED")
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=home.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=home.id,
* path_rules=[gcp.compute.URLMapPathMatcherPathRuleArgs(
* paths=["/home"],
* route_action=gcp.compute.URLMapPathMatcherPathRuleRouteActionArgs(
* cors_policy=gcp.compute.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs(
* allow_credentials=True,
* allow_headers=["Allowed content"],
* allow_methods=["GET"],
* allow_origin_regexes=["abc.*"],
* allow_origins=["Allowed origin"],
* expose_headers=["Exposed header"],
* max_age=30,
* disabled=False,
* ),
* fault_injection_policy=gcp.compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyArgs(
* abort=gcp.compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyAbortArgs(
* http_status=234,
* percentage=5.6,
* ),
* delay=gcp.compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayArgs(
* fixed_delay=gcp.compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayFixedDelayArgs(
* seconds="0",
* nanos=50000,
* ),
* percentage=7.8,
* ),
* ),
* request_mirror_policy=gcp.compute.URLMapPathMatcherPathRuleRouteActionRequestMirrorPolicyArgs(
* backend_service=home.id,
* ),
* retry_policy=gcp.compute.URLMapPathMatcherPathRuleRouteActionRetryPolicyArgs(
* num_retries=4,
* per_try_timeout=gcp.compute.URLMapPathMatcherPathRuleRouteActionRetryPolicyPerTryTimeoutArgs(
* seconds="30",
* ),
* retry_conditions=[
* "5xx",
* "deadline-exceeded",
* ],
* ),
* timeout=gcp.compute.URLMapPathMatcherPathRuleRouteActionTimeoutArgs(
* seconds="20",
* nanos=750000000,
* ),
* url_rewrite=gcp.compute.URLMapPathMatcherPathRuleRouteActionUrlRewriteArgs(
* host_rewrite="dev.example.com",
* path_prefix_rewrite="/v1/api/",
* ),
* weighted_backend_services=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs(
* backend_service=home.id,
* weight=400,
* header_action=gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs(
* request_headers_to_removes=["RemoveMe"],
* request_headers_to_adds=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs(
* header_name="AddMe",
* header_value="MyValue",
* replace=True,
* )],
* response_headers_to_removes=["RemoveMe"],
* response_headers_to_adds=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs(
* header_name="AddMe",
* header_value="MyValue",
* replace=False,
* )],
* ),
* )],
* ),
* )],
* )],
* tests=[gcp.compute.URLMapTestArgs(
* service=home.id,
* host="hi.com",
* path="/home",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HealthCheck("default", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var home = new Gcp.Compute.BackendService("home", new()
* {
* Name = "home",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = @default.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = home.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = home.Id,
* PathRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleArgs
* {
* Paths = new[]
* {
* "/home",
* },
* RouteAction = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionArgs
* {
* CorsPolicy = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs
* {
* AllowCredentials = true,
* AllowHeaders = new[]
* {
* "Allowed content",
* },
* AllowMethods = new[]
* {
* "GET",
* },
* AllowOriginRegexes = new[]
* {
* "abc.*",
* },
* AllowOrigins = new[]
* {
* "Allowed origin",
* },
* ExposeHeaders = new[]
* {
* "Exposed header",
* },
* MaxAge = 30,
* Disabled = false,
* },
* FaultInjectionPolicy = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyArgs
* {
* Abort = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyAbortArgs
* {
* HttpStatus = 234,
* Percentage = 5.6,
* },
* Delay = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayArgs
* {
* FixedDelay = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayFixedDelayArgs
* {
* Seconds = "0",
* Nanos = 50000,
* },
* Percentage = 7.8,
* },
* },
* RequestMirrorPolicy = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionRequestMirrorPolicyArgs
* {
* BackendService = home.Id,
* },
* RetryPolicy = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionRetryPolicyArgs
* {
* NumRetries = 4,
* PerTryTimeout = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionRetryPolicyPerTryTimeoutArgs
* {
* Seconds = "30",
* },
* RetryConditions = new[]
* {
* "5xx",
* "deadline-exceeded",
* },
* },
* Timeout = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionTimeoutArgs
* {
* Seconds = "20",
* Nanos = 750000000,
* },
* UrlRewrite = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionUrlRewriteArgs
* {
* HostRewrite = "dev.example.com",
* PathPrefixRewrite = "/v1/api/",
* },
* WeightedBackendServices = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs
* {
* BackendService = home.Id,
* Weight = 400,
* HeaderAction = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs
* {
* RequestHeadersToRemoves = new[]
* {
* "RemoveMe",
* },
* RequestHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs
* {
* HeaderName = "AddMe",
* HeaderValue = "MyValue",
* Replace = true,
* },
* },
* ResponseHeadersToRemoves = new[]
* {
* "RemoveMe",
* },
* ResponseHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs
* {
* HeaderName = "AddMe",
* HeaderValue = "MyValue",
* Replace = false,
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* Tests = new[]
* {
* new Gcp.Compute.Inputs.URLMapTestArgs
* {
* Service = home.Id,
* Host = "hi.com",
* Path = "/home",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHealthCheck(ctx, "default", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* home, err := compute.NewBackendService(ctx, "home", &compute.BackendServiceArgs{
* Name: pulumi.String("home"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: _default.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: home.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: home.ID(),
* PathRules: compute.URLMapPathMatcherPathRuleArray{
* &compute.URLMapPathMatcherPathRuleArgs{
* Paths: pulumi.StringArray{
* pulumi.String("/home"),
* },
* RouteAction: &compute.URLMapPathMatcherPathRuleRouteActionArgs{
* CorsPolicy: &compute.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs{
* AllowCredentials: pulumi.Bool(true),
* AllowHeaders: pulumi.StringArray{
* pulumi.String("Allowed content"),
* },
* AllowMethods: pulumi.StringArray{
* pulumi.String("GET"),
* },
* AllowOriginRegexes: pulumi.StringArray{
* pulumi.String("abc.*"),
* },
* AllowOrigins: pulumi.StringArray{
* pulumi.String("Allowed origin"),
* },
* ExposeHeaders: pulumi.StringArray{
* pulumi.String("Exposed header"),
* },
* MaxAge: pulumi.Int(30),
* Disabled: pulumi.Bool(false),
* },
* FaultInjectionPolicy: &compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyArgs{
* Abort: &compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyAbortArgs{
* HttpStatus: pulumi.Int(234),
* Percentage: pulumi.Float64(5.6),
* },
* Delay: &compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayArgs{
* FixedDelay: &compute.URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayFixedDelayArgs{
* Seconds: pulumi.String("0"),
* Nanos: pulumi.Int(50000),
* },
* Percentage: pulumi.Float64(7.8),
* },
* },
* RequestMirrorPolicy: &compute.URLMapPathMatcherPathRuleRouteActionRequestMirrorPolicyArgs{
* BackendService: home.ID(),
* },
* RetryPolicy: &compute.URLMapPathMatcherPathRuleRouteActionRetryPolicyArgs{
* NumRetries: pulumi.Int(4),
* PerTryTimeout: &compute.URLMapPathMatcherPathRuleRouteActionRetryPolicyPerTryTimeoutArgs{
* Seconds: pulumi.String("30"),
* },
* RetryConditions: pulumi.StringArray{
* pulumi.String("5xx"),
* pulumi.String("deadline-exceeded"),
* },
* },
* Timeout: &compute.URLMapPathMatcherPathRuleRouteActionTimeoutArgs{
* Seconds: pulumi.String("20"),
* Nanos: pulumi.Int(750000000),
* },
* UrlRewrite: &compute.URLMapPathMatcherPathRuleRouteActionUrlRewriteArgs{
* HostRewrite: pulumi.String("dev.example.com"),
* PathPrefixRewrite: pulumi.String("/v1/api/"),
* },
* WeightedBackendServices: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs{
* BackendService: home.ID(),
* Weight: pulumi.Int(400),
* HeaderAction: &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs{
* RequestHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe"),
* },
* RequestHeadersToAdds: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs{
* HeaderName: pulumi.String("AddMe"),
* HeaderValue: pulumi.String("MyValue"),
* Replace: pulumi.Bool(true),
* },
* },
* ResponseHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe"),
* },
* ResponseHeadersToAdds: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs{
* HeaderName: pulumi.String("AddMe"),
* HeaderValue: pulumi.String("MyValue"),
* Replace: pulumi.Bool(false),
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* Tests: compute.URLMapTestArray{
* &compute.URLMapTestArgs{
* Service: home.ID(),
* Host: pulumi.String("hi.com"),
* Path: pulumi.String("/home"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import com.pulumi.gcp.compute.inputs.URLMapTestArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HealthCheck("default", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var home = new BackendService("home", BackendServiceArgs.builder()
* .name("home")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(default_.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(home.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(home.id())
* .pathRules(URLMapPathMatcherPathRuleArgs.builder()
* .paths("/home")
* .routeAction(URLMapPathMatcherPathRuleRouteActionArgs.builder()
* .corsPolicy(URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs.builder()
* .allowCredentials(true)
* .allowHeaders("Allowed content")
* .allowMethods("GET")
* .allowOriginRegexes("abc.*")
* .allowOrigins("Allowed origin")
* .exposeHeaders("Exposed header")
* .maxAge(30)
* .disabled(false)
* .build())
* .faultInjectionPolicy(URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyArgs.builder()
* .abort(URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyAbortArgs.builder()
* .httpStatus(234)
* .percentage(5.6)
* .build())
* .delay(URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayArgs.builder()
* .fixedDelay(URLMapPathMatcherPathRuleRouteActionFaultInjectionPolicyDelayFixedDelayArgs.builder()
* .seconds(0)
* .nanos(50000)
* .build())
* .percentage(7.8)
* .build())
* .build())
* .requestMirrorPolicy(URLMapPathMatcherPathRuleRouteActionRequestMirrorPolicyArgs.builder()
* .backendService(home.id())
* .build())
* .retryPolicy(URLMapPathMatcherPathRuleRouteActionRetryPolicyArgs.builder()
* .numRetries(4)
* .perTryTimeout(URLMapPathMatcherPathRuleRouteActionRetryPolicyPerTryTimeoutArgs.builder()
* .seconds(30)
* .build())
* .retryConditions(
* "5xx",
* "deadline-exceeded")
* .build())
* .timeout(URLMapPathMatcherPathRuleRouteActionTimeoutArgs.builder()
* .seconds(20)
* .nanos(750000000)
* .build())
* .urlRewrite(URLMapPathMatcherPathRuleRouteActionUrlRewriteArgs.builder()
* .hostRewrite("dev.example.com")
* .pathPrefixRewrite("/v1/api/")
* .build())
* .weightedBackendServices(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs.builder()
* .backendService(home.id())
* .weight(400)
* .headerAction(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs.builder()
* .requestHeadersToRemoves("RemoveMe")
* .requestHeadersToAdds(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs.builder()
* .headerName("AddMe")
* .headerValue("MyValue")
* .replace(true)
* .build())
* .responseHeadersToRemoves("RemoveMe")
* .responseHeadersToAdds(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs.builder()
* .headerName("AddMe")
* .headerValue("MyValue")
* .replace(false)
* .build())
* .build())
* .build())
* .build())
* .build())
* .build())
* .tests(URLMapTestArgs.builder()
* .service(home.id())
* .host("hi.com")
* .path("/home")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${home.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${home.id}
* pathRules:
* - paths:
* - /home
* routeAction:
* corsPolicy:
* allowCredentials: true
* allowHeaders:
* - Allowed content
* allowMethods:
* - GET
* allowOriginRegexes:
* - abc.*
* allowOrigins:
* - Allowed origin
* exposeHeaders:
* - Exposed header
* maxAge: 30
* disabled: false
* faultInjectionPolicy:
* abort:
* httpStatus: 234
* percentage: 5.6
* delay:
* fixedDelay:
* seconds: 0
* nanos: 50000
* percentage: 7.8
* requestMirrorPolicy:
* backendService: ${home.id}
* retryPolicy:
* numRetries: 4
* perTryTimeout:
* seconds: 30
* retryConditions:
* - 5xx
* - deadline-exceeded
* timeout:
* seconds: 20
* nanos: 7.5e+08
* urlRewrite:
* hostRewrite: dev.example.com
* pathPrefixRewrite: /v1/api/
* weightedBackendServices:
* - backendService: ${home.id}
* weight: 400
* headerAction:
* requestHeadersToRemoves:
* - RemoveMe
* requestHeadersToAdds:
* - headerName: AddMe
* headerValue: MyValue
* replace: true
* responseHeadersToRemoves:
* - RemoveMe
* responseHeadersToAdds:
* - headerName: AddMe
* headerValue: MyValue
* replace: false
* tests:
* - service: ${home.id}
* host: hi.com
* path: /home
* home:
* type: gcp:compute:BackendService
* properties:
* name: home
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${default.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* default:
* type: gcp:compute:HealthCheck
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Url Map Traffic Director Path Partial
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HealthCheck("default", {
* name: "health-check",
* httpHealthCheck: {
* port: 80,
* },
* });
* const home = new gcp.compute.BackendService("home", {
* name: "home",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: _default.id,
* loadBalancingScheme: "INTERNAL_SELF_MANAGED",
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: home.id,
* hostRules: [{
* hosts: ["mysite.com"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: home.id,
* pathRules: [{
* paths: ["/home"],
* routeAction: {
* corsPolicy: {
* allowCredentials: true,
* allowHeaders: ["Allowed content"],
* allowMethods: ["GET"],
* allowOriginRegexes: ["abc.*"],
* allowOrigins: ["Allowed origin"],
* exposeHeaders: ["Exposed header"],
* maxAge: 30,
* disabled: false,
* },
* weightedBackendServices: [{
* backendService: home.id,
* weight: 400,
* headerAction: {
* requestHeadersToRemoves: ["RemoveMe"],
* requestHeadersToAdds: [{
* headerName: "AddMe",
* headerValue: "MyValue",
* replace: true,
* }],
* responseHeadersToRemoves: ["RemoveMe"],
* responseHeadersToAdds: [{
* headerName: "AddMe",
* headerValue: "MyValue",
* replace: false,
* }],
* },
* }],
* },
* }],
* }],
* tests: [{
* service: home.id,
* host: "hi.com",
* path: "/home",
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HealthCheck("default",
* name="health-check",
* http_health_check=gcp.compute.HealthCheckHttpHealthCheckArgs(
* port=80,
* ))
* home = gcp.compute.BackendService("home",
* name="home",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default.id,
* load_balancing_scheme="INTERNAL_SELF_MANAGED")
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=home.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=home.id,
* path_rules=[gcp.compute.URLMapPathMatcherPathRuleArgs(
* paths=["/home"],
* route_action=gcp.compute.URLMapPathMatcherPathRuleRouteActionArgs(
* cors_policy=gcp.compute.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs(
* allow_credentials=True,
* allow_headers=["Allowed content"],
* allow_methods=["GET"],
* allow_origin_regexes=["abc.*"],
* allow_origins=["Allowed origin"],
* expose_headers=["Exposed header"],
* max_age=30,
* disabled=False,
* ),
* weighted_backend_services=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs(
* backend_service=home.id,
* weight=400,
* header_action=gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs(
* request_headers_to_removes=["RemoveMe"],
* request_headers_to_adds=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs(
* header_name="AddMe",
* header_value="MyValue",
* replace=True,
* )],
* response_headers_to_removes=["RemoveMe"],
* response_headers_to_adds=[gcp.compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs(
* header_name="AddMe",
* header_value="MyValue",
* replace=False,
* )],
* ),
* )],
* ),
* )],
* )],
* tests=[gcp.compute.URLMapTestArgs(
* service=home.id,
* host="hi.com",
* path="/home",
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HealthCheck("default", new()
* {
* Name = "health-check",
* HttpHealthCheck = new Gcp.Compute.Inputs.HealthCheckHttpHealthCheckArgs
* {
* Port = 80,
* },
* });
* var home = new Gcp.Compute.BackendService("home", new()
* {
* Name = "home",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = @default.Id,
* LoadBalancingScheme = "INTERNAL_SELF_MANAGED",
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = home.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = home.Id,
* PathRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleArgs
* {
* Paths = new[]
* {
* "/home",
* },
* RouteAction = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionArgs
* {
* CorsPolicy = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs
* {
* AllowCredentials = true,
* AllowHeaders = new[]
* {
* "Allowed content",
* },
* AllowMethods = new[]
* {
* "GET",
* },
* AllowOriginRegexes = new[]
* {
* "abc.*",
* },
* AllowOrigins = new[]
* {
* "Allowed origin",
* },
* ExposeHeaders = new[]
* {
* "Exposed header",
* },
* MaxAge = 30,
* Disabled = false,
* },
* WeightedBackendServices = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs
* {
* BackendService = home.Id,
* Weight = 400,
* HeaderAction = new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs
* {
* RequestHeadersToRemoves = new[]
* {
* "RemoveMe",
* },
* RequestHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs
* {
* HeaderName = "AddMe",
* HeaderValue = "MyValue",
* Replace = true,
* },
* },
* ResponseHeadersToRemoves = new[]
* {
* "RemoveMe",
* },
* ResponseHeadersToAdds = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs
* {
* HeaderName = "AddMe",
* HeaderValue = "MyValue",
* Replace = false,
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* Tests = new[]
* {
* new Gcp.Compute.Inputs.URLMapTestArgs
* {
* Service = home.Id,
* Host = "hi.com",
* Path = "/home",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHealthCheck(ctx, "default", &compute.HealthCheckArgs{
* Name: pulumi.String("health-check"),
* HttpHealthCheck: &compute.HealthCheckHttpHealthCheckArgs{
* Port: pulumi.Int(80),
* },
* })
* if err != nil {
* return err
* }
* home, err := compute.NewBackendService(ctx, "home", &compute.BackendServiceArgs{
* Name: pulumi.String("home"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: _default.ID(),
* LoadBalancingScheme: pulumi.String("INTERNAL_SELF_MANAGED"),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: home.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: home.ID(),
* PathRules: compute.URLMapPathMatcherPathRuleArray{
* &compute.URLMapPathMatcherPathRuleArgs{
* Paths: pulumi.StringArray{
* pulumi.String("/home"),
* },
* RouteAction: &compute.URLMapPathMatcherPathRuleRouteActionArgs{
* CorsPolicy: &compute.URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs{
* AllowCredentials: pulumi.Bool(true),
* AllowHeaders: pulumi.StringArray{
* pulumi.String("Allowed content"),
* },
* AllowMethods: pulumi.StringArray{
* pulumi.String("GET"),
* },
* AllowOriginRegexes: pulumi.StringArray{
* pulumi.String("abc.*"),
* },
* AllowOrigins: pulumi.StringArray{
* pulumi.String("Allowed origin"),
* },
* ExposeHeaders: pulumi.StringArray{
* pulumi.String("Exposed header"),
* },
* MaxAge: pulumi.Int(30),
* Disabled: pulumi.Bool(false),
* },
* WeightedBackendServices: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs{
* BackendService: home.ID(),
* Weight: pulumi.Int(400),
* HeaderAction: &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs{
* RequestHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe"),
* },
* RequestHeadersToAdds: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs{
* HeaderName: pulumi.String("AddMe"),
* HeaderValue: pulumi.String("MyValue"),
* Replace: pulumi.Bool(true),
* },
* },
* ResponseHeadersToRemoves: pulumi.StringArray{
* pulumi.String("RemoveMe"),
* },
* ResponseHeadersToAdds: compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArray{
* &compute.URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs{
* HeaderName: pulumi.String("AddMe"),
* HeaderValue: pulumi.String("MyValue"),
* Replace: pulumi.Bool(false),
* },
* },
* },
* },
* },
* },
* },
* },
* },
* },
* Tests: compute.URLMapTestArray{
* &compute.URLMapTestArgs{
* Service: home.ID(),
* Host: pulumi.String("hi.com"),
* Path: pulumi.String("/home"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HealthCheck;
* import com.pulumi.gcp.compute.HealthCheckArgs;
* import com.pulumi.gcp.compute.inputs.HealthCheckHttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import com.pulumi.gcp.compute.inputs.URLMapTestArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HealthCheck("default", HealthCheckArgs.builder()
* .name("health-check")
* .httpHealthCheck(HealthCheckHttpHealthCheckArgs.builder()
* .port(80)
* .build())
* .build());
* var home = new BackendService("home", BackendServiceArgs.builder()
* .name("home")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(default_.id())
* .loadBalancingScheme("INTERNAL_SELF_MANAGED")
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(home.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(home.id())
* .pathRules(URLMapPathMatcherPathRuleArgs.builder()
* .paths("/home")
* .routeAction(URLMapPathMatcherPathRuleRouteActionArgs.builder()
* .corsPolicy(URLMapPathMatcherPathRuleRouteActionCorsPolicyArgs.builder()
* .allowCredentials(true)
* .allowHeaders("Allowed content")
* .allowMethods("GET")
* .allowOriginRegexes("abc.*")
* .allowOrigins("Allowed origin")
* .exposeHeaders("Exposed header")
* .maxAge(30)
* .disabled(false)
* .build())
* .weightedBackendServices(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceArgs.builder()
* .backendService(home.id())
* .weight(400)
* .headerAction(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionArgs.builder()
* .requestHeadersToRemoves("RemoveMe")
* .requestHeadersToAdds(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionRequestHeadersToAddArgs.builder()
* .headerName("AddMe")
* .headerValue("MyValue")
* .replace(true)
* .build())
* .responseHeadersToRemoves("RemoveMe")
* .responseHeadersToAdds(URLMapPathMatcherPathRuleRouteActionWeightedBackendServiceHeaderActionResponseHeadersToAddArgs.builder()
* .headerName("AddMe")
* .headerValue("MyValue")
* .replace(false)
* .build())
* .build())
* .build())
* .build())
* .build())
* .build())
* .tests(URLMapTestArgs.builder()
* .service(home.id())
* .host("hi.com")
* .path("/home")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${home.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${home.id}
* pathRules:
* - paths:
* - /home
* routeAction:
* corsPolicy:
* allowCredentials: true
* allowHeaders:
* - Allowed content
* allowMethods:
* - GET
* allowOriginRegexes:
* - abc.*
* allowOrigins:
* - Allowed origin
* exposeHeaders:
* - Exposed header
* maxAge: 30
* disabled: false
* weightedBackendServices:
* - backendService: ${home.id}
* weight: 400
* headerAction:
* requestHeadersToRemoves:
* - RemoveMe
* requestHeadersToAdds:
* - headerName: AddMe
* headerValue: MyValue
* replace: true
* responseHeadersToRemoves:
* - RemoveMe
* responseHeadersToAdds:
* - headerName: AddMe
* headerValue: MyValue
* replace: false
* tests:
* - service: ${home.id}
* host: hi.com
* path: /home
* home:
* type: gcp:compute:BackendService
* properties:
* name: home
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${default.id}
* loadBalancingScheme: INTERNAL_SELF_MANAGED
* default:
* type: gcp:compute:HealthCheck
* properties:
* name: health-check
* httpHealthCheck:
* port: 80
* ```
*
* ### Url Map Header Based Routing
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "default",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const service_a = new gcp.compute.BackendService("service-a", {
* name: "service-a",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const service_b = new gcp.compute.BackendService("service-b", {
* name: "service-b",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "header-based routing example",
* defaultService: _default.id,
* hostRules: [{
* hosts: ["*"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: _default.id,
* routeRules: [
* {
* priority: 1,
* service: service_a.id,
* matchRules: [{
* prefixMatch: "/",
* ignoreCase: true,
* headerMatches: [{
* headerName: "abtest",
* exactMatch: "a",
* }],
* }],
* },
* {
* priority: 2,
* service: service_b.id,
* matchRules: [{
* ignoreCase: true,
* prefixMatch: "/",
* headerMatches: [{
* headerName: "abtest",
* exactMatch: "b",
* }],
* }],
* },
* ],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="default",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* service_a = gcp.compute.BackendService("service-a",
* name="service-a",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* service_b = gcp.compute.BackendService("service-b",
* name="service-b",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="header-based routing example",
* default_service=default.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["*"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=default.id,
* route_rules=[
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=1,
* service=service_a.id,
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* prefix_match="/",
* ignore_case=True,
* header_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs(
* header_name="abtest",
* exact_match="a",
* )],
* )],
* ),
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=2,
* service=service_b.id,
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* ignore_case=True,
* prefix_match="/",
* header_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs(
* header_name="abtest",
* exact_match="b",
* )],
* )],
* ),
* ],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "default",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var service_a = new Gcp.Compute.BackendService("service-a", new()
* {
* Name = "service-a",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var service_b = new Gcp.Compute.BackendService("service-b", new()
* {
* Name = "service-b",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "header-based routing example",
* DefaultService = @default.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "*",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = @default.Id,
* RouteRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 1,
* Service = service_a.Id,
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* PrefixMatch = "/",
* IgnoreCase = true,
* HeaderMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs
* {
* HeaderName = "abtest",
* ExactMatch = "a",
* },
* },
* },
* },
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 2,
* Service = service_b.Id,
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* IgnoreCase = true,
* PrefixMatch = "/",
* HeaderMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs
* {
* HeaderName = "abtest",
* ExactMatch = "b",
* },
* },
* },
* },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("default"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "service-a", &compute.BackendServiceArgs{
* Name: pulumi.String("service-a"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "service-b", &compute.BackendServiceArgs{
* Name: pulumi.String("service-b"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("header-based routing example"),
* DefaultService: _default.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("*"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: _default.ID(),
* RouteRules: compute.URLMapPathMatcherRouteRuleArray{
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(1),
* Service: service_a.ID(),
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* PrefixMatch: pulumi.String("/"),
* IgnoreCase: pulumi.Bool(true),
* HeaderMatches: compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs{
* HeaderName: pulumi.String("abtest"),
* ExactMatch: pulumi.String("a"),
* },
* },
* },
* },
* },
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(2),
* Service: service_b.ID(),
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* IgnoreCase: pulumi.Bool(true),
* PrefixMatch: pulumi.String("/"),
* HeaderMatches: compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs{
* HeaderName: pulumi.String("abtest"),
* ExactMatch: pulumi.String("b"),
* },
* },
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("default")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var service_a = new BackendService("service-a", BackendServiceArgs.builder()
* .name("service-a")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var service_b = new BackendService("service-b", BackendServiceArgs.builder()
* .name("service-b")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("header-based routing example")
* .defaultService(default_.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("*")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(default_.id())
* .routeRules(
* URLMapPathMatcherRouteRuleArgs.builder()
* .priority(1)
* .service(service_a.id())
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .prefixMatch("/")
* .ignoreCase(true)
* .headerMatches(URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs.builder()
* .headerName("abtest")
* .exactMatch("a")
* .build())
* .build())
* .build(),
* URLMapPathMatcherRouteRuleArgs.builder()
* .priority(2)
* .service(service_b.id())
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .ignoreCase(true)
* .prefixMatch("/")
* .headerMatches(URLMapPathMatcherRouteRuleMatchRuleHeaderMatchArgs.builder()
* .headerName("abtest")
* .exactMatch("b")
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: header-based routing example
* defaultService: ${default.id}
* hostRules:
* - hosts:
* - '*'
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${default.id}
* routeRules:
* - priority: 1
* service: ${["service-a"].id}
* matchRules:
* - prefixMatch: /
* ignoreCase: true
* headerMatches:
* - headerName: abtest
* exactMatch: a
* - priority: 2
* service: ${["service-b"].id}
* matchRules:
* - ignoreCase: true
* prefixMatch: /
* headerMatches:
* - headerName: abtest
* exactMatch: b
* default:
* type: gcp:compute:BackendService
* properties:
* name: default
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* service-a:
* type: gcp:compute:BackendService
* properties:
* name: service-a
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* service-b:
* type: gcp:compute:BackendService
* properties:
* name: service-b
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Url Map Parameter Based Routing
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const defaultHttpHealthCheck = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const _default = new gcp.compute.BackendService("default", {
* name: "default",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const service_a = new gcp.compute.BackendService("service-a", {
* name: "service-a",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const service_b = new gcp.compute.BackendService("service-b", {
* name: "service-b",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* healthChecks: defaultHttpHealthCheck.id,
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "parameter-based routing example",
* defaultService: _default.id,
* hostRules: [{
* hosts: ["*"],
* pathMatcher: "allpaths",
* }],
* pathMatchers: [{
* name: "allpaths",
* defaultService: _default.id,
* routeRules: [
* {
* priority: 1,
* service: service_a.id,
* matchRules: [{
* prefixMatch: "/",
* ignoreCase: true,
* queryParameterMatches: [{
* name: "abtest",
* exactMatch: "a",
* }],
* }],
* },
* {
* priority: 2,
* service: service_b.id,
* matchRules: [{
* ignoreCase: true,
* prefixMatch: "/",
* queryParameterMatches: [{
* name: "abtest",
* exactMatch: "b",
* }],
* }],
* },
* ],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default_http_health_check = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* default = gcp.compute.BackendService("default",
* name="default",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* service_a = gcp.compute.BackendService("service-a",
* name="service-a",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* service_b = gcp.compute.BackendService("service-b",
* name="service-b",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* health_checks=default_http_health_check.id)
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="parameter-based routing example",
* default_service=default.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["*"],
* path_matcher="allpaths",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="allpaths",
* default_service=default.id,
* route_rules=[
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=1,
* service=service_a.id,
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* prefix_match="/",
* ignore_case=True,
* query_parameter_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs(
* name="abtest",
* exact_match="a",
* )],
* )],
* ),
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* priority=2,
* service=service_b.id,
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* ignore_case=True,
* prefix_match="/",
* query_parameter_matches=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs(
* name="abtest",
* exact_match="b",
* )],
* )],
* ),
* ],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var defaultHttpHealthCheck = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var @default = new Gcp.Compute.BackendService("default", new()
* {
* Name = "default",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var service_a = new Gcp.Compute.BackendService("service-a", new()
* {
* Name = "service-a",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var service_b = new Gcp.Compute.BackendService("service-b", new()
* {
* Name = "service-b",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* HealthChecks = defaultHttpHealthCheck.Id,
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "parameter-based routing example",
* DefaultService = @default.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "*",
* },
* PathMatcher = "allpaths",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "allpaths",
* DefaultService = @default.Id,
* RouteRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 1,
* Service = service_a.Id,
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* PrefixMatch = "/",
* IgnoreCase = true,
* QueryParameterMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs
* {
* Name = "abtest",
* ExactMatch = "a",
* },
* },
* },
* },
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* Priority = 2,
* Service = service_b.Id,
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* IgnoreCase = true,
* PrefixMatch = "/",
* QueryParameterMatches = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs
* {
* Name = "abtest",
* ExactMatch = "b",
* },
* },
* },
* },
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* defaultHttpHealthCheck, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "default", &compute.BackendServiceArgs{
* Name: pulumi.String("default"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "service-a", &compute.BackendServiceArgs{
* Name: pulumi.String("service-a"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "service-b", &compute.BackendServiceArgs{
* Name: pulumi.String("service-b"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* HealthChecks: defaultHttpHealthCheck.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("parameter-based routing example"),
* DefaultService: _default.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("*"),
* },
* PathMatcher: pulumi.String("allpaths"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("allpaths"),
* DefaultService: _default.ID(),
* RouteRules: compute.URLMapPathMatcherRouteRuleArray{
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(1),
* Service: service_a.ID(),
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* PrefixMatch: pulumi.String("/"),
* IgnoreCase: pulumi.Bool(true),
* QueryParameterMatches: compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs{
* Name: pulumi.String("abtest"),
* ExactMatch: pulumi.String("a"),
* },
* },
* },
* },
* },
* &compute.URLMapPathMatcherRouteRuleArgs{
* Priority: pulumi.Int(2),
* Service: service_b.ID(),
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* IgnoreCase: pulumi.Bool(true),
* PrefixMatch: pulumi.String("/"),
* QueryParameterMatches: compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs{
* Name: pulumi.String("abtest"),
* ExactMatch: pulumi.String("b"),
* },
* },
* },
* },
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var defaultHttpHealthCheck = new HttpHealthCheck("defaultHttpHealthCheck", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var default_ = new BackendService("default", BackendServiceArgs.builder()
* .name("default")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var service_a = new BackendService("service-a", BackendServiceArgs.builder()
* .name("service-a")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var service_b = new BackendService("service-b", BackendServiceArgs.builder()
* .name("service-b")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .healthChecks(defaultHttpHealthCheck.id())
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("parameter-based routing example")
* .defaultService(default_.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("*")
* .pathMatcher("allpaths")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("allpaths")
* .defaultService(default_.id())
* .routeRules(
* URLMapPathMatcherRouteRuleArgs.builder()
* .priority(1)
* .service(service_a.id())
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .prefixMatch("/")
* .ignoreCase(true)
* .queryParameterMatches(URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs.builder()
* .name("abtest")
* .exactMatch("a")
* .build())
* .build())
* .build(),
* URLMapPathMatcherRouteRuleArgs.builder()
* .priority(2)
* .service(service_b.id())
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .ignoreCase(true)
* .prefixMatch("/")
* .queryParameterMatches(URLMapPathMatcherRouteRuleMatchRuleQueryParameterMatchArgs.builder()
* .name("abtest")
* .exactMatch("b")
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: parameter-based routing example
* defaultService: ${default.id}
* hostRules:
* - hosts:
* - '*'
* pathMatcher: allpaths
* pathMatchers:
* - name: allpaths
* defaultService: ${default.id}
* routeRules:
* - priority: 1
* service: ${["service-a"].id}
* matchRules:
* - prefixMatch: /
* ignoreCase: true
* queryParameterMatches:
* - name: abtest
* exactMatch: a
* - priority: 2
* service: ${["service-b"].id}
* matchRules:
* - ignoreCase: true
* prefixMatch: /
* queryParameterMatches:
* - name: abtest
* exactMatch: b
* default:
* type: gcp:compute:BackendService
* properties:
* name: default
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* service-a:
* type: gcp:compute:BackendService
* properties:
* name: service-a
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* service-b:
* type: gcp:compute:BackendService
* properties:
* name: service-b
* portName: http
* protocol: HTTP
* timeoutSec: 10
* healthChecks: ${defaultHttpHealthCheck.id}
* defaultHttpHealthCheck:
* type: gcp:compute:HttpHealthCheck
* name: default
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* ```
*
* ### Url Map Path Template Match
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as gcp from "@pulumi/gcp";
* const _default = new gcp.compute.HttpHealthCheck("default", {
* name: "health-check",
* requestPath: "/",
* checkIntervalSec: 1,
* timeoutSec: 1,
* });
* const cart_backend = new gcp.compute.BackendService("cart-backend", {
* name: "cart-service",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* loadBalancingScheme: "EXTERNAL_MANAGED",
* healthChecks: _default.id,
* });
* const user_backend = new gcp.compute.BackendService("user-backend", {
* name: "user-service",
* portName: "http",
* protocol: "HTTP",
* timeoutSec: 10,
* loadBalancingScheme: "EXTERNAL_MANAGED",
* healthChecks: _default.id,
* });
* const staticBucket = new gcp.storage.Bucket("static", {
* name: "static-asset-bucket",
* location: "US",
* });
* const static = new gcp.compute.BackendBucket("static", {
* name: "static-asset-backend-bucket",
* bucketName: staticBucket.name,
* enableCdn: true,
* });
* const urlmap = new gcp.compute.URLMap("urlmap", {
* name: "urlmap",
* description: "a description",
* defaultService: static.id,
* hostRules: [{
* hosts: ["mysite.com"],
* pathMatcher: "mysite",
* }],
* pathMatchers: [{
* name: "mysite",
* defaultService: static.id,
* routeRules: [
* {
* matchRules: [{
* pathTemplateMatch: "/xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}",
* }],
* service: cart_backend.id,
* priority: 1,
* routeAction: {
* urlRewrite: {
* pathTemplateRewrite: "/{username}-{cartid}/",
* },
* },
* },
* {
* matchRules: [{
* pathTemplateMatch: "/xyzwebservices/v2/xyz/users/*/accountinfo/*",
* }],
* service: user_backend.id,
* priority: 2,
* },
* ],
* }],
* });
* ```
* ```python
* import pulumi
* import pulumi_gcp as gcp
* default = gcp.compute.HttpHealthCheck("default",
* name="health-check",
* request_path="/",
* check_interval_sec=1,
* timeout_sec=1)
* cart_backend = gcp.compute.BackendService("cart-backend",
* name="cart-service",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* load_balancing_scheme="EXTERNAL_MANAGED",
* health_checks=default.id)
* user_backend = gcp.compute.BackendService("user-backend",
* name="user-service",
* port_name="http",
* protocol="HTTP",
* timeout_sec=10,
* load_balancing_scheme="EXTERNAL_MANAGED",
* health_checks=default.id)
* static_bucket = gcp.storage.Bucket("static",
* name="static-asset-bucket",
* location="US")
* static = gcp.compute.BackendBucket("static",
* name="static-asset-backend-bucket",
* bucket_name=static_bucket.name,
* enable_cdn=True)
* urlmap = gcp.compute.URLMap("urlmap",
* name="urlmap",
* description="a description",
* default_service=static.id,
* host_rules=[gcp.compute.URLMapHostRuleArgs(
* hosts=["mysite.com"],
* path_matcher="mysite",
* )],
* path_matchers=[gcp.compute.URLMapPathMatcherArgs(
* name="mysite",
* default_service=static.id,
* route_rules=[
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* path_template_match="/xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}",
* )],
* service=cart_backend.id,
* priority=1,
* route_action=gcp.compute.URLMapPathMatcherRouteRuleRouteActionArgs(
* url_rewrite=gcp.compute.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs(
* path_template_rewrite="/{username}-{cartid}/",
* ),
* ),
* ),
* gcp.compute.URLMapPathMatcherRouteRuleArgs(
* match_rules=[gcp.compute.URLMapPathMatcherRouteRuleMatchRuleArgs(
* path_template_match="/xyzwebservices/v2/xyz/users/*/accountinfo/*",
* )],
* service=user_backend.id,
* priority=2,
* ),
* ],
* )])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Gcp = Pulumi.Gcp;
* return await Deployment.RunAsync(() =>
* {
* var @default = new Gcp.Compute.HttpHealthCheck("default", new()
* {
* Name = "health-check",
* RequestPath = "/",
* CheckIntervalSec = 1,
* TimeoutSec = 1,
* });
* var cart_backend = new Gcp.Compute.BackendService("cart-backend", new()
* {
* Name = "cart-service",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* LoadBalancingScheme = "EXTERNAL_MANAGED",
* HealthChecks = @default.Id,
* });
* var user_backend = new Gcp.Compute.BackendService("user-backend", new()
* {
* Name = "user-service",
* PortName = "http",
* Protocol = "HTTP",
* TimeoutSec = 10,
* LoadBalancingScheme = "EXTERNAL_MANAGED",
* HealthChecks = @default.Id,
* });
* var staticBucket = new Gcp.Storage.Bucket("static", new()
* {
* Name = "static-asset-bucket",
* Location = "US",
* });
* var @static = new Gcp.Compute.BackendBucket("static", new()
* {
* Name = "static-asset-backend-bucket",
* BucketName = staticBucket.Name,
* EnableCdn = true,
* });
* var urlmap = new Gcp.Compute.URLMap("urlmap", new()
* {
* Name = "urlmap",
* Description = "a description",
* DefaultService = @static.Id,
* HostRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapHostRuleArgs
* {
* Hosts = new[]
* {
* "mysite.com",
* },
* PathMatcher = "mysite",
* },
* },
* PathMatchers = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherArgs
* {
* Name = "mysite",
* DefaultService = @static.Id,
* RouteRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* PathTemplateMatch = "/xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}",
* },
* },
* Service = cart_backend.Id,
* Priority = 1,
* RouteAction = new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleRouteActionArgs
* {
* UrlRewrite = new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs
* {
* PathTemplateRewrite = "/{username}-{cartid}/",
* },
* },
* },
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleArgs
* {
* MatchRules = new[]
* {
* new Gcp.Compute.Inputs.URLMapPathMatcherRouteRuleMatchRuleArgs
* {
* PathTemplateMatch = "/xyzwebservices/v2/xyz/users/*/accountinfo/*",
* },
* },
* Service = user_backend.Id,
* Priority = 2,
* },
* },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/compute"
* "github.com/pulumi/pulumi-gcp/sdk/v7/go/gcp/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := compute.NewHttpHealthCheck(ctx, "default", &compute.HttpHealthCheckArgs{
* Name: pulumi.String("health-check"),
* RequestPath: pulumi.String("/"),
* CheckIntervalSec: pulumi.Int(1),
* TimeoutSec: pulumi.Int(1),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "cart-backend", &compute.BackendServiceArgs{
* Name: pulumi.String("cart-service"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* LoadBalancingScheme: pulumi.String("EXTERNAL_MANAGED"),
* HealthChecks: _default.ID(),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewBackendService(ctx, "user-backend", &compute.BackendServiceArgs{
* Name: pulumi.String("user-service"),
* PortName: pulumi.String("http"),
* Protocol: pulumi.String("HTTP"),
* TimeoutSec: pulumi.Int(10),
* LoadBalancingScheme: pulumi.String("EXTERNAL_MANAGED"),
* HealthChecks: _default.ID(),
* })
* if err != nil {
* return err
* }
* staticBucket, err := storage.NewBucket(ctx, "static", &storage.BucketArgs{
* Name: pulumi.String("static-asset-bucket"),
* Location: pulumi.String("US"),
* })
* if err != nil {
* return err
* }
* static, err := compute.NewBackendBucket(ctx, "static", &compute.BackendBucketArgs{
* Name: pulumi.String("static-asset-backend-bucket"),
* BucketName: staticBucket.Name,
* EnableCdn: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = compute.NewURLMap(ctx, "urlmap", &compute.URLMapArgs{
* Name: pulumi.String("urlmap"),
* Description: pulumi.String("a description"),
* DefaultService: static.ID(),
* HostRules: compute.URLMapHostRuleArray{
* &compute.URLMapHostRuleArgs{
* Hosts: pulumi.StringArray{
* pulumi.String("mysite.com"),
* },
* PathMatcher: pulumi.String("mysite"),
* },
* },
* PathMatchers: compute.URLMapPathMatcherArray{
* &compute.URLMapPathMatcherArgs{
* Name: pulumi.String("mysite"),
* DefaultService: static.ID(),
* RouteRules: compute.URLMapPathMatcherRouteRuleArray{
* &compute.URLMapPathMatcherRouteRuleArgs{
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* PathTemplateMatch: pulumi.String("/xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}"),
* },
* },
* Service: cart_backend.ID(),
* Priority: pulumi.Int(1),
* RouteAction: &compute.URLMapPathMatcherRouteRuleRouteActionArgs{
* UrlRewrite: &compute.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs{
* PathTemplateRewrite: pulumi.String("/{username}-{cartid}/"),
* },
* },
* },
* &compute.URLMapPathMatcherRouteRuleArgs{
* MatchRules: compute.URLMapPathMatcherRouteRuleMatchRuleArray{
* &compute.URLMapPathMatcherRouteRuleMatchRuleArgs{
* PathTemplateMatch: pulumi.String("/xyzwebservices/v2/xyz/users/*/accountinfo/*"),
* },
* },
* Service: user_backend.ID(),
* Priority: pulumi.Int(2),
* },
* },
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.gcp.compute.HttpHealthCheck;
* import com.pulumi.gcp.compute.HttpHealthCheckArgs;
* import com.pulumi.gcp.compute.BackendService;
* import com.pulumi.gcp.compute.BackendServiceArgs;
* import com.pulumi.gcp.storage.Bucket;
* import com.pulumi.gcp.storage.BucketArgs;
* import com.pulumi.gcp.compute.BackendBucket;
* import com.pulumi.gcp.compute.BackendBucketArgs;
* import com.pulumi.gcp.compute.URLMap;
* import com.pulumi.gcp.compute.URLMapArgs;
* import com.pulumi.gcp.compute.inputs.URLMapHostRuleArgs;
* import com.pulumi.gcp.compute.inputs.URLMapPathMatcherArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var default_ = new HttpHealthCheck("default", HttpHealthCheckArgs.builder()
* .name("health-check")
* .requestPath("/")
* .checkIntervalSec(1)
* .timeoutSec(1)
* .build());
* var cart_backend = new BackendService("cart-backend", BackendServiceArgs.builder()
* .name("cart-service")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .loadBalancingScheme("EXTERNAL_MANAGED")
* .healthChecks(default_.id())
* .build());
* var user_backend = new BackendService("user-backend", BackendServiceArgs.builder()
* .name("user-service")
* .portName("http")
* .protocol("HTTP")
* .timeoutSec(10)
* .loadBalancingScheme("EXTERNAL_MANAGED")
* .healthChecks(default_.id())
* .build());
* var staticBucket = new Bucket("staticBucket", BucketArgs.builder()
* .name("static-asset-bucket")
* .location("US")
* .build());
* var static_ = new BackendBucket("static", BackendBucketArgs.builder()
* .name("static-asset-backend-bucket")
* .bucketName(staticBucket.name())
* .enableCdn(true)
* .build());
* var urlmap = new URLMap("urlmap", URLMapArgs.builder()
* .name("urlmap")
* .description("a description")
* .defaultService(static_.id())
* .hostRules(URLMapHostRuleArgs.builder()
* .hosts("mysite.com")
* .pathMatcher("mysite")
* .build())
* .pathMatchers(URLMapPathMatcherArgs.builder()
* .name("mysite")
* .defaultService(static_.id())
* .routeRules(
* URLMapPathMatcherRouteRuleArgs.builder()
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .pathTemplateMatch("/xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}")
* .build())
* .service(cart_backend.id())
* .priority(1)
* .routeAction(URLMapPathMatcherRouteRuleRouteActionArgs.builder()
* .urlRewrite(URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs.builder()
* .pathTemplateRewrite("/{username}-{cartid}/")
* .build())
* .build())
* .build(),
* URLMapPathMatcherRouteRuleArgs.builder()
* .matchRules(URLMapPathMatcherRouteRuleMatchRuleArgs.builder()
* .pathTemplateMatch("/xyzwebservices/v2/xyz/users/*/accountinfo/*")
* .build())
* .service(user_backend.id())
* .priority(2)
* .build())
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* urlmap:
* type: gcp:compute:URLMap
* properties:
* name: urlmap
* description: a description
* defaultService: ${static.id}
* hostRules:
* - hosts:
* - mysite.com
* pathMatcher: mysite
* pathMatchers:
* - name: mysite
* defaultService: ${static.id}
* routeRules:
* - matchRules:
* - pathTemplateMatch: /xyzwebservices/v2/xyz/users/{username=*}/carts/{cartid=**}
* service: ${["cart-backend"].id}
* priority: 1
* routeAction:
* urlRewrite:
* pathTemplateRewrite: /{username}-{cartid}/
* - matchRules:
* - pathTemplateMatch: /xyzwebservices/v2/xyz/users/*/accountinfo/*
* service: ${["user-backend"].id}
* priority: 2
* cart-backend:
* type: gcp:compute:BackendService
* properties:
* name: cart-service
* portName: http
* protocol: HTTP
* timeoutSec: 10
* loadBalancingScheme: EXTERNAL_MANAGED
* healthChecks: ${default.id}
* user-backend:
* type: gcp:compute:BackendService
* properties:
* name: user-service
* portName: http
* protocol: HTTP
* timeoutSec: 10
* loadBalancingScheme: EXTERNAL_MANAGED
* healthChecks: ${default.id}
* default:
* type: gcp:compute:HttpHealthCheck
* properties:
* name: health-check
* requestPath: /
* checkIntervalSec: 1
* timeoutSec: 1
* static:
* type: gcp:compute:BackendBucket
* properties:
* name: static-asset-backend-bucket
* bucketName: ${staticBucket.name}
* enableCdn: true
* staticBucket:
* type: gcp:storage:Bucket
* name: static
* properties:
* name: static-asset-bucket
* location: US
* ```
*
* ## Import
* UrlMap can be imported using any of these accepted formats:
* * `projects/{{project}}/global/urlMaps/{{name}}`
* * `{{project}}/{{name}}`
* * `{{name}}`
* When using the `pulumi import` command, UrlMap can be imported using one of the formats above. For example:
* ```sh
* $ pulumi import gcp:compute/uRLMap:URLMap default projects/{{project}}/global/urlMaps/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/uRLMap:URLMap default {{project}}/{{name}}
* ```
* ```sh
* $ pulumi import gcp:compute/uRLMap:URLMap default {{name}}
* ```
* @property defaultRouteAction defaultRouteAction takes effect when none of the hostRules match. The load balancer performs advanced routing actions
* like URL rewrites, header transformations, etc. prior to forwarding the request to the selected backend.
* If defaultRouteAction specifies any weightedBackendServices, defaultService must not be set. Conversely if defaultService
* is set, defaultRouteAction cannot contain any weightedBackendServices.
* Only one of defaultRouteAction or defaultUrlRedirect must be set.
* Structure is documented below.
* @property defaultService The backend service or backend bucket to use when none of the given rules match.
* @property defaultUrlRedirect When none of the specified hostRules match, the request is redirected to a URL specified
* by defaultUrlRedirect. If defaultUrlRedirect is specified, defaultService or
* defaultRouteAction must not be set.
* Structure is documented below.
* @property description An optional description of this resource. Provide this property when you create
* the resource.
* @property headerAction Specifies changes to request and response headers that need to take effect for
* the selected backendService. The headerAction specified here take effect after
* headerAction specified under pathMatcher.
* Structure is documented below.
* @property hostRules The list of HostRules to use against the URL.
* Structure is documented below.
* @property name Name of the resource. Provided by the client when the resource is created. The
* name must be 1-63 characters long, and comply with RFC1035. Specifically, the
* name must be 1-63 characters long and match the regular expression
* `a-z?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit,
* except the last character, which cannot be a dash.
* - - -
* @property pathMatchers The list of named PathMatchers to use against the URL.
* Structure is documented below.
* @property project The ID of the project in which the resource belongs.
* If it is not provided, the provider project is used.
* @property tests The list of expected URL mapping tests. Request to update this UrlMap will
* succeed only if all of the test cases pass. You can specify a maximum of 100
* tests per UrlMap.
* Structure is documented below.
* */*/*/*/*/*/*/*/*/*/*/*/
*/
public data class URLMapArgs(
public val defaultRouteAction: Output? = null,
public val defaultService: Output? = null,
public val defaultUrlRedirect: Output? = null,
public val description: Output? = null,
public val headerAction: Output? = null,
public val hostRules: Output>? = null,
public val name: Output? = null,
public val pathMatchers: Output>? = null,
public val project: Output? = null,
public val tests: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.URLMapArgs =
com.pulumi.gcp.compute.URLMapArgs.builder()
.defaultRouteAction(
defaultRouteAction?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.defaultService(defaultService?.applyValue({ args0 -> args0 }))
.defaultUrlRedirect(
defaultUrlRedirect?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.description(description?.applyValue({ args0 -> args0 }))
.headerAction(headerAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.hostRules(
hostRules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.pathMatchers(
pathMatchers?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.project(project?.applyValue({ args0 -> args0 }))
.tests(
tests?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [URLMapArgs].
*/
@PulumiTagMarker
public class URLMapArgsBuilder internal constructor() {
private var defaultRouteAction: Output? = null
private var defaultService: Output? = null
private var defaultUrlRedirect: Output? = null
private var description: Output? = null
private var headerAction: Output? = null
private var hostRules: Output>? = null
private var name: Output? = null
private var pathMatchers: Output>? = null
private var project: Output? = null
private var tests: Output>? = null
/**
* @param value defaultRouteAction takes effect when none of the hostRules match. The load balancer performs advanced routing actions
* like URL rewrites, header transformations, etc. prior to forwarding the request to the selected backend.
* If defaultRouteAction specifies any weightedBackendServices, defaultService must not be set. Conversely if defaultService
* is set, defaultRouteAction cannot contain any weightedBackendServices.
* Only one of defaultRouteAction or defaultUrlRedirect must be set.
* Structure is documented below.
*/
@JvmName("totqkjnnhngyhicu")
public suspend fun defaultRouteAction(`value`: Output) {
this.defaultRouteAction = value
}
/**
* @param value The backend service or backend bucket to use when none of the given rules match.
*/
@JvmName("yymnlpmqlknimqyr")
public suspend fun defaultService(`value`: Output) {
this.defaultService = value
}
/**
* @param value When none of the specified hostRules match, the request is redirected to a URL specified
* by defaultUrlRedirect. If defaultUrlRedirect is specified, defaultService or
* defaultRouteAction must not be set.
* Structure is documented below.
*/
@JvmName("dguobtkqjqhrinbn")
public suspend fun defaultUrlRedirect(`value`: Output) {
this.defaultUrlRedirect = value
}
/**
* @param value An optional description of this resource. Provide this property when you create
* the resource.
*/
@JvmName("frdabauxjtqjtqkt")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value Specifies changes to request and response headers that need to take effect for
* the selected backendService. The headerAction specified here take effect after
* headerAction specified under pathMatcher.
* Structure is documented below.
*/
@JvmName("tjlmllyixgwarpki")
public suspend fun headerAction(`value`: Output) {
this.headerAction = value
}
/**
* @param value The list of HostRules to use against the URL.
* Structure is documented below.
*/
@JvmName("iefcrrvrcheuwdxn")
public suspend fun hostRules(`value`: Output>) {
this.hostRules = value
}
@JvmName("vosciojedxvachfe")
public suspend fun hostRules(vararg values: Output) {
this.hostRules = Output.all(values.asList())
}
/**
* @param values The list of HostRules to use against the URL.
* Structure is documented below.
*/
@JvmName("bkgjrqwljhqqjkfk")
public suspend fun hostRules(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy