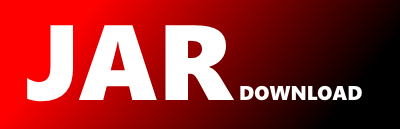
com.pulumi.gcp.compute.kotlin.inputs.RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backendService The full or partial URL to the default BackendService resource. Before forwarding the request to backendService, the load balancer applies any relevant headerActions specified as part of this backendServiceWeight.
* @property headerAction Specifies changes to request and response headers that need to take effect for the selected backendService.
* headerAction specified here take effect before headerAction in the enclosing HttpRouteRule, PathMatcher and UrlMap.
* headerAction is not supported for load balancers that have their loadBalancingScheme set to EXTERNAL.
* Not supported when the URL map is bound to a target gRPC proxy that has validateForProxyless field set to true.
* Structure is documented below.
* @property weight Specifies the fraction of traffic sent to a backend service, computed as weight / (sum of all weightedBackendService weights in routeAction) .
* The selection of a backend service is determined only for new traffic. Once a user's request has been directed to a backend service, subsequent requests are sent to the same backend service as determined by the backend service's session affinity policy.
* The value must be from 0 to 1000.
*/
public data class RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs(
public val backendService: Output? = null,
public val headerAction: Output? = null,
public val weight: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs =
com.pulumi.gcp.compute.inputs.RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs.builder()
.backendService(backendService?.applyValue({ args0 -> args0 }))
.headerAction(headerAction?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.weight(weight?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs].
*/
@PulumiTagMarker
public class RegionUrlMapDefaultRouteActionWeightedBackendServiceArgsBuilder internal constructor() {
private var backendService: Output? = null
private var headerAction:
Output? = null
private var weight: Output? = null
/**
* @param value The full or partial URL to the default BackendService resource. Before forwarding the request to backendService, the load balancer applies any relevant headerActions specified as part of this backendServiceWeight.
*/
@JvmName("resocwdnmpufxbmc")
public suspend fun backendService(`value`: Output) {
this.backendService = value
}
/**
* @param value Specifies changes to request and response headers that need to take effect for the selected backendService.
* headerAction specified here take effect before headerAction in the enclosing HttpRouteRule, PathMatcher and UrlMap.
* headerAction is not supported for load balancers that have their loadBalancingScheme set to EXTERNAL.
* Not supported when the URL map is bound to a target gRPC proxy that has validateForProxyless field set to true.
* Structure is documented below.
*/
@JvmName("phqredybuclxqnja")
public suspend fun headerAction(`value`: Output) {
this.headerAction = value
}
/**
* @param value Specifies the fraction of traffic sent to a backend service, computed as weight / (sum of all weightedBackendService weights in routeAction) .
* The selection of a backend service is determined only for new traffic. Once a user's request has been directed to a backend service, subsequent requests are sent to the same backend service as determined by the backend service's session affinity policy.
* The value must be from 0 to 1000.
*/
@JvmName("yxiqmcmrxvmbqcxo")
public suspend fun weight(`value`: Output) {
this.weight = value
}
/**
* @param value The full or partial URL to the default BackendService resource. Before forwarding the request to backendService, the load balancer applies any relevant headerActions specified as part of this backendServiceWeight.
*/
@JvmName("gydyqnkwakfdmrkm")
public suspend fun backendService(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backendService = mapped
}
/**
* @param value Specifies changes to request and response headers that need to take effect for the selected backendService.
* headerAction specified here take effect before headerAction in the enclosing HttpRouteRule, PathMatcher and UrlMap.
* headerAction is not supported for load balancers that have their loadBalancingScheme set to EXTERNAL.
* Not supported when the URL map is bound to a target gRPC proxy that has validateForProxyless field set to true.
* Structure is documented below.
*/
@JvmName("bxoubktjtchitykh")
public suspend fun headerAction(`value`: RegionUrlMapDefaultRouteActionWeightedBackendServiceHeaderActionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.headerAction = mapped
}
/**
* @param argument Specifies changes to request and response headers that need to take effect for the selected backendService.
* headerAction specified here take effect before headerAction in the enclosing HttpRouteRule, PathMatcher and UrlMap.
* headerAction is not supported for load balancers that have their loadBalancingScheme set to EXTERNAL.
* Not supported when the URL map is bound to a target gRPC proxy that has validateForProxyless field set to true.
* Structure is documented below.
*/
@JvmName("dexjohamgpywurdx")
public suspend fun headerAction(argument: suspend RegionUrlMapDefaultRouteActionWeightedBackendServiceHeaderActionArgsBuilder.() -> Unit) {
val toBeMapped =
RegionUrlMapDefaultRouteActionWeightedBackendServiceHeaderActionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.headerAction = mapped
}
/**
* @param value Specifies the fraction of traffic sent to a backend service, computed as weight / (sum of all weightedBackendService weights in routeAction) .
* The selection of a backend service is determined only for new traffic. Once a user's request has been directed to a backend service, subsequent requests are sent to the same backend service as determined by the backend service's session affinity policy.
* The value must be from 0 to 1000.
*/
@JvmName("vtbcgehbjrlqyejf")
public suspend fun weight(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.weight = mapped
}
internal fun build(): RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs =
RegionUrlMapDefaultRouteActionWeightedBackendServiceArgs(
backendService = backendService,
headerAction = headerAction,
weight = weight,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy