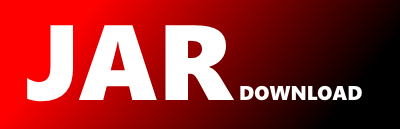
com.pulumi.gcp.compute.kotlin.inputs.RegionInstanceGroupManagerUpdatePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerUpdatePolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property instanceRedistributionType The instance redistribution policy for regional managed instance groups. Valid values are: `"PROACTIVE"`, `"NONE"`. If `PROACTIVE` (default), the group attempts to maintain an even distribution of VM instances across zones in the region. If `NONE`, proactive redistribution is disabled.
* @property maxSurgeFixed , The maximum number of instances that can be created above the specified targetSize during the update process. Conflicts with `max_surge_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
* @property maxSurgePercent , The maximum number of instances(calculated as percentage) that can be created above the specified targetSize during the update process. Conflicts with `max_surge_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
* @property maxUnavailableFixed , The maximum number of instances that can be unavailable during the update process. Conflicts with `max_unavailable_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
* @property maxUnavailablePercent , The maximum number of instances(calculated as percentage) that can be unavailable during the update process. Conflicts with `max_unavailable_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
* @property minReadySec , Minimum number of seconds to wait for after a newly created instance becomes available. This value must be from range [0, 3600]
* @property minimalAction Minimal action to be taken on an instance. You can specify either `REFRESH` to update without stopping instances, `RESTART` to restart existing instances or `REPLACE` to delete and create new instances from the target template. If you specify a `REFRESH`, the Updater will attempt to perform that action only. However, if the Updater determines that the minimal action you specify is not enough to perform the update, it might perform a more disruptive action.
* @property mostDisruptiveAllowedAction Most disruptive action that is allowed to be taken on an instance. You can specify either NONE to forbid any actions, REFRESH to allow actions that do not need instance restart, RESTART to allow actions that can be applied without instance replacing or REPLACE to allow all possible actions. If the Updater determines that the minimal update action needed is more disruptive than most disruptive allowed action you specify it will not perform the update at all.
* @property replacementMethod , The instance replacement method for managed instance groups. Valid values are: "RECREATE", "SUBSTITUTE". If SUBSTITUTE (default), the group replaces VM instances with new instances that have randomly generated names. If RECREATE, instance names are preserved. You must also set max_unavailable_fixed or max_unavailable_percent to be greater than 0.
* - - -
* @property type The type of update process. You can specify either `PROACTIVE` so that the instance group manager proactively executes actions in order to bring instances to their target versions or `OPPORTUNISTIC` so that no action is proactively executed but the update will be performed as part of other actions (for example, resizes or recreateInstances calls).
*/
public data class RegionInstanceGroupManagerUpdatePolicyArgs(
public val instanceRedistributionType: Output? = null,
public val maxSurgeFixed: Output? = null,
public val maxSurgePercent: Output? = null,
public val maxUnavailableFixed: Output? = null,
public val maxUnavailablePercent: Output? = null,
public val minReadySec: Output? = null,
public val minimalAction: Output,
public val mostDisruptiveAllowedAction: Output? = null,
public val replacementMethod: Output? = null,
public val type: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerUpdatePolicyArgs =
com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerUpdatePolicyArgs.builder()
.instanceRedistributionType(instanceRedistributionType?.applyValue({ args0 -> args0 }))
.maxSurgeFixed(maxSurgeFixed?.applyValue({ args0 -> args0 }))
.maxSurgePercent(maxSurgePercent?.applyValue({ args0 -> args0 }))
.maxUnavailableFixed(maxUnavailableFixed?.applyValue({ args0 -> args0 }))
.maxUnavailablePercent(maxUnavailablePercent?.applyValue({ args0 -> args0 }))
.minReadySec(minReadySec?.applyValue({ args0 -> args0 }))
.minimalAction(minimalAction.applyValue({ args0 -> args0 }))
.mostDisruptiveAllowedAction(mostDisruptiveAllowedAction?.applyValue({ args0 -> args0 }))
.replacementMethod(replacementMethod?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionInstanceGroupManagerUpdatePolicyArgs].
*/
@PulumiTagMarker
public class RegionInstanceGroupManagerUpdatePolicyArgsBuilder internal constructor() {
private var instanceRedistributionType: Output? = null
private var maxSurgeFixed: Output? = null
private var maxSurgePercent: Output? = null
private var maxUnavailableFixed: Output? = null
private var maxUnavailablePercent: Output? = null
private var minReadySec: Output? = null
private var minimalAction: Output? = null
private var mostDisruptiveAllowedAction: Output? = null
private var replacementMethod: Output? = null
private var type: Output? = null
/**
* @param value The instance redistribution policy for regional managed instance groups. Valid values are: `"PROACTIVE"`, `"NONE"`. If `PROACTIVE` (default), the group attempts to maintain an even distribution of VM instances across zones in the region. If `NONE`, proactive redistribution is disabled.
*/
@JvmName("cjqeymjwanpbgsqv")
public suspend fun instanceRedistributionType(`value`: Output) {
this.instanceRedistributionType = value
}
/**
* @param value , The maximum number of instances that can be created above the specified targetSize during the update process. Conflicts with `max_surge_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
*/
@JvmName("gtgavycrjwdovqgc")
public suspend fun maxSurgeFixed(`value`: Output) {
this.maxSurgeFixed = value
}
/**
* @param value , The maximum number of instances(calculated as percentage) that can be created above the specified targetSize during the update process. Conflicts with `max_surge_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
*/
@JvmName("fceqleaikseeojto")
public suspend fun maxSurgePercent(`value`: Output) {
this.maxSurgePercent = value
}
/**
* @param value , The maximum number of instances that can be unavailable during the update process. Conflicts with `max_unavailable_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
*/
@JvmName("haevaaytbywgopyo")
public suspend fun maxUnavailableFixed(`value`: Output) {
this.maxUnavailableFixed = value
}
/**
* @param value , The maximum number of instances(calculated as percentage) that can be unavailable during the update process. Conflicts with `max_unavailable_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
*/
@JvmName("dcncujdnewjvpsqe")
public suspend fun maxUnavailablePercent(`value`: Output) {
this.maxUnavailablePercent = value
}
/**
* @param value , Minimum number of seconds to wait for after a newly created instance becomes available. This value must be from range [0, 3600]
*/
@JvmName("hmwbsmskieyivdto")
public suspend fun minReadySec(`value`: Output) {
this.minReadySec = value
}
/**
* @param value Minimal action to be taken on an instance. You can specify either `REFRESH` to update without stopping instances, `RESTART` to restart existing instances or `REPLACE` to delete and create new instances from the target template. If you specify a `REFRESH`, the Updater will attempt to perform that action only. However, if the Updater determines that the minimal action you specify is not enough to perform the update, it might perform a more disruptive action.
*/
@JvmName("iflnaqxvsannusml")
public suspend fun minimalAction(`value`: Output) {
this.minimalAction = value
}
/**
* @param value Most disruptive action that is allowed to be taken on an instance. You can specify either NONE to forbid any actions, REFRESH to allow actions that do not need instance restart, RESTART to allow actions that can be applied without instance replacing or REPLACE to allow all possible actions. If the Updater determines that the minimal update action needed is more disruptive than most disruptive allowed action you specify it will not perform the update at all.
*/
@JvmName("hgvnlhemokujmoms")
public suspend fun mostDisruptiveAllowedAction(`value`: Output) {
this.mostDisruptiveAllowedAction = value
}
/**
* @param value , The instance replacement method for managed instance groups. Valid values are: "RECREATE", "SUBSTITUTE". If SUBSTITUTE (default), the group replaces VM instances with new instances that have randomly generated names. If RECREATE, instance names are preserved. You must also set max_unavailable_fixed or max_unavailable_percent to be greater than 0.
* - - -
*/
@JvmName("oggaonudqkqjmtir")
public suspend fun replacementMethod(`value`: Output) {
this.replacementMethod = value
}
/**
* @param value The type of update process. You can specify either `PROACTIVE` so that the instance group manager proactively executes actions in order to bring instances to their target versions or `OPPORTUNISTIC` so that no action is proactively executed but the update will be performed as part of other actions (for example, resizes or recreateInstances calls).
*/
@JvmName("edjrtvcaswxfbqby")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value The instance redistribution policy for regional managed instance groups. Valid values are: `"PROACTIVE"`, `"NONE"`. If `PROACTIVE` (default), the group attempts to maintain an even distribution of VM instances across zones in the region. If `NONE`, proactive redistribution is disabled.
*/
@JvmName("jwudwupwhqasifjm")
public suspend fun instanceRedistributionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.instanceRedistributionType = mapped
}
/**
* @param value , The maximum number of instances that can be created above the specified targetSize during the update process. Conflicts with `max_surge_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
*/
@JvmName("qdtkvetpjguswlxy")
public suspend fun maxSurgeFixed(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSurgeFixed = mapped
}
/**
* @param value , The maximum number of instances(calculated as percentage) that can be created above the specified targetSize during the update process. Conflicts with `max_surge_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
*/
@JvmName("riiodkfcsimialrl")
public suspend fun maxSurgePercent(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxSurgePercent = mapped
}
/**
* @param value , The maximum number of instances that can be unavailable during the update process. Conflicts with `max_unavailable_percent`. It has to be either 0 or at least equal to the number of zones. If fixed values are used, at least one of `max_unavailable_fixed` or `max_surge_fixed` must be greater than 0.
*/
@JvmName("vtspprudidrecbvy")
public suspend fun maxUnavailableFixed(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxUnavailableFixed = mapped
}
/**
* @param value , The maximum number of instances(calculated as percentage) that can be unavailable during the update process. Conflicts with `max_unavailable_fixed`. Percent value is only allowed for regional managed instance groups with size at least 10.
*/
@JvmName("popdqfcttuaruabs")
public suspend fun maxUnavailablePercent(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxUnavailablePercent = mapped
}
/**
* @param value , Minimum number of seconds to wait for after a newly created instance becomes available. This value must be from range [0, 3600]
*/
@JvmName("svfwqwesorcneafs")
public suspend fun minReadySec(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minReadySec = mapped
}
/**
* @param value Minimal action to be taken on an instance. You can specify either `REFRESH` to update without stopping instances, `RESTART` to restart existing instances or `REPLACE` to delete and create new instances from the target template. If you specify a `REFRESH`, the Updater will attempt to perform that action only. However, if the Updater determines that the minimal action you specify is not enough to perform the update, it might perform a more disruptive action.
*/
@JvmName("lhfucepqxxfeawuq")
public suspend fun minimalAction(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.minimalAction = mapped
}
/**
* @param value Most disruptive action that is allowed to be taken on an instance. You can specify either NONE to forbid any actions, REFRESH to allow actions that do not need instance restart, RESTART to allow actions that can be applied without instance replacing or REPLACE to allow all possible actions. If the Updater determines that the minimal update action needed is more disruptive than most disruptive allowed action you specify it will not perform the update at all.
*/
@JvmName("wsrovlujwdcrkqdm")
public suspend fun mostDisruptiveAllowedAction(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.mostDisruptiveAllowedAction = mapped
}
/**
* @param value , The instance replacement method for managed instance groups. Valid values are: "RECREATE", "SUBSTITUTE". If SUBSTITUTE (default), the group replaces VM instances with new instances that have randomly generated names. If RECREATE, instance names are preserved. You must also set max_unavailable_fixed or max_unavailable_percent to be greater than 0.
* - - -
*/
@JvmName("ghxjulomhfayaymn")
public suspend fun replacementMethod(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replacementMethod = mapped
}
/**
* @param value The type of update process. You can specify either `PROACTIVE` so that the instance group manager proactively executes actions in order to bring instances to their target versions or `OPPORTUNISTIC` so that no action is proactively executed but the update will be performed as part of other actions (for example, resizes or recreateInstances calls).
*/
@JvmName("krjbcqdiycbiuqsn")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): RegionInstanceGroupManagerUpdatePolicyArgs =
RegionInstanceGroupManagerUpdatePolicyArgs(
instanceRedistributionType = instanceRedistributionType,
maxSurgeFixed = maxSurgeFixed,
maxSurgePercent = maxSurgePercent,
maxUnavailableFixed = maxUnavailableFixed,
maxUnavailablePercent = maxUnavailablePercent,
minReadySec = minReadySec,
minimalAction = minimalAction ?: throw PulumiNullFieldException("minimalAction"),
mostDisruptiveAllowedAction = mostDisruptiveAllowedAction,
replacementMethod = replacementMethod,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy