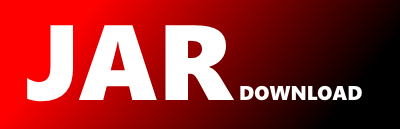
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getInstanceGroupManager.
* @property allInstancesConfigs
* @property autoHealingPolicies
* @property baseInstanceName
* @property creationTimestamp
* @property description
* @property fingerprint
* @property id The provider-assigned unique ID for this managed resource.
* @property instanceGroup
* @property instanceLifecyclePolicies
* @property listManagedInstancesResults
* @property name
* @property namedPorts
* @property operation
* @property params
* @property project
* @property selfLink
* @property statefulDisks
* @property statefulExternalIps
* @property statefulInternalIps
* @property statuses
* @property targetPools
* @property targetSize
* @property updatePolicies
* @property versions
* @property waitForInstances
* @property waitForInstancesStatus
* @property zone
*/
public data class GetInstanceGroupManagerResult(
public val allInstancesConfigs: List,
public val autoHealingPolicies: List,
public val baseInstanceName: String,
public val creationTimestamp: String,
public val description: String,
public val fingerprint: String,
public val id: String,
public val instanceGroup: String,
public val instanceLifecyclePolicies: List,
public val listManagedInstancesResults: String,
public val name: String? = null,
public val namedPorts: List,
public val operation: String,
public val params: List,
public val project: String? = null,
public val selfLink: String? = null,
public val statefulDisks: List,
public val statefulExternalIps: List,
public val statefulInternalIps: List,
public val statuses: List,
public val targetPools: List,
public val targetSize: Int,
public val updatePolicies: List,
public val versions: List,
public val waitForInstances: Boolean,
public val waitForInstancesStatus: String,
public val zone: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetInstanceGroupManagerResult): GetInstanceGroupManagerResult = GetInstanceGroupManagerResult(
allInstancesConfigs = javaType.allInstancesConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerAllInstancesConfig.Companion.toKotlin(args0)
})
}),
autoHealingPolicies = javaType.autoHealingPolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerAutoHealingPolicy.Companion.toKotlin(args0)
})
}),
baseInstanceName = javaType.baseInstanceName(),
creationTimestamp = javaType.creationTimestamp(),
description = javaType.description(),
fingerprint = javaType.fingerprint(),
id = javaType.id(),
instanceGroup = javaType.instanceGroup(),
instanceLifecyclePolicies = javaType.instanceLifecyclePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerInstanceLifecyclePolicy.Companion.toKotlin(args0)
})
}),
listManagedInstancesResults = javaType.listManagedInstancesResults(),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
namedPorts = javaType.namedPorts().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerNamedPort.Companion.toKotlin(args0)
})
}),
operation = javaType.operation(),
params = javaType.params().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerParam.Companion.toKotlin(args0)
})
}),
project = javaType.project().map({ args0 -> args0 }).orElse(null),
selfLink = javaType.selfLink().map({ args0 -> args0 }).orElse(null),
statefulDisks = javaType.statefulDisks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerStatefulDisk.Companion.toKotlin(args0)
})
}),
statefulExternalIps = javaType.statefulExternalIps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerStatefulExternalIp.Companion.toKotlin(args0)
})
}),
statefulInternalIps = javaType.statefulInternalIps().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerStatefulInternalIp.Companion.toKotlin(args0)
})
}),
statuses = javaType.statuses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerStatus.Companion.toKotlin(args0)
})
}),
targetPools = javaType.targetPools().map({ args0 -> args0 }),
targetSize = javaType.targetSize(),
updatePolicies = javaType.updatePolicies().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerUpdatePolicy.Companion.toKotlin(args0)
})
}),
versions = javaType.versions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.gcp.compute.kotlin.outputs.GetInstanceGroupManagerVersion.Companion.toKotlin(args0)
})
}),
waitForInstances = javaType.waitForInstances(),
waitForInstancesStatus = javaType.waitForInstancesStatus(),
zone = javaType.zone().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy