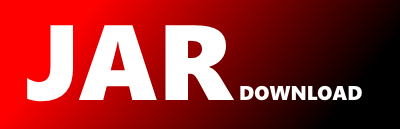
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getBackendService.
* @property affinityCookieTtlSec
* @property backends The set of backends that serve this Backend Service.
* @property cdnPolicies
* @property circuitBreakers
* @property compressionMode
* @property connectionDrainingTimeoutSec Time for which instance will be drained (not accept new connections, but still work to finish started ones).
* @property consistentHash
* @property creationTimestamp
* @property customRequestHeaders
* @property customResponseHeaders
* @property description Textual description for the Backend Service.
* @property edgeSecurityPolicy
* @property enableCdn Whether or not Cloud CDN is enabled on the Backend Service.
* @property fingerprint The fingerprint of the Backend Service.
* @property generatedId The unique identifier for the resource. This identifier is defined by the server.
* @property healthChecks The set of HTTP/HTTPS health checks used by the Backend Service.
* @property iaps
* @property id The provider-assigned unique ID for this managed resource.
* @property loadBalancingScheme
* @property localityLbPolicies
* @property localityLbPolicy
* @property logConfigs
* @property name
* @property outlierDetections
* @property portName The name of a service that has been added to an instance group in this backend.
* @property project
* @property protocol The protocol for incoming requests.
* @property securityPolicy
* @property securitySettings
* @property selfLink The URI of the Backend Service.
* @property sessionAffinity The Backend Service session stickiness configuration.
* @property timeoutSec The number of seconds to wait for a backend to respond to a request before considering the request failed.
*/
public data class GetBackendServiceResult(
public val affinityCookieTtlSec: Int,
public val backends: List,
public val cdnPolicies: List,
public val circuitBreakers: List,
public val compressionMode: String,
public val connectionDrainingTimeoutSec: Int,
public val consistentHash: List,
public val creationTimestamp: String,
public val customRequestHeaders: List,
public val customResponseHeaders: List,
public val description: String,
public val edgeSecurityPolicy: String,
public val enableCdn: Boolean,
public val fingerprint: String,
public val generatedId: Int,
public val healthChecks: List,
public val iaps: List,
public val id: String,
public val loadBalancingScheme: String,
public val localityLbPolicies: List,
public val localityLbPolicy: String,
public val logConfigs: List,
public val name: String,
public val outlierDetections: List,
public val portName: String,
public val project: String? = null,
public val protocol: String,
public val securityPolicy: String,
public val securitySettings: List,
public val selfLink: String,
public val sessionAffinity: String,
public val timeoutSec: Int,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetBackendServiceResult): GetBackendServiceResult = GetBackendServiceResult(
affinityCookieTtlSec = javaType.affinityCookieTtlSec(),
backends = javaType.backends().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceBackend.Companion.toKotlin(args0)
})
}),
cdnPolicies = javaType.cdnPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceCdnPolicy.Companion.toKotlin(args0)
})
}),
circuitBreakers = javaType.circuitBreakers().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceCircuitBreaker.Companion.toKotlin(args0)
})
}),
compressionMode = javaType.compressionMode(),
connectionDrainingTimeoutSec = javaType.connectionDrainingTimeoutSec(),
consistentHash = javaType.consistentHash().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceConsistentHash.Companion.toKotlin(args0)
})
}),
creationTimestamp = javaType.creationTimestamp(),
customRequestHeaders = javaType.customRequestHeaders().map({ args0 -> args0 }),
customResponseHeaders = javaType.customResponseHeaders().map({ args0 -> args0 }),
description = javaType.description(),
edgeSecurityPolicy = javaType.edgeSecurityPolicy(),
enableCdn = javaType.enableCdn(),
fingerprint = javaType.fingerprint(),
generatedId = javaType.generatedId(),
healthChecks = javaType.healthChecks().map({ args0 -> args0 }),
iaps = javaType.iaps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceIap.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
loadBalancingScheme = javaType.loadBalancingScheme(),
localityLbPolicies = javaType.localityLbPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceLocalityLbPolicy.Companion.toKotlin(args0)
})
}),
localityLbPolicy = javaType.localityLbPolicy(),
logConfigs = javaType.logConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceLogConfig.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
outlierDetections = javaType.outlierDetections().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceOutlierDetection.Companion.toKotlin(args0)
})
}),
portName = javaType.portName(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
protocol = javaType.protocol(),
securityPolicy = javaType.securityPolicy(),
securitySettings = javaType.securitySettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetBackendServiceSecuritySetting.Companion.toKotlin(args0)
})
}),
selfLink = javaType.selfLink(),
sessionAffinity = javaType.sessionAffinity(),
timeoutSec = javaType.timeoutSec(),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy