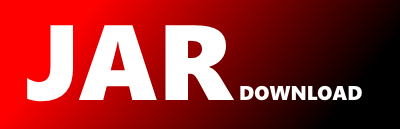
com.pulumi.gcp.compute.kotlin.outputs.AutoscalerAutoscalingPolicyMetric.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Double
import kotlin.String
import kotlin.Suppress
/**
*
* @property filter A filter string to be used as the filter string for
* a Stackdriver Monitoring TimeSeries.list API call.
* This filter is used to select a specific TimeSeries for
* the purpose of autoscaling and to determine whether the metric
* is exporting per-instance or per-group data.
* You can only use the AND operator for joining selectors.
* You can only use direct equality comparison operator (=) without
* any functions for each selector.
* You can specify the metric in both the filter string and in the
* metric field. However, if specified in both places, the metric must
* be identical.
* The monitored resource type determines what kind of values are
* expected for the metric. If it is a gce_instance, the autoscaler
* expects the metric to include a separate TimeSeries for each
* instance in a group. In such a case, you cannot filter on resource
* labels.
* If the resource type is any other value, the autoscaler expects
* this metric to contain values that apply to the entire autoscaled
* instance group and resource label filtering can be performed to
* point autoscaler at the correct TimeSeries to scale upon.
* This is called a per-group metric for the purpose of autoscaling.
* If not specified, the type defaults to gce_instance.
* You should provide a filter that is selective enough to pick just
* one TimeSeries for the autoscaled group or for each of the instances
* (if you are using gce_instance resource type). If multiple
* TimeSeries are returned upon the query execution, the autoscaler
* will sum their respective values to obtain its scaling value.
* @property name The identifier (type) of the Stackdriver Monitoring metric.
* The metric cannot have negative values.
* The metric must have a value type of INT64 or DOUBLE.
* @property singleInstanceAssignment If scaling is based on a per-group metric value that represents the
* total amount of work to be done or resource usage, set this value to
* an amount assigned for a single instance of the scaled group.
* The autoscaler will keep the number of instances proportional to the
* value of this metric, the metric itself should not change value due
* to group resizing.
* For example, a good metric to use with the target is
* `pubsub.googleapis.com/subscription/num_undelivered_messages`
* or a custom metric exporting the total number of requests coming to
* your instances.
* A bad example would be a metric exporting an average or median
* latency, since this value can't include a chunk assignable to a
* single instance, it could be better used with utilization_target
* instead.
* @property target The target value of the metric that autoscaler should
* maintain. This must be a positive value. A utilization
* metric scales number of virtual machines handling requests
* to increase or decrease proportionally to the metric.
* For example, a good metric to use as a utilizationTarget is
* www.googleapis.com/compute/instance/network/received_bytes_count.
* The autoscaler will work to keep this value constant for each
* of the instances.
* @property type Defines how target utilization value is expressed for a
* Stackdriver Monitoring metric.
* Possible values are: `GAUGE`, `DELTA_PER_SECOND`, `DELTA_PER_MINUTE`.
*/
public data class AutoscalerAutoscalingPolicyMetric(
public val filter: String? = null,
public val name: String,
public val singleInstanceAssignment: Double? = null,
public val target: Double? = null,
public val type: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.AutoscalerAutoscalingPolicyMetric): AutoscalerAutoscalingPolicyMetric = AutoscalerAutoscalingPolicyMetric(
filter = javaType.filter().map({ args0 -> args0 }).orElse(null),
name = javaType.name(),
singleInstanceAssignment = javaType.singleInstanceAssignment().map({ args0 -> args0 }).orElse(null),
target = javaType.target().map({ args0 -> args0 }).orElse(null),
type = javaType.type().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy