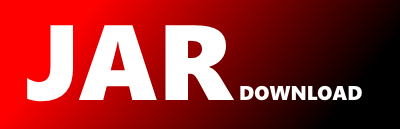
com.pulumi.gcp.compute.kotlin.inputs.ResourcePolicySnapshotSchedulePolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property retentionPolicy Retention policy applied to snapshots created by this resource policy.
* Structure is documented below.
* @property schedule Contains one of an `hourlySchedule`, `dailySchedule`, or `weeklySchedule`.
* Structure is documented below.
* @property snapshotProperties Properties with which the snapshots are created, such as labels.
* Structure is documented below.
*/
public data class ResourcePolicySnapshotSchedulePolicyArgs(
public val retentionPolicy: Output? =
null,
public val schedule: Output,
public val snapshotProperties: Output? =
null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyArgs =
com.pulumi.gcp.compute.inputs.ResourcePolicySnapshotSchedulePolicyArgs.builder()
.retentionPolicy(retentionPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.schedule(schedule.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.snapshotProperties(
snapshotProperties?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ResourcePolicySnapshotSchedulePolicyArgs].
*/
@PulumiTagMarker
public class ResourcePolicySnapshotSchedulePolicyArgsBuilder internal constructor() {
private var retentionPolicy: Output? =
null
private var schedule: Output? = null
private var snapshotProperties:
Output? = null
/**
* @param value Retention policy applied to snapshots created by this resource policy.
* Structure is documented below.
*/
@JvmName("dwiscxlrgbkcnftq")
public suspend fun retentionPolicy(`value`: Output) {
this.retentionPolicy = value
}
/**
* @param value Contains one of an `hourlySchedule`, `dailySchedule`, or `weeklySchedule`.
* Structure is documented below.
*/
@JvmName("esogruimxfjphykp")
public suspend fun schedule(`value`: Output) {
this.schedule = value
}
/**
* @param value Properties with which the snapshots are created, such as labels.
* Structure is documented below.
*/
@JvmName("kgymjnrvlqumllra")
public suspend fun snapshotProperties(`value`: Output) {
this.snapshotProperties = value
}
/**
* @param value Retention policy applied to snapshots created by this resource policy.
* Structure is documented below.
*/
@JvmName("hkyhgkfuoytfitnq")
public suspend fun retentionPolicy(`value`: ResourcePolicySnapshotSchedulePolicyRetentionPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionPolicy = mapped
}
/**
* @param argument Retention policy applied to snapshots created by this resource policy.
* Structure is documented below.
*/
@JvmName("hltwfgrisuxncvtf")
public suspend fun retentionPolicy(argument: suspend ResourcePolicySnapshotSchedulePolicyRetentionPolicyArgsBuilder.() -> Unit) {
val toBeMapped = ResourcePolicySnapshotSchedulePolicyRetentionPolicyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.retentionPolicy = mapped
}
/**
* @param value Contains one of an `hourlySchedule`, `dailySchedule`, or `weeklySchedule`.
* Structure is documented below.
*/
@JvmName("ldmjksiwbfpsmkmr")
public suspend fun schedule(`value`: ResourcePolicySnapshotSchedulePolicyScheduleArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.schedule = mapped
}
/**
* @param argument Contains one of an `hourlySchedule`, `dailySchedule`, or `weeklySchedule`.
* Structure is documented below.
*/
@JvmName("ftkuflwgvhonbjhf")
public suspend fun schedule(argument: suspend ResourcePolicySnapshotSchedulePolicyScheduleArgsBuilder.() -> Unit) {
val toBeMapped = ResourcePolicySnapshotSchedulePolicyScheduleArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.schedule = mapped
}
/**
* @param value Properties with which the snapshots are created, such as labels.
* Structure is documented below.
*/
@JvmName("siapeqehulwwuoxo")
public suspend fun snapshotProperties(`value`: ResourcePolicySnapshotSchedulePolicySnapshotPropertiesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.snapshotProperties = mapped
}
/**
* @param argument Properties with which the snapshots are created, such as labels.
* Structure is documented below.
*/
@JvmName("mxbhlgpjkcaaelum")
public suspend fun snapshotProperties(argument: suspend ResourcePolicySnapshotSchedulePolicySnapshotPropertiesArgsBuilder.() -> Unit) {
val toBeMapped =
ResourcePolicySnapshotSchedulePolicySnapshotPropertiesArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.snapshotProperties = mapped
}
internal fun build(): ResourcePolicySnapshotSchedulePolicyArgs =
ResourcePolicySnapshotSchedulePolicyArgs(
retentionPolicy = retentionPolicy,
schedule = schedule ?: throw PulumiNullFieldException("schedule"),
snapshotProperties = snapshotProperties,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy