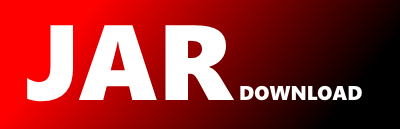
com.pulumi.gcp.compute.kotlin.inputs.SubnetworkLogConfigArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.SubnetworkLogConfigArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property aggregationInterval Can only be specified if VPC flow logging for this subnetwork is enabled.
* Toggles the aggregation interval for collecting flow logs. Increasing the
* interval time will reduce the amount of generated flow logs for long
* lasting connections. Default is an interval of 5 seconds per connection.
* Default value is `INTERVAL_5_SEC`.
* Possible values are: `INTERVAL_5_SEC`, `INTERVAL_30_SEC`, `INTERVAL_1_MIN`, `INTERVAL_5_MIN`, `INTERVAL_10_MIN`, `INTERVAL_15_MIN`.
* @property filterExpr Export filter used to define which VPC flow logs should be logged, as as CEL expression. See
* https://cloud.google.com/vpc/docs/flow-logs#filtering for details on how to format this field.
* The default value is 'true', which evaluates to include everything.
* @property flowSampling Can only be specified if VPC flow logging for this subnetwork is enabled.
* The value of the field must be in [0, 1]. Set the sampling rate of VPC
* flow logs within the subnetwork where 1.0 means all collected logs are
* reported and 0.0 means no logs are reported. Default is 0.5 which means
* half of all collected logs are reported.
* @property metadata Can only be specified if VPC flow logging for this subnetwork is enabled.
* Configures whether metadata fields should be added to the reported VPC
* flow logs.
* Default value is `INCLUDE_ALL_METADATA`.
* Possible values are: `EXCLUDE_ALL_METADATA`, `INCLUDE_ALL_METADATA`, `CUSTOM_METADATA`.
* @property metadataFields List of metadata fields that should be added to reported logs.
* Can only be specified if VPC flow logs for this subnetwork is enabled and "metadata" is set to CUSTOM_METADATA.
*/
public data class SubnetworkLogConfigArgs(
public val aggregationInterval: Output? = null,
public val filterExpr: Output? = null,
public val flowSampling: Output? = null,
public val metadata: Output? = null,
public val metadataFields: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.SubnetworkLogConfigArgs =
com.pulumi.gcp.compute.inputs.SubnetworkLogConfigArgs.builder()
.aggregationInterval(aggregationInterval?.applyValue({ args0 -> args0 }))
.filterExpr(filterExpr?.applyValue({ args0 -> args0 }))
.flowSampling(flowSampling?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0 }))
.metadataFields(metadataFields?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [SubnetworkLogConfigArgs].
*/
@PulumiTagMarker
public class SubnetworkLogConfigArgsBuilder internal constructor() {
private var aggregationInterval: Output? = null
private var filterExpr: Output? = null
private var flowSampling: Output? = null
private var metadata: Output? = null
private var metadataFields: Output>? = null
/**
* @param value Can only be specified if VPC flow logging for this subnetwork is enabled.
* Toggles the aggregation interval for collecting flow logs. Increasing the
* interval time will reduce the amount of generated flow logs for long
* lasting connections. Default is an interval of 5 seconds per connection.
* Default value is `INTERVAL_5_SEC`.
* Possible values are: `INTERVAL_5_SEC`, `INTERVAL_30_SEC`, `INTERVAL_1_MIN`, `INTERVAL_5_MIN`, `INTERVAL_10_MIN`, `INTERVAL_15_MIN`.
*/
@JvmName("pmgdlvqmedkmuloj")
public suspend fun aggregationInterval(`value`: Output) {
this.aggregationInterval = value
}
/**
* @param value Export filter used to define which VPC flow logs should be logged, as as CEL expression. See
* https://cloud.google.com/vpc/docs/flow-logs#filtering for details on how to format this field.
* The default value is 'true', which evaluates to include everything.
*/
@JvmName("eyjcqgbebihrfemv")
public suspend fun filterExpr(`value`: Output) {
this.filterExpr = value
}
/**
* @param value Can only be specified if VPC flow logging for this subnetwork is enabled.
* The value of the field must be in [0, 1]. Set the sampling rate of VPC
* flow logs within the subnetwork where 1.0 means all collected logs are
* reported and 0.0 means no logs are reported. Default is 0.5 which means
* half of all collected logs are reported.
*/
@JvmName("akdefuyjwwatoxkc")
public suspend fun flowSampling(`value`: Output) {
this.flowSampling = value
}
/**
* @param value Can only be specified if VPC flow logging for this subnetwork is enabled.
* Configures whether metadata fields should be added to the reported VPC
* flow logs.
* Default value is `INCLUDE_ALL_METADATA`.
* Possible values are: `EXCLUDE_ALL_METADATA`, `INCLUDE_ALL_METADATA`, `CUSTOM_METADATA`.
*/
@JvmName("yjstrrfsrlpvtcvj")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value List of metadata fields that should be added to reported logs.
* Can only be specified if VPC flow logs for this subnetwork is enabled and "metadata" is set to CUSTOM_METADATA.
*/
@JvmName("hedfpknhucprfiao")
public suspend fun metadataFields(`value`: Output>) {
this.metadataFields = value
}
@JvmName("afvximhddvxbvswu")
public suspend fun metadataFields(vararg values: Output) {
this.metadataFields = Output.all(values.asList())
}
/**
* @param values List of metadata fields that should be added to reported logs.
* Can only be specified if VPC flow logs for this subnetwork is enabled and "metadata" is set to CUSTOM_METADATA.
*/
@JvmName("qllkecmjejryqdxa")
public suspend fun metadataFields(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy