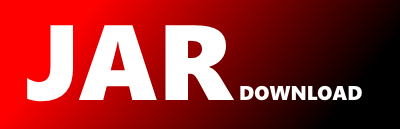
com.pulumi.gcp.compute.kotlin.inputs.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.gcp.compute.inputs.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property hostRewrite Prior to forwarding the request to the selected service, the request's host header is replaced
* with contents of hostRewrite.
* The value must be between 1 and 255 characters.
* @property pathPrefixRewrite Prior to forwarding the request to the selected backend service, the matching portion of the
* request's path is replaced by pathPrefixRewrite.
* The value must be between 1 and 1024 characters.
* @property pathTemplateRewrite Prior to forwarding the request to the selected origin, if the
* request matched a pathTemplateMatch, the matching portion of the
* request's path is replaced re-written using the pattern specified
* by pathTemplateRewrite.
* pathTemplateRewrite must be between 1 and 255 characters
* (inclusive), must start with a '/', and must only use variables
* captured by the route's pathTemplate matchers.
* pathTemplateRewrite may only be used when all of a route's
* MatchRules specify pathTemplate.
* Only one of pathPrefixRewrite and pathTemplateRewrite may be
* specified.
*/
public data class URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs(
public val hostRewrite: Output? = null,
public val pathPrefixRewrite: Output? = null,
public val pathTemplateRewrite: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.gcp.compute.inputs.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs =
com.pulumi.gcp.compute.inputs.URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs.builder()
.hostRewrite(hostRewrite?.applyValue({ args0 -> args0 }))
.pathPrefixRewrite(pathPrefixRewrite?.applyValue({ args0 -> args0 }))
.pathTemplateRewrite(pathTemplateRewrite?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs].
*/
@PulumiTagMarker
public class URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgsBuilder internal constructor() {
private var hostRewrite: Output? = null
private var pathPrefixRewrite: Output? = null
private var pathTemplateRewrite: Output? = null
/**
* @param value Prior to forwarding the request to the selected service, the request's host header is replaced
* with contents of hostRewrite.
* The value must be between 1 and 255 characters.
*/
@JvmName("raenqiuihfaapqrq")
public suspend fun hostRewrite(`value`: Output) {
this.hostRewrite = value
}
/**
* @param value Prior to forwarding the request to the selected backend service, the matching portion of the
* request's path is replaced by pathPrefixRewrite.
* The value must be between 1 and 1024 characters.
*/
@JvmName("ppjasgqyylivvnqr")
public suspend fun pathPrefixRewrite(`value`: Output) {
this.pathPrefixRewrite = value
}
/**
* @param value Prior to forwarding the request to the selected origin, if the
* request matched a pathTemplateMatch, the matching portion of the
* request's path is replaced re-written using the pattern specified
* by pathTemplateRewrite.
* pathTemplateRewrite must be between 1 and 255 characters
* (inclusive), must start with a '/', and must only use variables
* captured by the route's pathTemplate matchers.
* pathTemplateRewrite may only be used when all of a route's
* MatchRules specify pathTemplate.
* Only one of pathPrefixRewrite and pathTemplateRewrite may be
* specified.
*/
@JvmName("rwxrnjnnoaqtaiwm")
public suspend fun pathTemplateRewrite(`value`: Output) {
this.pathTemplateRewrite = value
}
/**
* @param value Prior to forwarding the request to the selected service, the request's host header is replaced
* with contents of hostRewrite.
* The value must be between 1 and 255 characters.
*/
@JvmName("bpesumtryxjklifg")
public suspend fun hostRewrite(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.hostRewrite = mapped
}
/**
* @param value Prior to forwarding the request to the selected backend service, the matching portion of the
* request's path is replaced by pathPrefixRewrite.
* The value must be between 1 and 1024 characters.
*/
@JvmName("hthpyydhnofejmva")
public suspend fun pathPrefixRewrite(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pathPrefixRewrite = mapped
}
/**
* @param value Prior to forwarding the request to the selected origin, if the
* request matched a pathTemplateMatch, the matching portion of the
* request's path is replaced re-written using the pattern specified
* by pathTemplateRewrite.
* pathTemplateRewrite must be between 1 and 255 characters
* (inclusive), must start with a '/', and must only use variables
* captured by the route's pathTemplate matchers.
* pathTemplateRewrite may only be used when all of a route's
* MatchRules specify pathTemplate.
* Only one of pathPrefixRewrite and pathTemplateRewrite may be
* specified.
*/
@JvmName("tsgydlwwtvnrvvhg")
public suspend fun pathTemplateRewrite(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pathTemplateRewrite = mapped
}
internal fun build(): URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs =
URLMapPathMatcherRouteRuleRouteActionUrlRewriteArgs(
hostRewrite = hostRewrite,
pathPrefixRewrite = pathPrefixRewrite,
pathTemplateRewrite = pathTemplateRewrite,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy