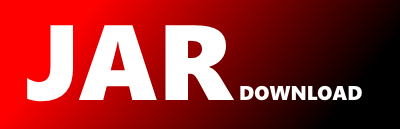
com.pulumi.gcp.compute.kotlin.inputs.InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property externalIpv6 The first IPv6 address of the external IPv6 range associated with this instance, prefix length is stored in externalIpv6PrefixLength in ipv6AccessConfig. To use a static external IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an external IPv6 address from the instance's subnetwork.
* @property externalIpv6PrefixLength The prefix length of the external IPv6 range.
* @property name A unique name for the resource, required by GCE.
* Changing this forces a new resource to be created.
* @property networkTier The service-level to be provided for IPv6 traffic when the subnet has an external subnet. Only PREMIUM tier is valid for IPv6
* @property publicPtrDomainName The domain name to be used when creating DNSv6 records for the external IPv6 ranges.
* @property securityPolicy A full or partial URL to a security policy to add to this instance. If this field is set to an empty string it will remove the associated security policy.
*/
public data class InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs(
public val externalIpv6: Output? = null,
public val externalIpv6PrefixLength: Output? = null,
public val name: Output? = null,
public val networkTier: Output,
public val publicPtrDomainName: Output? = null,
public val securityPolicy: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs =
com.pulumi.gcp.compute.inputs.InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs.builder()
.externalIpv6(externalIpv6?.applyValue({ args0 -> args0 }))
.externalIpv6PrefixLength(externalIpv6PrefixLength?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.networkTier(networkTier.applyValue({ args0 -> args0 }))
.publicPtrDomainName(publicPtrDomainName?.applyValue({ args0 -> args0 }))
.securityPolicy(securityPolicy?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs].
*/
@PulumiTagMarker
public class InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgsBuilder internal constructor() {
private var externalIpv6: Output? = null
private var externalIpv6PrefixLength: Output? = null
private var name: Output? = null
private var networkTier: Output? = null
private var publicPtrDomainName: Output? = null
private var securityPolicy: Output? = null
/**
* @param value The first IPv6 address of the external IPv6 range associated with this instance, prefix length is stored in externalIpv6PrefixLength in ipv6AccessConfig. To use a static external IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an external IPv6 address from the instance's subnetwork.
*/
@JvmName("qufckeffhriiygsx")
public suspend fun externalIpv6(`value`: Output) {
this.externalIpv6 = value
}
/**
* @param value The prefix length of the external IPv6 range.
*/
@JvmName("qcjsbgtcslexekra")
public suspend fun externalIpv6PrefixLength(`value`: Output) {
this.externalIpv6PrefixLength = value
}
/**
* @param value A unique name for the resource, required by GCE.
* Changing this forces a new resource to be created.
*/
@JvmName("miysyrvbjbekdlar")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The service-level to be provided for IPv6 traffic when the subnet has an external subnet. Only PREMIUM tier is valid for IPv6
*/
@JvmName("oufxggowshfrrhnw")
public suspend fun networkTier(`value`: Output) {
this.networkTier = value
}
/**
* @param value The domain name to be used when creating DNSv6 records for the external IPv6 ranges.
*/
@JvmName("nhkauirvoewivgwt")
public suspend fun publicPtrDomainName(`value`: Output) {
this.publicPtrDomainName = value
}
/**
* @param value A full or partial URL to a security policy to add to this instance. If this field is set to an empty string it will remove the associated security policy.
*/
@JvmName("hyfifsabcqxcxaln")
public suspend fun securityPolicy(`value`: Output) {
this.securityPolicy = value
}
/**
* @param value The first IPv6 address of the external IPv6 range associated with this instance, prefix length is stored in externalIpv6PrefixLength in ipv6AccessConfig. To use a static external IP address, it must be unused and in the same region as the instance's zone. If not specified, Google Cloud will automatically assign an external IPv6 address from the instance's subnetwork.
*/
@JvmName("qmsklwysisehthqn")
public suspend fun externalIpv6(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalIpv6 = mapped
}
/**
* @param value The prefix length of the external IPv6 range.
*/
@JvmName("flvjhsqcusjlvmfm")
public suspend fun externalIpv6PrefixLength(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.externalIpv6PrefixLength = mapped
}
/**
* @param value A unique name for the resource, required by GCE.
* Changing this forces a new resource to be created.
*/
@JvmName("imuntfqwhxaiacrf")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The service-level to be provided for IPv6 traffic when the subnet has an external subnet. Only PREMIUM tier is valid for IPv6
*/
@JvmName("flelvpukweitnnhw")
public suspend fun networkTier(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.networkTier = mapped
}
/**
* @param value The domain name to be used when creating DNSv6 records for the external IPv6 ranges.
*/
@JvmName("vvdoiltwfpwhqsqm")
public suspend fun publicPtrDomainName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.publicPtrDomainName = mapped
}
/**
* @param value A full or partial URL to a security policy to add to this instance. If this field is set to an empty string it will remove the associated security policy.
*/
@JvmName("gpimoyjfxdpoxonc")
public suspend fun securityPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityPolicy = mapped
}
internal fun build(): InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs =
InstanceFromMachineImageNetworkInterfaceIpv6AccessConfigArgs(
externalIpv6 = externalIpv6,
externalIpv6PrefixLength = externalIpv6PrefixLength,
name = name,
networkTier = networkTier ?: throw PulumiNullFieldException("networkTier"),
publicPtrDomainName = publicPtrDomainName,
securityPolicy = securityPolicy,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy