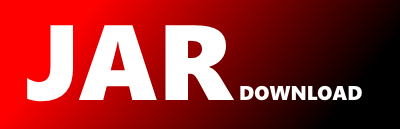
com.pulumi.gcp.compute.kotlin.inputs.InstanceTemplateAdvancedMachineFeaturesArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.InstanceTemplateAdvancedMachineFeaturesArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property enableNestedVirtualization Defines whether the instance should have nested virtualization enabled. Defaults to false.
* @property threadsPerCore The number of threads per physical core. To disable [simultaneous multithreading (SMT)](https://cloud.google.com/compute/docs/instances/disabling-smt) set this to 1.
* @property visibleCoreCount The number of physical cores to expose to an instance. [visible cores info (VC)](https://cloud.google.com/compute/docs/instances/customize-visible-cores).
*/
public data class InstanceTemplateAdvancedMachineFeaturesArgs(
public val enableNestedVirtualization: Output? = null,
public val threadsPerCore: Output? = null,
public val visibleCoreCount: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.InstanceTemplateAdvancedMachineFeaturesArgs =
com.pulumi.gcp.compute.inputs.InstanceTemplateAdvancedMachineFeaturesArgs.builder()
.enableNestedVirtualization(enableNestedVirtualization?.applyValue({ args0 -> args0 }))
.threadsPerCore(threadsPerCore?.applyValue({ args0 -> args0 }))
.visibleCoreCount(visibleCoreCount?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [InstanceTemplateAdvancedMachineFeaturesArgs].
*/
@PulumiTagMarker
public class InstanceTemplateAdvancedMachineFeaturesArgsBuilder internal constructor() {
private var enableNestedVirtualization: Output? = null
private var threadsPerCore: Output? = null
private var visibleCoreCount: Output? = null
/**
* @param value Defines whether the instance should have nested virtualization enabled. Defaults to false.
*/
@JvmName("csrfkukyfwottlyf")
public suspend fun enableNestedVirtualization(`value`: Output) {
this.enableNestedVirtualization = value
}
/**
* @param value The number of threads per physical core. To disable [simultaneous multithreading (SMT)](https://cloud.google.com/compute/docs/instances/disabling-smt) set this to 1.
*/
@JvmName("mrgwrgcljixcwcbc")
public suspend fun threadsPerCore(`value`: Output) {
this.threadsPerCore = value
}
/**
* @param value The number of physical cores to expose to an instance. [visible cores info (VC)](https://cloud.google.com/compute/docs/instances/customize-visible-cores).
*/
@JvmName("krruprrjucduxodm")
public suspend fun visibleCoreCount(`value`: Output) {
this.visibleCoreCount = value
}
/**
* @param value Defines whether the instance should have nested virtualization enabled. Defaults to false.
*/
@JvmName("fmeouuplalraenpt")
public suspend fun enableNestedVirtualization(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableNestedVirtualization = mapped
}
/**
* @param value The number of threads per physical core. To disable [simultaneous multithreading (SMT)](https://cloud.google.com/compute/docs/instances/disabling-smt) set this to 1.
*/
@JvmName("xxonebxhtesvsqiq")
public suspend fun threadsPerCore(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.threadsPerCore = mapped
}
/**
* @param value The number of physical cores to expose to an instance. [visible cores info (VC)](https://cloud.google.com/compute/docs/instances/customize-visible-cores).
*/
@JvmName("kvyosxopyorgkdrl")
public suspend fun visibleCoreCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.visibleCoreCount = mapped
}
internal fun build(): InstanceTemplateAdvancedMachineFeaturesArgs =
InstanceTemplateAdvancedMachineFeaturesArgs(
enableNestedVirtualization = enableNestedVirtualization,
threadsPerCore = threadsPerCore,
visibleCoreCount = visibleCoreCount,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy