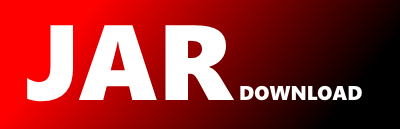
com.pulumi.gcp.compute.kotlin.inputs.RegionBackendServiceOutlierDetectionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionBackendServiceOutlierDetectionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property baseEjectionTime The base time that a host is ejected for. The real time is equal to the base
* time multiplied by the number of times the host has been ejected. Defaults to
* 30000ms or 30s.
* Structure is documented below.
* @property consecutiveErrors Number of errors before a host is ejected from the connection pool. When the
* backend host is accessed over HTTP, a 5xx return code qualifies as an error.
* Defaults to 5.
* @property consecutiveGatewayFailure The number of consecutive gateway failures (502, 503, 504 status or connection
* errors that are mapped to one of those status codes) before a consecutive
* gateway failure ejection occurs. Defaults to 5.
* @property enforcingConsecutiveErrors The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
* @property enforcingConsecutiveGatewayFailure The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
* @property enforcingSuccessRate The percentage chance that a host will be actually ejected when an outlier
* status is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
* @property interval Time interval between ejection sweep analysis. This can result in both new
* ejections as well as hosts being returned to service. Defaults to 10 seconds.
* Structure is documented below.
* @property maxEjectionPercent Maximum percentage of hosts in the load balancing pool for the backend service
* that can be ejected. Defaults to 10%.
* @property successRateMinimumHosts The number of hosts in a cluster that must have enough request volume to detect
* success rate outliers. If the number of hosts is less than this setting, outlier
* detection via success rate statistics is not performed for any host in the
* cluster. Defaults to 5.
* @property successRateRequestVolume The minimum number of total requests that must be collected in one interval (as
* defined by the interval duration above) to include this host in success rate
* based outlier detection. If the volume is lower than this setting, outlier
* detection via success rate statistics is not performed for that host. Defaults
* to 100.
* @property successRateStdevFactor This factor is used to determine the ejection threshold for success rate outlier
* ejection. The ejection threshold is the difference between the mean success
* rate, and the product of this factor and the standard deviation of the mean
* success rate: mean - (stdev * success_rate_stdev_factor). This factor is divided
* by a thousand to get a double. That is, if the desired factor is 1.9, the
* runtime value should be 1900. Defaults to 1900.
*/
public data class RegionBackendServiceOutlierDetectionArgs(
public val baseEjectionTime: Output? =
null,
public val consecutiveErrors: Output? = null,
public val consecutiveGatewayFailure: Output? = null,
public val enforcingConsecutiveErrors: Output? = null,
public val enforcingConsecutiveGatewayFailure: Output? = null,
public val enforcingSuccessRate: Output? = null,
public val interval: Output? = null,
public val maxEjectionPercent: Output? = null,
public val successRateMinimumHosts: Output? = null,
public val successRateRequestVolume: Output? = null,
public val successRateStdevFactor: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionBackendServiceOutlierDetectionArgs =
com.pulumi.gcp.compute.inputs.RegionBackendServiceOutlierDetectionArgs.builder()
.baseEjectionTime(baseEjectionTime?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.consecutiveErrors(consecutiveErrors?.applyValue({ args0 -> args0 }))
.consecutiveGatewayFailure(consecutiveGatewayFailure?.applyValue({ args0 -> args0 }))
.enforcingConsecutiveErrors(enforcingConsecutiveErrors?.applyValue({ args0 -> args0 }))
.enforcingConsecutiveGatewayFailure(
enforcingConsecutiveGatewayFailure?.applyValue({ args0 ->
args0
}),
)
.enforcingSuccessRate(enforcingSuccessRate?.applyValue({ args0 -> args0 }))
.interval(interval?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.maxEjectionPercent(maxEjectionPercent?.applyValue({ args0 -> args0 }))
.successRateMinimumHosts(successRateMinimumHosts?.applyValue({ args0 -> args0 }))
.successRateRequestVolume(successRateRequestVolume?.applyValue({ args0 -> args0 }))
.successRateStdevFactor(successRateStdevFactor?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionBackendServiceOutlierDetectionArgs].
*/
@PulumiTagMarker
public class RegionBackendServiceOutlierDetectionArgsBuilder internal constructor() {
private var baseEjectionTime: Output? =
null
private var consecutiveErrors: Output? = null
private var consecutiveGatewayFailure: Output? = null
private var enforcingConsecutiveErrors: Output? = null
private var enforcingConsecutiveGatewayFailure: Output? = null
private var enforcingSuccessRate: Output? = null
private var interval: Output? = null
private var maxEjectionPercent: Output? = null
private var successRateMinimumHosts: Output? = null
private var successRateRequestVolume: Output? = null
private var successRateStdevFactor: Output? = null
/**
* @param value The base time that a host is ejected for. The real time is equal to the base
* time multiplied by the number of times the host has been ejected. Defaults to
* 30000ms or 30s.
* Structure is documented below.
*/
@JvmName("uwsnnijuyvvtbyqe")
public suspend fun baseEjectionTime(`value`: Output) {
this.baseEjectionTime = value
}
/**
* @param value Number of errors before a host is ejected from the connection pool. When the
* backend host is accessed over HTTP, a 5xx return code qualifies as an error.
* Defaults to 5.
*/
@JvmName("mqkbnggvpbmfegep")
public suspend fun consecutiveErrors(`value`: Output) {
this.consecutiveErrors = value
}
/**
* @param value The number of consecutive gateway failures (502, 503, 504 status or connection
* errors that are mapped to one of those status codes) before a consecutive
* gateway failure ejection occurs. Defaults to 5.
*/
@JvmName("dmtvgwjgsaltcihi")
public suspend fun consecutiveGatewayFailure(`value`: Output) {
this.consecutiveGatewayFailure = value
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*/
@JvmName("bqblvfwkkeotvpwx")
public suspend fun enforcingConsecutiveErrors(`value`: Output) {
this.enforcingConsecutiveErrors = value
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*/
@JvmName("fhfcdckbjtwkbtkg")
public suspend fun enforcingConsecutiveGatewayFailure(`value`: Output) {
this.enforcingConsecutiveGatewayFailure = value
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*/
@JvmName("iitmbrfgwjkgmwhh")
public suspend fun enforcingSuccessRate(`value`: Output) {
this.enforcingSuccessRate = value
}
/**
* @param value Time interval between ejection sweep analysis. This can result in both new
* ejections as well as hosts being returned to service. Defaults to 10 seconds.
* Structure is documented below.
*/
@JvmName("mhhrkutxdeooafil")
public suspend fun interval(`value`: Output) {
this.interval = value
}
/**
* @param value Maximum percentage of hosts in the load balancing pool for the backend service
* that can be ejected. Defaults to 10%.
*/
@JvmName("ghunuwimolqhkwsd")
public suspend fun maxEjectionPercent(`value`: Output) {
this.maxEjectionPercent = value
}
/**
* @param value The number of hosts in a cluster that must have enough request volume to detect
* success rate outliers. If the number of hosts is less than this setting, outlier
* detection via success rate statistics is not performed for any host in the
* cluster. Defaults to 5.
*/
@JvmName("atdedhyvyewocmwq")
public suspend fun successRateMinimumHosts(`value`: Output) {
this.successRateMinimumHosts = value
}
/**
* @param value The minimum number of total requests that must be collected in one interval (as
* defined by the interval duration above) to include this host in success rate
* based outlier detection. If the volume is lower than this setting, outlier
* detection via success rate statistics is not performed for that host. Defaults
* to 100.
*/
@JvmName("dwrmuiqauxpilxfy")
public suspend fun successRateRequestVolume(`value`: Output) {
this.successRateRequestVolume = value
}
/**
* @param value This factor is used to determine the ejection threshold for success rate outlier
* ejection. The ejection threshold is the difference between the mean success
* rate, and the product of this factor and the standard deviation of the mean
* success rate: mean - (stdev * success_rate_stdev_factor). This factor is divided
* by a thousand to get a double. That is, if the desired factor is 1.9, the
* runtime value should be 1900. Defaults to 1900.
*/
@JvmName("ctsqtinabfynyhlh")
public suspend fun successRateStdevFactor(`value`: Output) {
this.successRateStdevFactor = value
}
/**
* @param value The base time that a host is ejected for. The real time is equal to the base
* time multiplied by the number of times the host has been ejected. Defaults to
* 30000ms or 30s.
* Structure is documented below.
*/
@JvmName("jgclnnaljtiajtxy")
public suspend fun baseEjectionTime(`value`: RegionBackendServiceOutlierDetectionBaseEjectionTimeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.baseEjectionTime = mapped
}
/**
* @param argument The base time that a host is ejected for. The real time is equal to the base
* time multiplied by the number of times the host has been ejected. Defaults to
* 30000ms or 30s.
* Structure is documented below.
*/
@JvmName("jxncalotrbcyiihw")
public suspend fun baseEjectionTime(argument: suspend RegionBackendServiceOutlierDetectionBaseEjectionTimeArgsBuilder.() -> Unit) {
val toBeMapped = RegionBackendServiceOutlierDetectionBaseEjectionTimeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.baseEjectionTime = mapped
}
/**
* @param value Number of errors before a host is ejected from the connection pool. When the
* backend host is accessed over HTTP, a 5xx return code qualifies as an error.
* Defaults to 5.
*/
@JvmName("miwonescaokovgge")
public suspend fun consecutiveErrors(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consecutiveErrors = mapped
}
/**
* @param value The number of consecutive gateway failures (502, 503, 504 status or connection
* errors that are mapped to one of those status codes) before a consecutive
* gateway failure ejection occurs. Defaults to 5.
*/
@JvmName("yrcohdkpyubgdpfp")
public suspend fun consecutiveGatewayFailure(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.consecutiveGatewayFailure = mapped
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive 5xx. This setting can be used to disable
* ejection or to ramp it up slowly. Defaults to 100.
*/
@JvmName("kinaeuluhnvdhytu")
public suspend fun enforcingConsecutiveErrors(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enforcingConsecutiveErrors = mapped
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through consecutive gateway failures. This setting can be
* used to disable ejection or to ramp it up slowly. Defaults to 0.
*/
@JvmName("xonwoaiuvltmcbwn")
public suspend fun enforcingConsecutiveGatewayFailure(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enforcingConsecutiveGatewayFailure = mapped
}
/**
* @param value The percentage chance that a host will be actually ejected when an outlier
* status is detected through success rate statistics. This setting can be used to
* disable ejection or to ramp it up slowly. Defaults to 100.
*/
@JvmName("celhniplhtkelkkg")
public suspend fun enforcingSuccessRate(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enforcingSuccessRate = mapped
}
/**
* @param value Time interval between ejection sweep analysis. This can result in both new
* ejections as well as hosts being returned to service. Defaults to 10 seconds.
* Structure is documented below.
*/
@JvmName("mqpgrcsttnmywxim")
public suspend fun interval(`value`: RegionBackendServiceOutlierDetectionIntervalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.interval = mapped
}
/**
* @param argument Time interval between ejection sweep analysis. This can result in both new
* ejections as well as hosts being returned to service. Defaults to 10 seconds.
* Structure is documented below.
*/
@JvmName("ynycgopwtsllxxuq")
public suspend fun interval(argument: suspend RegionBackendServiceOutlierDetectionIntervalArgsBuilder.() -> Unit) {
val toBeMapped = RegionBackendServiceOutlierDetectionIntervalArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.interval = mapped
}
/**
* @param value Maximum percentage of hosts in the load balancing pool for the backend service
* that can be ejected. Defaults to 10%.
*/
@JvmName("eymymyetyonwjtph")
public suspend fun maxEjectionPercent(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxEjectionPercent = mapped
}
/**
* @param value The number of hosts in a cluster that must have enough request volume to detect
* success rate outliers. If the number of hosts is less than this setting, outlier
* detection via success rate statistics is not performed for any host in the
* cluster. Defaults to 5.
*/
@JvmName("rvxnrawpuyyfgpel")
public suspend fun successRateMinimumHosts(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successRateMinimumHosts = mapped
}
/**
* @param value The minimum number of total requests that must be collected in one interval (as
* defined by the interval duration above) to include this host in success rate
* based outlier detection. If the volume is lower than this setting, outlier
* detection via success rate statistics is not performed for that host. Defaults
* to 100.
*/
@JvmName("bcplkxlqmeopopvp")
public suspend fun successRateRequestVolume(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successRateRequestVolume = mapped
}
/**
* @param value This factor is used to determine the ejection threshold for success rate outlier
* ejection. The ejection threshold is the difference between the mean success
* rate, and the product of this factor and the standard deviation of the mean
* success rate: mean - (stdev * success_rate_stdev_factor). This factor is divided
* by a thousand to get a double. That is, if the desired factor is 1.9, the
* runtime value should be 1900. Defaults to 1900.
*/
@JvmName("dkcricotutqlspnn")
public suspend fun successRateStdevFactor(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.successRateStdevFactor = mapped
}
internal fun build(): RegionBackendServiceOutlierDetectionArgs =
RegionBackendServiceOutlierDetectionArgs(
baseEjectionTime = baseEjectionTime,
consecutiveErrors = consecutiveErrors,
consecutiveGatewayFailure = consecutiveGatewayFailure,
enforcingConsecutiveErrors = enforcingConsecutiveErrors,
enforcingConsecutiveGatewayFailure = enforcingConsecutiveGatewayFailure,
enforcingSuccessRate = enforcingSuccessRate,
interval = interval,
maxEjectionPercent = maxEjectionPercent,
successRateMinimumHosts = successRateMinimumHosts,
successRateRequestVolume = successRateRequestVolume,
successRateStdevFactor = successRateStdevFactor,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy