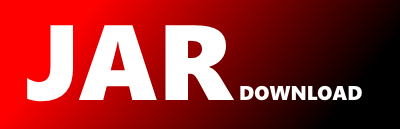
com.pulumi.gcp.compute.kotlin.inputs.BackendServiceLocalityLbPolicyPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.BackendServiceLocalityLbPolicyPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property name The name of a locality load balancer policy to be used. The value
* should be one of the predefined ones as supported by localityLbPolicy,
* although at the moment only ROUND_ROBIN is supported.
* This field should only be populated when the customPolicy field is not
* used.
* Note that specifying the same policy more than once for a backend is
* not a valid configuration and will be rejected.
* The possible values are:
* * `ROUND_ROBIN`: This is a simple policy in which each healthy backend
* is selected in round robin order.
* * `LEAST_REQUEST`: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests.
* * `RING_HASH`: The ring/modulo hash load balancer implements consistent
* hashing to backends. The algorithm has the property that the
* addition/removal of a host from a set of N hosts only affects
* 1/N of the requests.
* * `RANDOM`: The load balancer selects a random healthy host.
* * `ORIGINAL_DESTINATION`: Backend host is selected based on the client
* connection metadata, i.e., connections are opened
* to the same address as the destination address of
* the incoming connection before the connection
* was redirected to the load balancer.
* * `MAGLEV`: used as a drop in replacement for the ring hash load balancer.
* Maglev is not as stable as ring hash but has faster table lookup
* build times and host selection times. For more information about
* Maglev, refer to https://ai.google/research/pubs/pub44824
* Possible values are: `ROUND_ROBIN`, `LEAST_REQUEST`, `RING_HASH`, `RANDOM`, `ORIGINAL_DESTINATION`, `MAGLEV`.
*/
public data class BackendServiceLocalityLbPolicyPolicyArgs(
public val name: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.BackendServiceLocalityLbPolicyPolicyArgs =
com.pulumi.gcp.compute.inputs.BackendServiceLocalityLbPolicyPolicyArgs.builder()
.name(name.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BackendServiceLocalityLbPolicyPolicyArgs].
*/
@PulumiTagMarker
public class BackendServiceLocalityLbPolicyPolicyArgsBuilder internal constructor() {
private var name: Output? = null
/**
* @param value The name of a locality load balancer policy to be used. The value
* should be one of the predefined ones as supported by localityLbPolicy,
* although at the moment only ROUND_ROBIN is supported.
* This field should only be populated when the customPolicy field is not
* used.
* Note that specifying the same policy more than once for a backend is
* not a valid configuration and will be rejected.
* The possible values are:
* * `ROUND_ROBIN`: This is a simple policy in which each healthy backend
* is selected in round robin order.
* * `LEAST_REQUEST`: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests.
* * `RING_HASH`: The ring/modulo hash load balancer implements consistent
* hashing to backends. The algorithm has the property that the
* addition/removal of a host from a set of N hosts only affects
* 1/N of the requests.
* * `RANDOM`: The load balancer selects a random healthy host.
* * `ORIGINAL_DESTINATION`: Backend host is selected based on the client
* connection metadata, i.e., connections are opened
* to the same address as the destination address of
* the incoming connection before the connection
* was redirected to the load balancer.
* * `MAGLEV`: used as a drop in replacement for the ring hash load balancer.
* Maglev is not as stable as ring hash but has faster table lookup
* build times and host selection times. For more information about
* Maglev, refer to https://ai.google/research/pubs/pub44824
* Possible values are: `ROUND_ROBIN`, `LEAST_REQUEST`, `RING_HASH`, `RANDOM`, `ORIGINAL_DESTINATION`, `MAGLEV`.
*/
@JvmName("asjihqctyvbkcbin")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The name of a locality load balancer policy to be used. The value
* should be one of the predefined ones as supported by localityLbPolicy,
* although at the moment only ROUND_ROBIN is supported.
* This field should only be populated when the customPolicy field is not
* used.
* Note that specifying the same policy more than once for a backend is
* not a valid configuration and will be rejected.
* The possible values are:
* * `ROUND_ROBIN`: This is a simple policy in which each healthy backend
* is selected in round robin order.
* * `LEAST_REQUEST`: An O(1) algorithm which selects two random healthy
* hosts and picks the host which has fewer active requests.
* * `RING_HASH`: The ring/modulo hash load balancer implements consistent
* hashing to backends. The algorithm has the property that the
* addition/removal of a host from a set of N hosts only affects
* 1/N of the requests.
* * `RANDOM`: The load balancer selects a random healthy host.
* * `ORIGINAL_DESTINATION`: Backend host is selected based on the client
* connection metadata, i.e., connections are opened
* to the same address as the destination address of
* the incoming connection before the connection
* was redirected to the load balancer.
* * `MAGLEV`: used as a drop in replacement for the ring hash load balancer.
* Maglev is not as stable as ring hash but has faster table lookup
* build times and host selection times. For more information about
* Maglev, refer to https://ai.google/research/pubs/pub44824
* Possible values are: `ROUND_ROBIN`, `LEAST_REQUEST`, `RING_HASH`, `RANDOM`, `ORIGINAL_DESTINATION`, `MAGLEV`.
*/
@JvmName("dyekducxvelhnfjm")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
internal fun build(): BackendServiceLocalityLbPolicyPolicyArgs =
BackendServiceLocalityLbPolicyPolicyArgs(
name = name ?: throw PulumiNullFieldException("name"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy