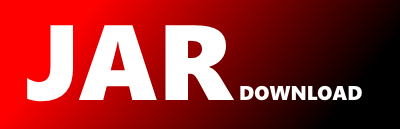
com.pulumi.gcp.compute.kotlin.inputs.GetAddressesPlainArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.gcp.compute.inputs.GetAddressesPlainArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* A collection of arguments for invoking getAddresses.
* @property filter A filter expression that
* filters resources listed in the response. The expression must specify
* the field name, an operator, and the value that you want to use for
* filtering. The value must be a string, a number, or a boolean. The
* operator must be either "=", "!=", ">", "<", "<=", ">=" or ":". For
* example, if you are filtering Compute Engine instances, you can
* exclude instances named "example-instance" by specifying "name !=
* example-instance". The ":" operator can be used with string fields to
* match substrings. For non-string fields it is equivalent to the "="
* operator. The ":*" comparison can be used to test whether a key has
* been defined. For example, to find all objects with "owner" label
* use: """ labels.owner:* """ You can also filter nested fields. For
* example, you could specify "scheduling.automaticRestart = false" to
* include instances only if they are not scheduled for automatic
* restarts. You can use filtering on nested fields to filter based on
* resource labels. To filter on multiple expressions, provide each
* separate expression within parentheses. For example: """
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake")
* """ By default, each expression is an "AND" expression. However, you
* can include "AND" and "OR" expressions explicitly. For example: """
* (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel Broadwell")
* AND (scheduling.automaticRestart = true)
* @property project The google project in which addresses are listed.
* Defaults to provider's configuration if missing.
* @property region Region that should be considered to search addresses.
* All regions are considered if missing.
*/
public data class GetAddressesPlainArgs(
public val filter: String? = null,
public val project: String? = null,
public val region: String? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.GetAddressesPlainArgs =
com.pulumi.gcp.compute.inputs.GetAddressesPlainArgs.builder()
.filter(filter?.let({ args0 -> args0 }))
.project(project?.let({ args0 -> args0 }))
.region(region?.let({ args0 -> args0 })).build()
}
/**
* Builder for [GetAddressesPlainArgs].
*/
@PulumiTagMarker
public class GetAddressesPlainArgsBuilder internal constructor() {
private var filter: String? = null
private var project: String? = null
private var region: String? = null
/**
* @param value A filter expression that
* filters resources listed in the response. The expression must specify
* the field name, an operator, and the value that you want to use for
* filtering. The value must be a string, a number, or a boolean. The
* operator must be either "=", "!=", ">", "<", "<=", ">=" or ":". For
* example, if you are filtering Compute Engine instances, you can
* exclude instances named "example-instance" by specifying "name !=
* example-instance". The ":" operator can be used with string fields to
* match substrings. For non-string fields it is equivalent to the "="
* operator. The ":*" comparison can be used to test whether a key has
* been defined. For example, to find all objects with "owner" label
* use: """ labels.owner:* """ You can also filter nested fields. For
* example, you could specify "scheduling.automaticRestart = false" to
* include instances only if they are not scheduled for automatic
* restarts. You can use filtering on nested fields to filter based on
* resource labels. To filter on multiple expressions, provide each
* separate expression within parentheses. For example: """
* (scheduling.automaticRestart = true) (cpuPlatform = "Intel Skylake")
* """ By default, each expression is an "AND" expression. However, you
* can include "AND" and "OR" expressions explicitly. For example: """
* (cpuPlatform = "Intel Skylake") OR (cpuPlatform = "Intel Broadwell")
* AND (scheduling.automaticRestart = true)
*/
@JvmName("dmmqmguumkkvrbfc")
public suspend fun filter(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.filter = mapped
}
/**
* @param value The google project in which addresses are listed.
* Defaults to provider's configuration if missing.
*/
@JvmName("bewiwtonlxnherhn")
public suspend fun project(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.project = mapped
}
/**
* @param value Region that should be considered to search addresses.
* All regions are considered if missing.
*/
@JvmName("xisjljuvglmoqpgn")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> args0 })
this.region = mapped
}
internal fun build(): GetAddressesPlainArgs = GetAddressesPlainArgs(
filter = filter,
project = project,
region = region,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy