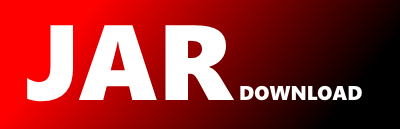
com.pulumi.gcp.compute.kotlin.inputs.URLMapPathMatcherDefaultRouteActionRetryPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.URLMapPathMatcherDefaultRouteActionRetryPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property numRetries Specifies the allowed number retries. This number must be > 0. If not specified, defaults to 1.
* @property perTryTimeout Specifies a non-zero timeout per retry attempt.
* If not specified, will use the timeout set in HttpRouteAction. If timeout in HttpRouteAction is not set,
* will use the largest timeout among all backend services associated with the route.
* Structure is documented below.
* @property retryConditions Specfies one or more conditions when this retry rule applies. Valid values are:
* * 5xx: Loadbalancer will attempt a retry if the backend service responds with any 5xx response code,
* or if the backend service does not respond at all, example: disconnects, reset, read timeout,
* * connection failure, and refused streams.
* * gateway-error: Similar to 5xx, but only applies to response codes 502, 503 or 504.
* * connect-failure: Loadbalancer will retry on failures connecting to backend services,
* for example due to connection timeouts.
* * retriable-4xx: Loadbalancer will retry for retriable 4xx response codes.
* Currently the only retriable error supported is 409.
* * refused-stream:Loadbalancer will retry if the backend service resets the stream with a REFUSED_STREAM error code.
* This reset type indicates that it is safe to retry.
* * cancelled: Loadbalancer will retry if the gRPC status code in the response header is set to cancelled
* * deadline-exceeded: Loadbalancer will retry if the gRPC status code in the response header is set to deadline-exceeded
* * resource-exhausted: Loadbalancer will retry if the gRPC status code in the response header is set to resource-exhausted
* * unavailable: Loadbalancer will retry if the gRPC status code in the response header is set to unavailable
*/
public data class URLMapPathMatcherDefaultRouteActionRetryPolicyArgs(
public val numRetries: Output? = null,
public val perTryTimeout: Output? =
null,
public val retryConditions: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.URLMapPathMatcherDefaultRouteActionRetryPolicyArgs =
com.pulumi.gcp.compute.inputs.URLMapPathMatcherDefaultRouteActionRetryPolicyArgs.builder()
.numRetries(numRetries?.applyValue({ args0 -> args0 }))
.perTryTimeout(perTryTimeout?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retryConditions(retryConditions?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [URLMapPathMatcherDefaultRouteActionRetryPolicyArgs].
*/
@PulumiTagMarker
public class URLMapPathMatcherDefaultRouteActionRetryPolicyArgsBuilder internal constructor() {
private var numRetries: Output? = null
private var perTryTimeout:
Output? = null
private var retryConditions: Output>? = null
/**
* @param value Specifies the allowed number retries. This number must be > 0. If not specified, defaults to 1.
*/
@JvmName("nvoswyqvsnuguiqt")
public suspend fun numRetries(`value`: Output) {
this.numRetries = value
}
/**
* @param value Specifies a non-zero timeout per retry attempt.
* If not specified, will use the timeout set in HttpRouteAction. If timeout in HttpRouteAction is not set,
* will use the largest timeout among all backend services associated with the route.
* Structure is documented below.
*/
@JvmName("dfybmrltsxjqyrqp")
public suspend fun perTryTimeout(`value`: Output) {
this.perTryTimeout = value
}
/**
* @param value Specfies one or more conditions when this retry rule applies. Valid values are:
* * 5xx: Loadbalancer will attempt a retry if the backend service responds with any 5xx response code,
* or if the backend service does not respond at all, example: disconnects, reset, read timeout,
* * connection failure, and refused streams.
* * gateway-error: Similar to 5xx, but only applies to response codes 502, 503 or 504.
* * connect-failure: Loadbalancer will retry on failures connecting to backend services,
* for example due to connection timeouts.
* * retriable-4xx: Loadbalancer will retry for retriable 4xx response codes.
* Currently the only retriable error supported is 409.
* * refused-stream:Loadbalancer will retry if the backend service resets the stream with a REFUSED_STREAM error code.
* This reset type indicates that it is safe to retry.
* * cancelled: Loadbalancer will retry if the gRPC status code in the response header is set to cancelled
* * deadline-exceeded: Loadbalancer will retry if the gRPC status code in the response header is set to deadline-exceeded
* * resource-exhausted: Loadbalancer will retry if the gRPC status code in the response header is set to resource-exhausted
* * unavailable: Loadbalancer will retry if the gRPC status code in the response header is set to unavailable
*/
@JvmName("bjbnbdqfkwfrmkkt")
public suspend fun retryConditions(`value`: Output>) {
this.retryConditions = value
}
@JvmName("wlcjscwlowcybvgf")
public suspend fun retryConditions(vararg values: Output) {
this.retryConditions = Output.all(values.asList())
}
/**
* @param values Specfies one or more conditions when this retry rule applies. Valid values are:
* * 5xx: Loadbalancer will attempt a retry if the backend service responds with any 5xx response code,
* or if the backend service does not respond at all, example: disconnects, reset, read timeout,
* * connection failure, and refused streams.
* * gateway-error: Similar to 5xx, but only applies to response codes 502, 503 or 504.
* * connect-failure: Loadbalancer will retry on failures connecting to backend services,
* for example due to connection timeouts.
* * retriable-4xx: Loadbalancer will retry for retriable 4xx response codes.
* Currently the only retriable error supported is 409.
* * refused-stream:Loadbalancer will retry if the backend service resets the stream with a REFUSED_STREAM error code.
* This reset type indicates that it is safe to retry.
* * cancelled: Loadbalancer will retry if the gRPC status code in the response header is set to cancelled
* * deadline-exceeded: Loadbalancer will retry if the gRPC status code in the response header is set to deadline-exceeded
* * resource-exhausted: Loadbalancer will retry if the gRPC status code in the response header is set to resource-exhausted
* * unavailable: Loadbalancer will retry if the gRPC status code in the response header is set to unavailable
*/
@JvmName("gnoyjdjhqehjjukf")
public suspend fun retryConditions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy