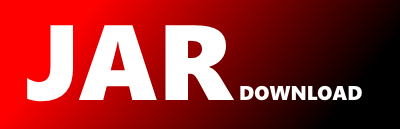
com.pulumi.gcp.compute.kotlin.inputs.SubnetworkSecondaryIpRangeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.SubnetworkSecondaryIpRangeArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property ipCidrRange The range of IP addresses belonging to this subnetwork secondary
* range. Provide this property when you create the subnetwork.
* Ranges must be unique and non-overlapping with all primary and
* secondary IP ranges within a network. Only IPv4 is supported.
* @property rangeName The name associated with this subnetwork secondary range, used
* when adding an alias IP range to a VM instance. The name must
* be 1-63 characters long, and comply with RFC1035. The name
* must be unique within the subnetwork.
*/
public data class SubnetworkSecondaryIpRangeArgs(
public val ipCidrRange: Output,
public val rangeName: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.SubnetworkSecondaryIpRangeArgs =
com.pulumi.gcp.compute.inputs.SubnetworkSecondaryIpRangeArgs.builder()
.ipCidrRange(ipCidrRange.applyValue({ args0 -> args0 }))
.rangeName(rangeName.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubnetworkSecondaryIpRangeArgs].
*/
@PulumiTagMarker
public class SubnetworkSecondaryIpRangeArgsBuilder internal constructor() {
private var ipCidrRange: Output? = null
private var rangeName: Output? = null
/**
* @param value The range of IP addresses belonging to this subnetwork secondary
* range. Provide this property when you create the subnetwork.
* Ranges must be unique and non-overlapping with all primary and
* secondary IP ranges within a network. Only IPv4 is supported.
*/
@JvmName("kqpwuabgbuykaynp")
public suspend fun ipCidrRange(`value`: Output) {
this.ipCidrRange = value
}
/**
* @param value The name associated with this subnetwork secondary range, used
* when adding an alias IP range to a VM instance. The name must
* be 1-63 characters long, and comply with RFC1035. The name
* must be unique within the subnetwork.
*/
@JvmName("bqfgmdeawbbmmhxn")
public suspend fun rangeName(`value`: Output) {
this.rangeName = value
}
/**
* @param value The range of IP addresses belonging to this subnetwork secondary
* range. Provide this property when you create the subnetwork.
* Ranges must be unique and non-overlapping with all primary and
* secondary IP ranges within a network. Only IPv4 is supported.
*/
@JvmName("dsxbeysrntdoaysp")
public suspend fun ipCidrRange(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.ipCidrRange = mapped
}
/**
* @param value The name associated with this subnetwork secondary range, used
* when adding an alias IP range to a VM instance. The name must
* be 1-63 characters long, and comply with RFC1035. The name
* must be unique within the subnetwork.
*/
@JvmName("swulnmiorqmkxbhi")
public suspend fun rangeName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.rangeName = mapped
}
internal fun build(): SubnetworkSecondaryIpRangeArgs = SubnetworkSecondaryIpRangeArgs(
ipCidrRange = ipCidrRange ?: throw PulumiNullFieldException("ipCidrRange"),
rangeName = rangeName ?: throw PulumiNullFieldException("rangeName"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy