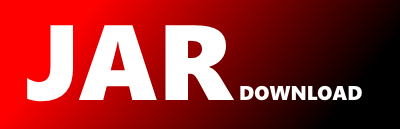
com.pulumi.gcp.compute.kotlin.inputs.InstanceFromMachineImageSchedulingArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.InstanceFromMachineImageSchedulingArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property automaticRestart Specifies if the instance should be restarted if it was terminated by Compute Engine (not a user).
* @property instanceTerminationAction Specifies the action GCE should take when SPOT VM is preempted.
* @property localSsdRecoveryTimeout Specifies the maximum amount of time a Local Ssd Vm should wait while
* recovery of the Local Ssd state is attempted. Its value should be in
* between 0 and 168 hours with hour granularity and the default value being 1
* hour.
* @property maintenanceInterval Specifies the frequency of planned maintenance events. The accepted values are: PERIODIC
* @property maxRunDuration The timeout for new network connections to hosts.
* @property minNodeCpus
* @property nodeAffinities Specifies node affinities or anti-affinities to determine which sole-tenant nodes your instances and managed instance groups will use as host systems.
* @property onHostMaintenance Describes maintenance behavior for the instance. One of MIGRATE or TERMINATE,
* @property preemptible Whether the instance is preemptible.
* @property provisioningModel Whether the instance is spot. If this is set as SPOT.
*/
public data class InstanceFromMachineImageSchedulingArgs(
public val automaticRestart: Output? = null,
public val instanceTerminationAction: Output? = null,
public val localSsdRecoveryTimeout: Output? = null,
public val maintenanceInterval: Output? = null,
public val maxRunDuration: Output? = null,
public val minNodeCpus: Output? = null,
public val nodeAffinities: Output>? =
null,
public val onHostMaintenance: Output? = null,
public val preemptible: Output? = null,
public val provisioningModel: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.InstanceFromMachineImageSchedulingArgs =
com.pulumi.gcp.compute.inputs.InstanceFromMachineImageSchedulingArgs.builder()
.automaticRestart(automaticRestart?.applyValue({ args0 -> args0 }))
.instanceTerminationAction(instanceTerminationAction?.applyValue({ args0 -> args0 }))
.localSsdRecoveryTimeout(
localSsdRecoveryTimeout?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.maintenanceInterval(maintenanceInterval?.applyValue({ args0 -> args0 }))
.maxRunDuration(maxRunDuration?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.minNodeCpus(minNodeCpus?.applyValue({ args0 -> args0 }))
.nodeAffinities(
nodeAffinities?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.onHostMaintenance(onHostMaintenance?.applyValue({ args0 -> args0 }))
.preemptible(preemptible?.applyValue({ args0 -> args0 }))
.provisioningModel(provisioningModel?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceFromMachineImageSchedulingArgs].
*/
@PulumiTagMarker
public class InstanceFromMachineImageSchedulingArgsBuilder internal constructor() {
private var automaticRestart: Output? = null
private var instanceTerminationAction: Output? = null
private var localSsdRecoveryTimeout:
Output? = null
private var maintenanceInterval: Output? = null
private var maxRunDuration: Output? = null
private var minNodeCpus: Output? = null
private var nodeAffinities: Output>? =
null
private var onHostMaintenance: Output? = null
private var preemptible: Output? = null
private var provisioningModel: Output? = null
/**
* @param value Specifies if the instance should be restarted if it was terminated by Compute Engine (not a user).
*/
@JvmName("ebmjvcxuxprvcyyu")
public suspend fun automaticRestart(`value`: Output) {
this.automaticRestart = value
}
/**
* @param value Specifies the action GCE should take when SPOT VM is preempted.
*/
@JvmName("kpbrxdvcktscctjl")
public suspend fun instanceTerminationAction(`value`: Output) {
this.instanceTerminationAction = value
}
/**
* @param value Specifies the maximum amount of time a Local Ssd Vm should wait while
* recovery of the Local Ssd state is attempted. Its value should be in
* between 0 and 168 hours with hour granularity and the default value being 1
* hour.
*/
@JvmName("imworwxfrvtimbbl")
public suspend fun localSsdRecoveryTimeout(`value`: Output) {
this.localSsdRecoveryTimeout = value
}
/**
* @param value Specifies the frequency of planned maintenance events. The accepted values are: PERIODIC
*/
@JvmName("fokelsfygvlklgjm")
public suspend fun maintenanceInterval(`value`: Output) {
this.maintenanceInterval = value
}
/**
* @param value The timeout for new network connections to hosts.
*/
@JvmName("eidteniypmdhnqdp")
public suspend fun maxRunDuration(`value`: Output) {
this.maxRunDuration = value
}
/**
* @param value
*/
@JvmName("edashrkolvsdeuji")
public suspend fun minNodeCpus(`value`: Output) {
this.minNodeCpus = value
}
/**
* @param value Specifies node affinities or anti-affinities to determine which sole-tenant nodes your instances and managed instance groups will use as host systems.
*/
@JvmName("sgeutgdseobywgox")
public suspend fun nodeAffinities(`value`: Output>) {
this.nodeAffinities = value
}
@JvmName("rfjiajdyyfvrpvjs")
public suspend fun nodeAffinities(vararg values: Output) {
this.nodeAffinities = Output.all(values.asList())
}
/**
* @param values Specifies node affinities or anti-affinities to determine which sole-tenant nodes your instances and managed instance groups will use as host systems.
*/
@JvmName("nensfvhnirmjbdgm")
public suspend fun nodeAffinities(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy