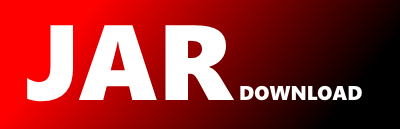
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceBackend.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Double
import kotlin.Int
import kotlin.String
import kotlin.Suppress
/**
*
* @property balancingMode Specifies the balancing mode for this backend.
* For global HTTP(S) or TCP/SSL load balancing, the default is
* UTILIZATION. Valid values are UTILIZATION, RATE (for HTTP(S))
* and CONNECTION (for TCP/SSL).
* See the [Backend Services Overview](https://cloud.google.com/load-balancing/docs/backend-service#balancing-mode)
* for an explanation of load balancing modes. Default value: "UTILIZATION" Possible values: ["UTILIZATION", "RATE", "CONNECTION"]
* @property capacityScaler A multiplier applied to the group's maximum servicing capacity
* (based on UTILIZATION, RATE or CONNECTION).
* Default value is 1, which means the group will serve up to 100%
* of its configured capacity (depending on balancingMode). A
* setting of 0 means the group is completely drained, offering
* 0% of its available Capacity. Valid range is [0.0,1.0].
* @property description Textual description for the Backend Service.
* @property group The fully-qualified URL of an Instance Group or Network Endpoint
* Group resource. In case of instance group this defines the list
* of instances that serve traffic. Member virtual machine
* instances from each instance group must live in the same zone as
* the instance group itself. No two backends in a backend service
* are allowed to use same Instance Group resource.
* For Network Endpoint Groups this defines list of endpoints. All
* endpoints of Network Endpoint Group must be hosted on instances
* located in the same zone as the Network Endpoint Group.
* Backend services cannot mix Instance Group and
* Network Endpoint Group backends.
* Note that you must specify an Instance Group or Network Endpoint
* Group resource using the fully-qualified URL, rather than a
* partial URL.
* @property maxConnections The max number of simultaneous connections for the group. Can
* be used with either CONNECTION or UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or one
* of maxConnectionsPerInstance or maxConnectionsPerEndpoint,
* as appropriate for group type, must be set.
* @property maxConnectionsPerEndpoint The max number of simultaneous connections that a single backend
* network endpoint can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either
* maxConnections or maxConnectionsPerEndpoint must be set.
* @property maxConnectionsPerInstance The max number of simultaneous connections that a single
* backend instance can handle. This is used to calculate the
* capacity of the group. Can be used in either CONNECTION or
* UTILIZATION balancing modes.
* For CONNECTION mode, either maxConnections or
* maxConnectionsPerInstance must be set.
* @property maxRate The max requests per second (RPS) of the group.
* Can be used with either RATE or UTILIZATION balancing modes,
* but required if RATE mode. For RATE mode, either maxRate or one
* of maxRatePerInstance or maxRatePerEndpoint, as appropriate for
* group type, must be set.
* @property maxRatePerEndpoint The max requests per second (RPS) that a single backend network
* endpoint can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerEndpoint must be set.
* @property maxRatePerInstance The max requests per second (RPS) that a single backend
* instance can handle. This is used to calculate the capacity of
* the group. Can be used in either balancing mode. For RATE mode,
* either maxRate or maxRatePerInstance must be set.
* @property maxUtilization Used when balancingMode is UTILIZATION. This ratio defines the
* CPU utilization target for the group. Valid range is [0.0, 1.0].
*/
public data class GetBackendServiceBackend(
public val balancingMode: String,
public val capacityScaler: Double,
public val description: String,
public val group: String,
public val maxConnections: Int,
public val maxConnectionsPerEndpoint: Int,
public val maxConnectionsPerInstance: Int,
public val maxRate: Int,
public val maxRatePerEndpoint: Double,
public val maxRatePerInstance: Double,
public val maxUtilization: Double,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetBackendServiceBackend): GetBackendServiceBackend = GetBackendServiceBackend(
balancingMode = javaType.balancingMode(),
capacityScaler = javaType.capacityScaler(),
description = javaType.description(),
group = javaType.group(),
maxConnections = javaType.maxConnections(),
maxConnectionsPerEndpoint = javaType.maxConnectionsPerEndpoint(),
maxConnectionsPerInstance = javaType.maxConnectionsPerInstance(),
maxRate = javaType.maxRate(),
maxRatePerEndpoint = javaType.maxRatePerEndpoint(),
maxRatePerInstance = javaType.maxRatePerInstance(),
maxUtilization = javaType.maxUtilization(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy