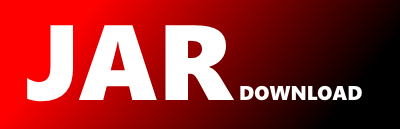
com.pulumi.gcp.compute.kotlin.outputs.GetRouterStatusBestRoutesForRouter.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-gcp-kotlin Show documentation
Show all versions of pulumi-gcp-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.gcp.compute.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property description An optional description of this resource. Provide this property
* when you create the resource.
* @property destRange The destination range of outgoing packets that this route applies to.
* Only IPv4 is supported.
* @property name The name of the router.
* @property network The network name or resource link to the parent
* network of this subnetwork.
* @property nextHopGateway URL to a gateway that should handle matching packets.
* Currently, you can only specify the internet gateway, using a full or
* partial valid URL:
* * 'https://www.googleapis.com/compute/v1/projects/project/global/gateways/default-internet-gateway'
* * 'projects/project/global/gateways/default-internet-gateway'
* * 'global/gateways/default-internet-gateway'
* * The string 'default-internet-gateway'.
* @property nextHopIlb The IP address or URL to a forwarding rule of type
* loadBalancingScheme=INTERNAL that should handle matching
* packets.
* With the GA provider you can only specify the forwarding
* rule as a partial or full URL. For example, the following
* are all valid values:
* * 10.128.0.56
* * https://www.googleapis.com/compute/v1/projects/project/regions/region/forwardingRules/forwardingRule
* * regions/region/forwardingRules/forwardingRule
* When the beta provider, you can also specify the IP address
* of a forwarding rule from the same VPC or any peered VPC.
* Note that this can only be used when the destinationRange is
* a public (non-RFC 1918) IP CIDR range.
* @property nextHopInstance URL to an instance that should handle matching packets.
* You can specify this as a full or partial URL. For example:
* * 'https://www.googleapis.com/compute/v1/projects/project/zones/zone/instances/instance'
* * 'projects/project/zones/zone/instances/instance'
* * 'zones/zone/instances/instance'
* * Just the instance name, with the zone in 'next_hop_instance_zone'.
* @property nextHopInstanceZone The zone of the instance specified in next_hop_instance. Omit if next_hop_instance is specified as a URL.
* @property nextHopIp Network IP address of an instance that should handle matching packets.
* @property nextHopNetwork URL to a Network that should handle matching packets.
* @property nextHopVpnTunnel URL to a VpnTunnel that should handle matching packets.
* @property priority The priority of this route. Priority is used to break ties in cases
* where there is more than one matching route of equal prefix length.
* In the case of two routes with equal prefix length, the one with the
* lowest-numbered priority value wins.
* Default value is 1000. Valid range is 0 through 65535.
* @property project The ID of the project in which the resource
* belongs. If it is not provided, the provider project is used.
* @property selfLink
* @property tags A list of instance tags to which this route applies.
*/
public data class GetRouterStatusBestRoutesForRouter(
public val description: String,
public val destRange: String,
public val name: String,
public val network: String,
public val nextHopGateway: String,
public val nextHopIlb: String,
public val nextHopInstance: String,
public val nextHopInstanceZone: String,
public val nextHopIp: String,
public val nextHopNetwork: String,
public val nextHopVpnTunnel: String,
public val priority: Int,
public val project: String,
public val selfLink: String,
public val tags: List,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.gcp.compute.outputs.GetRouterStatusBestRoutesForRouter): GetRouterStatusBestRoutesForRouter = GetRouterStatusBestRoutesForRouter(
description = javaType.description(),
destRange = javaType.destRange(),
name = javaType.name(),
network = javaType.network(),
nextHopGateway = javaType.nextHopGateway(),
nextHopIlb = javaType.nextHopIlb(),
nextHopInstance = javaType.nextHopInstance(),
nextHopInstanceZone = javaType.nextHopInstanceZone(),
nextHopIp = javaType.nextHopIp(),
nextHopNetwork = javaType.nextHopNetwork(),
nextHopVpnTunnel = javaType.nextHopVpnTunnel(),
priority = javaType.priority(),
project = javaType.project(),
selfLink = javaType.selfLink(),
tags = javaType.tags().map({ args0 -> args0 }),
)
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy