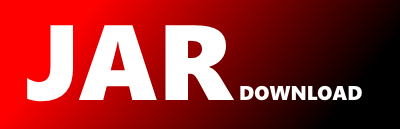
com.pulumi.gcp.compute.kotlin.inputs.BackendServiceCircuitBreakersArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.BackendServiceCircuitBreakersArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property connectTimeout The timeout for new network connections to hosts.
* Structure is documented below.
* @property maxConnections The maximum number of connections to the backend cluster.
* Defaults to 1024.
* @property maxPendingRequests The maximum number of pending requests to the backend cluster.
* Defaults to 1024.
* @property maxRequests The maximum number of parallel requests to the backend cluster.
* Defaults to 1024.
* @property maxRequestsPerConnection Maximum requests for a single backend connection. This parameter
* is respected by both the HTTP/1.1 and HTTP/2 implementations. If
* not specified, there is no limit. Setting this parameter to 1
* will effectively disable keep alive.
* @property maxRetries The maximum number of parallel retries to the backend cluster.
* Defaults to 3.
*/
public data class BackendServiceCircuitBreakersArgs(
public val connectTimeout: Output? = null,
public val maxConnections: Output? = null,
public val maxPendingRequests: Output? = null,
public val maxRequests: Output? = null,
public val maxRequestsPerConnection: Output? = null,
public val maxRetries: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.BackendServiceCircuitBreakersArgs =
com.pulumi.gcp.compute.inputs.BackendServiceCircuitBreakersArgs.builder()
.connectTimeout(connectTimeout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxConnections(maxConnections?.applyValue({ args0 -> args0 }))
.maxPendingRequests(maxPendingRequests?.applyValue({ args0 -> args0 }))
.maxRequests(maxRequests?.applyValue({ args0 -> args0 }))
.maxRequestsPerConnection(maxRequestsPerConnection?.applyValue({ args0 -> args0 }))
.maxRetries(maxRetries?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendServiceCircuitBreakersArgs].
*/
@PulumiTagMarker
public class BackendServiceCircuitBreakersArgsBuilder internal constructor() {
private var connectTimeout: Output? = null
private var maxConnections: Output? = null
private var maxPendingRequests: Output? = null
private var maxRequests: Output? = null
private var maxRequestsPerConnection: Output? = null
private var maxRetries: Output? = null
/**
* @param value The timeout for new network connections to hosts.
* Structure is documented below.
*/
@JvmName("jufqjwulkpeouhum")
public suspend fun connectTimeout(`value`: Output) {
this.connectTimeout = value
}
/**
* @param value The maximum number of connections to the backend cluster.
* Defaults to 1024.
*/
@JvmName("fhqjgdofmpdochmp")
public suspend fun maxConnections(`value`: Output) {
this.maxConnections = value
}
/**
* @param value The maximum number of pending requests to the backend cluster.
* Defaults to 1024.
*/
@JvmName("edbwopidagsnjild")
public suspend fun maxPendingRequests(`value`: Output) {
this.maxPendingRequests = value
}
/**
* @param value The maximum number of parallel requests to the backend cluster.
* Defaults to 1024.
*/
@JvmName("gxwvjdtnkrsjfexn")
public suspend fun maxRequests(`value`: Output) {
this.maxRequests = value
}
/**
* @param value Maximum requests for a single backend connection. This parameter
* is respected by both the HTTP/1.1 and HTTP/2 implementations. If
* not specified, there is no limit. Setting this parameter to 1
* will effectively disable keep alive.
*/
@JvmName("wrcjoouaajmlrkkw")
public suspend fun maxRequestsPerConnection(`value`: Output) {
this.maxRequestsPerConnection = value
}
/**
* @param value The maximum number of parallel retries to the backend cluster.
* Defaults to 3.
*/
@JvmName("hxmfkjcpobxqjwva")
public suspend fun maxRetries(`value`: Output) {
this.maxRetries = value
}
/**
* @param value The timeout for new network connections to hosts.
* Structure is documented below.
*/
@JvmName("ldllqookqafoisjd")
public suspend fun connectTimeout(`value`: BackendServiceCircuitBreakersConnectTimeoutArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectTimeout = mapped
}
/**
* @param argument The timeout for new network connections to hosts.
* Structure is documented below.
*/
@JvmName("nmpswsipldyrvoyh")
public suspend fun connectTimeout(argument: suspend BackendServiceCircuitBreakersConnectTimeoutArgsBuilder.() -> Unit) {
val toBeMapped = BackendServiceCircuitBreakersConnectTimeoutArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.connectTimeout = mapped
}
/**
* @param value The maximum number of connections to the backend cluster.
* Defaults to 1024.
*/
@JvmName("yuvubtqkqvobjmfr")
public suspend fun maxConnections(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxConnections = mapped
}
/**
* @param value The maximum number of pending requests to the backend cluster.
* Defaults to 1024.
*/
@JvmName("sgwfprfegnvwbnji")
public suspend fun maxPendingRequests(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxPendingRequests = mapped
}
/**
* @param value The maximum number of parallel requests to the backend cluster.
* Defaults to 1024.
*/
@JvmName("enialqofyxwpucga")
public suspend fun maxRequests(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRequests = mapped
}
/**
* @param value Maximum requests for a single backend connection. This parameter
* is respected by both the HTTP/1.1 and HTTP/2 implementations. If
* not specified, there is no limit. Setting this parameter to 1
* will effectively disable keep alive.
*/
@JvmName("pjkqyusmjahdsywq")
public suspend fun maxRequestsPerConnection(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRequestsPerConnection = mapped
}
/**
* @param value The maximum number of parallel retries to the backend cluster.
* Defaults to 3.
*/
@JvmName("lfmylqlxwlnvvwnk")
public suspend fun maxRetries(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxRetries = mapped
}
internal fun build(): BackendServiceCircuitBreakersArgs = BackendServiceCircuitBreakersArgs(
connectTimeout = connectTimeout,
maxConnections = maxConnections,
maxPendingRequests = maxPendingRequests,
maxRequests = maxRequests,
maxRequestsPerConnection = maxRequestsPerConnection,
maxRetries = maxRetries,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy