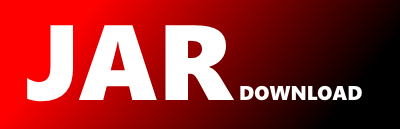
com.pulumi.gcp.compute.kotlin.inputs.RegionNetworkEndpointGroupServerlessDeploymentArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionNetworkEndpointGroupServerlessDeploymentArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property platform The platform of the NEG backend target(s). Possible values:
* API Gateway: apigateway.googleapis.com
* @property resource The user-defined name of the workload/instance. This value must be provided explicitly or in the urlMask.
* The resource identified by this value is platform-specific and is as follows: API Gateway: The gateway ID, App Engine: The service name,
* Cloud Functions: The function name, Cloud Run: The service name
* @property urlMask A template to parse platform-specific fields from a request URL. URL mask allows for routing to multiple resources
* on the same serverless platform without having to create multiple Network Endpoint Groups and backend resources.
* The fields parsed by this template are platform-specific and are as follows: API Gateway: The gateway ID,
* App Engine: The service and version, Cloud Functions: The function name, Cloud Run: The service and tag
* @property version The optional resource version. The version identified by this value is platform-specific and is follows:
* API Gateway: Unused, App Engine: The service version, Cloud Functions: Unused, Cloud Run: The service tag
*/
public data class RegionNetworkEndpointGroupServerlessDeploymentArgs(
public val platform: Output,
public val resource: Output? = null,
public val urlMask: Output? = null,
public val version: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionNetworkEndpointGroupServerlessDeploymentArgs =
com.pulumi.gcp.compute.inputs.RegionNetworkEndpointGroupServerlessDeploymentArgs.builder()
.platform(platform.applyValue({ args0 -> args0 }))
.resource(resource?.applyValue({ args0 -> args0 }))
.urlMask(urlMask?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionNetworkEndpointGroupServerlessDeploymentArgs].
*/
@PulumiTagMarker
public class RegionNetworkEndpointGroupServerlessDeploymentArgsBuilder internal constructor() {
private var platform: Output? = null
private var resource: Output? = null
private var urlMask: Output? = null
private var version: Output? = null
/**
* @param value The platform of the NEG backend target(s). Possible values:
* API Gateway: apigateway.googleapis.com
*/
@JvmName("ftlldmeuyitjvrhe")
public suspend fun platform(`value`: Output) {
this.platform = value
}
/**
* @param value The user-defined name of the workload/instance. This value must be provided explicitly or in the urlMask.
* The resource identified by this value is platform-specific and is as follows: API Gateway: The gateway ID, App Engine: The service name,
* Cloud Functions: The function name, Cloud Run: The service name
*/
@JvmName("pbwwigqrpeguveko")
public suspend fun resource(`value`: Output) {
this.resource = value
}
/**
* @param value A template to parse platform-specific fields from a request URL. URL mask allows for routing to multiple resources
* on the same serverless platform without having to create multiple Network Endpoint Groups and backend resources.
* The fields parsed by this template are platform-specific and are as follows: API Gateway: The gateway ID,
* App Engine: The service and version, Cloud Functions: The function name, Cloud Run: The service and tag
*/
@JvmName("tqrclnbbitkagocr")
public suspend fun urlMask(`value`: Output) {
this.urlMask = value
}
/**
* @param value The optional resource version. The version identified by this value is platform-specific and is follows:
* API Gateway: Unused, App Engine: The service version, Cloud Functions: Unused, Cloud Run: The service tag
*/
@JvmName("ktrroatycwltohar")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value The platform of the NEG backend target(s). Possible values:
* API Gateway: apigateway.googleapis.com
*/
@JvmName("hwytgagwlrponvlh")
public suspend fun platform(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.platform = mapped
}
/**
* @param value The user-defined name of the workload/instance. This value must be provided explicitly or in the urlMask.
* The resource identified by this value is platform-specific and is as follows: API Gateway: The gateway ID, App Engine: The service name,
* Cloud Functions: The function name, Cloud Run: The service name
*/
@JvmName("jppqcmyulcpytysu")
public suspend fun resource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resource = mapped
}
/**
* @param value A template to parse platform-specific fields from a request URL. URL mask allows for routing to multiple resources
* on the same serverless platform without having to create multiple Network Endpoint Groups and backend resources.
* The fields parsed by this template are platform-specific and are as follows: API Gateway: The gateway ID,
* App Engine: The service and version, Cloud Functions: The function name, Cloud Run: The service and tag
*/
@JvmName("cyinhnntsvqlyurg")
public suspend fun urlMask(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.urlMask = mapped
}
/**
* @param value The optional resource version. The version identified by this value is platform-specific and is follows:
* API Gateway: Unused, App Engine: The service version, Cloud Functions: Unused, Cloud Run: The service tag
*/
@JvmName("vmlyrktpkohnojta")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): RegionNetworkEndpointGroupServerlessDeploymentArgs =
RegionNetworkEndpointGroupServerlessDeploymentArgs(
platform = platform ?: throw PulumiNullFieldException("platform"),
resource = resource,
urlMask = urlMask,
version = version,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy