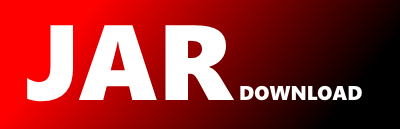
com.pulumi.gcp.compute.kotlin.inputs.RegionHealthCheckGrpcHealthCheckArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionHealthCheckGrpcHealthCheckArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property grpcServiceName The gRPC service name for the health check.
* The value of grpcServiceName has the following meanings by convention:
* * Empty serviceName means the overall status of all services at the backend.
* * Non-empty serviceName means the health of that gRPC service, as defined by the owner of the service.
* The grpcServiceName can only be ASCII.
* @property port The port number for the health check request.
* Must be specified if portName and portSpecification are not set
* or if port_specification is USE_FIXED_PORT. Valid values are 1 through 65535.
* @property portName Port name as defined in InstanceGroup#NamedPort#name. If both port and
* port_name are defined, port takes precedence.
* @property portSpecification Specifies how port is selected for health checking, can be one of the
* following values:
* * `USE_FIXED_PORT`: The port number in `port` is used for health checking.
* * `USE_NAMED_PORT`: The `portName` is used for health checking.
* * `USE_SERVING_PORT`: For NetworkEndpointGroup, the port specified for each
* network endpoint is used for health checking. For other backends, the
* port or named port specified in the Backend Service is used for health
* checking.
* If not specified, gRPC health check follows behavior specified in `port` and
* `portName` fields.
* Possible values are: `USE_FIXED_PORT`, `USE_NAMED_PORT`, `USE_SERVING_PORT`.
*/
public data class RegionHealthCheckGrpcHealthCheckArgs(
public val grpcServiceName: Output? = null,
public val port: Output? = null,
public val portName: Output? = null,
public val portSpecification: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionHealthCheckGrpcHealthCheckArgs =
com.pulumi.gcp.compute.inputs.RegionHealthCheckGrpcHealthCheckArgs.builder()
.grpcServiceName(grpcServiceName?.applyValue({ args0 -> args0 }))
.port(port?.applyValue({ args0 -> args0 }))
.portName(portName?.applyValue({ args0 -> args0 }))
.portSpecification(portSpecification?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionHealthCheckGrpcHealthCheckArgs].
*/
@PulumiTagMarker
public class RegionHealthCheckGrpcHealthCheckArgsBuilder internal constructor() {
private var grpcServiceName: Output? = null
private var port: Output? = null
private var portName: Output? = null
private var portSpecification: Output? = null
/**
* @param value The gRPC service name for the health check.
* The value of grpcServiceName has the following meanings by convention:
* * Empty serviceName means the overall status of all services at the backend.
* * Non-empty serviceName means the health of that gRPC service, as defined by the owner of the service.
* The grpcServiceName can only be ASCII.
*/
@JvmName("hrwrfwoggvijqhwt")
public suspend fun grpcServiceName(`value`: Output) {
this.grpcServiceName = value
}
/**
* @param value The port number for the health check request.
* Must be specified if portName and portSpecification are not set
* or if port_specification is USE_FIXED_PORT. Valid values are 1 through 65535.
*/
@JvmName("lotwuyqmvykxxlnp")
public suspend fun port(`value`: Output) {
this.port = value
}
/**
* @param value Port name as defined in InstanceGroup#NamedPort#name. If both port and
* port_name are defined, port takes precedence.
*/
@JvmName("bhxdqxsekbmnwfyr")
public suspend fun portName(`value`: Output) {
this.portName = value
}
/**
* @param value Specifies how port is selected for health checking, can be one of the
* following values:
* * `USE_FIXED_PORT`: The port number in `port` is used for health checking.
* * `USE_NAMED_PORT`: The `portName` is used for health checking.
* * `USE_SERVING_PORT`: For NetworkEndpointGroup, the port specified for each
* network endpoint is used for health checking. For other backends, the
* port or named port specified in the Backend Service is used for health
* checking.
* If not specified, gRPC health check follows behavior specified in `port` and
* `portName` fields.
* Possible values are: `USE_FIXED_PORT`, `USE_NAMED_PORT`, `USE_SERVING_PORT`.
*/
@JvmName("npjkkoxupcloptlj")
public suspend fun portSpecification(`value`: Output) {
this.portSpecification = value
}
/**
* @param value The gRPC service name for the health check.
* The value of grpcServiceName has the following meanings by convention:
* * Empty serviceName means the overall status of all services at the backend.
* * Non-empty serviceName means the health of that gRPC service, as defined by the owner of the service.
* The grpcServiceName can only be ASCII.
*/
@JvmName("tojgwjryveogqnjw")
public suspend fun grpcServiceName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.grpcServiceName = mapped
}
/**
* @param value The port number for the health check request.
* Must be specified if portName and portSpecification are not set
* or if port_specification is USE_FIXED_PORT. Valid values are 1 through 65535.
*/
@JvmName("lgsdihppmerorxdt")
public suspend fun port(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.port = mapped
}
/**
* @param value Port name as defined in InstanceGroup#NamedPort#name. If both port and
* port_name are defined, port takes precedence.
*/
@JvmName("mhlorvugkyhpqulg")
public suspend fun portName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.portName = mapped
}
/**
* @param value Specifies how port is selected for health checking, can be one of the
* following values:
* * `USE_FIXED_PORT`: The port number in `port` is used for health checking.
* * `USE_NAMED_PORT`: The `portName` is used for health checking.
* * `USE_SERVING_PORT`: For NetworkEndpointGroup, the port specified for each
* network endpoint is used for health checking. For other backends, the
* port or named port specified in the Backend Service is used for health
* checking.
* If not specified, gRPC health check follows behavior specified in `port` and
* `portName` fields.
* Possible values are: `USE_FIXED_PORT`, `USE_NAMED_PORT`, `USE_SERVING_PORT`.
*/
@JvmName("xfievtmhmvyjstmp")
public suspend fun portSpecification(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.portSpecification = mapped
}
internal fun build(): RegionHealthCheckGrpcHealthCheckArgs = RegionHealthCheckGrpcHealthCheckArgs(
grpcServiceName = grpcServiceName,
port = port,
portName = portName,
portSpecification = portSpecification,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy