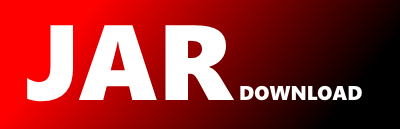
com.pulumi.gcp.compute.kotlin.inputs.RegionCommitmentResourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionCommitmentResourceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property acceleratorType Name of the accelerator type resource. Applicable only when the type is ACCELERATOR.
* @property amount The amount of the resource purchased (in a type-dependent unit,
* such as bytes). For vCPUs, this can just be an integer. For memory,
* this must be provided in MB. Memory must be a multiple of 256 MB,
* with up to 6.5GB of memory per every vCPU.
* @property type Type of resource for which this commitment applies.
* Possible values are VCPU, MEMORY, LOCAL_SSD, and ACCELERATOR.
*/
public data class RegionCommitmentResourceArgs(
public val acceleratorType: Output? = null,
public val amount: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionCommitmentResourceArgs =
com.pulumi.gcp.compute.inputs.RegionCommitmentResourceArgs.builder()
.acceleratorType(acceleratorType?.applyValue({ args0 -> args0 }))
.amount(amount?.applyValue({ args0 -> args0 }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionCommitmentResourceArgs].
*/
@PulumiTagMarker
public class RegionCommitmentResourceArgsBuilder internal constructor() {
private var acceleratorType: Output? = null
private var amount: Output? = null
private var type: Output? = null
/**
* @param value Name of the accelerator type resource. Applicable only when the type is ACCELERATOR.
*/
@JvmName("humndicrgofjcgcq")
public suspend fun acceleratorType(`value`: Output) {
this.acceleratorType = value
}
/**
* @param value The amount of the resource purchased (in a type-dependent unit,
* such as bytes). For vCPUs, this can just be an integer. For memory,
* this must be provided in MB. Memory must be a multiple of 256 MB,
* with up to 6.5GB of memory per every vCPU.
*/
@JvmName("gmlhxkrewixxbbbd")
public suspend fun amount(`value`: Output) {
this.amount = value
}
/**
* @param value Type of resource for which this commitment applies.
* Possible values are VCPU, MEMORY, LOCAL_SSD, and ACCELERATOR.
*/
@JvmName("hrbdoatlgvajykrg")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value Name of the accelerator type resource. Applicable only when the type is ACCELERATOR.
*/
@JvmName("lbuvdmlxtjurcipv")
public suspend fun acceleratorType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.acceleratorType = mapped
}
/**
* @param value The amount of the resource purchased (in a type-dependent unit,
* such as bytes). For vCPUs, this can just be an integer. For memory,
* this must be provided in MB. Memory must be a multiple of 256 MB,
* with up to 6.5GB of memory per every vCPU.
*/
@JvmName("eqiayfimjmqnotnj")
public suspend fun amount(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.amount = mapped
}
/**
* @param value Type of resource for which this commitment applies.
* Possible values are VCPU, MEMORY, LOCAL_SSD, and ACCELERATOR.
*/
@JvmName("gajfllicnxoiichl")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): RegionCommitmentResourceArgs = RegionCommitmentResourceArgs(
acceleratorType = acceleratorType,
amount = amount,
type = type,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy