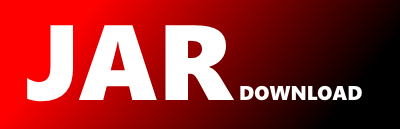
com.pulumi.gcp.compute.kotlin.inputs.RegionBackendServiceConnectionTrackingPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionBackendServiceConnectionTrackingPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property connectionPersistenceOnUnhealthyBackends Specifies connection persistence when backends are unhealthy.
* If set to `DEFAULT_FOR_PROTOCOL`, the existing connections persist on
* unhealthy backends only for connection-oriented protocols (TCP and SCTP)
* and only if the Tracking Mode is PER_CONNECTION (default tracking mode)
* or the Session Affinity is configured for 5-tuple. They do not persist
* for UDP.
* If set to `NEVER_PERSIST`, after a backend becomes unhealthy, the existing
* connections on the unhealthy backend are never persisted on the unhealthy
* backend. They are always diverted to newly selected healthy backends
* (unless all backends are unhealthy).
* If set to `ALWAYS_PERSIST`, existing connections always persist on
* unhealthy backends regardless of protocol and session affinity. It is
* generally not recommended to use this mode overriding the default.
* Default value is `DEFAULT_FOR_PROTOCOL`.
* Possible values are: `DEFAULT_FOR_PROTOCOL`, `NEVER_PERSIST`, `ALWAYS_PERSIST`.
* @property enableStrongAffinity Enable Strong Session Affinity for Network Load Balancing. This option is not available publicly.
* @property idleTimeoutSec Specifies how long to keep a Connection Tracking entry while there is
* no matching traffic (in seconds).
* For L4 ILB the minimum(default) is 10 minutes and maximum is 16 hours.
* For NLB the minimum(default) is 60 seconds and the maximum is 16 hours.
* @property trackingMode Specifies the key used for connection tracking. There are two options:
* `PER_CONNECTION`: The Connection Tracking is performed as per the
* Connection Key (default Hash Method) for the specific protocol.
* `PER_SESSION`: The Connection Tracking is performed as per the
* configured Session Affinity. It matches the configured Session Affinity.
* Default value is `PER_CONNECTION`.
* Possible values are: `PER_CONNECTION`, `PER_SESSION`.
*/
public data class RegionBackendServiceConnectionTrackingPolicyArgs(
public val connectionPersistenceOnUnhealthyBackends: Output? = null,
public val enableStrongAffinity: Output? = null,
public val idleTimeoutSec: Output? = null,
public val trackingMode: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionBackendServiceConnectionTrackingPolicyArgs =
com.pulumi.gcp.compute.inputs.RegionBackendServiceConnectionTrackingPolicyArgs.builder()
.connectionPersistenceOnUnhealthyBackends(
connectionPersistenceOnUnhealthyBackends?.applyValue({ args0 ->
args0
}),
)
.enableStrongAffinity(enableStrongAffinity?.applyValue({ args0 -> args0 }))
.idleTimeoutSec(idleTimeoutSec?.applyValue({ args0 -> args0 }))
.trackingMode(trackingMode?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionBackendServiceConnectionTrackingPolicyArgs].
*/
@PulumiTagMarker
public class RegionBackendServiceConnectionTrackingPolicyArgsBuilder internal constructor() {
private var connectionPersistenceOnUnhealthyBackends: Output? = null
private var enableStrongAffinity: Output? = null
private var idleTimeoutSec: Output? = null
private var trackingMode: Output? = null
/**
* @param value Specifies connection persistence when backends are unhealthy.
* If set to `DEFAULT_FOR_PROTOCOL`, the existing connections persist on
* unhealthy backends only for connection-oriented protocols (TCP and SCTP)
* and only if the Tracking Mode is PER_CONNECTION (default tracking mode)
* or the Session Affinity is configured for 5-tuple. They do not persist
* for UDP.
* If set to `NEVER_PERSIST`, after a backend becomes unhealthy, the existing
* connections on the unhealthy backend are never persisted on the unhealthy
* backend. They are always diverted to newly selected healthy backends
* (unless all backends are unhealthy).
* If set to `ALWAYS_PERSIST`, existing connections always persist on
* unhealthy backends regardless of protocol and session affinity. It is
* generally not recommended to use this mode overriding the default.
* Default value is `DEFAULT_FOR_PROTOCOL`.
* Possible values are: `DEFAULT_FOR_PROTOCOL`, `NEVER_PERSIST`, `ALWAYS_PERSIST`.
*/
@JvmName("bicjshlovyavemjk")
public suspend fun connectionPersistenceOnUnhealthyBackends(`value`: Output) {
this.connectionPersistenceOnUnhealthyBackends = value
}
/**
* @param value Enable Strong Session Affinity for Network Load Balancing. This option is not available publicly.
*/
@JvmName("dteslelqxxaoqodk")
public suspend fun enableStrongAffinity(`value`: Output) {
this.enableStrongAffinity = value
}
/**
* @param value Specifies how long to keep a Connection Tracking entry while there is
* no matching traffic (in seconds).
* For L4 ILB the minimum(default) is 10 minutes and maximum is 16 hours.
* For NLB the minimum(default) is 60 seconds and the maximum is 16 hours.
*/
@JvmName("qcquqdrysugbowvy")
public suspend fun idleTimeoutSec(`value`: Output) {
this.idleTimeoutSec = value
}
/**
* @param value Specifies the key used for connection tracking. There are two options:
* `PER_CONNECTION`: The Connection Tracking is performed as per the
* Connection Key (default Hash Method) for the specific protocol.
* `PER_SESSION`: The Connection Tracking is performed as per the
* configured Session Affinity. It matches the configured Session Affinity.
* Default value is `PER_CONNECTION`.
* Possible values are: `PER_CONNECTION`, `PER_SESSION`.
*/
@JvmName("bejclcosykmoxgwp")
public suspend fun trackingMode(`value`: Output) {
this.trackingMode = value
}
/**
* @param value Specifies connection persistence when backends are unhealthy.
* If set to `DEFAULT_FOR_PROTOCOL`, the existing connections persist on
* unhealthy backends only for connection-oriented protocols (TCP and SCTP)
* and only if the Tracking Mode is PER_CONNECTION (default tracking mode)
* or the Session Affinity is configured for 5-tuple. They do not persist
* for UDP.
* If set to `NEVER_PERSIST`, after a backend becomes unhealthy, the existing
* connections on the unhealthy backend are never persisted on the unhealthy
* backend. They are always diverted to newly selected healthy backends
* (unless all backends are unhealthy).
* If set to `ALWAYS_PERSIST`, existing connections always persist on
* unhealthy backends regardless of protocol and session affinity. It is
* generally not recommended to use this mode overriding the default.
* Default value is `DEFAULT_FOR_PROTOCOL`.
* Possible values are: `DEFAULT_FOR_PROTOCOL`, `NEVER_PERSIST`, `ALWAYS_PERSIST`.
*/
@JvmName("nugsrtpnwrvujqmw")
public suspend fun connectionPersistenceOnUnhealthyBackends(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.connectionPersistenceOnUnhealthyBackends = mapped
}
/**
* @param value Enable Strong Session Affinity for Network Load Balancing. This option is not available publicly.
*/
@JvmName("pghfxfcqxvsgbiyx")
public suspend fun enableStrongAffinity(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableStrongAffinity = mapped
}
/**
* @param value Specifies how long to keep a Connection Tracking entry while there is
* no matching traffic (in seconds).
* For L4 ILB the minimum(default) is 10 minutes and maximum is 16 hours.
* For NLB the minimum(default) is 60 seconds and the maximum is 16 hours.
*/
@JvmName("rwftieoaasvbbqox")
public suspend fun idleTimeoutSec(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.idleTimeoutSec = mapped
}
/**
* @param value Specifies the key used for connection tracking. There are two options:
* `PER_CONNECTION`: The Connection Tracking is performed as per the
* Connection Key (default Hash Method) for the specific protocol.
* `PER_SESSION`: The Connection Tracking is performed as per the
* configured Session Affinity. It matches the configured Session Affinity.
* Default value is `PER_CONNECTION`.
* Possible values are: `PER_CONNECTION`, `PER_SESSION`.
*/
@JvmName("uxvpsqvkdwomfjgr")
public suspend fun trackingMode(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.trackingMode = mapped
}
internal fun build(): RegionBackendServiceConnectionTrackingPolicyArgs =
RegionBackendServiceConnectionTrackingPolicyArgs(
connectionPersistenceOnUnhealthyBackends = connectionPersistenceOnUnhealthyBackends,
enableStrongAffinity = enableStrongAffinity,
idleTimeoutSec = idleTimeoutSec,
trackingMode = trackingMode,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy