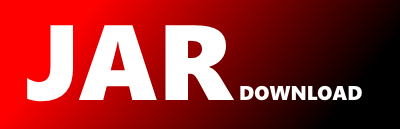
com.pulumi.gcp.compute.kotlin.outputs.GetDiskResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getDisk.
* @property asyncPrimaryDisks
* @property creationTimestamp Creation timestamp in RFC3339 text format.
* @property description The optional description of this resource.
* @property diskEncryptionKeys
* @property diskId
* @property effectiveLabels
* @property enableConfidentialCompute
* @property guestOsFeatures
* @property id The provider-assigned unique ID for this managed resource.
* @property image The image from which to initialize this disk.
* @property interface
* @property labelFingerprint The fingerprint used for optimistic locking of this resource. Used
* internally during updates.
* @property labels All of labels (key/value pairs) present on the resource in GCP, including the labels configured through Pulumi, other clients and services.
* @property lastAttachTimestamp Last attach timestamp in RFC3339 text format.
* @property lastDetachTimestamp Last detach timestamp in RFC3339 text format.
* @property licenses
* @property multiWriter
* @property name
* @property physicalBlockSizeBytes Physical block size of the persistent disk, in bytes.
* @property project
* @property provisionedIops
* @property provisionedThroughput
* @property pulumiLabels
* @property resourcePolicies
* @property selfLink The URI of the created resource.
* @property size Size of the persistent disk, specified in GB.
* @property snapshot The source snapshot used to create this disk.
* @property sourceDisk
* @property sourceDiskId
* @property sourceImageEncryptionKeys The customer-supplied encryption key of the source image.
* @property sourceImageId The ID value of the image used to create this disk. This value
* identifies the exact image that was used to create this persistent
* disk. For example, if you created the persistent disk from an image
* that was later deleted and recreated under the same name, the source
* image ID would identify the exact version of the image that was used.
* @property sourceSnapshotEncryptionKeys The customer-supplied encryption key of the source snapshot.
* @property sourceSnapshotId The unique ID of the snapshot used to create this disk. This value
* identifies the exact snapshot that was used to create this persistent
* disk. For example, if you created the persistent disk from a snapshot
* that was later deleted and recreated under the same name, the source
* snapshot ID would identify the exact version of the snapshot that was
* used.
* @property type URL of the disk type resource describing which disk type to use to
* create the disk.
* @property users Links to the users of the disk (attached instances) in form:
* project/zones/zone/instances/instance
* @property zone A reference to the zone where the disk resides.
*/
public data class GetDiskResult(
public val asyncPrimaryDisks: List,
public val creationTimestamp: String,
public val description: String,
public val diskEncryptionKeys: List,
public val diskId: String,
public val effectiveLabels: Map,
public val enableConfidentialCompute: Boolean,
public val guestOsFeatures: List,
public val id: String,
public val image: String,
public val `interface`: String,
public val labelFingerprint: String,
public val labels: Map,
public val lastAttachTimestamp: String,
public val lastDetachTimestamp: String,
public val licenses: List,
public val multiWriter: Boolean,
public val name: String,
public val physicalBlockSizeBytes: Int,
public val project: String? = null,
public val provisionedIops: Int,
public val provisionedThroughput: Int,
public val pulumiLabels: Map,
public val resourcePolicies: List,
public val selfLink: String,
public val size: Int,
public val snapshot: String,
public val sourceDisk: String,
public val sourceDiskId: String,
public val sourceImageEncryptionKeys: List,
public val sourceImageId: String,
public val sourceSnapshotEncryptionKeys: List,
public val sourceSnapshotId: String,
public val type: String,
public val users: List,
public val zone: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetDiskResult): GetDiskResult =
GetDiskResult(
asyncPrimaryDisks = javaType.asyncPrimaryDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetDiskAsyncPrimaryDisk.Companion.toKotlin(args0)
})
}),
creationTimestamp = javaType.creationTimestamp(),
description = javaType.description(),
diskEncryptionKeys = javaType.diskEncryptionKeys().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetDiskDiskEncryptionKey.Companion.toKotlin(args0)
})
}),
diskId = javaType.diskId(),
effectiveLabels = javaType.effectiveLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
enableConfidentialCompute = javaType.enableConfidentialCompute(),
guestOsFeatures = javaType.guestOsFeatures().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetDiskGuestOsFeature.Companion.toKotlin(args0)
})
}),
id = javaType.id(),
image = javaType.image(),
`interface` = javaType.interface_(),
labelFingerprint = javaType.labelFingerprint(),
labels = javaType.labels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
lastAttachTimestamp = javaType.lastAttachTimestamp(),
lastDetachTimestamp = javaType.lastDetachTimestamp(),
licenses = javaType.licenses().map({ args0 -> args0 }),
multiWriter = javaType.multiWriter(),
name = javaType.name(),
physicalBlockSizeBytes = javaType.physicalBlockSizeBytes(),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
provisionedIops = javaType.provisionedIops(),
provisionedThroughput = javaType.provisionedThroughput(),
pulumiLabels = javaType.pulumiLabels().map({ args0 -> args0.key.to(args0.value) }).toMap(),
resourcePolicies = javaType.resourcePolicies().map({ args0 -> args0 }),
selfLink = javaType.selfLink(),
size = javaType.size(),
snapshot = javaType.snapshot(),
sourceDisk = javaType.sourceDisk(),
sourceDiskId = javaType.sourceDiskId(),
sourceImageEncryptionKeys = javaType.sourceImageEncryptionKeys().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetDiskSourceImageEncryptionKey.Companion.toKotlin(args0)
})
}),
sourceImageId = javaType.sourceImageId(),
sourceSnapshotEncryptionKeys = javaType.sourceSnapshotEncryptionKeys().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetDiskSourceSnapshotEncryptionKey.Companion.toKotlin(args0)
})
}),
sourceSnapshotId = javaType.sourceSnapshotId(),
type = javaType.type(),
users = javaType.users().map({ args0 -> args0 }),
zone = javaType.zone().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy