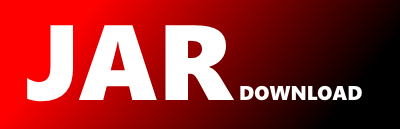
com.pulumi.gcp.compute.kotlin.inputs.BackendServiceBackendArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.BackendServiceBackendArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property balancingMode Specifies the balancing mode for this backend.
* For global HTTP(S) or TCP/SSL load balancing, the default is
* UTILIZATION. Valid values are UTILIZATION, RATE (for HTTP(S))
* and CONNECTION (for TCP/SSL).
* See the [Backend Services Overview](https://cloud.google.com/load-balancing/docs/backend-service#balancing-mode)
* for an explanation of load balancing modes.
* Default value is `UTILIZATION`.
* Possible values are: `UTILIZATION`, `RATE`, `CONNECTION`.
* @property capacityScaler A multiplier applied to the group's maximum servicing capacity
* (based on UTILIZATION, RATE or CONNECTION).
* Default value is 1, which means the group will serve up to 100%
* of its configured capacity (depending on balancingMode). A
* setting of 0 means the group is completely drained, offering
* 0% of its available Capacity. Valid range is [0.0,1.0].
* @property description An optional description of this resource.
* Provide this property when you create the resource.
* @property group The fully-qualified URL of an Instance Group or Network Endpoint
* Group resource. In case of instance group this defines the list
* of instances that serve traffic. Member virtual machine
* instances from each instance group must live in the same zone as
* the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
* For Network Endpoint Groups this defines list of endpoints. All
* endpoints of Network Endpoint Group must be hosted on instances
* located in the same zone as the Network Endpoint Group.
* Backend services cannot mix Instance Group and
* Network Endpoint Group backends.
* Note that you must specify an Instance Group or Network Endpoint
* Group resource using the fully-qualified URL, rather than a
* partial URL.
* @property maxConnections The max number of simultaneous connections for the group. Can
* be used with either CONNECTION or UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or one
* of maxConnectionsPerInstance or maxConnectionsPerEndpoint,
* as appropriate for group type, must be set.
* @property maxConnectionsPerEndpoint The max number of simultaneous connections that a single backend
* network endpoint can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either
* maxConnections or maxConnectionsPerEndpoint must be set.
* @property maxConnectionsPerInstance The max number of simultaneous connections that a single
* backend instance can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or
* maxConnectionsPerInstance must be set.
* @property maxRate The max requests per second (RPS) of the group.
* Can be used with either RATE or UTILIZATION balancing modes,
* but required if RATE mode. For RATE mode, either maxRate or one
* of maxRatePerInstance or maxRatePerEndpoint, as appropriate for
* group type, must be set.
* @property maxRatePerEndpoint The max requests per second (RPS) that a single backend network
* endpoint can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerEndpoint must be set.
* @property maxRatePerInstance The max requests per second (RPS) that a single backend
* instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerInstance must be set.
* @property maxUtilization Used when balancingMode is UTILIZATION. This ratio defines the
* CPU utilization target for the group. Valid range is [0.0, 1.0].
*/
public data class BackendServiceBackendArgs(
public val balancingMode: Output? = null,
public val capacityScaler: Output? = null,
public val description: Output? = null,
public val group: Output,
public val maxConnections: Output? = null,
public val maxConnectionsPerEndpoint: Output? = null,
public val maxConnectionsPerInstance: Output? = null,
public val maxRate: Output? = null,
public val maxRatePerEndpoint: Output? = null,
public val maxRatePerInstance: Output? = null,
public val maxUtilization: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.BackendServiceBackendArgs =
com.pulumi.gcp.compute.inputs.BackendServiceBackendArgs.builder()
.balancingMode(balancingMode?.applyValue({ args0 -> args0 }))
.capacityScaler(capacityScaler?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.group(group.applyValue({ args0 -> args0 }))
.maxConnections(maxConnections?.applyValue({ args0 -> args0 }))
.maxConnectionsPerEndpoint(maxConnectionsPerEndpoint?.applyValue({ args0 -> args0 }))
.maxConnectionsPerInstance(maxConnectionsPerInstance?.applyValue({ args0 -> args0 }))
.maxRate(maxRate?.applyValue({ args0 -> args0 }))
.maxRatePerEndpoint(maxRatePerEndpoint?.applyValue({ args0 -> args0 }))
.maxRatePerInstance(maxRatePerInstance?.applyValue({ args0 -> args0 }))
.maxUtilization(maxUtilization?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendServiceBackendArgs].
*/
@PulumiTagMarker
public class BackendServiceBackendArgsBuilder internal constructor() {
private var balancingMode: Output? = null
private var capacityScaler: Output? = null
private var description: Output? = null
private var group: Output? = null
private var maxConnections: Output? = null
private var maxConnectionsPerEndpoint: Output? = null
private var maxConnectionsPerInstance: Output? = null
private var maxRate: Output? = null
private var maxRatePerEndpoint: Output? = null
private var maxRatePerInstance: Output? = null
private var maxUtilization: Output? = null
/**
* @param value Specifies the balancing mode for this backend.
* For global HTTP(S) or TCP/SSL load balancing, the default is
* UTILIZATION. Valid values are UTILIZATION, RATE (for HTTP(S))
* and CONNECTION (for TCP/SSL).
* See the [Backend Services Overview](https://cloud.google.com/load-balancing/docs/backend-service#balancing-mode)
* for an explanation of load balancing modes.
* Default value is `UTILIZATION`.
* Possible values are: `UTILIZATION`, `RATE`, `CONNECTION`.
*/
@JvmName("qmteoqvictaruirj")
public suspend fun balancingMode(`value`: Output) {
this.balancingMode = value
}
/**
* @param value A multiplier applied to the group's maximum servicing capacity
* (based on UTILIZATION, RATE or CONNECTION).
* Default value is 1, which means the group will serve up to 100%
* of its configured capacity (depending on balancingMode). A
* setting of 0 means the group is completely drained, offering
* 0% of its available Capacity. Valid range is [0.0,1.0].
*/
@JvmName("rjesoahyvdyvrcad")
public suspend fun capacityScaler(`value`: Output) {
this.capacityScaler = value
}
/**
* @param value An optional description of this resource.
* Provide this property when you create the resource.
*/
@JvmName("wcnhdehdpkkpljwn")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value The fully-qualified URL of an Instance Group or Network Endpoint
* Group resource. In case of instance group this defines the list
* of instances that serve traffic. Member virtual machine
* instances from each instance group must live in the same zone as
* the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
* For Network Endpoint Groups this defines list of endpoints. All
* endpoints of Network Endpoint Group must be hosted on instances
* located in the same zone as the Network Endpoint Group.
* Backend services cannot mix Instance Group and
* Network Endpoint Group backends.
* Note that you must specify an Instance Group or Network Endpoint
* Group resource using the fully-qualified URL, rather than a
* partial URL.
*/
@JvmName("emomndqufrtnnnsu")
public suspend fun group(`value`: Output) {
this.group = value
}
/**
* @param value The max number of simultaneous connections for the group. Can
* be used with either CONNECTION or UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or one
* of maxConnectionsPerInstance or maxConnectionsPerEndpoint,
* as appropriate for group type, must be set.
*/
@JvmName("hmqdiylicgvpfrva")
public suspend fun maxConnections(`value`: Output) {
this.maxConnections = value
}
/**
* @param value The max number of simultaneous connections that a single backend
* network endpoint can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either
* maxConnections or maxConnectionsPerEndpoint must be set.
*/
@JvmName("axffsjftubhdycph")
public suspend fun maxConnectionsPerEndpoint(`value`: Output) {
this.maxConnectionsPerEndpoint = value
}
/**
* @param value The max number of simultaneous connections that a single
* backend instance can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or
* maxConnectionsPerInstance must be set.
*/
@JvmName("dojtcctutqfqbedt")
public suspend fun maxConnectionsPerInstance(`value`: Output) {
this.maxConnectionsPerInstance = value
}
/**
* @param value The max requests per second (RPS) of the group.
* Can be used with either RATE or UTILIZATION balancing modes,
* but required if RATE mode. For RATE mode, either maxRate or one
* of maxRatePerInstance or maxRatePerEndpoint, as appropriate for
* group type, must be set.
*/
@JvmName("crtakbmimchqrkny")
public suspend fun maxRate(`value`: Output) {
this.maxRate = value
}
/**
* @param value The max requests per second (RPS) that a single backend network
* endpoint can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerEndpoint must be set.
*/
@JvmName("bjoqcegesiatcljc")
public suspend fun maxRatePerEndpoint(`value`: Output) {
this.maxRatePerEndpoint = value
}
/**
* @param value The max requests per second (RPS) that a single backend
* instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerInstance must be set.
*/
@JvmName("gnmlunlsrgovxkmt")
public suspend fun maxRatePerInstance(`value`: Output) {
this.maxRatePerInstance = value
}
/**
* @param value Used when balancingMode is UTILIZATION. This ratio defines the
* CPU utilization target for the group. Valid range is [0.0, 1.0].
*/
@JvmName("xnvreiykkcbxnqes")
public suspend fun maxUtilization(`value`: Output) {
this.maxUtilization = value
}
/**
* @param value Specifies the balancing mode for this backend.
* For global HTTP(S) or TCP/SSL load balancing, the default is
* UTILIZATION. Valid values are UTILIZATION, RATE (for HTTP(S))
* and CONNECTION (for TCP/SSL).
* See the [Backend Services Overview](https://cloud.google.com/load-balancing/docs/backend-service#balancing-mode)
* for an explanation of load balancing modes.
* Default value is `UTILIZATION`.
* Possible values are: `UTILIZATION`, `RATE`, `CONNECTION`.
*/
@JvmName("qsbjqufdcpwesvds")
public suspend fun balancingMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.balancingMode = mapped
}
/**
* @param value A multiplier applied to the group's maximum servicing capacity
* (based on UTILIZATION, RATE or CONNECTION).
* Default value is 1, which means the group will serve up to 100%
* of its configured capacity (depending on balancingMode). A
* setting of 0 means the group is completely drained, offering
* 0% of its available Capacity. Valid range is [0.0,1.0].
*/
@JvmName("gvtrvwiwmvonwqec")
public suspend fun capacityScaler(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.capacityScaler = mapped
}
/**
* @param value An optional description of this resource.
* Provide this property when you create the resource.
*/
@JvmName("xuwtbbqjximrdpay")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value The fully-qualified URL of an Instance Group or Network Endpoint
* Group resource. In case of instance group this defines the list
* of instances that serve traffic. Member virtual machine
* instances from each instance group must live in the same zone as
* the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
* For Network Endpoint Groups this defines list of endpoints. All
* endpoints of Network Endpoint Group must be hosted on instances
* located in the same zone as the Network Endpoint Group.
* Backend services cannot mix Instance Group and
* Network Endpoint Group backends.
* Note that you must specify an Instance Group or Network Endpoint
* Group resource using the fully-qualified URL, rather than a
* partial URL.
*/
@JvmName("edylwscxetarxqqq")
public suspend fun group(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.group = mapped
}
/**
* @param value The max number of simultaneous connections for the group. Can
* be used with either CONNECTION or UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or one
* of maxConnectionsPerInstance or maxConnectionsPerEndpoint,
* as appropriate for group type, must be set.
*/
@JvmName("rxrxpbdphrxnkdyg")
public suspend fun maxConnections(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConnections = mapped
}
/**
* @param value The max number of simultaneous connections that a single backend
* network endpoint can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either
* maxConnections or maxConnectionsPerEndpoint must be set.
*/
@JvmName("jfdnnabbfijauhfv")
public suspend fun maxConnectionsPerEndpoint(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConnectionsPerEndpoint = mapped
}
/**
* @param value The max number of simultaneous connections that a single
* backend instance can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or
* maxConnectionsPerInstance must be set.
*/
@JvmName("lyieyfraigxvptad")
public suspend fun maxConnectionsPerInstance(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConnectionsPerInstance = mapped
}
/**
* @param value The max requests per second (RPS) of the group.
* Can be used with either RATE or UTILIZATION balancing modes,
* but required if RATE mode. For RATE mode, either maxRate or one
* of maxRatePerInstance or maxRatePerEndpoint, as appropriate for
* group type, must be set.
*/
@JvmName("ibkjtrhwverrleiy")
public suspend fun maxRate(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRate = mapped
}
/**
* @param value The max requests per second (RPS) that a single backend network
* endpoint can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerEndpoint must be set.
*/
@JvmName("aabmxiqnxvgdolfb")
public suspend fun maxRatePerEndpoint(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRatePerEndpoint = mapped
}
/**
* @param value The max requests per second (RPS) that a single backend
* instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerInstance must be set.
*/
@JvmName("fbhwrjsgynjiecdr")
public suspend fun maxRatePerInstance(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRatePerInstance = mapped
}
/**
* @param value Used when balancingMode is UTILIZATION. This ratio defines the
* CPU utilization target for the group. Valid range is [0.0, 1.0].
*/
@JvmName("pgroscoecnebklaw")
public suspend fun maxUtilization(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxUtilization = mapped
}
internal fun build(): BackendServiceBackendArgs = BackendServiceBackendArgs(
balancingMode = balancingMode,
capacityScaler = capacityScaler,
description = description,
group = group ?: throw PulumiNullFieldException("group"),
maxConnections = maxConnections,
maxConnectionsPerEndpoint = maxConnectionsPerEndpoint,
maxConnectionsPerInstance = maxConnectionsPerInstance,
maxRate = maxRate,
maxRatePerEndpoint = maxRatePerEndpoint,
maxRatePerInstance = maxRatePerInstance,
maxUtilization = maxUtilization,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy