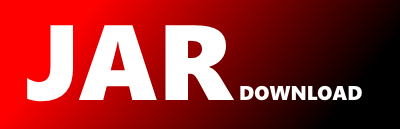
com.pulumi.gcp.compute.kotlin.inputs.RegionInstanceGroupManagerVersionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerVersionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property instanceTemplate The full URL to an instance template from which all new instances of this version will be created.
* @property name Version name.
* @property targetSize The number of instances calculated as a fixed number or a percentage depending on the settings. Structure is documented below.
* > Exactly one `version` you specify must not have a `target_size` specified. During a rolling update, the instance group manager will fulfill the `target_size`
* constraints of every other `version`, and any remaining instances will be provisioned with the version where `target_size` is unset.
*/
public data class RegionInstanceGroupManagerVersionArgs(
public val instanceTemplate: Output,
public val name: Output? = null,
public val targetSize: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerVersionArgs =
com.pulumi.gcp.compute.inputs.RegionInstanceGroupManagerVersionArgs.builder()
.instanceTemplate(instanceTemplate.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.targetSize(targetSize?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RegionInstanceGroupManagerVersionArgs].
*/
@PulumiTagMarker
public class RegionInstanceGroupManagerVersionArgsBuilder internal constructor() {
private var instanceTemplate: Output? = null
private var name: Output? = null
private var targetSize: Output? = null
/**
* @param value The full URL to an instance template from which all new instances of this version will be created.
*/
@JvmName("hdmvqxmtqyhsjbyp")
public suspend fun instanceTemplate(`value`: Output) {
this.instanceTemplate = value
}
/**
* @param value Version name.
*/
@JvmName("gevqppggaposgamm")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The number of instances calculated as a fixed number or a percentage depending on the settings. Structure is documented below.
* > Exactly one `version` you specify must not have a `target_size` specified. During a rolling update, the instance group manager will fulfill the `target_size`
* constraints of every other `version`, and any remaining instances will be provisioned with the version where `target_size` is unset.
*/
@JvmName("fyvveqtojwuwropq")
public suspend fun targetSize(`value`: Output) {
this.targetSize = value
}
/**
* @param value The full URL to an instance template from which all new instances of this version will be created.
*/
@JvmName("jebrrmdioeeyluks")
public suspend fun instanceTemplate(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.instanceTemplate = mapped
}
/**
* @param value Version name.
*/
@JvmName("hijspahlferqnfor")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The number of instances calculated as a fixed number or a percentage depending on the settings. Structure is documented below.
* > Exactly one `version` you specify must not have a `target_size` specified. During a rolling update, the instance group manager will fulfill the `target_size`
* constraints of every other `version`, and any remaining instances will be provisioned with the version where `target_size` is unset.
*/
@JvmName("omktjkylfnutvuse")
public suspend fun targetSize(`value`: RegionInstanceGroupManagerVersionTargetSizeArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.targetSize = mapped
}
/**
* @param argument The number of instances calculated as a fixed number or a percentage depending on the settings. Structure is documented below.
* > Exactly one `version` you specify must not have a `target_size` specified. During a rolling update, the instance group manager will fulfill the `target_size`
* constraints of every other `version`, and any remaining instances will be provisioned with the version where `target_size` is unset.
*/
@JvmName("xkaoydvnhohpyucl")
public suspend fun targetSize(argument: suspend RegionInstanceGroupManagerVersionTargetSizeArgsBuilder.() -> Unit) {
val toBeMapped = RegionInstanceGroupManagerVersionTargetSizeArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.targetSize = mapped
}
internal fun build(): RegionInstanceGroupManagerVersionArgs =
RegionInstanceGroupManagerVersionArgs(
instanceTemplate = instanceTemplate ?: throw PulumiNullFieldException("instanceTemplate"),
name = name,
targetSize = targetSize,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy